BluezQt::Adapter
#include <BluezQt/Adapter>
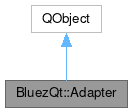
Properties | |
quint32 | adapterClass |
QString | address |
QList< DevicePtr > | devices |
bool | discoverable |
quint32 | discoverableTimeout |
bool | discovering |
LEAdvertisingManagerPtr | leAdvertisingManager |
MediaPtr | media |
QString | modalias |
QString | name |
bool | pairable |
quint32 | pairableTimeout |
bool | powered |
QString | systemName |
QString | ubi |
QStringList | uuids |
![]() | |
objectName | |
Signals | |
void | adapterChanged (AdapterPtr adapter) |
void | adapterClassChanged (quint32 adapterClass) |
void | adapterRemoved (AdapterPtr adapter) |
void | deviceAdded (DevicePtr device) |
void | deviceChanged (DevicePtr device) |
void | deviceRemoved (DevicePtr device) |
void | discoverableChanged (bool discoverable) |
void | discoverableTimeoutChanged (quint32 timeout) |
void | discoveringChanged (bool discovering) |
void | gattManagerChanged (GattManagerPtr gattManager) |
void | leAdvertisingManagerChanged (LEAdvertisingManagerPtr leAdvertisingManager) |
void | mediaChanged (MediaPtr media) |
void | modaliasChanged (const QString &modalias) |
void | nameChanged (const QString &name) |
void | pairableChanged (bool pairable) |
void | pairableTimeoutChanged (quint32 timeout) |
void | poweredChanged (bool powered) |
void | systemNameChanged (const QString &systemName) |
void | uuidsChanged (const QStringList &uuids) |
Public Member Functions | |
~Adapter () override | |
quint32 | adapterClass () const |
QString | address () const |
DevicePtr | deviceForAddress (const QString &address) const |
QList< DevicePtr > | devices () const |
quint32 | discoverableTimeout () const |
GattManagerPtr | gattManager () const |
PendingCall * | getDiscoveryFilters () |
bool | isDiscoverable () const |
bool | isDiscovering () |
bool | isPairable () const |
bool | isPowered () const |
LEAdvertisingManagerPtr | leAdvertisingManager () const |
MediaPtr | media () const |
QString | modalias () const |
QString | name () const |
quint32 | pairableTimeout () const |
PendingCall * | removeDevice (DevicePtr device) |
PendingCall * | setDiscoverable (bool discoverable) |
PendingCall * | setDiscoverableTimeout (quint32 timeout) |
PendingCall * | setDiscoveryFilter (const QVariantMap &filter) |
PendingCall * | setName (const QString &name) |
PendingCall * | setPairable (bool pairable) |
PendingCall * | setPairableTimeout (quint32 timeout) |
PendingCall * | setPowered (bool powered) |
PendingCall * | startDiscovery () |
PendingCall * | stopDiscovery () |
QString | systemName () const |
AdapterPtr | toSharedPtr () const |
QString | ubi () const |
QStringList | uuids () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Bluetooth adapter.
This class represents a Bluetooth adapter.
Property Documentation
◆ adapterClass
◆ address
◆ devices
◆ discoverable
◆ discoverableTimeout
◆ discovering
◆ leAdvertisingManager
|
read |
◆ media
◆ modalias
◆ name
◆ pairable
◆ pairableTimeout
◆ powered
◆ systemName
◆ ubi
◆ uuids
|
read |
Constructor & Destructor Documentation
◆ ~Adapter()
|
overridedefault |
Destroys an Adapter object.
Member Function Documentation
◆ adapterChanged
|
signal |
Indicates that at least one of the adapter's properties have changed.
◆ adapterClass()
quint32 BluezQt::Adapter::adapterClass | ( | ) | const |
◆ adapterClassChanged
|
signal |
Indicates that adapter's class have changed.
◆ adapterRemoved
|
signal |
Indicates that the adapter was removed.
◆ address()
QString BluezQt::Adapter::address | ( | ) | const |
Returns an address of the adapter.
Example address: "1C:E5:C3:BC:94:7E"
- Returns
- address of adapter
Definition at line 35 of file adapter.cpp.
◆ deviceAdded
|
signal |
Indicates that a new device was added (eg.
found by discovery).
◆ deviceChanged
|
signal |
Indicates that at least one of the device's properties have changed.
◆ deviceForAddress()
Returns a device for specified address.
