IncidenceEditorNG::EditorItemManager
#include <editoritemmanager.h>
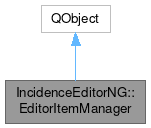
Public Types | |
enum | ItemState { AfterSave , BeforeSave } |
enum | ItipPrivacy { ItipPrivacyPlain = 0 , ItipPrivacySign = 1 , ItipPrivacyEncrypt = 2 } |
typedef QFlags< ItipPrivacy > | ItipPrivacyFlags |
enum | SaveAction { Create , Modify , None , Move , MoveAndModify } |
![]() | |
typedef | QObjectList |
Signals | |
void | itemSaveFailed (IncidenceEditorNG::EditorItemManager::SaveAction action, const QString &message) |
void | itemSaveFinished (IncidenceEditorNG::EditorItemManager::SaveAction action) |
void | revertFailed (const QString &message) |
void | revertFinished () |
Public Member Functions | |
EditorItemManager (ItemEditorUi *ui, Akonadi::IncidenceChanger *changer=nullptr) | |
~EditorItemManager () override | |
Akonadi::Item | item (ItemState state=AfterSave) const |
void | load (const Akonadi::Item &item) |
void | save (ItipPrivacyFlags itipPrivacy=ItipPrivacyPlain) |
void | setIsCounterProposal (bool isCounterProposal) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Helper class for creating dialogs that let the user create and edit the payload of Akonadi items (e.g.
events, contacts, etc). This class supports editing of one item at a time and handles all Akonadi specific logic like Item creation, Item modifying and monitoring of changes to the item during editing.
Definition at line 35 of file editoritemmanager.h.
Member Typedef Documentation
◆ ItipPrivacyFlags
QFlags< ItipPrivacy > IncidenceEditorNG::EditorItemManager::ItipPrivacyFlags |
Definition at line 44 of file editoritemmanager.h.
Member Enumeration Documentation
◆ ItemState
Enumerator | |
---|---|
AfterSave | Returns the last saved item. |
BeforeSave | Returns an item with the original payload before the last save call. |
Definition at line 58 of file editoritemmanager.h.
◆ ItipPrivacy
enum IncidenceEditorNG::EditorItemManager::ItipPrivacy |
Definition at line 39 of file editoritemmanager.h.
◆ SaveAction
Definition at line 81 of file editoritemmanager.h.
Constructor & Destructor Documentation
◆ EditorItemManager()
|
explicit |
Creates an ItemEditor for a new Item.
ItemEditor.
Receives an option IncidenceChanger, so you can share the undo/redo stack with your application.
Definition at line 266 of file editoritemmanager.cpp.
◆ ~EditorItemManager()
|
overridedefault |
Destructs the ItemEditor.
Unsaved changes will get lost at this point.
Member Function Documentation
◆ item()
|
nodiscard |
Returns the last saved item with payload or an invalid item when save is not called yet.
Definition at line 275 of file editoritemmanager.cpp.
◆ load()
void IncidenceEditorNG::EditorItemManager::load | ( | const Akonadi::Item & | item | ) |
Loads the.
- Parameters
-
item into the editor. The item passed must be a valid item.
Definition at line 301 of file editoritemmanager.cpp.
◆ save()
void IncidenceEditorNG::EditorItemManager::save | ( | ItipPrivacyFlags | itipPrivacy = ItipPrivacyPlain | ) |
Saves the new or modified item.
This method does nothing when the ui is not dirty.
Definition at line 313 of file editoritemmanager.cpp.
◆ setIsCounterProposal()
void IncidenceEditorNG::EditorItemManager::setIsCounterProposal | ( | bool | isCounterProposal | ) |
Definition at line 366 of file editoritemmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:15 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.