KHamburgerMenu
#include <KHamburgerMenu>
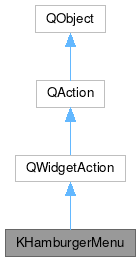
Signals | |
void | aboutToShowMenu () |
Public Member Functions | |
KHamburgerMenu (QObject *parent) | |
void | addToMenu (QMenu *menu) |
void | hideActionsOf (QWidget *widget) |
void | insertIntoMenuBefore (QMenu *menu, QAction *before) |
QMenuBar * | menuBar () const |
bool | menuBarAdvertised () const |
void | setMenuBar (QMenuBar *menuBar) |
void | setMenuBarAdvertised (bool advertise) |
void | setShowMenuBarAction (QAction *showMenuBarAction) |
void | showActionsOf (QWidget *widget) |
![]() | |
QWidgetAction (QObject *parent) | |
QWidget * | defaultWidget () const const |
void | releaseWidget (QWidget *widget) |
QWidget * | requestWidget (QWidget *parent) |
void | setDefaultWidget (QWidget *widget) |
![]() | |
QAction (const QIcon &icon, const QString &text, QObject *parent) | |
QAction (const QString &text, QObject *parent) | |
QAction (QObject *parent) | |
QActionGroup * | actionGroup () const const |
void | activate (ActionEvent event) |
QList< QGraphicsWidget * > | associatedGraphicsWidgets () const const |
QList< QObject * > | associatedObjects () const const |
QList< QWidget * > | associatedWidgets () const const |
bool | autoRepeat () const const |
void | changed () |
void | checkableChanged (bool checkable) |
QVariant | data () const const |
void | enabledChanged (bool enabled) |
QFont | font () const const |
void | hover () |
void | hovered () |
QIcon | icon () const const |
QString | iconText () const const |
bool | isCheckable () const const |
bool | isChecked () const const |
bool | isEnabled () const const |
bool | isIconVisibleInMenu () const const |
bool | isSeparator () const const |
bool | isShortcutVisibleInContextMenu () const const |
bool | isVisible () const const |
QMenu * | menu () const const |
MenuRole | menuRole () const const |
QWidget * | parentWidget () const const |
Priority | priority () const const |
void | resetEnabled () |
void | setActionGroup (QActionGroup *group) |
void | setAutoRepeat (bool) |
void | setCheckable (bool) |
void | setChecked (bool) |
void | setData (const QVariant &data) |
void | setDisabled (bool b) |
void | setEnabled (bool) |
void | setFont (const QFont &font) |
void | setIcon (const QIcon &icon) |
void | setIconText (const QString &text) |
void | setIconVisibleInMenu (bool visible) |
void | setMenu (QMenu *menu) |
void | setMenuRole (MenuRole menuRole) |
void | setPriority (Priority priority) |
void | setSeparator (bool b) |
void | setShortcut (const QKeySequence &shortcut) |
void | setShortcutContext (Qt::ShortcutContext context) |
void | setShortcuts (const QList< QKeySequence > &shortcuts) |
void | setShortcuts (QKeySequence::StandardKey key) |
void | setShortcutVisibleInContextMenu (bool show) |
void | setStatusTip (const QString &statusTip) |
void | setText (const QString &text) |
void | setToolTip (const QString &tip) |
void | setVisible (bool) |
void | setWhatsThis (const QString &what) |
QKeySequence | shortcut () const const |
Qt::ShortcutContext | shortcutContext () const const |
QList< QKeySequence > | shortcuts () const const |
bool | showStatusText (QObject *object) |
QString | statusTip () const const |
QString | text () const const |
void | toggle () |
void | toggled (bool checked) |
QString | toolTip () const const |
void | trigger () |
void | triggered (bool checked) |
void | visibleChanged () |
QString | whatsThis () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
QWidget * | createWidget (QWidget *parent) override |
![]() | |
QList< QWidget * > | createdWidgets () const const |
virtual void | deleteWidget (QWidget *widget) |
virtual bool | event (QEvent *event) override |
virtual bool | eventFilter (QObject *obj, QEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ActionEvent |
enum | MenuRole |
enum | Priority |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
shortcutVisibleInContextMenu | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
AboutQtRole | |
AboutRole | |
ApplicationSpecificRole | |
HighPriority | |
Hover | |
LowPriority | |
NormalPriority | |
NoRole | |
PreferencesRole | |
QuitRole | |
TextHeuristicRole | |
Trigger | |
![]() | |
typedef | QObjectList |
Detailed Description
A menu that substitutes a menu bar when necessary.
Allowing users to toggle the visibility of the menu bar and/or toolbars, while pretty/"simple by default", can lead to various grave usability issues. This class makes it easy to prevent all of them.
Simply add a KHamburgerMenu to your UI (typically to a QToolBar) and make it aware of a QMenuBar like this:
The added menu button will only be visible when the QMenuBar is hidden. With this minimal initialisation it will contain the contents of the menu bar. If a user (also) hides the container the KHamburgerMenu was added to they might find themselves without a way to get a menu back. To prevent this, it is recommended to add the hamburgerMenu to prominent context menus like the one of your central widget preferably at the first position. Simply write:
The added menu will only be visible if the QMenuBar is hidden and the hamburgerMenu->createdWidgets() are all invisible to the user.
Populating the KHamburgerMenu
This is easy:
You probably do not want this to happen on startup. Therefore KHamburgerMenu provides the signal aboutToShowMenu that you can connect to a function containing the previous statements.
