KGantt::GraphicsScene
#include <kganttgraphicsscene.h>
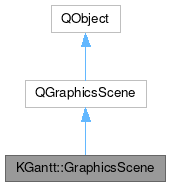
Signals | |
void | clicked (const QModelIndex &index) |
void | entered (const QModelIndex &index) |
void | gridChanged () |
void | pressed (const QModelIndex &index) |
void | qrealClicked (const QModelIndex &index) |
Public Slots | |
void | setConstraintModel (KGantt::ConstraintModel *) |
void | setModel (QAbstractItemModel *) |
void | setReadOnly (bool) |
void | setRootIndex (const QModelIndex &idx) |
void | setSelectionModel (QItemSelectionModel *selectionmodel) |
void | setSummaryHandlingModel (QAbstractProxyModel *) |
Public Member Functions | |
GraphicsScene (QObject *parent=nullptr) | |
void | clearItems () |
ConstraintModel * | constraintModel () const |
GraphicsItem * | createItem (ItemType type) const |
void | deleteSubtree (const QModelIndex &) |
GraphicsItem * | dragSource () const |
ConstraintGraphicsItem * | findConstraintItem (const Constraint &) const |
QList< ConstraintGraphicsItem * > | findConstraintItems (const QModelIndex &idx) const |
GraphicsItem * | findItem (const QModelIndex &) const |
GraphicsItem * | findItem (const QPersistentModelIndex &) const |
const AbstractGrid * | getGrid () const |
AbstractGrid * | grid () const |
void | insertItem (const QPersistentModelIndex &, GraphicsItem *) |
bool | isReadOnly () const |
void | itemClicked (const QModelIndex &) |
ItemDelegate * | itemDelegate () const |
void | itemDoubleClicked (const QModelIndex &) |
void | itemEntered (const QModelIndex &) |
void | itemPressed (const QModelIndex &idx, QGraphicsSceneMouseEvent *event) |
QAbstractItemModel * | model () const |
void | print (QPainter *painter, const QRectF &target=QRectF(), bool drawRowLabels=true, bool drawColumnLabels=true) |
void | print (QPainter *painter, qreal start, qreal end, const QRectF &target=QRectF(), bool drawRowLabels=true, bool drawColumnLabels=true) |
void | print (QPrinter *printer, bool drawRowLabels=true, bool drawColumnLabels=true) |
void | print (QPrinter *printer, qreal start, qreal end, bool drawRowLabels=true, bool drawColumnLabels=true) |
void | printDiagram (QPrinter *printer, const PrintingContext &context) |
void | removeItem (const QModelIndex &) |
void | removeItem (QGraphicsItem *item) |
QModelIndex | rootIndex () const |
AbstractRowController * | rowController () const |
QItemSelectionModel * | selectionModel () const |
void | setDragSource (GraphicsItem *item) |
void | setGrid (AbstractGrid *grid) |
void | setItemDelegate (ItemDelegate *) |
void | setRowController (AbstractRowController *rc) |
QAbstractProxyModel * | summaryHandlingModel () const |
AbstractGrid * | takeGrid () |
void | updateItems () |
void | updateRow (const QModelIndex &idx) |
![]() | |
QGraphicsScene (const QRectF &sceneRect, QObject *parent) | |
QGraphicsScene (QObject *parent) | |
QGraphicsScene (qreal x, qreal y, qreal width, qreal height, QObject *parent) | |
QGraphicsItem * | activePanel () const const |
QGraphicsWidget * | activeWindow () const const |
QGraphicsEllipseItem * | addEllipse (const QRectF &rect, const QPen &pen, const QBrush &brush) |
QGraphicsEllipseItem * | addEllipse (qreal x, qreal y, qreal w, qreal h, const QPen &pen, const QBrush &brush) |
void | addItem (QGraphicsItem *item) |
QGraphicsLineItem * | addLine (const QLineF &line, const QPen &pen) |
QGraphicsLineItem * | addLine (qreal x1, qreal y1, qreal x2, qreal y2, const QPen &pen) |
QGraphicsPathItem * | addPath (const QPainterPath &path, const QPen &pen, const QBrush &brush) |
QGraphicsPixmapItem * | addPixmap (const QPixmap &pixmap) |
QGraphicsPolygonItem * | addPolygon (const QPolygonF &polygon, const QPen &pen, const QBrush &brush) |
QGraphicsRectItem * | addRect (const QRectF &rect, const QPen &pen, const QBrush &brush) |
QGraphicsRectItem * | addRect (qreal x, qreal y, qreal w, qreal h, const QPen &pen, const QBrush &brush) |
