Kirigami::Platform::Settings
#include <settings.h>
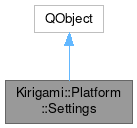
Properties | |
QVariant | applicationWindowIcon |
bool | hasPlatformMenuBar |
bool | hasTransientTouchInput |
QStringList | information |
bool | isMobile |
int | mouseWheelScrollLines |
bool | smoothScroll |
QString | style |
bool | tabletMode |
QML_SINGLETONbool | tabletModeAvailable |
![]() | |
objectName | |
Signals | |
void | hasTransientTouchInputChanged () |
void | isMobileChanged () |
void | smoothScrollChanged () |
void | tabletModeAvailableChanged () |
void | tabletModeChanged () |
Public Member Functions | |
Settings (QObject *parent=nullptr) | |
QVariant | applicationWindowIcon () const |
bool | hasPlatformMenuBar () const |
bool | hasTransientTouchInput () const |
QStringList | information () const |
bool | isMobile () const |
bool | isTabletModeAvailable () const |
int | mouseWheelScrollLines () const |
void | setIsMobile (bool mobile) |
void | setStyle (const QString &style) |
void | setTabletMode (bool tablet) |
void | setTabletModeAvailable (bool mobile) |
void | setTransientTouchInput (bool touch) |
bool | smoothScroll () const |
QString | style () const |
bool | tabletMode () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
This class contains global kirigami settings about the current device setup It is exposed to QML as the singleton "Settings".
Definition at line 23 of file settings.h.
Property Documentation
◆ applicationWindowIcon
|
read |
This property holds the name of the application window icon.
- See also
- QGuiApplication::windowIcon()
- Since
- 5.62
- org.kde.kirigami 2.10
Definition at line 101 of file settings.h.
◆ hasPlatformMenuBar
|
read |
This property holds whether the system has a platform menu bar; e.g.
a user is on macOS or has a global menu on KDE Plasma.
- Warning
- Android has a platform menu bar; which may not be what you expected.
Definition at line 60 of file settings.h.
◆ hasTransientTouchInput
|
read |
This property holds whether the user in this moment is interacting with the app with the touch screen.
Definition at line 66 of file settings.h.
◆ information
|
read |
This property holds the runtime information about the libraries in use.
- Since
- 5.52
- org.kde.kirigami 2.6
Definition at line 92 of file settings.h.
◆ isMobile
|
read |
This property holds whether the application is running on a small mobile device such as a mobile phone.
This is used when we want to do specific adaptations to the UI for small screen form factors, such as having bigger touch areas.
Definition at line 42 of file settings.h.
◆ mouseWheelScrollLines
|
read |
This property holds the number of lines of text the mouse wheel should scroll.
Definition at line 78 of file settings.h.
◆ smoothScroll
|
read |
This property holds whether to display animated transitions when scrolling with a mouse wheel or the keyboard.
Definition at line 84 of file settings.h.
◆ style
|
read |
This property holds the name of the QtQuickControls2 style the application is using, for instance org.kde.desktop, Plasma, Material, Universal etc.
Definition at line 72 of file settings.h.
◆ tabletMode
|
read |
This property holds whether the application is running on a device that is behaving like a tablet.
- Note
- This doesn't mean exactly a tablet form factor, but that the preferred input mode for the device is the touch screen and that pointer and keyboard are either secondary or not available.
Definition at line 52 of file settings.h.
◆ tabletModeAvailable
|
read |
This property holds whether the system can dynamically enter and exit tablet mode (or the device is actually a tablet).
This is the case for foldable convertibles and transformable laptops that support keyboard detachment.
Definition at line 35 of file settings.h.
Constructor & Destructor Documentation
◆ Settings()
Kirigami::Platform::Settings::Settings | ( | QObject * | parent = nullptr | ) |
Definition at line 38 of file settings.cpp.
◆ ~Settings()
|
override |
Definition at line 105 of file settings.cpp.
Member Function Documentation
◆ applicationWindowIcon()
QVariant Kirigami::Platform::Settings::applicationWindowIcon | ( | ) | const |
Definition at line 225 of file settings.cpp.
◆ eventFilter()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 109 of file settings.cpp.
◆ hasPlatformMenuBar()
bool Kirigami::Platform::Settings::hasPlatformMenuBar | ( | ) | const |
Definition at line 234 of file settings.cpp.
◆ hasTransientTouchInput()
bool Kirigami::Platform::Settings::hasTransientTouchInput | ( | ) | const |
Definition at line 190 of file settings.cpp.
◆ information()
QStringList Kirigami::Platform::Settings::information | ( | ) | const |
Definition at line 215 of file settings.cpp.
◆ isMobile()
bool Kirigami::Platform::Settings::isMobile | ( | ) | const |
Definition at line 158 of file settings.cpp.
◆ isTabletModeAvailable()
bool Kirigami::Platform::Settings::isTabletModeAvailable | ( | ) | const |
Definition at line 143 of file settings.cpp.
◆ mouseWheelScrollLines()
int Kirigami::Platform::Settings::mouseWheelScrollLines | ( | ) | const |
Definition at line 205 of file settings.cpp.
◆ setIsMobile()
void Kirigami::Platform::Settings::setIsMobile | ( | bool | mobile | ) |
Definition at line 148 of file settings.cpp.
◆ setStyle()
void Kirigami::Platform::Settings::setStyle | ( | const QString & | style | ) |
Definition at line 200 of file settings.cpp.
◆ setTabletMode()
void Kirigami::Platform::Settings::setTabletMode | ( | bool | tablet | ) |
Definition at line 163 of file settings.cpp.
◆ setTabletModeAvailable()
void Kirigami::Platform::Settings::setTabletModeAvailable | ( | bool | mobile | ) |
Definition at line 133 of file settings.cpp.
◆ setTransientTouchInput()
void Kirigami::Platform::Settings::setTransientTouchInput | ( | bool | touch | ) |
Definition at line 178 of file settings.cpp.
◆ smoothScroll()
bool Kirigami::Platform::Settings::smoothScroll | ( | ) | const |
Definition at line 210 of file settings.cpp.
◆ style()
QString Kirigami::Platform::Settings::style | ( | ) | const |
Definition at line 195 of file settings.cpp.
◆ tabletMode()
bool Kirigami::Platform::Settings::tabletMode | ( | ) | const |
Definition at line 173 of file settings.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:49:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.