CalendarSupport::CalPrintPluginBase
#include <calprintpluginbase.h>
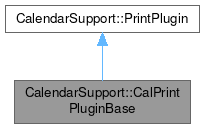
Public Types | |
enum | DisplayFlags { Text = 0x0001 , TimeBoxes = 0x0002 } |
![]() | |
using | List = QList<PrintPlugin *> |
Public Member Functions | |
CalPrintPluginBase () | |
int | borderWidth () const |
QWidget * | createConfigWidget (QWidget *) override |
void | doLoadConfig () override |
void | doPrint (QPrinter *printer) override |
void | doSaveConfig () override |
void | drawAgendaDayBox (QPainter &p, const KCalendarCore::Event::List &eventList, QDate qd, bool expandable, QTime fromTime, QTime toTime, QRect box, bool includeDescription, bool includeCategories, bool excludeTime, const QList< QDate > &workDays) |
void | drawAgendaItem (PrintCellItem *item, QPainter &p, const QDateTime &startPrintDate, const QDateTime &endPrintDate, float minlen, QRect box, bool includeDescription, bool includeCategories, bool excludeTime) |
int | drawBoxWithCaption (QPainter &p, QRect box, const QString &caption, const QString &contents, bool sameLine, bool expand, const QFont &captionFont, const QFont &textFont, bool richContents=false) |
void | drawDayBox (QPainter &p, QDate qd, QTime fromTime, QTime toTime, QRect box, bool fullDate=false, bool printRecurDaily=true, bool printRecurWeekly=true, bool singleLineLimit=true, bool includeDescription=false, bool includeCategories=false) |
void | drawDaysOfWeek (QPainter &p, QDate fromDate, QDate toDate, QRect box) |
void | drawDaysOfWeekBox (QPainter &p, QDate qd, QRect box) |
int | drawFooter (QPainter &p, QRect box) |
int | drawHeader (QPainter &p, const QString &title, QDate month1, QDate month2, QRect box, bool expand=false, QColor backColor=QColor()) |
void | drawMonth (QPainter &p, QDate dt, QRect box, int maxdays=-1, int subDailyFlags=TimeBoxes, int holidaysFlags=Text) |
void | drawMonthTable (QPainter &p, QDate qd, QTime fromTime, QTime toTime, bool weeknumbers, bool recurDaily, bool recurWeekly, bool singleLineLimit, bool includeDescription, bool includeCategories, QRect box) |
void | drawNoteLines (QPainter &p, QRect box, int startY) |
void | drawSmallMonth (QPainter &p, QDate qd, QRect box) |
void | drawSplitHeaderRight (QPainter &p, QDate fd, QDate td, QDate cd, int width, int height) |
void | drawSubHeaderBox (QPainter &p, const QString &str, QRect box) |
void | drawTextLines (QPainter &p, const QString &entry, int x, int &y, int width, int pageHeight, bool richTextEntry) |
void | drawTimeLine (QPainter &p, QTime fromTime, QTime toTime, QRect box) |
void | drawTodo (int &count, const KCalendarCore::Todo::Ptr &todo, QPainter &p, KCalendarCore::TodoSortField sortField, KCalendarCore::SortDirection sortDir, bool connectSubTodos, bool strikeoutCompleted, bool desc, int posPriority, int posSummary, int posCategories, int posStartDt, int posDueDt, int posPercentComplete, int level, int x, int &y, int width, int pageHeight, const KCalendarCore::Todo::List &todoList, TodoParentStart *r) |
void | drawVerticalBox (QPainter &p, int linewidth, QRect box, const QString &str, int flags=-1) |
int | footerHeight () const |
int | headerHeight () const |
int | margin () const |
QPageLayout::Orientation | orientation () const |
int | padding () const |
virtual void | print (QPainter &p, int width, int height)=0 |
void | printEventString (QPainter &p, QRect box, const QString &str, int flags=-1) |
bool | printFooter () const |
void | setBorderWidth (const int border) |
void | setFooterHeight (const int height) |
void | setHeaderHeight (const int height) |
void | setMargin (const int margin) |
void | setPadding (const int margin) |
void | setPrintFooter (bool printFooter) |
void | setSubHeaderHeight (const int height) |
void | setUseColors (bool useColors) |