QGraphicsSimpleTextItem * | addSimpleText (const QString &text, const QFont &font) |
QGraphicsTextItem * | addText (const QString &text, const QFont &font) |
QGraphicsProxyWidget * | addWidget (QWidget *widget, Qt::WindowFlags wFlags) |
void | advance () |
QBrush | backgroundBrush () const const |
int | bspTreeDepth () const const |
void | changed (const QList< QRectF > ®ion) |
void | clear () |
void | clearFocus () |
void | clearSelection () |
QList< QGraphicsItem * > | collidingItems (const QGraphicsItem *item, Qt::ItemSelectionMode mode) const const |
QGraphicsItemGroup * | createItemGroup (const QList< QGraphicsItem * > &items) |
void | destroyItemGroup (QGraphicsItemGroup *group) |
QGraphicsItem * | focusItem () const const |
void | focusItemChanged (QGraphicsItem *newFocusItem, QGraphicsItem *oldFocusItem, Qt::FocusReason reason) |
bool | focusOnTouch () const const |
QFont | font () const const |
QBrush | foregroundBrush () const const |
bool | hasFocus () const const |
qreal | height () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | invalidate (const QRectF &rect, SceneLayers layers) |
void | invalidate (qreal x, qreal y, qreal w, qreal h, SceneLayers layers) |
bool | isActive () const const |
QGraphicsItem * | itemAt (const QPointF &position, const QTransform &deviceTransform) const const |
QGraphicsItem * | itemAt (qreal x, qreal y, const QTransform &deviceTransform) const const |
ItemIndexMethod | itemIndexMethod () const const |
QList< QGraphicsItem * > | items (const QPainterPath &path, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const const |
QList< QGraphicsItem * > | items (const QPointF &pos, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const const |
QList< QGraphicsItem * > | items (const QPolygonF &polygon, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const const |
QList< QGraphicsItem * > | items (const QRectF &rect, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const const |
QList< QGraphicsItem * > | items (qreal x, qreal y, qreal w, qreal h, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const const |
QList< QGraphicsItem * > | items (Qt::SortOrder order) const const |
QRectF | itemsBoundingRect () const const |
qreal | minimumRenderSize () const const |
QGraphicsItem * | mouseGrabberItem () const const |
QPalette | palette () const const |
void | removeItem (QGraphicsItem *item) |
void | render (QPainter *painter, const QRectF &target, const QRectF &source, Qt::AspectRatioMode aspectRatioMode) |
QRectF | sceneRect () const const |
void | sceneRectChanged (const QRectF &rect) |
QList< QGraphicsItem * > | selectedItems () const const |
QPainterPath | selectionArea () const const |
void | selectionChanged () |
bool | sendEvent (QGraphicsItem *item, QEvent *event) |
void | setActivePanel (QGraphicsItem *item) |
void | setActiveWindow (QGraphicsWidget *widget) |
void | setBackgroundBrush (const QBrush &brush) |
void | setBspTreeDepth (int depth) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusItem (QGraphicsItem *item, Qt::FocusReason focusReason) |
void | setFocusOnTouch (bool enabled) |
void | setFont (const QFont &font) |
void | setForegroundBrush (const QBrush &brush) |
void | setItemIndexMethod (ItemIndexMethod method) |
void | setMinimumRenderSize (qreal minSize) |
void | setPalette (const QPalette &palette) |
void | setSceneRect (const QRectF &rect) |
void | setSceneRect (qreal x, qreal y, qreal w, qreal h) |
void | setSelectionArea (const QPainterPath &path, const QTransform &deviceTransform) |
void | setSelectionArea (const QPainterPath &path, Qt::ItemSelectionOperation selectionOperation, Qt::ItemSelectionMode mode, const QTransform &deviceTransform) |
void | setStickyFocus (bool enabled) |
void | setStyle (QStyle *style) |
bool | stickyFocus () const const |
QStyle * | style () const const |
void | update (const QRectF &rect) |
