ToolBarLayout
#include <toolbarlayout.h>
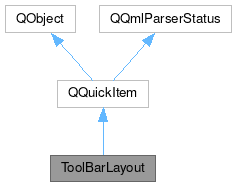
Public Types | |
using | ActionsProperty = QQmlListProperty<QObject> |
enum | HeightMode { AlwaysCenter , AlwaysFill , ConstrainIfLarger } |
![]() | |
enum | Flag |
typedef | Flags |
enum | ItemChange |
enum | TransformOrigin |
![]() | |
typedef | QObjectList |
Properties | |
QML_ELEMENTQQmlListProperty< QObject > | actions |
Qt::Alignment | alignment |
QQmlComponent * | fullDelegate |
HeightMode | heightMode |
QList< QObject * > | hiddenActions |
QQmlComponent * | iconDelegate |
Qt::LayoutDirection | layoutDirection |
qreal | minimumWidth |
QQmlComponent * | moreButton |
QQmlComponent * | separatorDelegate |
qreal | spacing |
qreal | visibleWidth |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Public Member Functions | |
ToolBarLayout (QQuickItem *parent=nullptr) | |
Q_SIGNAL void | actionsChanged () |
ActionsProperty | actionsProperty () const |
void | addAction (QObject *action) |
Qt::Alignment | alignment () const |
Q_SIGNAL void | alignmentChanged () |
void | clearActions () |
QQmlComponent * | fullDelegate () const |
Q_SIGNAL void | fullDelegateChanged () |
HeightMode | heightMode () const |
Q_SIGNAL void | heightModeChanged () |
QList< QObject * > | hiddenActions () const |
Q_SIGNAL void | hiddenActionsChanged () |
QQmlComponent * | iconDelegate () const |
Q_SIGNAL void | iconDelegateChanged () |
Qt::LayoutDirection | layoutDirection () const |
Q_SIGNAL void | layoutDirectionChanged () |
qreal | minimumWidth () const |
Q_SIGNAL void | minimumWidthChanged () |
QQmlComponent * | moreButton () const |
Q_SIGNAL void | moreButtonChanged () |
Q_SLOT void | relayout () |
void | removeAction (QObject *action) |
QQmlComponent * | separatorDelegate () const |
Q_SIGNAL void | separatorDelegateChanged () |
void | setAlignment (Qt::Alignment newAlignment) |
void | setFullDelegate (QQmlComponent *newFullDelegate) |
void | setHeightMode (HeightMode newHeightMode) |
void | setIconDelegate (QQmlComponent *newIconDelegate) |
void | setLayoutDirection (Qt::LayoutDirection &newLayoutDirection) |
void | setMoreButton (QQmlComponent *newMoreButton) |
void | setSeparatorDelegate (QQmlComponent *newSeparatorDelegate) |
void | setSpacing (qreal newSpacing) |
qreal | spacing () const |
Q_SIGNAL void | spacingChanged () |
qreal | visibleWidth () const |
Q_SIGNAL void | visibleWidthChanged () |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Static Public Member Functions | |
static ToolBarLayoutAttached * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
void | componentComplete () override |
void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) override |
void | itemChange (QQuickItem::ItemChange change, const QQuickItem::ItemChangeData &data) override |
void | updatePolish () override |
![]() | |
virtual bool | childMouseEventFilter (QQuickItem *item, QEvent *event) |
virtual void | classBegin () override |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | mouseUngrabEvent () |
virtual void | releaseResources () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An item that creates delegates for actions and lays them out in a row.
This effectively combines RowLayout and Repeater in a single item, with the addition of some extra performance enhancing tweaks. It will create instances of fullDelegate and ::itemDelegate for each action in actions . These are then positioned horizontally. Any action that ends up being placed outside the width of the item is hidden and will be part of hiddenActions.
The items created as delegates are always created asynchronously, to avoid creation lag spikes. Each delegate has access to the action it was created for through the ToolBarLayoutAttached attached property.
Definition at line 48 of file toolbarlayout.h.
Member Typedef Documentation
◆ ActionsProperty
Definition at line 144 of file toolbarlayout.h.
