KSaneIface::KSaneWidget
#include <ksanewidget.h>
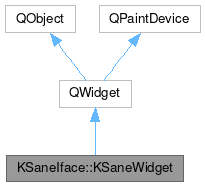
Public Types | |
enum | ScanStatus { NoError , ErrorCannotSegment , ErrorGeneral , Information } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | availableDevices (const QList< KSaneIface::KSaneWidget::DeviceInfo > &deviceList) |
void | buttonPressed (const QString &optionName, const QString &optionLabel, bool pressed) |
void | openedDeviceInfoUpdated (const QString &deviceName, const QString &deivceVendor, const QString &deviceModel) |
void | scanDone (int status, const QString &strStatus) |
void | scannedImageReady (const QImage &scannedImage) |
void | scanProgress (int percent) |
void | userMessage (int type, const QString &strStatus) |
Public Slots | |
void | cancelScan () |
void | startPreviewScan () |
void | startScan () |
Public Member Functions | |
KSaneWidget (QWidget *parent=nullptr) | |
~KSaneWidget () override | |
bool | closeDevice () |
QString | deviceModel () const |
QString | deviceName () const |
QString | deviceVendor () const |
void | enableAutoSelect (bool enable) |
bool | getOptionValue (const QString &option, QString &value) |
void | getOptionValues (QMap< QString, QString > &options) |
bool | openDevice (const QString &device_name) |
float | scanAreaHeight () |
float | scanAreaWidth () |
QString | selectDevice (QWidget *parent=nullptr) |
bool | setOptionValue (const QString &option, const QString &value) |
int | setOptionValues (const QMap< QString, QString > &options) |
void | setPreviewResolution (float dpi) |
void | setSelection (QPointF topLeft, QPointF bottomRight) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Detailed Description
This class provides the widget containing the scan options and the preview.
Definition at line 26 of file ksanewidget.h.
Member Enumeration Documentation
◆ ScanStatus
- Note
- There might come more enumerations in the future.
Definition at line 34 of file ksanewidget.h.
Constructor & Destructor Documentation
◆ KSaneWidget()
KSaneIface::KSaneWidget::KSaneWidget | ( | QWidget * | parent = nullptr | ) |
This constructor initializes the private class variables, but the widget is left empty.
The options and the preview are added with the call to openDevice().
Definition at line 38 of file ksanewidget.cpp.
◆ ~KSaneWidget()
|
override |
Standard destructor.
Definition at line 204 of file ksanewidget.cpp.
Member Function Documentation
◆ availableDevices
|
signal |
This signal is emitted every time the device list is updated or after initGetDeviceList() is called.
- Parameters
-
deviceList is a QList of KSaneWidget::DeviceInfo that contain the device name, model, vendor and type of the attached scanners.
- Note
- The list is only a snapshot of the current available devices. Devices might be added or removed/opened after the signal is emitted.
◆ buttonPressed
|
signal |
This Signal is emitted when a hardware button is pressed.
- Parameters
-
optionName is the untranslated technical name of the sane-option. optionLabel is the translated user visible label of the sane-option. pressed indicates if the value is true or false.
- Note
- The SANE standard does not specify hardware buttons and their behaviors, so this signal is emitted for sane-options that behave like hardware buttons. That is the sane-options are read-only and type boolean. The naming of hardware buttons also differ from backend to backend.
◆ cancelScan
|
slot |
This method can be used to cancel a scan or prevent an automatic new scan.
Definition at line 363 of file ksanewidget.cpp.
◆ closeDevice()
bool KSaneIface::KSaneWidget::closeDevice | ( | ) |
This method closes the currently open scanner device.
- Returns
- 'true' if all goes well and 'false' if no device is open.
Definition at line 326 of file ksanewidget.cpp.
◆ deviceModel()
QString KSaneIface::KSaneWidget::deviceModel | ( | ) | const |
This method returns the model of the currently opened scanner.
Definition at line 220 of file ksanewidget.cpp.
◆ deviceName()
QString KSaneIface::KSaneWidget::deviceName | ( | ) | const |
This method returns the internal device name of the currently opened scanner.
Definition at line 210 of file ksanewidget.cpp.
◆ deviceVendor()
QString KSaneIface::KSaneWidget::deviceVendor | ( | ) | const |
This method returns the vendor name of the currently opened scanner.
Definition at line 215 of file ksanewidget.cpp.
◆ enableAutoSelect()
void KSaneIface::KSaneWidget::enableAutoSelect | ( | bool | enable | ) |
This function can be used to enable/disable automatic selections on previews.