void | showEventBox (QPainter &p, int linewidth, QRect box, const KCalendarCore::Incidence::Ptr &incidence, const QString &str, int flags=-1) |
int | subHeaderHeight () const |
bool | useColors () const |
![]() | |
QWidget * | configWidget (QWidget *w) |
virtual QPageLayout::Orientation | defaultOrientation () const |
virtual QString | description () const =0 |
virtual bool | enabled () const |
virtual QString | groupName () const =0 |
virtual QString | info () const =0 |
virtual void | readSettingsWidget () |
virtual KCalendarCore::Incidence::List | selectedIncidences () const |
virtual void | setCalendar (const KCalendarCore::Calendar::Ptr &cal) |
virtual void | setConfig (KConfig *cfg) |
virtual void | setDateRange (const QDate &from, const QDate &to) |
virtual void | setSelectedIncidences (const KCalendarCore::Incidence::List &inc) |
virtual void | setSettingsWidget () |
virtual int | sortID () const |
Static Public Member Functions | |
static void | drawBox (QPainter &p, int linewidth, QRect rect) |
static void | drawShadedBox (QPainter &p, int linewidth, const QBrush &brush, QRect rect) |
static int | weekdayColumn (int weekday) |
Protected Member Functions | |
QColor | categoryBgColor (const KCalendarCore::Incidence::Ptr &incidence) const |
QTime | dayStart () const |
void | drawIncidence (QPainter &p, QRect dayBox, const QString &time, const QString &summary, const QString &description, int &textY, bool singleLineLimit, bool includeDescription, bool richDescription) |
void | drawTodoLines (QPainter &p, const QString &entry, int x, int &y, int width, int pageHeight, bool richTextEntry, QList< TodoParentStart * > &startPoints, bool connectSubTodos) |
KCalendarCore::Event::Ptr | holidayEvent (QDate date) const |
QString | toPlainText (const QString &htmlText) |
Protected Attributes | |
int | mBorder |
bool | mExcludeConfidential |
bool | mExcludePrivate |
int | mFooterHeight |
int | mHeaderHeight |
int | mMargin |
int | mPadding |
bool | mPrintFooter |
bool | mShowNoteLines |
int | mSubHeaderHeight |
bool | mUseColors |
![]() | |
KCalendarCore::Calendar::Ptr | mCalendar |
KConfig * | mConfig = nullptr |
QPointer< QWidget > | mConfigWidget |
QDate | mFromDate |
QPrinter * | mPrinter = nullptr |
KCalendarCore::Incidence::List | mSelectedIncidences |
QDate | mToDate |
Static Protected Attributes | |
static const QColor | sHolidayBackground = QColor(244, 244, 244) |
Detailed Description
Base class for Calendar printing classes.
Each sub class represents one calendar print format.
Definition at line 43 of file calprintpluginbase.h.
Member Enumeration Documentation
◆ DisplayFlags
enum CalendarSupport::CalPrintPluginBase::DisplayFlags |
Definition at line 46 of file calprintpluginbase.h.
Constructor & Destructor Documentation
◆ CalPrintPluginBase()
CalPrintPluginBase::CalPrintPluginBase | ( | ) |
Constructor.
Definition at line 116 of file calprintpluginbase.cpp.
Member Function Documentation
◆ borderWidth()
|
nodiscard |
Definition at line 388 of file calprintpluginbase.cpp.
◆ categoryBgColor()
|
protected |
Definition at line 282 of file calprintpluginbase.cpp.
◆ createConfigWidget()
Returns widget for configuring the print format.
Implements CalendarSupport::PrintPlugin.
Definition at line 130 of file calprintpluginbase.cpp.
◆ dayStart()
|
protected |
Definition at line 249 of file calprintpluginbase.cpp.
◆ doLoadConfig()
|
overridevirtual |
Load complete configuration.
Each implementation calls its parent's implementation to load parent configuration options, then loads its own.
Reimplemented from CalendarSupport::PrintPlugin.
Definition at line 180 of file calprintpluginbase.cpp.
◆ doPrint()
|
overridevirtual |
Start printing.
Implements CalendarSupport::PrintPlugin.
Definition at line 149 of file calprintpluginbase.cpp.
◆ doSaveConfig()
|
overridevirtual |
Save complete configuration.