Member Enumeration Documentation
◆ HeightMode
An enum describing several modes that can be used to deal with items with a height that does not match the toolbar's height.
Definition at line 150 of file toolbarlayout.h.
Property Documentation
◆ actions
|
read |
The actions this layout should create delegates for.
Definition at line 56 of file toolbarlayout.h.
◆ alignment
|
readwrite |
How to align the delegates within this layout.
When there is more space available than required by the visible delegates, we need to determine how to place the delegates. This property determines how to do that. Note that the moreButton, if visible, will always be placed at the end of the layout.
Definition at line 106 of file toolbarlayout.h.
◆ fullDelegate
|
readwrite |
A component that is used to create full-size delegates from.
Each delegate has three states, it can be full-size, icon-only or hidden. By default, the full-size delegate is used. When the action has the DisplayHint::IconOnly hint set, it will always use the iconDelegate. When it has the DisplayHint::KeepVisible hint set, it will use the full-size delegate when it fits. If not, it will use the iconDelegate, unless even that does not fit, in which case it will still be hidden.
Definition at line 71 of file toolbarlayout.h.
◆ heightMode
|
readwrite |
How to handle items that do not match the toolbar's height.
When toolbar items do not match the height of the toolbar, there are several ways we can deal with this. This property sets the preferred way.
The default is HeightMode::ConstrainIfLarger .
- See also
- HeightMode
Definition at line 141 of file toolbarlayout.h.
◆ hiddenActions
A list of actions that do not fit in the current view and are thus hidden.
Definition at line 60 of file toolbarlayout.h.
◆ iconDelegate
|
readwrite |
A component that is used to create icon-only delegates from.
- See also
- fullDelegate
Definition at line 77 of file toolbarlayout.h.
◆ layoutDirection
|
readwrite |
Which direction to layout in.
This is primarily intended to support right-to-left layouts. When set to LeftToRight, delegates will be layout with the first item on the left and following items to the right of that. The more button will be placed at the rightmost position. Alignment flags work normally.
When set to RightToLeft, delegates will be layout with the first item on the right and following items to the left of that. The more button will be placed at the leftmost position. Alignment flags are inverted, so AlignLeft will align items to the right, and vice-versa.
Definition at line 130 of file toolbarlayout.h.
◆ minimumWidth
|
read |
The minimum width this layout can have.
This is equal to the width of the moreButton.
Definition at line 116 of file toolbarlayout.h.
◆ moreButton
|
readwrite |
A component that is used to create the "more button" item from.
The more button is shown when there are actions that do not fit the current view. It is intended to have functionality to show these hidden actions, like popup a menu with them showing.
Definition at line 93 of file toolbarlayout.h.
◆ separatorDelegate
|
readwrite |
A component that is used to create separator delegates from.
- Since
- 6.7
- See also
- fullDelegate
Definition at line 85 of file toolbarlayout.h.
◆ spacing
|
readwrite |
The amount of spacing between individual delegates.
Definition at line 97 of file toolbarlayout.h.
◆ visibleWidth
|
read |
The combined width of visible delegates in this layout.
Definition at line 110 of file toolbarlayout.h.
Constructor & Destructor Documentation
◆ ToolBarLayout()
ToolBarLayout::ToolBarLayout | ( | QQuickItem * | parent = nullptr | ) |
Definition at line 94 of file toolbarlayout.cpp.
◆ ~ToolBarLayout()
|
override |
Definition at line 121 of file toolbarlayout.cpp.
Member Function Documentation
◆ actionsProperty()
ToolBarLayout::ActionsProperty ToolBarLayout::actionsProperty | ( | ) | const |
Definition at line 125 of file toolbarlayout.cpp.
◆ addAction()
void ToolBarLayout::addAction | ( | QObject * | action | ) |
Add an action to the list of actions.
- Parameters
-
action The action to add.
Definition at line 130 of file toolbarlayout.cpp.
◆ alignment()
Qt::Alignment ToolBarLayout::alignment | ( | ) | const |
Definition at line 276 of file toolbarlayout.cpp.
