BluezQt::Device
#include <BluezQt/Device>
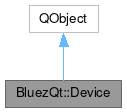
Public Types | |
enum | Type { Phone , Modem , Computer , Network , Headset , Headphones , AudioVideo , Keyboard , Mouse , Joypad , Tablet , Peripheral , Camera , Printer , Imaging , Wearable , Toy , Health , Uncategorized } |
Properties | |
AdapterPtr | adapter |
QString | address |
quint16 | appearance |
BatteryPtr | battery |
bool | blocked |
bool | connected |
quint32 | deviceClass |
QString | friendlyName |
QList< GattServiceRemotePtr > | gattServices |
QString | icon |
InputPtr | input |
bool | legacyPairing |
ManData | manufacturerData |
MediaPlayerPtr | mediaPlayer |
MediaTransportPtr | mediaTransport |
QString | modalias |
QString | name |
bool | paired |
QString | remoteName |
qint16 | rssi |
bool | servicesResolved |
bool | trusted |
Type | type |
QString | ubi |
QStringList | uuids |
![]() | |
objectName | |
Public Slots | |
PendingCall * | cancelPairing () |
PendingCall * | connectProfile (const QString &uuid) |
PendingCall * | connectToDevice () |
PendingCall * | disconnectFromDevice () |
PendingCall * | disconnectProfile (const QString &uuid) |
PendingCall * | pair () |
Public Member Functions | |
~Device () override | |
AdapterPtr | adapter () const |
QString | address () const |
quint16 | appearance () const |
BatteryPtr | battery () const |
quint32 | deviceClass () const |
QString | friendlyName () const |
QList< GattServiceRemotePtr > | gattServices () const |
bool | hasLegacyPairing () const |
QString | icon () const |
InputPtr | input () const |
bool | isBlocked () const |
bool | isConnected () const |
bool | isPaired () const |
bool | isServicesResolved () const |
bool | isTrusted () const |
ManData | manufacturerData () const |
MediaPlayerPtr | mediaPlayer () const |
MediaTransportPtr | mediaTransport () const |
QString | modalias () const |
QString | name () const |
QString | remoteName () const |
qint16 | rssi () const |
QHash< QString, QByteArray > | serviceData () const |
PendingCall * | setBlocked (bool blocked) |
PendingCall * | setName (const QString &name) |
PendingCall * | setTrusted (bool trusted) |
DevicePtr | toSharedPtr () const |
Device::Type | type () const |
QString | ubi () const |
QStringList | uuids () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Device::Type | stringToType (const QString &typeString) |
static QString | typeToString (Device::Type type) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Member Enumeration Documentation
◆ Type
Device types.
Property Documentation
◆ adapter
|
read |
◆ address
◆ appearance
◆ battery
|
read |
◆ blocked
◆ connected
◆ deviceClass
◆ friendlyName
◆ gattServices
|
read |
◆ icon
◆ input
◆ legacyPairing
◆ manufacturerData
◆ mediaPlayer
|
read |
◆ mediaTransport
|
read |
◆ modalias
◆ name
◆ paired
◆ remoteName
◆ rssi
◆ servicesResolved
◆ trusted
◆ type
◆ ubi
◆ uuids
|
read |
Constructor & Destructor Documentation
◆ ~Device()
|
overridedefault |
Destroys a Device object.
Member Function Documentation
◆ adapter()
AdapterPtr BluezQt::Device::adapter | ( | ) | const |
Returns an adapter that discovered this device.
- Returns
- adapter of device
Definition at line 181 of file device.cpp.
◆ address()
QString BluezQt::Device::address | ( | ) | const |
Returns an address of the device.
Example address: "40:79:6A:0C:39:75"
- Returns
- address of device
Definition at line 34 of file device.cpp.
◆ addressChanged
|
signal |
Indicates that device's address have changed.
◆ appearance()
quint16 BluezQt::Device::appearance | ( | ) | const |
Returns an appearance of the device.
- Returns
- appearance of device
Definition at line 79 of file device.cpp.
◆ appearanceChanged
|
signal |
Indicates that device's appearance have changed.
◆ battery()
BatteryPtr BluezQt::Device::battery | ( | ) | const |
Returns the battery interface for the device.
- Returns
- null if device has no battery
- Since
- 5.66
Definition at line 161 of file device.cpp.
◆ batteryChanged
|
signal |
Indicates that device's battery has changed.
◆ blockedChanged
|
signal |
Indicates that device's blocked state have changed.
◆ cancelPairing
|
slot |
Cancels a pairing with the device.
This method can be used to cancel pairing operation initiated with pair().
Possible errors: PendingCall::DoesNotExist, PendingCall::Failed
- Returns
- void pending call
Definition at line 302 of file device.cpp.
◆ connectedChanged
|
signal |
Indicates that device's connected state have changed.
◆ connectProfile
|
slot |
Connects a specific profile of the device.
Possible errors: PendingCall::DoesNotExist, PendingCall::AlreadyConnected, PendingCall::ConnectFailed
- Parameters
-
uuid service UUID
- Returns
- void pending call
Definition at line 287 of file device.cpp.