Each implementation saves its own configuration options, then calls its parent's implementation to save parent options.
Reimplemented from CalendarSupport::PrintPlugin.
Definition at line 198 of file calprintpluginbase.cpp.
◆ drawAgendaDayBox()
void CalPrintPluginBase::drawAgendaDayBox | ( | QPainter & | p, |
const KCalendarCore::Event::List & | eventList, | ||
QDate | qd, | ||
bool | expandable, | ||
QTime | fromTime, | ||
QTime | toTime, | ||
QRect | box, | ||
bool | includeDescription, | ||
bool | includeCategories, | ||
bool | excludeTime, | ||
const QList< QDate > & | workDays ) |
Draw the agenda box for the day print style (the box showing all events of that day).
Also draws a grid with half-hour spacing of the grid lines. Does NOT draw allday events. Use drawAllDayBox for allday events.
Obeys configuration options mExcludeConfidential, #excludePrivate.
- Parameters
-
p QPainter of the printout eventList The list of the events that are supposed to be printed inside this box qd The date of the currently printed day expandable If true, the start and end times are adjusted to include the whole range of all events of that day, not just of the given time range. The height of the box will not be affected by this (but the height of one hour will be scaled down so that the whole range fits into the box. fromTime and toTime receive the actual time range printed by this function). fromTime Start of the time range to be printed. Might be adjusted to include all events if expandable==true toTime End of the time range to be printed. Might be adjusted to include all events if expandable==true box coordinates of the agenda day box. includeDescription Whether to print the event description as well. includeCategories Whether to print the event categories (tags) as well. excludeTime Whether the time is printed in the detail area. workDays List of workDays
Definition at line 764 of file calprintpluginbase.cpp.
◆ drawAgendaItem()
void CalPrintPluginBase::drawAgendaItem | ( | PrintCellItem * | item, |
QPainter & | p, | ||
const QDateTime & | startPrintDate, | ||
const QDateTime & | endPrintDate, | ||
float | minlen, | ||
QRect | box, | ||
bool | includeDescription, | ||
bool | includeCategories, | ||
bool | excludeTime ) |
Definition at line 885 of file calprintpluginbase.cpp.
◆ drawBox()
Draw a box with given width at the given coordinates.
- Parameters
-
p The printer to be used linewidth The border width of the box rect The rectangle of the box
Definition at line 398 of file calprintpluginbase.cpp.
◆ drawBoxWithCaption()
int CalPrintPluginBase::drawBoxWithCaption | ( | QPainter & | p, |
QRect | box, | ||
const QString & | caption, | ||
const QString & | contents, | ||
bool | sameLine, | ||
bool | expand, | ||
const QFont & | captionFont, | ||
const QFont & | textFont, | ||
bool | richContents = false ) |
Draw a component box with a heading (printed in bold).
- Parameters
-
p QPainter of the printout box Coordinates of the box caption Caption string to be printed inside the box contents Normal text contents of the box. If contents.isNull(), then no text will be printed, only the caption. sameLine Whether the contents should start on the same line as the caption (the space below the caption text will be used as indentation in the subsequent lines) or on the next line (no indentation of the contents) expand Whether to expand the box vertically to fit the whole text in it. rickContents Whether contents contains rich text.
- Returns
- The bottom of the printed box. If expand==true, the bottom of the drawn box is returned, if expand==false, the vertical end of the printed contents inside the box is returned. If you want to print some custom graphics or text below the contents, use the return value as the top-value of your custom contents in that case.
Definition at line 470 of file calprintpluginbase.cpp.
◆ drawDayBox()
void CalPrintPluginBase::drawDayBox | ( | QPainter & | p, |
QDate | qd, | ||
QTime | fromTime, | ||
QTime | toTime, | ||
QRect | box, | ||
bool | fullDate = false, | ||
bool | printRecurDaily = true, | ||
bool | printRecurWeekly = true, | ||
bool | singleLineLimit = true, | ||
bool | includeDescription = false, | ||
bool | includeCategories = false ) |
Draw the box containing a list of all events of the given day (with their times, of course).
Used in the Filofax and the month print style.
Obeys configuration options mExcludeConfidential, mExcludePrivate, mShowNoteLines, mUseColors.