- Parameters
-
address address of device (eg. "40:79:6A:0C:39:75")
- Returns
- null if there is no device with specified address
Definition at line 145 of file adapter.cpp.
◆ deviceRemoved
|
signal |
Indicates that a device was removed.
◆ devices()
Returns list of devices known by the adapter.
- Returns
- list of devices
Definition at line 140 of file adapter.cpp.
◆ discoverableChanged
|
signal |
Indicates that adapter's discoverable state have changed.
◆ discoverableTimeout()
quint32 BluezQt::Adapter::discoverableTimeout | ( | ) | const |
Returns the discoverable timeout in seconds of the adapter.
Discoverable timeout defines how long the adapter stays in discoverable state after calling setDiscoverable(true).
Timeout 0 means infinitely.
- Returns
- discoverable timeout of adapter
Definition at line 80 of file adapter.cpp.
◆ discoverableTimeoutChanged
|
signal |
Indicates that adapter's discoverable timeout have changed.
◆ discoveringChanged
|
signal |
Indicates that adapter's discovering state have changed.
◆ gattManager()
GattManagerPtr BluezQt::Adapter::gattManager | ( | ) | const |
Returns the GATT manager interface for the adapter.
- Returns
- null if adapter have no GATT manager
Definition at line 125 of file adapter.cpp.
◆ gattManagerChanged
|
signal |
Indicates that adapter's GATT manager have changed.
◆ getDiscoveryFilters()
PendingCall * BluezQt::Adapter::getDiscoveryFilters | ( | ) |
Get the discovery filters for the caller.
This returns the available filters that can be given to setDiscoveryFilter, for details see Bluez documentation for Adapter object
Possible errors: PendingCall::Failed
- Returns
- string list pending call
Definition at line 175 of file adapter.cpp.
◆ isDiscoverable()
bool BluezQt::Adapter::isDiscoverable | ( | ) | const |
Returns whether the adapter is discoverable by other devices.
- Returns
- true if adapter is discoverable
Definition at line 70 of file adapter.cpp.
◆ isDiscovering()
bool BluezQt::Adapter::isDiscovering | ( | ) |
Returns whether the adapter is discovering for other devices.
- Returns
- true if adapter is discovering
Definition at line 110 of file adapter.cpp.
◆ isPairable()
bool BluezQt::Adapter::isPairable | ( | ) | const |
Returns whether the adapter is pairable with other devices.
- Returns
- true if adapter is pairable
Definition at line 90 of file adapter.cpp.
◆ isPowered()
bool BluezQt::Adapter::isPowered | ( | ) | const |
Returns whether the adapter is powered on.
- Returns
- true if adapter is powered on
Definition at line 60 of file adapter.cpp.
◆ leAdvertisingManager()
LEAdvertisingManagerPtr BluezQt::Adapter::leAdvertisingManager | ( | ) | const |
Returns the LE advertising manager interface for the adapter.
- Returns
- null if adapter have no Bluetooth LE
Definition at line 130 of file adapter.cpp.
◆ leAdvertisingManagerChanged
|
signal |
Indicates that adapter's LE advertising manager have changed.
◆ media()
MediaPtr BluezQt::Adapter::media | ( | ) | const |
Returns the media interface for the adapter.
- Returns
- null if adapter have no media
Definition at line 135 of file adapter.cpp.
◆ mediaChanged
|
signal |
Indicates that adapter's media have changed.
◆ modalias()
QString BluezQt::Adapter::modalias | ( | ) | const |
Returns local device ID in modalias format.
- Returns
- adapter modalias
Definition at line 120 of file adapter.cpp.
◆ modaliasChanged
|
signal |
Indicates that adapter's modalias have changed.
◆ name()
QString BluezQt::Adapter::name | ( | ) | const |
◆ nameChanged
|
signal |
Indicates that adapter's name have changed.
◆ pairableChanged
|
signal |
Indicates that adapter's pairable state have changed.
◆ pairableTimeout()
quint32 BluezQt::Adapter::pairableTimeout | ( | ) | const |
Returns the pairable timeout in seconds of the adapter.
Pairable timeout defines how long the adapter stays in pairable state after calling setPairable(true).
Timeout 0 means infinitely.
- Returns
- pairable timeout of adapter
Definition at line 100 of file adapter.cpp.
◆ pairableTimeoutChanged
|
signal |
Indicates that adapter's pairable timeout have changed.