The default state is enabled.
- Parameters
-
enable specifies if the auto selection should be turned on or off.
Definition at line 458 of file ksanewidget.cpp.
◆ getOptionValue()
This function reads one parameter value into a string.
- Parameters
-
optname is the name of the parameter to read. value is the string representation of the value.
- Returns
- this function returns true if the read was successful.
Definition at line 380 of file ksanewidget.cpp.
◆ getOptionValues()
This method reads the available parameters and their values and returns them in a QMap (Name, value)
- Parameters
-
opts is a QMap with the parameter names and values.
Definition at line 374 of file ksanewidget.cpp.
◆ openDevice()
bool KSaneIface::KSaneWidget::openDevice | ( | const QString & | device_name | ) |
This method opens the specified scanner device and adds the scan options to the KSane widget.
- Parameters
-
device_name is the libsane device name for the scanner to open.
- Returns
- 'true' if all goes well and 'false' if the specified scanner can not be opened.
Definition at line 242 of file ksanewidget.cpp.
◆ openedDeviceInfoUpdated
|
signal |
This signal is not emitted anymore.
◆ scanAreaHeight()
float KSaneIface::KSaneWidget::scanAreaHeight | ( | ) |
This method returns the scan area's height in mm.
- Returns
- Height of the scannable area in mm
Definition at line 485 of file ksanewidget.cpp.
◆ scanAreaWidth()
float KSaneIface::KSaneWidget::scanAreaWidth | ( | ) |
This method returns the scan area's width in mm.
- Returns
- Width of the scannable area in mm
Definition at line 463 of file ksanewidget.cpp.
◆ scanDone
|
signal |
This signal is emitted when the scanning has ended.
- Parameters
-
status contains a ScanStatus status code. strStatus If an error has occurred this string will contain an error message. otherwise the string is empty.
◆ scannedImageReady
|
signal |
This signal is emitted when a final scan is ready.
- Parameters
-
scannedImage is the QImage containing the scanned image data.
◆ scanProgress
|
signal |
This Signal is emitted for progress information during a scan.
The GUI already has a progress bar, but if the GUI is hidden, this can be used to display a progress bar.
- Parameters
-
percent is the percentage of the scan progress (0-100).
◆ selectDevice()
This helper method displays a dialog for selecting a scanner.
The libsane device name of the selected scanner device is returned.
Definition at line 225 of file ksanewidget.cpp.
◆ setOptionValue()
This function writes one parameter value into a string.
- Parameters
-
optname is the name of the parameter to write. value is the string representation of the value.
- Returns
- this function returns true if the write was successful and false if it was unsuccessful or scanning is in progress.
Definition at line 421 of file ksanewidget.cpp.
◆ setOptionValues()
This method can be used to write many parameter values at once.
- Parameters
-
opts is a QMap with the parameter names and values.
- Returns
- This function returns the number of successful writes or -1 if scanning is in progress.
Definition at line 394 of file ksanewidget.cpp.
◆ setPreviewResolution()
void KSaneIface::KSaneWidget::setPreviewResolution | ( | float | dpi | ) |
This function is used to set the preferred resolution for scanning the preview.
- Parameters
-
dpi is the wanted scan resolution for the preview
- Note
- if the set value is not supported, the cloasest one is used
- setting the value 0 means that the default calculated value should be used
Definition at line 369 of file ksanewidget.cpp.
◆ setSelection()
This method sets the selection according to the given points.
- Note
- The points are defined with respect to the scan areas top-left corner in mm
- Parameters
-
topLeft Upper left corner of the selection (in mm) bottomRight Lower right corner of the selection (in mm)
Definition at line 507 of file ksanewidget.cpp.
◆ startPreviewScan
|
slot |
This method can be used to start a preview scan.
Definition at line 352 of file ksanewidget.cpp.
◆ startScan
|
slot |
This method can be used to start a scan (if no GUI is needed).
- Note
- libksane may return one or more images as a result of one invocation of this slot. If no more images are wanted cancelScan should be called in the slot handling the imageReady signal.
Definition at line 341 of file ksanewidget.cpp.
◆ userMessage
|
signal |
This signal is emitted when the user is to be notified about something.
- Note
- If no slot is connected to this signal the message will be displayed in a KMessageBox.
- Parameters
-
type contains a ScanStatus code to identify the type of message (error/info/...). strStatus If an error has occurred this string will contain an error message. otherwise the string is empty.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 12:03:00 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.