void | update (qreal x, qreal y, qreal w, qreal h) |
QList< QGraphicsView * > | views () const const |
qreal | width () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QModelIndex | dataIndex (const QModelIndex &idx) |
static QModelIndex | mainIndex (const QModelIndex &idx) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
void | drawBackground (QPainter *painter, const QRectF &rect) override |
void | drawForeground (QPainter *painter, const QRectF &rect) override |
void | helpEvent (QGraphicsSceneHelpEvent *helpEvent) override |
![]() | |
virtual void | contextMenuEvent (QGraphicsSceneContextMenuEvent *contextMenuEvent) |
virtual void | dragEnterEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | dragLeaveEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | dragMoveEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | drawItems (QPainter *painter, int numItems, QGraphicsItem *[] items, const QStyleOptionGraphicsItem[] options, QWidget *widget) |
virtual void | dropEvent (QGraphicsSceneDragDropEvent *event) |
virtual bool | event (QEvent *event) override |
virtual bool | eventFilter (QObject *watched, QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *focusEvent) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *focusEvent) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *keyEvent) |
virtual void | keyReleaseEvent (QKeyEvent *keyEvent) |
virtual void | mouseDoubleClickEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mouseMoveEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mousePressEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mouseReleaseEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | wheelEvent (QGraphicsSceneWheelEvent *wheelEvent) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ItemIndexMethod |
enum | SceneLayer |
![]() | |
backgroundBrush | |
bspTreeDepth | |
focusOnTouch | |
font | |
foregroundBrush | |
itemIndexMethod | |
minimumRenderSize | |
palette | |
sceneRect | |
stickyFocus | |
![]() | |
objectName | |
![]() | |
AllLayers | |
BackgroundLayer | |
BspTreeIndex | |
ForegroundLayer | |
ItemLayer | |
NoIndex | |
typedef | SceneLayers |
![]() | |
typedef | QObjectList |
Detailed Description
Definition at line 43 of file kganttgraphicsscene.h.
Constructor & Destructor Documentation
◆ GraphicsScene()
|
explicit |
Definition at line 192 of file kganttgraphicsscene.cpp.
◆ ~GraphicsScene()
|
override |
Definition at line 198 of file kganttgraphicsscene.cpp.
Member Function Documentation
◆ clearItems()
void GraphicsScene::clearItems | ( | ) |
Definition at line 554 of file kganttgraphicsscene.cpp.
◆ constraintModel()
ConstraintModel * GraphicsScene::constraintModel | ( | ) | const |
Definition at line 264 of file kganttgraphicsscene.cpp.
◆ createItem()
GraphicsItem * GraphicsScene::createItem | ( | ItemType | type | ) | const |
Creates a new item of type type.
Definition at line 395 of file kganttgraphicsscene.cpp.
◆ dataIndex()
|
static |
Returns the index pointing to the last column in the same row as idx. This can be thought of as in "inverse" of mainIndex()
Definition at line 380 of file kganttgraphicsscene.cpp.
◆ deleteSubtree()
void GraphicsScene::deleteSubtree | ( | const QModelIndex & | _idx | ) |
Definition at line 570 of file kganttgraphicsscene.cpp.
◆ dragSource()
GraphicsItem * GraphicsScene::dragSource | ( | ) | const |
Definition at line 717 of file kganttgraphicsscene.cpp.
◆ drawBackground()
|
overrideprotectedvirtual |
Reimplemented from QGraphicsScene.
Definition at line 646 of file kganttgraphicsscene.cpp.
◆ drawForeground()
|
overrideprotectedvirtual |
Reimplemented from QGraphicsScene.
Definition at line 678 of file kganttgraphicsscene.cpp.
◆ findConstraintItem()
ConstraintGraphicsItem * GraphicsScene::findConstraintItem | ( | const Constraint & | c | ) | const |
Definition at line 586 of file kganttgraphicsscene.cpp.