- Parameters
-
p QPainter of the printout qd The date of the currently printed day. All events of the calendar that appear on that day will be printed. fromTime Start time of the time range to display toTime End time of the time range to display box coordinates of the day box. fullDate Whether the title bar of the box should contain the full date string or just a short. printRecurDaily Whether daily recurring incidences should be printed. printRecurWeekly Whether weekly recurring incidences should be printed. singleLineLimit Whether Incidence text wraps or truncates. includeDescription Whether to print the event description as well. includeCategories Whether to print the event categories (tags) as well.
Definition at line 966 of file calprintpluginbase.cpp.
◆ drawDaysOfWeek()
Draw a horizontal bar with the weekday names of the given date range in the given area of the painter.
This is used for the weekday-bar on top of the timetable view and the month view.
- Parameters
-
p QPainter of the printout fromDate First date of the printed dates toDate Last date of the printed dates box coordinates of the box for the days of the week
Definition at line 680 of file calprintpluginbase.cpp.
◆ drawDaysOfWeekBox()
Draw a single weekday name in a box inside the given area of the painter.
This is called in a loop by drawDaysOfWeek.
- Parameters
-
p QPainter of the printout qd Date of the printed day box coordinates of the weekbox
Definition at line 696 of file calprintpluginbase.cpp.
◆ drawFooter()
Draw a page footer containing the printing date and possibly other things, like a page number.
- Parameters
-
p QPainter of the printout box coordinates of the footer
- Returns
- The bottom of the printed box.
Definition at line 609 of file calprintpluginbase.cpp.
◆ drawHeader()
int CalPrintPluginBase::drawHeader | ( | QPainter & | p, |
const QString & | title, | ||
QDate | month1, | ||
QDate | month2, | ||
QRect | box, | ||
bool | expand = false, | ||
QColor | backColor = QColor() ) |
Draw the gray header bar of the printout to the QPainter.
It prints the given text and optionally one or two small month views, as specified by the two QDate. The printed text can also contain a line feed. If month2 is invalid, only the month that contains month1 is printed. E.g. the filofax week view draws just the current month, while the month view draws the previous and the next month.
- Parameters
-
p QPainter of the printout title The string printed as the title of the page (e.g. the date, date range or todo list title) month1 Date specifying the month for the left one of the small month views in the title bar. If left empty, only month2 will be printed (or none, it that is invalid as well). month2 Date specifying the month for the right one of the small month views in the title bar. If left empty, only month1 will be printed (or none, it that is invalid as well). box coordinates of the title bar expand Whether to expand the box vertically to fit the whole title in it. backColor background color for the header box.
- Returns
- The bottom of the printed box. If expand==false, this is box.bottom, otherwise it is larger than box.bottom and matches the y-coordinate of the surrounding rectangle.
Definition at line 554 of file calprintpluginbase.cpp.
◆ drawIncidence()
|
protected |
Definition at line 1145 of file calprintpluginbase.cpp.
◆ drawMonth()
void CalPrintPluginBase::drawMonth | ( | QPainter & | p, |
QDate | dt, | ||
QRect | box, | ||
int | maxdays = -1, | ||
int | subDailyFlags = TimeBoxes, | ||
int | holidaysFlags = Text ) |
Draw a vertical representation of the month containing the date dt.
Each day gets one line.
Obeys configuration options mExcludeConfidential, #excludePrivate.
- Parameters
-
p QPainter of the printout dt Arbitrary date within the month to be printed box coordinates of the box reserved for the month maxdays Days to print. If a value of -1 is given, the number of days is deduced from the month. If maxdays is larger than the number of days in the month, the remaining boxes are shaded to indicate they are not days of the month. subDailyFlags Bitfield consisting of DisplayFlags flags to determine how events that do not cross midnight should be printed. holidaysFlags Bitfield consisting of DisplayFlags flags to determine how holidays should be printed.
Definition at line 1260 of file calprintpluginbase.cpp.
◆ drawMonthTable()
void CalPrintPluginBase::drawMonthTable | ( | QPainter & | p, |
QDate | qd, | ||
QTime | fromTime, | ||
QTime | toTime, | ||
bool | weeknumbers, | ||
bool | recurDaily, | ||
bool | recurWeekly, | ||
bool | singleLineLimit, | ||
bool | includeDescription, | ||
bool | includeCategories, | ||
QRect | box ) |
Draw the month table of the month containing the date qd.