◆ connectToDevice
|
slot |
Connects all auto-connectable profiles of the device.
This method indicates success if at least one profile was connected.
Possible errors: PendingCall::NotReady, PendingCall::Failed, PendingCall::InProgress, PendingCall::AlreadyConnected
- Returns
- void pending call
Definition at line 277 of file device.cpp.
◆ deviceChanged
|
signal |
Indicates that at least one of the device's properties have changed.
◆ deviceClass()
quint32 BluezQt::Device::deviceClass | ( | ) | const |
Returns a class of the device.
In case of Bluetooth Low Energy only devices, device class is invalid (0).
- See also
- type() const
- Returns
- class of device
Definition at line 65 of file device.cpp.
◆ deviceClassChanged
|
signal |
Indicates that device's class have changed.
◆ deviceRemoved
|
signal |
Indicates that the device was removed.
◆ disconnectFromDevice
|
slot |
Disconnects all connected profiles of the device.
This method can be used to cancel not-yet finished connectDevice() call.
Possible errors: PendingCall::NotConnected
- Returns
- void pending call
Definition at line 282 of file device.cpp.
◆ disconnectProfile
|
slot |
Disconnects a specific profile of the device.
Possible errors: PendingCall::DoesNotExist, PendingCall::Failed, PendingCall::NotConnected, PendingCall::NotSupported
- Parameters
-
uuid service UUID
- Returns
- void pending call
Definition at line 292 of file device.cpp.
◆ friendlyName()
QString BluezQt::Device::friendlyName | ( | ) | const |
Returns a friendly name of the device.
Friendly name is a string "name (remoteName)". If the remoteName is same as name, it returns just name.
- Returns
- friendly name of device
Definition at line 49 of file device.cpp.
◆ friendlyNameChanged
|
signal |
Indicates that device's friendly name have changed.
◆ gattServiceAdded
|
signal |
Indicates that a new service was added (eg.
found by connection).
◆ gattServiceChanged
|
signal |
Indicates that at least one of the device's services have changed.
◆ gattServiceRemoved
|
signal |
Indicates that a service was removed.
◆ gattServices()
QList< GattServiceRemotePtr > BluezQt::Device::gattServices | ( | ) | const |
Returns list of services known by the device.
- Returns
- list of services
Definition at line 186 of file device.cpp.
◆ gattServicesChanged
|
signal |
Indicates that device GATT services list has changed.
◆ hasLegacyPairing()
bool BluezQt::Device::hasLegacyPairing | ( | ) | const |
Returns whether the device has legacy pairing.
- Returns
- true if device has legacy pairing
Definition at line 121 of file device.cpp.
◆ icon()
QString BluezQt::Device::icon | ( | ) | const |
Returns an icon name of the device.
In case the icon is empty, "preferences-system-bluetooth" is returned.
- Returns
- icon name of device
Definition at line 84 of file device.cpp.
◆ iconChanged
|
signal |
Indicates that device's icon have changed.
◆ input()
InputPtr BluezQt::Device::input | ( | ) | const |
Returns the input interface for the device.
Only input devices will have valid input interface.
- Returns
- null if device have no input
Definition at line 166 of file device.cpp.
◆ inputChanged
|
signal |
Indicates that device's input have changed.
◆ isBlocked()
bool BluezQt::Device::isBlocked | ( | ) | const |
Returns whether the device is blocked.
- Returns
- true if device is blocked
Definition at line 111 of file device.cpp.
◆ isConnected()
bool BluezQt::Device::isConnected | ( | ) | const |
Returns whether the device is connected.
- Returns
- true if connected
Definition at line 141 of file device.cpp.
◆ isPaired()
bool BluezQt::Device::isPaired | ( | ) | const |
Returns whether the device is paired.
- Returns
- true if device is paired
Definition at line 96 of file device.cpp.
◆ isServicesResolved()
bool BluezQt::Device::isServicesResolved | ( | ) | const |
Returns whether or not service discovery has been resolved.
- Returns
- true if servicesResolved
Definition at line 136 of file device.cpp.
◆ isTrusted()
bool BluezQt::Device::isTrusted | ( | ) | const |
Returns whether the device is trusted.
- Returns
- true if device is trusted
Definition at line 101 of file device.cpp.
◆ legacyPairingChanged
|
signal |
Indicates that device's legacy pairing state have changed.
◆ manufacturerData()
ManData BluezQt::Device::manufacturerData | ( | ) | const |
Returns manufacturer specific advertisement data.
- Note
- Keys are 16 bits Manufacturer ID followed by its byte array value.
- Returns
- manufacturerData of device.
Definition at line 131 of file device.cpp.
◆ manufacturerDataChanged
|
signal |
Indicates that device's manufacturer data have changed.
◆ mediaPlayer()
MediaPlayerPtr BluezQt::Device::mediaPlayer | ( | ) | const |
Returns the media player interface for the device.
Only devices with connected appropriate profile will have valid media player interface.