◆ findItem() [1/2]
GraphicsItem * GraphicsScene::findItem | ( | const QModelIndex & | idx | ) | const |
Definition at line 538 of file kganttgraphicsscene.cpp.
◆ findItem() [2/2]
GraphicsItem * GraphicsScene::findItem | ( | const QPersistentModelIndex & | idx | ) | const |
Definition at line 546 of file kganttgraphicsscene.cpp.
◆ getGrid()
const AbstractGrid * GraphicsScene::getGrid | ( | ) | const |
- Returns
- the current grid.
Definition at line 346 of file kganttgraphicsscene.cpp.
◆ grid()
AbstractGrid * GraphicsScene::grid | ( | ) | const |
- Returns
- the grid set with setGrid(). Note: Returns nullptr if no grid has been set.
Definition at line 340 of file kganttgraphicsscene.cpp.
◆ helpEvent()
|
overrideprotectedvirtual |
Reimplemented from QGraphicsScene.
Definition at line 632 of file kganttgraphicsscene.cpp.
◆ insertItem()
void GraphicsScene::insertItem | ( | const QPersistentModelIndex & | idx, |
GraphicsItem * | item ) |
Definition at line 478 of file kganttgraphicsscene.cpp.
◆ isReadOnly()
bool GraphicsScene::isReadOnly | ( | ) | const |
Definition at line 356 of file kganttgraphicsscene.cpp.
◆ itemClicked()
void GraphicsScene::itemClicked | ( | const QModelIndex & | idx | ) |
Definition at line 702 of file kganttgraphicsscene.cpp.
◆ itemDelegate()
ItemDelegate * GraphicsScene::itemDelegate | ( | ) | const |
Definition at line 224 of file kganttgraphicsscene.cpp.
◆ itemDoubleClicked()
void GraphicsScene::itemDoubleClicked | ( | const QModelIndex & | idx | ) |
Definition at line 707 of file kganttgraphicsscene.cpp.
◆ itemEntered()
void GraphicsScene::itemEntered | ( | const QModelIndex & | idx | ) |
Definition at line 683 of file kganttgraphicsscene.cpp.
◆ itemPressed()
void GraphicsScene::itemPressed | ( | const QModelIndex & | idx, |
QGraphicsSceneMouseEvent * | event ) |
Definition at line 688 of file kganttgraphicsscene.cpp.
◆ mainIndex()
|
static |
Definition at line 366 of file kganttgraphicsscene.cpp.
◆ model()
QAbstractItemModel * GraphicsScene::model | ( | ) | const |
Definition at line 229 of file kganttgraphicsscene.cpp.
◆ print() [1/4]
void GraphicsScene::print | ( | QPainter * | painter, |
const QRectF & | target = QRectF(), | ||
bool | drawRowLabels = true, | ||
bool | drawColumnLabels = true ) |
Render the GanttView inside the rectangle target using the painter painter. If drawRowLabels is true (the default), each row will have it's label printed on the left side. If drawColumnLabels is true (the default), each column will have it's label printed at the top side.
Definition at line 755 of file kganttgraphicsscene.cpp.
◆ print() [2/4]
void GraphicsScene::print | ( | QPainter * | painter, |
qreal | start, | ||
qreal | end, | ||
const QRectF & | target = QRectF(), | ||
bool | drawRowLabels = true, | ||
bool | drawColumnLabels = true ) |
Render the GanttView inside the rectangle target using the painter painter. If drawRowLabels is true (the default), each row will have it's label printed on the left side. If drawColumnLabels is true (the default), each column will have it's label printed at the top side.
To print a certain range of a chart with a DateTimeGrid, use qreal DateTimeGrid::mapFromDateTime( const QDateTime& dt) const to figure out the values for start and end.
Definition at line 766 of file kganttgraphicsscene.cpp.
◆ print() [3/4]
void GraphicsScene::print | ( | QPrinter * | printer, |
bool | drawRowLabels = true, | ||
bool | drawColumnLabels = true ) |
Print the Gantt chart using printer. If drawRowLabels is true (the default), each row will have it's label printed on the left side. If drawColumnLabels is true (the default), each column will have it's label printed at the top side.