Each day gets one box (using drawDayBox) that contains a list of all events on that day. They are arranged in a matrix, with the first column being the first day of the week (so it might display some days of the previous and the next month). Above the matrix there is a bar showing the weekdays (drawn using drawDaysOfWeek).
Obeys configuration options mExcludeConfidential, mExcludePrivate, mShowNoteLines, mUseColors.
- Parameters
-
p QPainter of the printout qd Arbitrary date within the month to be printed. fromTime Start time of the displayed time range toTime End time of the displayed time range weeknumbers Whether the week numbers are printed left of each row of the matrix recurDaily Whether daily recurring incidences should be printed. recurWeekly Whether weekly recurring incidences should be printed. singleLineLimit Whether Incidence text wraps or truncates. includeDescription Whether descriptions are printed. includeCategories Whether to print the event categories (tags) as well. box coordinates of the month.
Definition at line 1456 of file calprintpluginbase.cpp.
◆ drawNoteLines()
Draws dotted lines for notes in a box.
- Parameters
-
p QPainter of the printout box coordinates of the box where the lines will be placed startY starting y-coordinate for the first line
Definition at line 1920 of file calprintpluginbase.cpp.
◆ drawShadedBox()
|
static |
Draw a shaded box with given width at the given coordinates.
- Parameters
-
p The printer to be used linewidth The border width of the box brush The brush to fill the box rect The rectangle of the box
Definition at line 413 of file calprintpluginbase.cpp.
◆ drawSmallMonth()
Draw a small calendar with the days of a month into the given area.
Used for example in the title bar of the sheet.
- Parameters
-
p QPainter of the printout qd Arbitrary Date within the month to be printed. box coordinates of the small calendar
Definition at line 620 of file calprintpluginbase.cpp.
◆ drawSplitHeaderRight()
void CalPrintPluginBase::drawSplitHeaderRight | ( | QPainter & | p, |
QDate | fd, | ||
QDate | td, | ||
QDate | cd, | ||
int | width, | ||
int | height ) |
Definition at line 1869 of file calprintpluginbase.cpp.
◆ drawSubHeaderBox()
Draw a subheader box with a shaded background and the given string.
- Parameters
-
p QPainter of the printout str Text to be printed inside the box box Coordinates of the box
Definition at line 447 of file calprintpluginbase.cpp.
◆ drawTextLines()
void CalPrintPluginBase::drawTextLines | ( | QPainter & | p, |
const QString & | entry, | ||
int | x, | ||
int & | y, | ||
int | width, | ||
int | pageHeight, | ||
bool | richTextEntry ) |
Draws text lines splitting on page boundaries.
- Parameters
-
p QPainter of the printout x x-coordinate of the upper left coordinate of the first item y y-coordinate of the upper left coordinate of the first item width width of the whole list pageHeight size of the page. A new page is started when the text reaches the end of the page.
Definition at line 1841 of file calprintpluginbase.cpp.
◆ drawTimeLine()
Draw a (vertical) time scale from time fromTime to toTime inside the given area of the painter.
Every hour will have a one-pixel line over the whole width, every half-hour the line will only span the left half of the width. This is used in the day and timetable print styles
- Parameters
-
p QPainter of the printout fromTime Start time of the time range to display toTime End time of the time range to display box coordinates of the timeline
Definition at line 701 of file calprintpluginbase.cpp.
◆ drawTodo()
void CalPrintPluginBase::drawTodo | ( | int & | count, |
const KCalendarCore::Todo::Ptr & | todo, | ||
QPainter & | p, | ||
KCalendarCore::TodoSortField | sortField, | ||
KCalendarCore::SortDirection | sortDir, | ||
bool | connectSubTodos, | ||
bool | strikeoutCompleted, | ||
bool | desc, | ||
int | posPriority, | ||
int | posSummary, | ||
int | posCategories, | ||
int | posStartDt, | ||
int | posDueDt, | ||
int | posPercentComplete, | ||
int | level, | ||
int | x, | ||
int & | y, | ||
int | width, | ||
int | pageHeight, | ||
const KCalendarCore::Todo::List & | todoList, | ||
TodoParentStart * | r ) |
Draws single to-do and its (indented) sub-to-dos, optionally connects them by a tree-like line, and optionally shows due date, summary, description and priority.
