KNSCore::Question
#include <question.h>
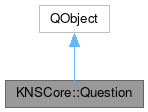
Public Member Functions | |
Question (QuestionType=YesNoQuestion, QObject *parent=nullptr) | |
Response | ask () |
Entry | entry () const |
QStringList | list () const |
QString | question () const |
QuestionType | questionType () const |
QString | response () const |
void | setEntry (const Entry &entry) |
void | setList (const QStringList &newList) |
void | setQuestion (const QString &newQuestion) |
void | setQuestionType (QuestionType newType=YesNoQuestion) |
void | setResponse (const QString &response) |
void | setResponse (Response response) |
void | setTitle (const QString &newTitle) |
QString | title () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A way to ask a user a question from inside a GUI-less library (like KNewStuffCore)
Rather than using a message box (which is a UI thing), when you want to ask your user a question, create an instance of this class and use that instead. The consuming library (in most cases KNewStuff or KNewStuffQuick) will listen to any question being asked, and act appropriately (that is, KNewStuff will show a dialog with an appropriate dialog box, and KNewStuffQuick will either request a question be asked if the developer is using the plugin directly, or ask the question using an appropriate method for Qt Quick based applications)
The following is an example of a question asking the user to select an item from a list.
Definition at line 46 of file question.h.
Member Enumeration Documentation
◆ QuestionType
enum KNSCore::Question::QuestionType |
Definition at line 60 of file question.h.
◆ Response
enum KNSCore::Question::Response |
Definition at line 50 of file question.h.
Constructor & Destructor Documentation
◆ Question()
|
explicit |
Definition at line 38 of file question.cpp.
Member Function Documentation
◆ ask()
Question::Response Question::ask | ( | ) |
Definition at line 47 of file question.cpp.
◆ entry()
Entry Question::entry | ( | ) | const |
Definition at line 117 of file question.cpp.
◆ list()
QStringList Question::list | ( | ) | const |
Definition at line 91 of file question.cpp.
◆ question()
QString Question::question | ( | ) | const |
Definition at line 71 of file question.cpp.
◆ questionType()
Question::QuestionType Question::questionType | ( | ) | const |
Definition at line 56 of file question.cpp.
◆ response()
QString Question::response | ( | ) | const |
Definition at line 107 of file question.cpp.
◆ setEntry()
void Question::setEntry | ( | const Entry & | entry | ) |
Definition at line 112 of file question.cpp.
◆ setList()
void Question::setList | ( | const QStringList & | newList | ) |
Definition at line 86 of file question.cpp.
◆ setQuestion()
void Question::setQuestion | ( | const QString & | newQuestion | ) |
Definition at line 66 of file question.cpp.
◆ setQuestionType()
void Question::setQuestionType | ( | Question::QuestionType | newType = YesNoQuestion | ) |
Definition at line 61 of file question.cpp.
◆ setResponse() [1/2]
void Question::setResponse | ( | const QString & | response | ) |
If the user has any way of inputting data to go along with the response above, consider this a part of the response.
As such, you can set, and later get, that response as well. This does NOT mark the question as answered (
- See also
- setResponse(Response) ).
- Parameters
-
response This sets the string response for the question
Definition at line 102 of file question.cpp.
◆ setResponse() [2/2]
void Question::setResponse | ( | Response | response | ) |
When the user makes a choice on a question, that is a response.
This is the return value in ask().
- Parameters
-
response This will set the response, and mark the question as answered
Definition at line 96 of file question.cpp.
◆ setTitle()
void Question::setTitle | ( | const QString & | newTitle | ) |
Definition at line 76 of file question.cpp.
◆ title()
QString Question::title | ( | ) | const |
Definition at line 81 of file question.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:58:07 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.