This version of print() will print multiple pages.
Definition at line 723 of file kganttgraphicsscene.cpp.
◆ print() [4/4]
void GraphicsScene::print | ( | QPrinter * | printer, |
qreal | start, | ||
qreal | end, | ||
bool | drawRowLabels = true, | ||
bool | drawColumnLabels = true ) |
Print part of the Gantt chart from start to end using printer. If drawRowLabels is true (the default), each row will have it's label printed on the left side. If drawColumnLabels is true (the default), each column will have it's label printed at the top side.
This version of print() will print multiple pages.
To print a certain range of a chart with a DateTimeGrid, use qreal DateTimeGrid::mapFromDateTime( const QDateTime& dt) const to figure out the values for start and end.
Definition at line 738 of file kganttgraphicsscene.cpp.
◆ printDiagram()
void GraphicsScene::printDiagram | ( | QPrinter * | printer, |
const PrintingContext & | context ) |
Print the Gantt chart on the printer in accordance with the PrintingContext context
- See also
- PrintingContext
- Since
- 2.8.0
Definition at line 777 of file kganttgraphicsscene.cpp.
◆ removeItem()
void GraphicsScene::removeItem | ( | const QModelIndex & | idx | ) |
Definition at line 513 of file kganttgraphicsscene.cpp.
◆ rootIndex()
QModelIndex GraphicsScene::rootIndex | ( | ) | const |
Definition at line 259 of file kganttgraphicsscene.cpp.
◆ rowController()
AbstractRowController * GraphicsScene::rowController | ( | ) | const |
Definition at line 308 of file kganttgraphicsscene.cpp.
◆ selectionModel()
QItemSelectionModel * GraphicsScene::selectionModel | ( | ) | const |
Definition at line 298 of file kganttgraphicsscene.cpp.
◆ setConstraintModel
|
slot |
Definition at line 269 of file kganttgraphicsscene.cpp.
◆ setDragSource()
void GraphicsScene::setDragSource | ( | GraphicsItem * | item | ) |
Definition at line 712 of file kganttgraphicsscene.cpp.
◆ setGrid()
void GraphicsScene::setGrid | ( | AbstractGrid * | grid | ) |
Set the grid to grid
.
The current grid (if set) is deleted. If grid
is nullptr, the scene reverts to use the default_grid
Definition at line 325 of file kganttgraphicsscene.cpp.
◆ setItemDelegate()
void GraphicsScene::setItemDelegate | ( | ItemDelegate * | delegate | ) |
Definition at line 217 of file kganttgraphicsscene.cpp.
◆ setModel
|
slot |
Definition at line 235 of file kganttgraphicsscene.cpp.
◆ setReadOnly
|
slot |
Definition at line 351 of file kganttgraphicsscene.cpp.
◆ setRootIndex
|
slot |
Definition at line 254 of file kganttgraphicsscene.cpp.
◆ setRowController()
void GraphicsScene::setRowController | ( | AbstractRowController * | rc | ) |
Definition at line 303 of file kganttgraphicsscene.cpp.
◆ setSelectionModel
|
slot |
Definition at line 284 of file kganttgraphicsscene.cpp.
◆ setSummaryHandlingModel
|
slot |
Definition at line 248 of file kganttgraphicsscene.cpp.
◆ summaryHandlingModel()
QAbstractProxyModel * GraphicsScene::summaryHandlingModel | ( | ) | const |
Definition at line 243 of file kganttgraphicsscene.cpp.
◆ takeGrid()
AbstractGrid * GraphicsScene::takeGrid | ( | ) |
- Returns
- the grid set with setGrid() Note: Returns nullptr if no grid has been set. The scene reverts to use the default_grid.
Definition at line 313 of file kganttgraphicsscene.cpp.
◆ updateItems()
void GraphicsScene::updateItems | ( | ) |
Definition at line 559 of file kganttgraphicsscene.cpp.
◆ updateRow()
void GraphicsScene::updateRow | ( | const QModelIndex & | idx | ) |
Definition at line 428 of file kganttgraphicsscene.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 14 2025 11:51:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.