BluezQt::Job
#include <BluezQt/Job>
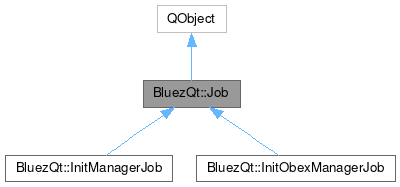
Public Types | |
enum | Error { NoError = 0 , UserDefinedError = 100 } |
Properties | |
int | error |
QString | errorText |
bool | finished |
bool | running |
![]() | |
objectName | |
Public Slots | |
void | kill () |
void | start () |
Public Member Functions | |
Job (QObject *parent=nullptr) | |
~Job () override | |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isFinished () const |
bool | isRunning () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
virtual void | doStart ()=0 |
Protected Member Functions | |
virtual void | doEmitResult ()=0 |
void | emitResult () |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
This class represents an asynchronous job performed by BluezQt, it is usually not used directly but instead it is inherit by some other class.
There are two ways of using this class, one is via exec() which will block the thread until a result is fetched, the other is via connecting to the signal result()
Please, think twice before using exec(), it should be used only in either unittest or cli apps.
- Note
- Job and its subclasses are meant to be used in a fire-and-forget way. Jobs will delete themselves when they finish using deleteLater().
- Even given their asynchronous nature, Jobs are still executed in the main thread, so any blocking code executed in it will block the app calling it.
- See also
- InitManagerJob
- InitObexManagerJob
Member Enumeration Documentation
◆ Error
enum BluezQt::Job::Error |
Property Documentation
◆ error
◆ errorText
◆ finished
◆ running
Constructor & Destructor Documentation
◆ Job()
|
explicit |
◆ ~Job()
|
overridedefault |
Destroys a Job object.
Member Function Documentation
◆ doEmitResult()
|
protectedpure virtual |
Implementation for emitting the result signal.
This function is needed to be able to emit result() signal with the job pointer's type being subclass
◆ doStart
|
protectedpure virtualslot |
Implementation for start() that will be executed in next loop.
This slot is always called in the next loop, triggered by start().
When implementing this method is important to remember that jobs are not executed on a different thread (unless done that way), so any blocking task has to be done in a different thread or process.
◆ emitResult()
|
protected |
Utility function to emit the result signal, and remove this job.
- Note
- Deletes this job using deleteLater().
- See also
- result() const
◆ error()
int BluezQt::Job::error | ( | ) | const |
◆ errorText()
QString BluezQt::Job::errorText | ( | ) | const |
Returns the error text if there has been an error.
Only call if error is not 0.
This is usually some extra data associated with the error, such as a URL. Use errorString() to get a human-readable, translated message.
- Returns
- a string to help understand the error
◆ exec()
bool BluezQt::Job::exec | ( | ) |
Executes the job synchronously.
This will start a nested QEventLoop internally. Nested event loop can be dangerous and can have unintended side effects, you should avoid calling exec() whenever you can and use the asynchronous interface of Job instead.
Should you indeed call this method, you need to make sure that all callers are reentrant, so that events delivered by the inner event loop don't cause non-reentrant functions to be called, which usually wreaks havoc.
Note that the event loop started by this method does not process user input events, which means your user interface will effectively be blocked. Other events like paint or network events are still being processed. The advantage of not processing user input events is that the chance of accidental reentrancy is greatly reduced. Still you should avoid calling this function.
- Warning
- This method blocks until the job finishes!
- Returns
- true if the job has been executed without error, false otherwise
◆ isFinished()
bool BluezQt::Job::isFinished | ( | ) | const |
◆ isRunning()
bool BluezQt::Job::isRunning | ( | ) | const |
◆ kill
|
slot |
Kills the job.
This method will kill the job and then call deleteLater(). Only jobs started with start() can be killed.
It will not emit result signal.
◆ setError()
|
protected |
Sets the error code.
It should be called when an error is encountered in the job, just before calling emitResult().
You should define an enum of error codes, with values starting at Job::UserDefinedError, and use those. For example:
- Parameters
-
errorCode the error code
- See also
- emitResult()
◆ setErrorText()
|
protected |
Sets the error text.
It should be called when an error is encountered in the job, just before calling emitResult().
Provides extra information about the error that cannot be determined directly from the error code. For example, a URL or filename. This string is not normally translatable.
- Parameters
-
errorText the error text
- See also
- emitResult(), setError()
◆ start
|
slot |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:48:04 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.