KDesktopFile
#include <KDesktopFile>
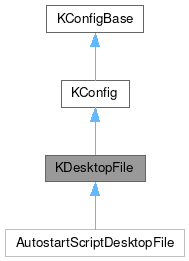
Static Public Member Functions | |
static bool | isAuthorizedDesktopFile (const QString &path) |
static bool | isDesktopFile (const QString &path) |
static QString | locateLocal (const QString &path) |
![]() | |
static QString | mainConfigName () |
static void | setMainConfigName (const QString &str) |
Additional Inherited Members | |
![]() | |
enum | OpenFlag { IncludeGlobals = 0x01 , CascadeConfig = 0x02 , SimpleConfig = 0x00 , NoCascade = IncludeGlobals , NoGlobals = CascadeConfig , FullConfig = IncludeGlobals | CascadeConfig } |
typedef QFlags< OpenFlag > | OpenFlags |
![]() | |
enum | AccessMode { NoAccess , ReadOnly , ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01 , Global = 0x02 , Localized = 0x04 , Notify = 0x08 | Persistent , Normal = Persistent } |
typedef QFlags< WriteConfigFlag > | WriteConfigFlags |
![]() | |
KCONFIGCORE_NO_EXPORT | KConfig (KConfigPrivate &d) |
void | deleteGroupImpl (const QString &groupName, WriteConfigFlags flags=Normal) override |
const KConfigGroup | groupImpl (const QString &groupName) const override |
KConfigGroup | groupImpl (const QString &groupName) override |
bool | hasGroupImpl (const QString &groupName) const override |
bool | isGroupImmutableImpl (const QString &groupName) const override |
void | virtual_hook (int id, void *data) override |
![]() | |
KConfigPrivate *const | d_ptr |
Detailed Description
KDE Desktop File Management.
This class implements KDE's support for the freedesktop.org Desktop Entry Spec.
- See also
- KConfigBase KConfig
- Desktop Entry Spec
Definition at line 27 of file kdesktopfile.h.
Constructor & Destructor Documentation
◆ KDesktopFile() [1/2]
|
explicit |
Constructs a KDesktopFile object.
See QStandardPaths for more information on resources.
- Parameters
-
resourceType Allows you to change what sort of resource to search for if fileName
is not absolute. For instance, you might want to specify GenericConfigLocation.fileName The name or path of the desktop file. If it is not absolute, it will be located using the resource type resType
.
Definition at line 40 of file kdesktopfile.cpp.
◆ KDesktopFile() [2/2]
|
explicit |
Constructs a KDesktopFile object.
See QStandardPaths for more information on resources.
- Parameters
-
fileName The name or path of the desktop file. If it is not absolute, it will be located using the resource type ApplicationsLocation
Definition at line 48 of file kdesktopfile.cpp.
◆ ~KDesktopFile()
|
overridedefault |
Destructs the KDesktopFile object.
Writes back any changed configuration entries.
Member Function Documentation
◆ actionGroup() [1/2]
KConfigGroup KDesktopFile::actionGroup | ( | const QString & | group | ) |
Sets the desktop action group.
- Parameters
-
group the new action group
Definition at line 225 of file kdesktopfile.cpp.
◆ actionGroup() [2/2]
KConfigGroup KDesktopFile::actionGroup | ( | const QString & | group | ) | const |
Definition at line 230 of file kdesktopfile.cpp.
◆ actions()
QList< KDesktopFileAction > KDesktopFile::actions | ( | ) | const |
- Since
- 6.0
Definition at line 310 of file kdesktopfile.cpp.
◆ copyTo()
KDesktopFile * KDesktopFile::copyTo | ( | const QString & | file | ) | const |
Copies all entries from this config object to a new KDesktopFile object that will save itself to file
.
Actual saving to file
happens when the returned object is destructed or when sync() is called upon it.
- Parameters
-
file the new KDesktopFile object it will save itself to.
Definition at line 292 of file kdesktopfile.cpp.
◆ desktopGroup()
KConfigGroup KDesktopFile::desktopGroup | ( | ) | const |
Returns the main config group (named "Desktop Entry") in a .desktop file.
Definition at line 55 of file kdesktopfile.cpp.
◆ fileName()
QString KDesktopFile::fileName | ( | ) | const |
Returns the name of the .desktop file that was used to construct this KDesktopFile.
Definition at line 299 of file kdesktopfile.cpp.
◆ hasActionGroup()
bool KDesktopFile::hasActionGroup | ( | const QString & | group | ) | const |
Returns true if the action group exists, false otherwise.
- Parameters
-
group the action group to test
- Returns
- true if the action group exists
Definition at line 235 of file kdesktopfile.cpp.
