KJobTrackerInterface
#include <KJobTrackerInterface>
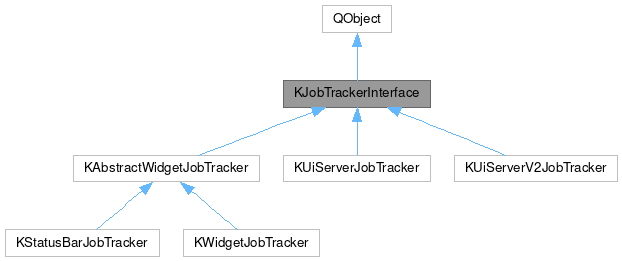
Public Slots | |
virtual void | registerJob (KJob *job) |
virtual void | unregisterJob (KJob *job) |
Public Member Functions | |
KJobTrackerInterface (QObject *parent=nullptr) | |
~KJobTrackerInterface () override | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
virtual void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1, const QPair< QString, QString > &field2) |
virtual void | finished (KJob *job) |
virtual void | infoMessage (KJob *job, const QString &message) |
virtual void | percent (KJob *job, unsigned long percent) |
virtual void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
virtual void | resumed (KJob *job) |
virtual void | speed (KJob *job, unsigned long value) |
virtual void | suspended (KJob *job) |
virtual void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
virtual void | warning (KJob *job, const QString &message) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The interface to implement to track the progresses of a job.
Definition at line 25 of file kjobtrackerinterface.h.
Constructor & Destructor Documentation
◆ KJobTrackerInterface()
|
explicit |
Creates a new KJobTrackerInterface.
- Parameters
-
parent the parent object
Definition at line 24 of file kjobtrackerinterface.cpp.
◆ ~KJobTrackerInterface()
|
overridedefault |
Destroys a KJobTrackerInterface.
Member Function Documentation
◆ description
|
protectedvirtualslot |
Called to display general description of a job.
A description has a title and two optional fields which can be used to complete the description.
Examples of titles are "Copying", "Creating resource", etc. The fields of the description can be "Source" with an URL, and, "Destination" with an URL for a "Copying" description.
- Parameters
-
job the job that emitted this signal title the general description of the job field1 first field (localized name and value) field2 second field (localized name and value)
Definition at line 70 of file kjobtrackerinterface.cpp.
◆ finished
|
protectedvirtualslot |
Called when a job is finished, in any case.
It is used to notify that the job is terminated and that progress UI (if any) can be hidden.
- Parameters
-
job the job that emitted this signal
Definition at line 55 of file kjobtrackerinterface.cpp.
◆ infoMessage
Called to display state information about a job.
Examples of message are "Resolving host", "Connecting to host...", etc.
- Parameters
-
job the job that emitted this signal message the info message
Definition at line 78 of file kjobtrackerinterface.cpp.
◆ percent
|
protectedvirtualslot |
Called to show the overall progress of the job.
Note that this is not called for finished jobs.
- Parameters
-
job the job that emitted this signal percent the percentage
Definition at line 104 of file kjobtrackerinterface.cpp.
◆ processedAmount
|
protectedvirtualslot |
Regularly called to show the progress of a job by giving the current amount.
The unit of this amount is provided too. It can be called several times for a given job if the job manages several different units.
- Parameters
-
job the job that emitted this signal unit the unit of the processed amount amount the processed amount
Definition at line 97 of file kjobtrackerinterface.cpp.
◆ registerJob
|
virtualslot |
Register a new job in this tracker.
The default implementation connects the following KJob signals to the respective protected slots of this class:
- finished() (also connected to the unregisterJob() slot)
- suspended()
- resumed()
- description()
- infoMessage()
- totalAmount()
- processedAmount()
- percent()
- speed()
If you re-implement this method, you may want to call the default implementation or add at least:
so that you won't have to manually call unregisterJob().
- Parameters
-
job the job to register
- See also
- unregisterJob()
Reimplemented in KStatusBarJobTracker, KUiServerJobTracker, and KUiServerV2JobTracker.
Definition at line 34 of file kjobtrackerinterface.cpp.
◆ resumed
|
protectedvirtualslot |
Called when a job is resumed.
- Parameters
-
job the job that emitted this signal
Definition at line 65 of file kjobtrackerinterface.cpp.
◆ speed
|
protectedvirtualslot |
Called to show the speed of the job.
- Parameters
-
job the job that emitted this signal value the current speed of the job
Definition at line 110 of file kjobtrackerinterface.cpp.
◆ suspended
|
protectedvirtualslot |
Called when a job is suspended.
- Parameters
-
job the job that emitted this signal
Definition at line 60 of file kjobtrackerinterface.cpp.
◆ totalAmount
|
protectedvirtualslot |
Called when we know the amount a job will have to process.
The unit of this amount is provided too. It can be called several times for a given job if the job manages several different units.
- Parameters
-
job the job that emitted this signal unit the unit of the total amount amount the total amount
Definition at line 90 of file kjobtrackerinterface.cpp.
◆ unregisterJob
|
virtualslot |
Unregister a job from this tracker.
- Note
- You need to manually call this method only if you re-implemented registerJob() without connecting KJob::finished to this slot.
- Parameters
-
job the job to unregister
- See also
- registerJob()
Reimplemented in KStatusBarJobTracker, KUiServerJobTracker, and KUiServerV2JobTracker.
Definition at line 50 of file kjobtrackerinterface.cpp.
◆ warning
Emitted to display a warning about a job.
- Parameters
-
job the job that emitted this signal message the warning message
Definition at line 84 of file kjobtrackerinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:04:24 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.