KNetworkMounts
#include <KNetworkMounts>
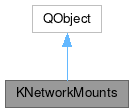
Public Types | |
enum | KNetworkMountOption { LowSideEffectsOptimizations , MediumSideEffectsOptimizations , StrongSideEffectsOptimizations , KDirWatchDontAddWatches , SymlinkPathsUseCache } |
enum | KNetworkMountsType { NfsPaths , SmbPaths , SymlinkDirectory , SymlinkToNetworkMount , Any } |
Public Member Functions | |
void | addPath (const QString &path, KNetworkMountsType type) |
QString | canonicalSymlinkPath (const QString &path) |
void | clearCache () |
bool | isEnabled () const |
bool | isOptionEnabled (const KNetworkMountOption option, const bool defaultValue=false) const |
bool | isOptionEnabledForPath (const QString &path, KNetworkMountOption option) |
bool | isSlowPath (const QString &path, KNetworkMountsType type=Any) |
QStringList | paths (KNetworkMountsType type=Any) const |
void | setEnabled (bool value) |
void | setOption (const KNetworkMountOption option, const bool value) |
void | setPaths (const QStringList &paths, KNetworkMountsType type) |
void | sync () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KNetworkMounts * | self () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Performance control on network mounts.
This class provides methods for deciding whether operations on slow network mounts should be performed or not.
Configuration is read from a configuration file network_mounts in the user's QStandardPaths::ConfigLocation. This file can be filled by using the network mounts performance configuration module or directly via setEnabled, setPaths, addPath and setOption
Use KNetworkMounts like this to check if the given url is on a configured slow path and the KNetworkMountOption LowSideEffectsOptimizations is enabled:
If called for the first time, this creates a singleton instance and reads the config file. Subsequent calls just use this instance without reading the config file again.
- Since
- 5.85
Definition at line 58 of file knetworkmounts.h.
Member Enumeration Documentation
◆ KNetworkMountOption
The KNetworkMountOption enum.
Uses are:
Enumerator | |
---|---|
LowSideEffectsOptimizations | Don't run KDiskFreeSpaceInfo if slow path.
|
MediumSideEffectsOptimizations | Don't return project for dir, avoid QFileInfo().absoluteDir() |
StrongSideEffectsOptimizations | Turn off symbolic link resolution. |
KDirWatchDontAddWatches | Disables dir watching completely for slow paths, avoids stat() calls on added dirs and subdirs. |
SymlinkPathsUseCache | Cache resolved symlink paths. |
Definition at line 75 of file knetworkmounts.h.
◆ KNetworkMountsType
The KNetworkMountsType enum.
Definition at line 102 of file knetworkmounts.h.
Member Function Documentation
◆ addPath()
void KNetworkMounts::addPath | ( | const QString & | path, |
KNetworkMountsType | type ) |
Add a path for which optimizations are to take place.
- Parameters
-
path the path to add type the type of the path. Do not use Any
- See also
- KNetworkMountsType
Definition at line 109 of file knetworkmounts.cpp.
◆ canonicalSymlinkPath()
Resolves a path
that may contain symbolic links to mounted network shares.
A symlink path is either a directory which contains symbolic links to slow network mounts (SymlinkDirectory) or a direct symbolic link to a slow network mount (SymlinkToNfsOrSmbPaths).
Example: There are some Samba shares mounted below /mnt. These are paths of type SmbPaths
A (logged in) user may have symbolic links to them in his home directory below netshares. The directory /home/user/netshares is a SymlinkDirectory:
There is a direct symbolic link from /home/user/share1 to /mnt/server1/share1. This is of type SymlinkToNfsOrSmbPaths:
Both types of symbolic links from symlink paths to the real mounted shares are resolved even if KNetworkMountOption StrongSideEffectsOptimizations is enabled.
If the setup is like above a path
would be resolved to
and a path
would also be resolved to
Resolved paths are cached in a hash.