◆ hasApplicationType()
bool KDesktopFile::hasApplicationType | ( | ) | const |
Checks whether there is an entry "Type=Application".
- Returns
- true if there is a "Type=Application" entry
Definition at line 245 of file kdesktopfile.cpp.
◆ hasDeviceType()
bool KDesktopFile::hasDeviceType | ( | ) | const |
Checks whether there is an entry "Type=FSDevice".
- Returns
- true if there is a "Type=FSDevice" entry
Definition at line 250 of file kdesktopfile.cpp.
◆ hasLinkType()
bool KDesktopFile::hasLinkType | ( | ) | const |
Checks whether there is a "Type=Link" entry.
The link points to the "URL=" entry.
- Returns
- true if there is a "Type=Link" entry
Definition at line 240 of file kdesktopfile.cpp.
◆ isAuthorizedDesktopFile()
|
static |
Checks whether the user is authorized to run this desktop file.
By default users are authorized to run all desktop files but the KIOSK framework can be used to activate certain restrictions. See README.kiosk for more information.
Note that desktop files that are not in a standard location (as specified by XDG_DATA_DIRS) must have their executable bit set to be authorized, regardless of KIOSK settings, to prevent users from inadvertently running trojan desktop files.
- Parameters
-
path the file to check
- Returns
- true if the user is authorized to run the file
Definition at line 95 of file kdesktopfile.cpp.
◆ isDesktopFile()
|
static |
Checks whether this is really a desktop file.
The check is performed looking at the file extension (the file is not opened). Currently, the only valid extension is ".desktop".
- Parameters
-
path the path of the file to check
- Returns
- true if the file appears to be a desktop file.
Definition at line 90 of file kdesktopfile.cpp.
◆ locateLocal()
Returns the location where changes for the .desktop file path
should be written to.
Definition at line 61 of file kdesktopfile.cpp.
◆ noDisplay()
bool KDesktopFile::noDisplay | ( | ) | const |
Whether the entry should be suppressed in menus.
This handles the NoDisplay key
- Returns
- true to suppress this desktop file
- Since
- 4.1
Definition at line 304 of file kdesktopfile.cpp.
◆ readActions()
QStringList KDesktopFile::readActions | ( | ) | const |
Returns a list of the "Actions=" entries.
- Returns
- the list of actions
Definition at line 213 of file kdesktopfile.cpp.
◆ readComment()
QString KDesktopFile::readComment | ( | ) | const |
Returns the value of the "Comment=" entry.
- Returns
- the comment or QString() if not specified
Definition at line 175 of file kdesktopfile.cpp.
◆ readDocPath()
QString KDesktopFile::readDocPath | ( | ) | const |
Returns the value of the "X-DocPath=" Or "DocPath=" entry.
- Returns
- The value of the "X-DocPath=" Or "DocPath=" entry.
Definition at line 286 of file kdesktopfile.cpp.
◆ readGenericName()
QString KDesktopFile::readGenericName | ( | ) | const |
Returns the value of the "GenericName=" entry.
- Returns
- the generic name or QString() if not specified
Definition at line 181 of file kdesktopfile.cpp.
◆ readIcon()
QString KDesktopFile::readIcon | ( | ) | const |
Returns the value of the "Icon=" entry.
- Returns
- the icon or QString() if not specified
Definition at line 163 of file kdesktopfile.cpp.
◆ readMimeTypes()
QStringList KDesktopFile::readMimeTypes | ( | ) | const |
Returns a list of the "MimeType=" entries.
- Returns
- the list of mime types
- Since
- 5.15
Definition at line 219 of file kdesktopfile.cpp.
◆ readName()
QString KDesktopFile::readName | ( | ) | const |
Returns the value of the "Name=" entry.
- Returns
- the name or QString() if not specified
Definition at line 169 of file kdesktopfile.cpp.
◆ readPath()
QString KDesktopFile::readPath | ( | ) | const |
Returns the value of the "Path=" entry.
- Returns
- the path or QString() if not specified
Definition at line 187 of file kdesktopfile.cpp.
◆ readType()
QString KDesktopFile::readType | ( | ) | const |
Returns the value of the "Type=" entry.
- Returns
- the type or QString() if not specified
Definition at line 157 of file kdesktopfile.cpp.
◆ readUrl()
QString KDesktopFile::readUrl | ( | ) | const |
Returns the value of the "URL=" entry.
- Returns
- the URL or QString() if not specified
Definition at line 197 of file kdesktopfile.cpp.
◆ tryExec()
bool KDesktopFile::tryExec | ( | ) | const |
Checks whether the TryExec field contains a binary which is found on the local system.
- Returns
- true if TryExec contains an existing binary
Definition at line 255 of file kdesktopfile.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:59:53 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.