◆ clearActions()
void ToolBarLayout::clearActions | ( | ) |
Clear the list of actions.
Definition at line 168 of file toolbarlayout.cpp.
◆ componentComplete()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 340 of file toolbarlayout.cpp.
◆ fullDelegate()
QQmlComponent * ToolBarLayout::fullDelegate | ( | ) | const |
Definition at line 189 of file toolbarlayout.cpp.
◆ geometryChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 347 of file toolbarlayout.cpp.
◆ heightMode()
ToolBarLayout::HeightMode ToolBarLayout::heightMode | ( | ) | const |
Definition at line 318 of file toolbarlayout.cpp.
◆ hiddenActions()
Definition at line 184 of file toolbarlayout.cpp.
◆ iconDelegate()
QQmlComponent * ToolBarLayout::iconDelegate | ( | ) | const |
Definition at line 206 of file toolbarlayout.cpp.
◆ itemChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 359 of file toolbarlayout.cpp.
◆ layoutDirection()
Qt::LayoutDirection ToolBarLayout::layoutDirection | ( | ) | const |
Definition at line 302 of file toolbarlayout.cpp.
◆ minimumWidth()
qreal ToolBarLayout::minimumWidth | ( | ) | const |
Definition at line 297 of file toolbarlayout.cpp.
◆ moreButton()
QQmlComponent * ToolBarLayout::moreButton | ( | ) | const |
Definition at line 240 of file toolbarlayout.cpp.
◆ qmlAttachedProperties()
|
inlinestatic |
Definition at line 227 of file toolbarlayout.h.
◆ relayout()
void ToolBarLayout::relayout | ( | ) |
Queue a relayout of this layout.
- Note
- The layouting happens during the next scene graph polishing phase.
Definition at line 334 of file toolbarlayout.cpp.
◆ removeAction()
void ToolBarLayout::removeAction | ( | QObject * | action | ) |
Remove an action from the list of actions.
- Parameters
-
action The action to remove.
Definition at line 153 of file toolbarlayout.cpp.
◆ separatorDelegate()
QQmlComponent * ToolBarLayout::separatorDelegate | ( | ) | const |
Definition at line 223 of file toolbarlayout.cpp.
◆ setAlignment()
void ToolBarLayout::setAlignment | ( | Qt::Alignment | newAlignment | ) |
Definition at line 281 of file toolbarlayout.cpp.
◆ setFullDelegate()
void ToolBarLayout::setFullDelegate | ( | QQmlComponent * | newFullDelegate | ) |
Definition at line 194 of file toolbarlayout.cpp.
◆ setHeightMode()
void ToolBarLayout::setHeightMode | ( | HeightMode | newHeightMode | ) |
Definition at line 323 of file toolbarlayout.cpp.
◆ setIconDelegate()
void ToolBarLayout::setIconDelegate | ( | QQmlComponent * | newIconDelegate | ) |
Definition at line 211 of file toolbarlayout.cpp.
◆ setLayoutDirection()
void ToolBarLayout::setLayoutDirection | ( | Qt::LayoutDirection & | newLayoutDirection | ) |
Definition at line 307 of file toolbarlayout.cpp.
◆ setMoreButton()
void ToolBarLayout::setMoreButton | ( | QQmlComponent * | newMoreButton | ) |
Definition at line 245 of file toolbarlayout.cpp.
◆ setSeparatorDelegate()
void ToolBarLayout::setSeparatorDelegate | ( | QQmlComponent * | newSeparatorDelegate | ) |
Definition at line 228 of file toolbarlayout.cpp.
◆ setSpacing()
void ToolBarLayout::setSpacing | ( | qreal | newSpacing | ) |
Definition at line 265 of file toolbarlayout.cpp.
◆ spacing()
qreal ToolBarLayout::spacing | ( | ) | const |
Definition at line 260 of file toolbarlayout.cpp.
◆ updatePolish()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 367 of file toolbarlayout.cpp.
◆ visibleWidth()
qreal ToolBarLayout::visibleWidth | ( | ) | const |
Definition at line 292 of file toolbarlayout.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:13:25 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.