- Parameters
-
count The number of the currently printed to-do (count will be incremented for each to-do drawn) todo The to-do to be printed. It's sub-to-dos are recursively drawn, so drawTodo should only be called on the to-dos of the highest level. p QPainter of the printout sortField Specifies on which attribute of the todo you want to sort. sortDir Specifies if you want to sort ascending or descending. connectSubTodos Whether sub-to-dos shall be connected with their parent by a line (tree-like). strikeoutCompleted Whether completed to-dos should be printed with strike-out summaries. desc Whether to print the whole description of the to-do (the summary is always printed). posPriority x-coordinate where the priority is supposed to be printed. If negative, no priority will be printed. posSummary x-coordinate where the summary of the to-do is supposed to be printed. posCategories x-coordinate where the categories (tags) should be printed. If negative, no categories will be printed. posStartDt x-coordinate where the due date is supposed to the be printed. If negative, no start date will be printed. posDueDt x-coordinate where the due date is supposed to the be printed. If negative, no due date will be printed. posPercentComplete x-coordinate where the percentage complete is supposed to be printed. If negative, percentage complete will not be printed. level Level of the current to-do in the to-do hierarchy (0 means highest level of printed to-dos, 1 are their sub-to-dos, etc.) x x-coordinate of the upper left coordinate of the first to-do. y y-coordinate of the upper left coordinate of the first to-do. width width of the whole to-do list. pageHeight Total height allowed for the to-do list on a page. If an to-do would be below that line, a new page is started. todoList Contains a list of sub-todos for the specified todo
.r Internal (used when printing sub-to-dos to give information about its parent)
Definition at line 1584 of file calprintpluginbase.cpp.
◆ drawTodoLines()
|
protected |
Definition at line 1534 of file calprintpluginbase.cpp.
◆ drawVerticalBox()
void CalPrintPluginBase::drawVerticalBox | ( | QPainter & | p, |
int | linewidth, | ||
QRect | box, | ||
const QString & | str, | ||
int | flags = -1 ) |
Draw an event box with vertical text.
- Parameters
-
p QPainter of the printout linewidth is the width of the line used to draw the box, ignored if less than 1. box Coordinates of the box str ext to be printed inside the box flags is a bitwise OR of Qt::AlignmentFlags and Qt::TextFlags values.
Definition at line 456 of file calprintpluginbase.cpp.
◆ footerHeight()
|
nodiscard |
Returns the height of the page footer.
If the height was explicitly set using setFooterHeight, that value is returned, otherwise a default value based on the printer orientation.
- Returns
- height of the page footer of the printout
Definition at line 348 of file calprintpluginbase.cpp.
◆ headerHeight()
|
nodiscard |
Returns the height of the page header.
If the height was explicitly set using setHeaderHeight, that value is returned, otherwise a default value based on the printer orientation.
- Returns
- height of the page header of the printout
Definition at line 322 of file calprintpluginbase.cpp.
◆ holidayEvent()
|
protected |
Definition at line 303 of file calprintpluginbase.cpp.
◆ margin()
|
nodiscard |
Definition at line 368 of file calprintpluginbase.cpp.
◆ orientation()
QPageLayout::Orientation CalPrintPluginBase::orientation | ( | ) | const |
Definition at line 238 of file calprintpluginbase.cpp.
◆ padding()
|
nodiscard |
Definition at line 378 of file calprintpluginbase.cpp.
◆ print()
|
pure virtual |
Actually do the printing.
- Parameters
-
p QPainter the print result is painted to width Width of printable area height Height of printable area
◆ printEventString()
void CalPrintPluginBase::printEventString | ( | QPainter & | p, |
QRect | box, | ||
const QString & | str, | ||
int | flags = -1 ) |
Print the given string (event summary) in the given rectangle.
Margins and justification (centered or not) are automatically adjusted.
- Parameters
-
p QPainter of the printout box Coordinates of the surrounding event box str The text to be printed in the box
Definition at line 421 of file calprintpluginbase.cpp.