- Returns
- null if device have no media player
Definition at line 171 of file device.cpp.
◆ mediaPlayerChanged
|
signal |
Indicates that device's media player have changed.
◆ mediaTransport()
MediaTransportPtr BluezQt::Device::mediaTransport | ( | ) | const |
Returns the media transport interface for the device.
Only devices with connected appropriate profile will have valid media transport interface.
- Returns
- null if device have no media transport
Definition at line 176 of file device.cpp.
◆ mediaTransportChanged
|
signal |
Indicates that device's media transport have changed.
◆ modalias()
QString BluezQt::Device::modalias | ( | ) | const |
Returns remote device ID in modalias format.
- Returns
- device modalias
Definition at line 151 of file device.cpp.
◆ modaliasChanged
|
signal |
Indicates that device's modalias have changed.
◆ name()
QString BluezQt::Device::name | ( | ) | const |
Returns a name of the device.
If the name of the device wasn't previously changed, remoteName is returned.
- Returns
- name of device
Definition at line 39 of file device.cpp.
◆ nameChanged
|
signal |
Indicates that device's name have changed.
◆ pair
|
slot |
Initiates a pairing with the device.
Possible errors: PendingCall::InvalidArguments, PendingCall::Failed, PendingCall::AlreadyExists, PendingCall::AuthenticationCanceled, PendingCall::AuthenticationFailed, PendingCall::AuthenticationRejected, PendingCall::AuthenticationTimeout, PendingCall::ConnectionAttemptFailed
- Returns
- void pending call
Definition at line 297 of file device.cpp.
◆ pairedChanged
|
signal |
Indicates that device's paired state have changed.
◆ remoteName()
QString BluezQt::Device::remoteName | ( | ) | const |
Returns a remote name of the device.
- Returns
- remote name of device
Definition at line 60 of file device.cpp.
◆ remoteNameChanged
|
signal |
Indicates that device's remote name have changed.
◆ rssi()
qint16 BluezQt::Device::rssi | ( | ) | const |
Returns Received Signal Strength Indicator of the device.
The bigger value indicates better signal strength.
- Note
- RSSI is only updated during discovery.
- Returns
- RSSI of device
Definition at line 126 of file device.cpp.
◆ rssiChanged
|
signal |
Indicates that device's RSSI have changed.
◆ serviceData()
QHash< QString, QByteArray > BluezQt::Device::serviceData | ( | ) | const |
Returns the service advertisement data.
- Returns
- A hash with keys being the UUIDs in and values being the raw service data value.
- Since
- 5.72
Definition at line 156 of file device.cpp.
◆ serviceDataChanged
|
signal |
Indicates that the device's service data has changed.
- Since
- 5.72
◆ servicesResolvedChanged
|
signal |
Indicates that device's servicesResolved state have changed.
◆ setBlocked()
PendingCall * BluezQt::Device::setBlocked | ( | bool | blocked | ) |
Sets the blocked state of the device.
- Parameters
-
blocked blocked state
- Returns
- void pending call
Definition at line 116 of file device.cpp.
◆ setName()
PendingCall * BluezQt::Device::setName | ( | const QString & | name | ) |
Sets the name of the device.
- Parameters
-
name name for device
- Returns
- void pending call
Definition at line 44 of file device.cpp.
◆ setTrusted()
PendingCall * BluezQt::Device::setTrusted | ( | bool | trusted | ) |
Sets the trusted state of the device.
- Parameters
-
trusted trusted state
- Returns
- void pending call
Definition at line 106 of file device.cpp.
◆ stringToType()
|
static |
Returns a device type for string.
- Parameters
-
typeString type string
- Returns
- device type
Definition at line 235 of file device.cpp.
◆ toSharedPtr()
DevicePtr BluezQt::Device::toSharedPtr | ( | ) | const |
◆ trustedChanged
|
signal |
Indicates that device's trusted state have changed.
◆ type()
Device::Type BluezQt::Device::type | ( | ) | const |
Returns a type of the device.
Type of device is deduced from its class (for Bluetooth Classic devices) or its appearance (for Bluetooth Low Energy devices).
- See also
- deviceClass() const
- appearance() const
- Returns
- type of device
Definition at line 70 of file device.cpp.
◆ typeChanged
|
signal |
Indicates that device's type have changed.
◆ typeToString()
|
static |
Returns a string for device type.
- Parameters
-
type device type
- Returns
- device type string
Definition at line 191 of file device.cpp.
◆ ubi()
QString BluezQt::Device::ubi | ( | ) | const |
Returns an UBI of the device.
Example UBI: "/org/bluez/hci0/dev_40_79_6A_0C_39_75"
- Returns
- UBI of device
Definition at line 29 of file device.cpp.
◆ uuids()
QStringList BluezQt::Device::uuids | ( | ) | const |
Returns UUIDs of supported services by the device.
UUIDs will always be returned in uppercase.
- Returns
- UUIDs of supported services
Definition at line 146 of file device.cpp.
◆ uuidsChanged
|
signal |
Indicates that device's UUIDs have changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:48:04 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.