KGantt::DateTimeTimeLine
#include <kganttdatetimetimeline.h>
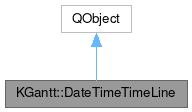
Public Types | |
enum | Option { Foreground = 1 , Background = 2 , UseCustomPen = 4 , MaxOptions = 0xFFFF } |
typedef QFlags< Option > | Options |
![]() | |
typedef | QObjectList |
Signals | |
void | updated () |
Public Member Functions | |
DateTimeTimeLine () | |
QPen | customPen () const |
QDateTime | dateTime () const |
int | interval () const |
DateTimeTimeLine::Options | options () const |
QPen | pen () const |
void | setDateTime (const QDateTime &dt) |
void | setInterval (int msec) |
void | setOptions (DateTimeTimeLine::Options options) |
void | setPen (const QPen &pen) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class implements a timeline.
The timeline can optionally be shown in the Background or in the Foreground. Default is Foreground.
The pen can be set with setPen(), and must be activated by setting the option UseCustomPen.
The datetime can be set using setDateTime().
The timeline can priodically moved to the current datetime by setting the interval > 0 with setInterval(). Setting a zero interval turns the periodically update off.
The timeline is off by default.
For example:
Member Typedef Documentation
◆ Options
Definition at line 58 of file kganttdatetimetimeline.h.
Member Enumeration Documentation
◆ Option
Enumerator | |
---|---|
Foreground | Display the timeline in the foreground. |
Background | Display the timeline in the background. |
UseCustomPen | Paint the timeline using the pen set with setPen(). |
Definition at line 52 of file kganttdatetimetimeline.h.
Constructor & Destructor Documentation
◆ DateTimeTimeLine()
DateTimeTimeLine::DateTimeTimeLine | ( | ) |
Create a timeline object.
By default, no timeline is displayed.
Definition at line 40 of file kganttdatetimetimeline.cpp.
Member Function Documentation
◆ customPen()
QPen DateTimeTimeLine::customPen | ( | ) | const |
- Returns
- the pen that has been set with setPen()
Definition at line 112 of file kganttdatetimetimeline.cpp.
◆ dateTime()
QDateTime DateTimeTimeLine::dateTime | ( | ) | const |
- Returns
- the datetime If the datetime is not valid, the current datetime is returned.
Definition at line 66 of file kganttdatetimetimeline.cpp.
◆ interval()
int DateTimeTimeLine::interval | ( | ) | const |
- Returns
- the update interval in milliseconds
Definition at line 79 of file kganttdatetimetimeline.cpp.
◆ options()
DateTimeTimeLine::Options DateTimeTimeLine::options | ( | ) | const |
- Returns
- options
Definition at line 50 of file kganttdatetimetimeline.cpp.
◆ pen()
QPen DateTimeTimeLine::pen | ( | ) | const |
- Returns
- the pen that will be used for rendering the timeline If no pen has been set with setPen(), a default pen is returned.
Definition at line 96 of file kganttdatetimetimeline.cpp.
◆ setDateTime()
void DateTimeTimeLine::setDateTime | ( | const QDateTime & | dt | ) |
Set datetime to dt
.
Definition at line 72 of file kganttdatetimetimeline.cpp.
◆ setInterval()
void DateTimeTimeLine::setInterval | ( | int | msec | ) |
Set timer interval to msecs
milliseconds.
Setting a zero time disables the timer.
Definition at line 85 of file kganttdatetimetimeline.cpp.
◆ setOptions()
void DateTimeTimeLine::setOptions | ( | DateTimeTimeLine::Options | options | ) |
Set options to options
.
If both Background and Foreground are set, Foreground is used.
Definition at line 56 of file kganttdatetimetimeline.cpp.
◆ setPen()
void DateTimeTimeLine::setPen | ( | const QPen & | pen | ) |
Set the custom pen to pen
.
Definition at line 105 of file kganttdatetimetimeline.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:09:26 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.