Purpose::Configuration
#include <configuration.h>
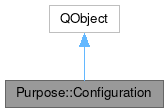
Properties | |
QUrl | configSourceCode |
QJsonObject | data |
bool | isReady |
QJsonArray | neededArguments |
QString | pluginName |
QString | pluginTypeName |
![]() | |
objectName | |
Signals | |
void | dataChanged () |
Public Member Functions | |
Configuration (const QJsonObject &inputData, const QString &pluginTypeName, const KPluginMetaData &pluginInformation, QObject *parent=nullptr) | |
Configuration (const QJsonObject &inputData, const QString &pluginTypeName, const QJsonObject &pluginType, const KPluginMetaData &pluginInformation, QObject *parent=nullptr) | |
QUrl | configSourceCode () const |
Q_SCRIPTABLE Purpose::Job * | createJob () |
QJsonObject | data () const |
bool | isReady () const |
QJsonArray | neededArguments () const |
QString | pluginName () const |
QString | pluginTypeName () const |
void | setData (const QJsonObject &data) |
void | setUseSeparateProcess (bool separate) |
bool | useSeparateProcess () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class will be in charge of figuring out the job configuration.
Once it's figured out, it can proceed to create the job.
The object will be destroyed as soon as the job finishes.
Definition at line 32 of file configuration.h.
Property Documentation
◆ configSourceCode
|
read |
Specifies the qml source code to be used, to configure the current job.
- See also
- JobController QtQuick component
Definition at line 59 of file configuration.h.
◆ data
|
readwrite |
Represents the data the job will have available to perform its task.
Definition at line 47 of file configuration.h.
◆ isReady
|
read |
Tells whether there's still information to be provided, to be able to run the job.
- See also
- X-Purpose-MandatoryArguments and X-Purpose-AdditionalArguments
Definition at line 42 of file configuration.h.
◆ neededArguments
|
read |
Specifies the arguments the config file and the job will be sharing.
Definition at line 52 of file configuration.h.
◆ pluginName
|
read |
- Returns
- the plugin name to display
Definition at line 69 of file configuration.h.
◆ pluginTypeName
|
read |
- Returns
- the plugin type name to display
Definition at line 64 of file configuration.h.
Constructor & Destructor Documentation
◆ Configuration() [1/2]
Configuration::Configuration | ( | const QJsonObject & | inputData, |
const QString & | pluginTypeName, | ||
const QJsonObject & | pluginType, | ||
const KPluginMetaData & | pluginInformation, | ||
QObject * | parent = nullptr ) |
Definition at line 79 of file configuration.cpp.
◆ Configuration() [2/2]
Configuration::Configuration | ( | const QJsonObject & | inputData, |
const QString & | pluginTypeName, | ||
const KPluginMetaData & | pluginInformation, | ||
QObject * | parent = nullptr ) |
Definition at line 74 of file configuration.cpp.
◆ ~Configuration()
|
override |
Definition at line 89 of file configuration.cpp.
Member Function Documentation
◆ configSourceCode()
QUrl Configuration::configSourceCode | ( | ) | const |
Definition at line 156 of file configuration.cpp.
◆ createJob()
Purpose::Job * Configuration::createJob | ( | ) |
- Returns
- the configured job ready to be started.
Before calling it, make sure that all information has been filled by checking isReady().
Definition at line 136 of file configuration.cpp.
◆ data()
QJsonObject Configuration::data | ( | ) | const |
Definition at line 105 of file configuration.cpp.
◆ isReady()
bool Configuration::isReady | ( | ) | const |
Definition at line 111 of file configuration.cpp.
◆ neededArguments()
QJsonArray Configuration::neededArguments | ( | ) | const |
Definition at line 125 of file configuration.cpp.
◆ pluginName()
QString Configuration::pluginName | ( | ) | const |
Definition at line 194 of file configuration.cpp.
◆ pluginTypeName()
QString Configuration::pluginTypeName | ( | ) | const |
Definition at line 187 of file configuration.cpp.
◆ setData()
void Configuration::setData | ( | const QJsonObject & | data | ) |
Definition at line 94 of file configuration.cpp.
◆ setUseSeparateProcess()
void Configuration::setUseSeparateProcess | ( | bool | separate | ) |
separate
will specify whether the process will be forced to execute in-process or in a separate process.
Definition at line 181 of file configuration.cpp.
◆ useSeparateProcess()
bool Configuration::useSeparateProcess | ( | ) | const |
- Returns
- whether the job will be run in the same process.
By default it will be true, unless the environment variable KDE_PURPOSE_LOCAL_JOBS is defined
Definition at line 175 of file configuration.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 12:02:54 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.