KDecoration3::DecorationSettings
#include <decorationsettings.h>
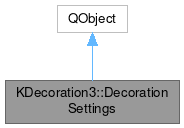
Properties | |
bool | alphaChannelSupported |
KDecoration3::BorderSize | borderSize |
bool | closeOnDoubleClickOnMenu |
QList< KDecoration3::DecorationButtonType > | decorationButtonsLeft |
QList< KDecoration3::DecorationButtonType > | decorationButtonsRight |
QFont | font |
int | gridUnit |
int | largeSpacing |
bool | onAllDesktopsAvailable |
int | smallSpacing |
![]() | |
objectName | |
Signals | |
void | alphaChannelSupportedChanged (bool) |
void | borderSizeChanged (KDecoration3::BorderSize size) |
void | closeOnDoubleClickOnMenuChanged (bool) |
void | decorationButtonsLeftChanged (const QList< KDecoration3::DecorationButtonType > &) |
void | decorationButtonsRightChanged (const QList< KDecoration3::DecorationButtonType > &) |
void | fontChanged (const QFont &font) |
void | gridUnitChanged (int) |
void | onAllDesktopsAvailableChanged (bool) |
void | reconfigured () |
void | spacingChanged () |
Public Member Functions | |
DecorationSettings (DecorationBridge *bridge, QObject *parent=nullptr) | |
BorderSize | borderSize () const |
QList< DecorationButtonType > | decorationButtonsLeft () const |
QList< DecorationButtonType > | decorationButtonsRight () const |
QFont | font () const |
QFontMetricsF | fontMetrics () const |
int | gridUnit () const |
bool | isAlphaChannelSupported () const |
bool | isCloseOnDoubleClickOnMenu () const |
bool | isOnAllDesktopsAvailable () const |
int | largeSpacing () const |
int | smallSpacing () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Common settings for the Decoration.
This class gets injected into the Decoration and provides recommendations for the Decoration. The Decoration is suggested to honor the settings, but may decide that some settings don't fit the design and ignore them.
- See also
- Decoration
Definition at line 30 of file decorationsettings.h.
Property Documentation
◆ alphaChannelSupported
|
read |
Whether the Decoration will be rendered with an alpha channel.
If no alpha channel is available a Decoration should not use round borders.
Definition at line 45 of file decorationsettings.h.
◆ borderSize
|
read |
The suggested border size.
Definition at line 62 of file decorationsettings.h.
◆ closeOnDoubleClickOnMenu
|
read |
Whether the Decoration should close the DecoratedWindow when double clicking on the DecorationButtonType::Menu.
Definition at line 50 of file decorationsettings.h.
◆ decorationButtonsLeft
|
read |
The suggested ordering of the decoration buttons on the left.
Definition at line 54 of file decorationsettings.h.
◆ decorationButtonsRight
|
read |
The suggested ordering of the decoration buttons on the right.
Definition at line 58 of file decorationsettings.h.
◆ font
|
read |
The recommended font for the Decoration's caption.
Definition at line 71 of file decorationsettings.h.
◆ gridUnit
|
read |
The fundamental unit of space that should be used for sizes, expressed in pixels.
Given the screen has an accurate DPI settings, it corresponds to a millimeter
Definition at line 67 of file decorationsettings.h.
◆ largeSpacing
|
read |
largeSpacing is the amount of spacing that should be used inside bigger UI elements, for example between an icon and the corresponding text.
Internally, this size depends on the size of the default font as rendered on the screen, so it takes user-configured font size and DPI into account.
Definition at line 86 of file decorationsettings.h.
◆ onAllDesktopsAvailable
|
read |
Whether the feature to put a DecoratedWindow on all desktops is available.
If this feature is not available a Decoration might decide to not show the DecorationButtonType::OnAllDesktops.
Definition at line 39 of file decorationsettings.h.
◆ smallSpacing
|
read |
smallSpacing is the amount of spacing that should be used around smaller UI elements, for example as spacing in Columns.
Internally, this size depends on the size of the default font as rendered on the screen, so it takes user-configured font size and DPI into account.
Definition at line 78 of file decorationsettings.h.
Constructor & Destructor Documentation
◆ DecorationSettings()
|
explicit |
Definition at line 14 of file decorationsettings.cpp.
Member Function Documentation
◆ borderSize()
BorderSize KDecoration3::DecorationSettings::borderSize | ( | ) | const |
Definition at line 65 of file decorationsettings.cpp.
◆ decorationButtonsLeft()
QList< DecorationButtonType > KDecoration3::DecorationSettings::decorationButtonsLeft | ( | ) | const |
Definition at line 55 of file decorationsettings.cpp.
◆ decorationButtonsRight()
QList< DecorationButtonType > KDecoration3::DecorationSettings::decorationButtonsRight | ( | ) | const |
Definition at line 60 of file decorationsettings.cpp.
◆ font()
QFont KDecoration3::DecorationSettings::font | ( | ) | const |
Definition at line 70 of file decorationsettings.cpp.
◆ fontMetrics()
QFontMetricsF KDecoration3::DecorationSettings::fontMetrics | ( | ) | const |
The fontMetrics for the recommended font.
- See also
- font
Definition at line 75 of file decorationsettings.cpp.
◆ gridUnit()
int KDecoration3::DecorationSettings::gridUnit | ( | ) | const |
Definition at line 80 of file decorationsettings.cpp.
◆ isAlphaChannelSupported()
bool KDecoration3::DecorationSettings::isAlphaChannelSupported | ( | ) | const |
Definition at line 45 of file decorationsettings.cpp.
◆ isCloseOnDoubleClickOnMenu()
bool KDecoration3::DecorationSettings::isCloseOnDoubleClickOnMenu | ( | ) | const |
Definition at line 50 of file decorationsettings.cpp.
◆ isOnAllDesktopsAvailable()
bool KDecoration3::DecorationSettings::isOnAllDesktopsAvailable | ( | ) | const |
Definition at line 40 of file decorationsettings.cpp.
◆ largeSpacing()
int KDecoration3::DecorationSettings::largeSpacing | ( | ) | const |
Definition at line 90 of file decorationsettings.cpp.
◆ reconfigured
|
signal |
This signal is emitted when the backend got reconfigured.
If the plugin uses custom settings, it is recommended to re-read them after this signal got emitted.
◆ smallSpacing()
int KDecoration3::DecorationSettings::smallSpacing | ( | ) | const |
Definition at line 85 of file decorationsettings.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 12:01:53 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.