KUnifiedPush::AbstractPushProvider
#include <abstractpushprovider.h>
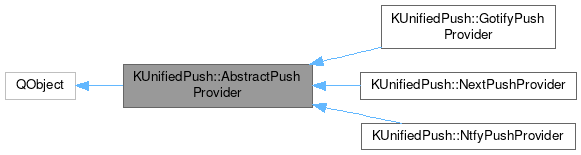
Public Types | |
enum | Error { NoError , ProviderRejected , TransientNetworkError } |
Signals | |
void | clientRegistered (const KUnifiedPush::Client &client, KUnifiedPush::AbstractPushProvider::Error error=NoError, const QString &errorMsg={}) |
void | clientUnregistered (const KUnifiedPush::Client &client, KUnifiedPush::AbstractPushProvider::Error error=NoError) |
void | connected () |
void | disconnected (KUnifiedPush::AbstractPushProvider::Error error, const QString &errorMsg={}) |
void | messageAcknowledged (const KUnifiedPush::Client &client, const QString &messageIdentifier) |
void | messageReceived (const KUnifiedPush::Message &msg) |
void | urgencyChanged () |
Public Member Functions | |
virtual void | acknowledgeMessage (const Client &client, const QString &messageIdentifier) |
void | changeUrgency (Urgency urgency) |
virtual void | connectToProvider (Urgency urgency)=0 |
virtual void | disconnectFromProvider ()=0 |
virtual bool | loadSettings (const QSettings &settings)=0 |
QLatin1StringView | providerId () const |
virtual void | registerClient (const Client &client)=0 |
virtual void | resetSettings (QSettings &settings) |
virtual void | unregisterClient (const Client &client)=0 |
Urgency | urgency () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
AbstractPushProvider (QLatin1StringView providerId, QObject *parent) | |
virtual void | doChangeUrgency (Urgency urgency) |
QNetworkAccessManager * | nam () |
void | setUrgency (Urgency urgency) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Base class for push provider protocol implementations.
Needed to support different push providers as that part of the protocol is not part of the UnifiedPush spec.
Definition at line 25 of file abstractpushprovider.h.
Member Enumeration Documentation
◆ Error
Enumerator | |
---|---|
NoError | operation succeeded |
ProviderRejected | communication worked, but the provider refused to complete the operation |
TransientNetworkError | temporary network error, try again |
Definition at line 31 of file abstractpushprovider.h.
Constructor & Destructor Documentation
◆ AbstractPushProvider()
|
explicitprotected |
Definition at line 13 of file abstractpushprovider.cpp.
Member Function Documentation
◆ acknowledgeMessage()
|
virtual |
Acknowledge a message.
The default implementation does nothing apart from indicating successful completion.
Reimplemented in KUnifiedPush::AutopushProvider, and KUnifiedPush::NextPushProvider.
Definition at line 25 of file abstractpushprovider.cpp.
◆ changeUrgency()
void AbstractPushProvider::changeUrgency | ( | Urgency | urgency | ) |
Change urgency level as needed.
Reimplement doChangeUrgency if your provider does this as a separate command.
Definition at line 30 of file abstractpushprovider.cpp.
◆ clientRegistered
|
signal |
Emitted after successful client registration.
◆ clientUnregistered
|
signal |
Emitted after successful client unregistration.
◆ connected
|
signal |
Emitted after the connection to the push provider has been established successfully.
◆ connectToProvider()
|
pure virtual |
Attempt to establish a connection to the push provider.
Implemented in KUnifiedPush::AutopushProvider, KUnifiedPush::GotifyPushProvider, KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
◆ disconnected
|
signal |
Emitted after the connection to the push provider disconnected or failed to be established.
◆ disconnectFromProvider()
|
pure virtual |
Disconnect and existing connection to the push provider.
Implemented in KUnifiedPush::AutopushProvider, KUnifiedPush::GotifyPushProvider, KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
◆ doChangeUrgency()
|
protectedvirtual |
Re-implement if urgency leve changes are done as a separate command.
The default implementation assumes urgency levels aren't supported by this provider.
Reimplemented in KUnifiedPush::AutopushProvider, and KUnifiedPush::NextPushProvider.
Definition at line 40 of file abstractpushprovider.cpp.
◆ loadSettings()
|
pure virtual |
Load connection settings.
- Parameters
-
settings can be read on the top level, the correct group is already selected.
- Returns
true
if the settings are valid,false
otherwise.
Implemented in KUnifiedPush::AutopushProvider, KUnifiedPush::GotifyPushProvider, KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
◆ messageAcknowledged
|
signal |
Emitted after a message reception has been acknowledge to the push server.
◆ messageReceived
|
signal |
Inform about a received push notification.
◆ nam()
|
protected |
Definition at line 56 of file abstractpushprovider.cpp.
◆ providerId()
|
nodiscard |
◆ registerClient()
|
pure virtual |
Register a new client with the provider.
Implemented in KUnifiedPush::AutopushProvider, KUnifiedPush::GotifyPushProvider, KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
◆ resetSettings()
|
virtual |
Reset any internal state for a fresh setup connecting to a different push server instance.
The default implementation does nothing.
Reimplemented in KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
Definition at line 21 of file abstractpushprovider.cpp.
◆ setUrgency()
|
inlineprotected |
Definition at line 107 of file abstractpushprovider.h.
◆ unregisterClient()
|
pure virtual |
Unregister a client from the provider.
Implemented in KUnifiedPush::AutopushProvider, KUnifiedPush::GotifyPushProvider, KUnifiedPush::NextPushProvider, and KUnifiedPush::NtfyPushProvider.
◆ urgency()
|
nodiscard |
The urgency level currently used by this provider.
This might not yet be the requested one if changing that is an asynchronous operation.
Definition at line 51 of file abstractpushprovider.cpp.
◆ urgencyChanged
|
signal |
Emitted when the urgency level change request has been executed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 12:05:39 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.