◆ poweredChanged
|
signal |
Indicates that adapter's powered state have changed.
◆ removeDevice()
PendingCall * BluezQt::Adapter::removeDevice | ( | DevicePtr | device | ) |
Removes the specified device.
It will also remove the pairing information.
Possible errors: PendingCall::InvalidArguments, PendingCall::Failed
- Parameters
-
device device to be removed
- Returns
- void pending call
Definition at line 165 of file adapter.cpp.
◆ setDiscoverable()
PendingCall * BluezQt::Adapter::setDiscoverable | ( | bool | discoverable | ) |
Sets the discoverable state of the adapter.
- Parameters
-
discoverable discoverable state
- Returns
- void pending call
Definition at line 75 of file adapter.cpp.
◆ setDiscoverableTimeout()
PendingCall * BluezQt::Adapter::setDiscoverableTimeout | ( | quint32 | timeout | ) |
Sets the discoverable timeout of the adapter.
- Parameters
-
timeout timeout in seconds
- Returns
- void pending call
Definition at line 85 of file adapter.cpp.
◆ setDiscoveryFilter()
PendingCall * BluezQt::Adapter::setDiscoveryFilter | ( | const QVariantMap & | filter | ) |
Set the discovery filter for the caller.
When this method is called with no filter parameter, the filter is removed.
For details and available filter options, see Bluez documentation for Adapter object
Possible errors: PendingCall::InvalidArguments, PendingCall::Failed
- Parameters
-
filter options dictionary
- Returns
- void pending call
Definition at line 170 of file adapter.cpp.
◆ setName()
PendingCall * BluezQt::Adapter::setName | ( | const QString & | name | ) |
Sets the name of the adapter.
- Parameters
-
name name of adapter
- Returns
- void pending call
Definition at line 45 of file adapter.cpp.
◆ setPairable()
PendingCall * BluezQt::Adapter::setPairable | ( | bool | pairable | ) |
Sets the pairable state of the adapter.
- Parameters
-
pairable pairable state
- Returns
- void pending call
Definition at line 95 of file adapter.cpp.
◆ setPairableTimeout()
PendingCall * BluezQt::Adapter::setPairableTimeout | ( | quint32 | timeout | ) |
Sets the pairable timeout of the adapter.
- Parameters
-
timeout timeout in seconds
- Returns
- void pending call
Definition at line 105 of file adapter.cpp.
◆ setPowered()
PendingCall * BluezQt::Adapter::setPowered | ( | bool | powered | ) |
Sets the powered state of the adapter.
- Parameters
-
powered powered state
- Returns
- void pending call
Definition at line 65 of file adapter.cpp.
◆ startDiscovery()
PendingCall * BluezQt::Adapter::startDiscovery | ( | ) |
Starts device discovery.
Possible errors: PendingCall::NotReady, PendingCall::Failed
- Returns
- void pending call
- See also
- discoverableTimeout() const
Definition at line 155 of file adapter.cpp.
◆ stopDiscovery()
PendingCall * BluezQt::Adapter::stopDiscovery | ( | ) |
Stops device discovery.
Possible errors: PendingCall::NotReady, PendingCall::Failed, PendingCall::NotAuthorized
- Returns
- void pending call
Definition at line 160 of file adapter.cpp.
◆ systemName()
QString BluezQt::Adapter::systemName | ( | ) | const |
Returns a system name (hostname) of the adapter.
- Returns
- system name of adapter
Definition at line 50 of file adapter.cpp.
◆ systemNameChanged
|
signal |
Indicates that adapter's system name have changed.
◆ toSharedPtr()
AdapterPtr BluezQt::Adapter::toSharedPtr | ( | ) | const |
◆ ubi()
QString BluezQt::Adapter::ubi | ( | ) | const |
Returns an UBI of the adapter.
Example UBI: "/org/bluez/hci0"
- Returns
- UBI of adapter
Definition at line 30 of file adapter.cpp.
◆ uuids()
QStringList BluezQt::Adapter::uuids | ( | ) | const |
Returns UUIDs of supported services by the adapter.
UUIDs will always be returned in uppercase.
- Returns
- UUIDs of supported services
Definition at line 115 of file adapter.cpp.
◆ uuidsChanged
|
signal |
Indicates that adapter's UUIDs have changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Nov 22 2024 12:00:59 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.