Deciding what to put on the hamburger menu
- Be sure to add all of the most important actions. Actions which are already visible on QToolBars, etc. will not show up in the hamburgerMenu. To manage which containers KHamburgerMenu should watch for redundancy use hideActionsOf(QWidget *) and showActionsOf(QWidget *). When a KHamburgerMenu is added to a widget, hideActionsOf(that widget) will automatically be called.
- Do not worry about adding all actions the application has to offer. The KHamburgerMenu will automatically have a section advertising excluded actions which can be found in the QMenuBar. There will also be the showMenuBarAction if you set it with setShowMenuBarAction().
- Do not worry about the help menu. KHamburgerMenu will automatically contain a help menu as the second to last item (if you set a QMenuBar which is expected to have the help menu as the last action).
Open menu by shortcut
For visually impaired users it is important to have a consistent way to open a general-purpose menu. Triggering the keyboard shortcut bound to KHamburgerMenu will always open a menu.
- If setMenuBar() was called and that menu bar is visible, the shortcut will open the first menu of that menu bar.
- Otherwise, if there is a visible KHamburgerMenu button in the user interface, that menu will open.
- Otherwise, KHamburgerMenu's menu will open at the mouse cursor position.
- Since
- 5.81
Definition at line 104 of file khamburgermenu.h.
Constructor & Destructor Documentation
◆ KHamburgerMenu()
|
explicit |
Definition at line 25 of file khamburgermenu.cpp.
Member Function Documentation
◆ aboutToShowMenu
|
signal |
This signal is emitted when a hamburger menu button is about to be pressed down.
It is also emitted when a QMenu that contains a visible KHamburgerMenu emits QMenu::aboutToShow.
◆ addToMenu()
void KHamburgerMenu::addToMenu | ( | QMenu * | menu | ) |
Adds this KHamburgerMenu to menu
.
It will only be visible in the menu if both the menu bar and all of this QWidgetAction's createdWidgets() are invisible. If it is visible in the menu, then opening the menu emits the aboutToShowMenu signal.
- Parameters
-
menu The menu this KHamburgerMenu is supposed to appear in.
Definition at line 108 of file khamburgermenu.cpp.
◆ createWidget()
- See also
- QWidgetAction::createWidget
Reimplemented from QWidgetAction.
Definition at line 186 of file khamburgermenu.cpp.
◆ hideActionsOf()
void KHamburgerMenu::hideActionsOf | ( | QWidget * | widget | ) |
Adds widget
to a list of widgets that should be monitored for their actions().
If the widget is a QMenu, its actions will be treated as known to the user. If the widget isn't a QMenu, its actions will only be treated as known to the user when the widget is actually visible.
- Parameters
-
widget A widget that contains actions which should not show up in the KHamburgerMenu redundantly.
Definition at line 142 of file khamburgermenu.cpp.
◆ insertIntoMenuBefore()
Inserts this KHamburgerMenu to menu's
list of actions, before the action before
.
It will only be visible in the menu if both the menu bar and all of this QWidgetAction's createdWidgets() are invisible. If it is visible in the menu, then opening the menu emits the aboutToShowMenu signal.
- Parameters
-
before The action before which KHamburgerMenu should be inserted. menu The menu this KHamburgerMenu is supposed to appear in.
- Since
- 5.99
Definition at line 114 of file khamburgermenu.cpp.
◆ menuBar()
QMenuBar * KHamburgerMenu::menuBar | ( | ) | const |
- See also
- setMenuBar()
Definition at line 64 of file khamburgermenu.cpp.
◆ menuBarAdvertised()
bool KHamburgerMenu::menuBarAdvertised | ( | ) | const |
- See also
- setMenuBarAdvertised()
Definition at line 86 of file khamburgermenu.cpp.
◆ setMenuBar()
void KHamburgerMenu::setMenuBar | ( | QMenuBar * | menuBar | ) |
Associates this KHamburgerMenu with menuBar
.
The KHamburgerMenu will from now on only be visible when menuBar
is hidden. (Menu bars with QMenuBar::isNativeMenuBar() == true are considered hidden.)
Furthermore the KHamburgerMenu will have the help menu from the menuBar
added at the end. There will also be a special sub-menu advertising actions which are only available in the menu bar unless advertiseMenuBar(false) was called.
- Parameters
-
menuBar The QMenuBar the KHamburgerMenu should be associated with. This can be set to nullptr.
Definition at line 44 of file khamburgermenu.cpp.
◆ setMenuBarAdvertised()
void KHamburgerMenu::setMenuBarAdvertised | ( | bool | advertise | ) |
By default the KHamburgerMenu contains a special sub-menu that advertises actions of the menu bar which would otherwise not be visible or discoverable for the user.
This method removes or re-adds that sub-menu.
- Parameters
-
advertise sets whether the special sub-menu that advertises menu bar only actions should exist.
Definition at line 75 of file khamburgermenu.cpp.
◆ setShowMenuBarAction()
void KHamburgerMenu::setShowMenuBarAction | ( | QAction * | showMenuBarAction | ) |
Adds the showMenuBarAction
as the first item of the sub-menu which advertises actions from the menu bar.
- See also
- setMenuBarAdvertised()
Definition at line 97 of file khamburgermenu.cpp.
◆ showActionsOf()
void KHamburgerMenu::showActionsOf | ( | QWidget * | widget | ) |
Reverses a hideActionsOf(widget) method call.
- See also
- hideActionsOf()
Definition at line 169 of file khamburgermenu.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:56:04 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.