- Parameters
-
path the path to resolve
- Returns
- the resolved path or
path
ifpath
is not a symlink path or no symlink found
- See also
- KNetworkMountsType
- clearCache
- isSlowPath
Definition at line 121 of file knetworkmounts.cpp.
◆ clearCache()
void KNetworkMounts::clearCache | ( | ) |
Clears the canonical symlink path cache.
Call this if directory structures on mounted network drives changed. Don't enable the cache (SymlinkPathsUseCache) if this happens often and the drives are usually accessed via the symlinks. This method exists mainly for the KCM.
- See also
- canonicalSymlinkPath
Definition at line 207 of file knetworkmounts.cpp.
◆ isEnabled()
bool KNetworkMounts::isEnabled | ( | ) | const |
Query if the performance optimizations are switched on.
- Returns
true
if on,false
otherwise
Definition at line 67 of file knetworkmounts.cpp.
◆ isOptionEnabled()
bool KNetworkMounts::isOptionEnabled | ( | const KNetworkMountOption | option, |
const bool | defaultValue = false ) const |
Query a performance option.
- Parameters
-
option the option to query defaultValue the value to return if the option is not configured
- Returns
true
if option is on,false
if not
- See also
- KNetworkMountOption
Definition at line 77 of file knetworkmounts.cpp.
◆ isOptionEnabledForPath()
bool KNetworkMounts::isOptionEnabledForPath | ( | const QString & | path, |
KNetworkMountOption | option ) |
Query if path
is configured to be a slow path and option
is enabled.
- Parameters
-
path the path to query option the option to query
- Returns
true
ifpath
is a configured slow path and optionoption
is enabled
Definition at line 54 of file knetworkmounts.cpp.
◆ isSlowPath()
bool KNetworkMounts::isSlowPath | ( | const QString & | path, |
KNetworkMountsType | type = Any ) |
Query if path
is configured to be a slow path of type type
.
- Parameters
-
path the path to query type the type to query. If omitted, any type matches
- Returns
true
ifpath
is a configured slow path of typetype
This function is also used to determine the filesystem type in KFileSystemType::fileSystemType (KFileSystemType::Smb or KFileSystemType::Nfs) without an expensive call to stafs(). For this to work the types of paths need to be correctly assigned in setPath or addPath
Definition at line 49 of file knetworkmounts.cpp.
◆ paths()
QStringList KNetworkMounts::paths | ( | KNetworkMountsType | type = Any | ) | const |
Query the configured paths for which optimizations are to take place.
- Returns
- a list of paths
Definition at line 87 of file knetworkmounts.cpp.
◆ self()
|
static |
Returns (and creates if necessary) the singleton instance.
- Returns
- the singleton instance
Definition at line 23 of file knetworkmounts.cpp.
◆ setEnabled()
void KNetworkMounts::setEnabled | ( | bool | value | ) |
Switch the performance optimizations on or off.
- Parameters
-
value the value to set
Definition at line 72 of file knetworkmounts.cpp.
◆ setOption()
void KNetworkMounts::setOption | ( | const KNetworkMountOption | option, |
const bool | value ) |
Switch a performance option on or off.
- Parameters
-
option the option to change value the value to set
- See also
- KNetworkMountOption
Definition at line 82 of file knetworkmounts.cpp.
◆ setPaths()
void KNetworkMounts::setPaths | ( | const QStringList & | paths, |
KNetworkMountsType | type ) |
Set the paths for which optimizations are to take place.
- Parameters
-
paths the paths to set type the type of paths. Do not use Any
- See also
- KNetworkMountsType
Definition at line 102 of file knetworkmounts.cpp.
◆ sync()
void KNetworkMounts::sync | ( | ) |
Synchronizes to config file.
QSettings synchronization also takes place automatically at regular intervals and from QSettings destructor, see QSettings::sync() documentation.
Calls QSettings::sync()
Definition at line 214 of file knetworkmounts.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:47:38 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.