◆ printFooter()
|
nodiscard |
Definition at line 228 of file calprintpluginbase.cpp.
◆ setBorderWidth()
void CalPrintPluginBase::setBorderWidth | ( | const int | border | ) |
Definition at line 393 of file calprintpluginbase.cpp.
◆ setFooterHeight()
void CalPrintPluginBase::setFooterHeight | ( | const int | height | ) |
Definition at line 363 of file calprintpluginbase.cpp.
◆ setHeaderHeight()
void CalPrintPluginBase::setHeaderHeight | ( | const int | height | ) |
Definition at line 333 of file calprintpluginbase.cpp.
◆ setMargin()
void CalPrintPluginBase::setMargin | ( | const int | margin | ) |
Definition at line 373 of file calprintpluginbase.cpp.
◆ setPadding()
void CalPrintPluginBase::setPadding | ( | const int | margin | ) |
Definition at line 383 of file calprintpluginbase.cpp.
◆ setPrintFooter()
void CalPrintPluginBase::setPrintFooter | ( | bool | printFooter | ) |
Definition at line 233 of file calprintpluginbase.cpp.
◆ setSubHeaderHeight()
void CalPrintPluginBase::setSubHeaderHeight | ( | const int | height | ) |
Definition at line 343 of file calprintpluginbase.cpp.
◆ setUseColors()
void CalPrintPluginBase::setUseColors | ( | bool | useColors | ) |
Definition at line 223 of file calprintpluginbase.cpp.
◆ showEventBox()
void CalPrintPluginBase::showEventBox | ( | QPainter & | p, |
int | linewidth, | ||
QRect | box, | ||
const KCalendarCore::Incidence::Ptr & | incidence, | ||
const QString & | str, | ||
int | flags = -1 ) |
Print the box for the given event with the given string.
- Parameters
-
p QPainter of the printout linewidth is the width of the line used to draw the box, ignored if less than 1. box Coordinates of the event's box incidence The incidence (if available), from which the category color will be deduced, if applicable. str The string to print inside the box flags is a bitwise OR of Qt::AlignmentFlags and Qt::TextFlags values.
Definition at line 428 of file calprintpluginbase.cpp.
◆ subHeaderHeight()
int CalPrintPluginBase::subHeaderHeight | ( | ) | const |
Definition at line 338 of file calprintpluginbase.cpp.
◆ toPlainText()
Definition at line 1938 of file calprintpluginbase.cpp.
◆ useColors()
|
nodiscard |
HELPER FUNCTIONS.
Definition at line 218 of file calprintpluginbase.cpp.
◆ weekdayColumn()
|
static |
Determines the column of the given weekday ( 1=Monday, 7=Sunday ), taking the start of the week setting into account as given in kcontrol.
- Parameters
-
weekday Index of the weekday
Definition at line 1835 of file calprintpluginbase.cpp.
Member Data Documentation
◆ mBorder
|
protected |
Definition at line 552 of file calprintpluginbase.h.
◆ mExcludeConfidential
|
protected |
Whether or not to print incidences with secrecy "confidential".
Definition at line 545 of file calprintpluginbase.h.
◆ mExcludePrivate
|
protected |
Whether or not to print incidences with secrecy "private".
Definition at line 546 of file calprintpluginbase.h.
◆ mFooterHeight
|
protected |
Definition at line 549 of file calprintpluginbase.h.
◆ mHeaderHeight
|
protected |
Definition at line 547 of file calprintpluginbase.h.
◆ mMargin
|
protected |
Definition at line 550 of file calprintpluginbase.h.
◆ mPadding
|
protected |
Definition at line 551 of file calprintpluginbase.h.
◆ mPrintFooter
|
protected |
Whether or not to print a footer at the bottoms of pages.
Definition at line 543 of file calprintpluginbase.h.
◆ mShowNoteLines
|
protected |
Whether or not to print horizontal lines in note areas.
Definition at line 544 of file calprintpluginbase.h.
◆ mSubHeaderHeight
|
protected |
Definition at line 548 of file calprintpluginbase.h.
◆ mUseColors
|
protected |
Whether or not to use event category colors to draw the events.
Definition at line 542 of file calprintpluginbase.h.
◆ sHolidayBackground
Definition at line 554 of file calprintpluginbase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:57:26 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.