ColumnViewAttached
#include <columnview.h>
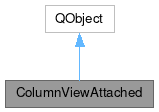
Properties | |
bool | fillWidth |
QQuickItem * | globalFooter |
QQuickItem * | globalHeader |
int | index |
bool | inViewport |
bool | pinned |
bool | preventStealing |
qreal | reservedSpace |
ColumnView * | view |
![]() | |
objectName | |
Signals | |
void | fillWidthChanged () |
void | globalFooterChanged (QQuickItem *oldFooter, QQuickItem *newFooter) |
void | globalHeaderChanged (QQuickItem *oldHeader, QQuickItem *newHeader) |
void | indexChanged () |
void | inViewportChanged () |
void | pinnedChanged () |
void | preventStealingChanged () |
void | reservedSpaceChanged () |
void | scrollIntention (ScrollIntentionEvent *event) |
void | viewChanged () |
Public Member Functions | |
ColumnViewAttached (QObject *parent=nullptr) | |
bool | fillWidth () const |
QQuickItem * | globalFooter () const |
QQuickItem * | globalHeader () const |
int | index () const |
bool | inViewport () const |
bool | isPinned () const |
QQuickItem * | originalParent () const |
bool | preventStealing () const |
qreal | reservedSpace () const |
void | setFillWidth (bool fill) |
void | setGlobalFooter (QQuickItem *footer) |
void | setGlobalHeader (QQuickItem *header) |
void | setIndex (int index) |
void | setInViewport (bool inViewport) |
void | setOriginalParent (QQuickItem *parent) |
void | setPinned (bool pinned) |
void | setPreventStealing (bool prevent) |
void | setReservedSpace (qreal space) |
void | setShouldDeleteOnRemove (bool del) |
void | setView (ColumnView *view) |
bool | shouldDeleteOnRemove () const |
ColumnView * | view () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This is an attached property to every item that is inserted in the ColumnView, used to access the view and page information such as the position and information for layouting, such as fillWidth.
- Since
- 2.7
Definition at line 38 of file columnview.h.
Property Documentation
◆ fillWidth
|
readwrite |
If true, the column will expand to take the whole viewport space minus reservedSpace.
Definition at line 50 of file columnview.h.
◆ globalFooter
|
readwrite |
Definition at line 82 of file columnview.h.
◆ globalHeader
|
readwrite |
Definition at line 81 of file columnview.h.
◆ index
|
readwrite |
The index position of the column in the view, starting from 0.
Definition at line 45 of file columnview.h.
◆ inViewport
|
read |
True if this column is at least partly visible in the ColumnView's viewport.
- Since
- 5.77
Definition at line 79 of file columnview.h.
◆ pinned
|
readwrite |
If true the page will never go out of view, but will stay either at the right or left side of the ColumnView.
Definition at line 68 of file columnview.h.
◆ preventStealing
|
readwrite |
Like the same property of MouseArea, when this is true, the column view won't try to manage events by itself when filtering from a child, not disturbing user interaction.
Definition at line 62 of file columnview.h.
◆ reservedSpace
|
readwrite |
When a column is fillWidth, it will keep reservedSpace amount of pixels from going to fill the full viewport width.
Definition at line 55 of file columnview.h.
◆ view
|
read |
The view this column belongs to.
Definition at line 73 of file columnview.h.
Constructor & Destructor Documentation
◆ ColumnViewAttached()
ColumnViewAttached::ColumnViewAttached | ( | QObject * | parent = nullptr | ) |
Definition at line 132 of file columnview.cpp.
◆ ~ColumnViewAttached()
|
override |
Definition at line 137 of file columnview.cpp.
Member Function Documentation
◆ fillWidth()
bool ColumnViewAttached::fillWidth | ( | ) | const |
Definition at line 183 of file columnview.cpp.
◆ globalFooter()
QQuickItem * ColumnViewAttached::globalFooter | ( | ) | const |
Definition at line 342 of file columnview.cpp.
◆ globalHeader()
QQuickItem * ColumnViewAttached::globalHeader | ( | ) | const |
Definition at line 317 of file columnview.cpp.
◆ index()
int ColumnViewAttached::index | ( | ) | const |
Definition at line 159 of file columnview.cpp.
◆ inViewport()
bool ColumnViewAttached::inViewport | ( | ) | const |
Definition at line 301 of file columnview.cpp.
◆ isPinned()
bool ColumnViewAttached::isPinned | ( | ) | const |
Definition at line 281 of file columnview.cpp.
◆ originalParent()
QQuickItem * ColumnViewAttached::originalParent | ( | ) | const |
Definition at line 246 of file columnview.cpp.
◆ preventStealing()
bool ColumnViewAttached::preventStealing | ( | ) | const |
Definition at line 266 of file columnview.cpp.
◆ reservedSpace()
qreal ColumnViewAttached::reservedSpace | ( | ) | const |
Definition at line 188 of file columnview.cpp.
◆ setFillWidth()
void ColumnViewAttached::setFillWidth | ( | bool | fill | ) |
Definition at line 164 of file columnview.cpp.
◆ setGlobalFooter()
void ColumnViewAttached::setGlobalFooter | ( | QQuickItem * | footer | ) |
Definition at line 347 of file columnview.cpp.
◆ setGlobalHeader()
void ColumnViewAttached::setGlobalHeader | ( | QQuickItem * | header | ) |
Definition at line 322 of file columnview.cpp.
◆ setIndex()
void ColumnViewAttached::setIndex | ( | int | index | ) |
Definition at line 141 of file columnview.cpp.
◆ setInViewport()
void ColumnViewAttached::setInViewport | ( | bool | inViewport | ) |
Definition at line 306 of file columnview.cpp.
◆ setOriginalParent()
void ColumnViewAttached::setOriginalParent | ( | QQuickItem * | parent | ) |
Definition at line 251 of file columnview.cpp.
◆ setPinned()
void ColumnViewAttached::setPinned | ( | bool | pinned | ) |
Definition at line 286 of file columnview.cpp.
◆ setPreventStealing()
void ColumnViewAttached::setPreventStealing | ( | bool | prevent | ) |
Definition at line 271 of file columnview.cpp.
◆ setReservedSpace()
void ColumnViewAttached::setReservedSpace | ( | qreal | space | ) |
Definition at line 193 of file columnview.cpp.
◆ setShouldDeleteOnRemove()
void ColumnViewAttached::setShouldDeleteOnRemove | ( | bool | del | ) |
Definition at line 261 of file columnview.cpp.
◆ setView()
void ColumnViewAttached::setView | ( | ColumnView * | view | ) |
Definition at line 217 of file columnview.cpp.
◆ shouldDeleteOnRemove()
bool ColumnViewAttached::shouldDeleteOnRemove | ( | ) | const |
Definition at line 256 of file columnview.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:13:25 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.