Baloo::FileIndexerConfig
#include <fileindexerconfig.h>
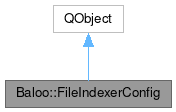
Public Slots | |
void | forceConfigUpdate () |
Public Member Functions | |
FileIndexerConfig (QObject *parent=nullptr) | |
bool | canBeSearched (const QString &folder) const |
int | databaseVersion () const |
QStringList | excludeFilters () const |
QStringList | excludeFolders () const |
QStringList | excludeMimetypes () const |
bool | folderInFolderList (const QString &path, QString &folder) const |
QStringList | includeFolders () const |
bool | indexHiddenFilesAndFolders () const |
bool | indexingEnabled () const |
uint | maxUncomittedFiles () const |
bool | onlyBasicIndexing () const |
void | setDatabaseVersion (int version) |
bool | shouldBeIndexed (const QString &path) const |
bool | shouldFileBeIndexed (const QString &fileName) const |
bool | shouldFolderBeIndexed (const QString &path) const |
bool | shouldMimeTypeBeIndexed (const QString &mimeType) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Active config class which emits signals if the config was changed, for example if the KCM saved the config file.
Definition at line 30 of file fileindexerconfig.h.
Constructor & Destructor Documentation
◆ FileIndexerConfig()
|
explicit |
Definition at line 37 of file fileindexerconfig.cpp.
◆ ~FileIndexerConfig()
|
override |
Definition at line 48 of file fileindexerconfig.cpp.
Member Function Documentation
◆ canBeSearched()
bool Baloo::FileIndexerConfig::canBeSearched | ( | const QString & | folder | ) | const |
Check if folder
can be searched.
folder
can be searched if itself or one of its descendants is indexed.
Example: if ~/foo is not indexed and ~/foo/bar is indexed then ~/foo can be searched.
- Returns
true
if thefolder
can be searched.
Definition at line 121 of file fileindexerconfig.cpp.
◆ databaseVersion()
int Baloo::FileIndexerConfig::databaseVersion | ( | ) | const |
Returns the internal version number of the Baloo database.
Definition at line 359 of file fileindexerconfig.cpp.
◆ excludeFilters()
QStringList Baloo::FileIndexerConfig::excludeFilters | ( | ) | const |
Definition at line 86 of file fileindexerconfig.cpp.
◆ excludeFolders()
QStringList Baloo::FileIndexerConfig::excludeFolders | ( | ) | const |
Folders that are excluded from indexing.
(Descendant folders of an excluded folder can be added and they will be indexed.)
- Returns
- list of paths.
Definition at line 73 of file fileindexerconfig.cpp.
◆ excludeMimetypes()
QStringList Baloo::FileIndexerConfig::excludeMimetypes | ( | ) | const |
Definition at line 106 of file fileindexerconfig.cpp.
◆ folderInFolderList()
Check if path
is in the list of folders to be indexed taking include and exclude folders into account.
folder
is set to the folder which was the reason for the decision.
Definition at line 205 of file fileindexerconfig.cpp.
◆ forceConfigUpdate
|
slot |
Reread the config from disk and update the configuration cache.
This is only required for testing as normally the config updates itself whenever the config file on disk changes.
- Returns
true
if the config has actually changed
Definition at line 347 of file fileindexerconfig.cpp.
◆ includeFolders()
QStringList Baloo::FileIndexerConfig::includeFolders | ( | ) | const |
Folders to search for files to index and analyze.
- Returns
- list of paths.
Definition at line 60 of file fileindexerconfig.cpp.
◆ indexHiddenFilesAndFolders()
bool Baloo::FileIndexerConfig::indexHiddenFilesAndFolders | ( | ) | const |
Definition at line 111 of file fileindexerconfig.cpp.
◆ indexingEnabled()
bool Baloo::FileIndexerConfig::indexingEnabled | ( | ) | const |
Definition at line 370 of file fileindexerconfig.cpp.
◆ maxUncomittedFiles()
uint Baloo::FileIndexerConfig::maxUncomittedFiles | ( | ) | const |
Returns batch size.
Definition at line 375 of file fileindexerconfig.cpp.
◆ onlyBasicIndexing()
bool Baloo::FileIndexerConfig::onlyBasicIndexing | ( | ) | const |
Definition at line 116 of file fileindexerconfig.cpp.
◆ setDatabaseVersion()
void Baloo::FileIndexerConfig::setDatabaseVersion | ( | int | version | ) |
Definition at line 364 of file fileindexerconfig.cpp.
◆ shouldBeIndexed()
bool Baloo::FileIndexerConfig::shouldBeIndexed | ( | const QString & | path | ) | const |
Check if file or folder path
should be indexed taking into account the includeFolders(), the excludeFolders(), and the excludeFilters().
Inclusion takes precedence.
Be aware that this method does not check if parent dirs match any of the exclude filters. Only the path of the dir itself it checked.
- Returns
true
if the file or folder atpath
should be indexed according to the configuration.
Definition at line 143 of file fileindexerconfig.cpp.
◆ shouldFileBeIndexed()
bool Baloo::FileIndexerConfig::shouldFileBeIndexed | ( | const QString & | fileName | ) | const |
Check fileName
for all exclude filters.
This does not take file paths into account.
- Returns
true
if a file with namefilename
should be indexed according to the configuration.
Definition at line 192 of file fileindexerconfig.cpp.
◆ shouldFolderBeIndexed()
bool Baloo::FileIndexerConfig::shouldFolderBeIndexed | ( | const QString & | path | ) | const |
Check if the folder at path
should be indexed.
Be aware that this method does not check if parent dirs match any of the exclude filters. Only the name of the dir itself it checked.
- Returns
true
if the folder atpath
should be indexed according to the configuration.
Definition at line 155 of file fileindexerconfig.cpp.
◆ shouldMimeTypeBeIndexed()
bool Baloo::FileIndexerConfig::shouldMimeTypeBeIndexed | ( | const QString & | mimeType | ) | const |
Checks if mimeType
should be indexed.
- Returns
true
if the mimetype should be indexed according to the configuration
Definition at line 200 of file fileindexerconfig.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 12:02:21 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.