KDESu::SuProcess
#include <KDESu/SuProcess>
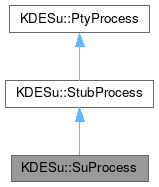
Public Types | |
enum | checkMode { NoCheck = 0 , Install = 1 , NeedPassword = 2 } |
enum | Errors { SuNotFound = 1 , SuNotAllowed , SuIncorrectPassword } |
![]() | |
enum | Scheduler { SchedNormal , SchedRealtime } |
![]() | |
enum | checkPidStatus { Error = -1 , NotExited = -2 , Killed = -3 } |
Public Member Functions | |
SuProcess (const QByteArray &user=nullptr, const QByteArray &command=nullptr) | |
int | checkInstall (const char *password) |
int | checkNeedPassword () |
int | exec (const char *password, int check=NoCheck) |
QString | superUserCommand () |
bool | useUsersOwnPassword () |
![]() | |
void | setCommand (const QByteArray &command) |
void | setPriority (int prio) |
void | setScheduler (int sched) |
void | setUser (const QByteArray &user) |
void | setXOnly (bool xonly) |
![]() | |
int | enableLocalEcho (bool enable=true) |
int | exec (const QByteArray &command, const QList< QByteArray > &args) |
int | fd () const |
int | pid () const |
QByteArray | readAll (bool block=true) |
QByteArray | readLine (bool block=true) |
void | setEnvironment (const QList< QByteArray > &env) |
void | setErase (bool erase) |
void | setExitString (const QByteArray &exit) |
void | setTerminal (bool terminal) |
void | unreadLine (const QByteArray &line, bool addNewline=true) |
int | waitForChild () |
int | waitSlave () |
void | writeLine (const QByteArray &line, bool addNewline=true) |
Protected Member Functions | |
void | virtual_hook (int id, void *data) override |
![]() | |
KDESU_NO_EXPORT | StubProcess (StubProcessPrivate &dd) |
int | converseStub (int check) |
virtual QByteArray | display () |
virtual QByteArray | displayAuth () |
![]() | |
KDESU_NO_EXPORT | PtyProcess (PtyProcessPrivate &dd) |
QList< QByteArray > | environment () const |
Additional Inherited Members | |
![]() | |
static bool | checkPid (pid_t pid) |
static int | checkPidExited (pid_t pid) |
static int | waitMS (int fd, int ms) |
![]() | |
QByteArray | m_command |
KDESuPrivate::KCookie * | m_cookie |
int | m_priority |
int | m_scheduler |
QByteArray | m_user |
bool | m_XOnly |
![]() | |
std::unique_ptr< PtyProcessPrivate > const | d_ptr |
QByteArray | m_command |
bool | m_erase |
QByteArray | m_exitString |
int | m_pid |
bool | m_terminal |
Detailed Description
Executes a command under elevated privileges, using su.
Definition at line 23 of file suprocess.h.
Member Enumeration Documentation
◆ checkMode
Executes the command.
This will wait for the command to finish.
Definition at line 35 of file suprocess.h.
◆ Errors
enum KDESu::SuProcess::Errors |
Definition at line 26 of file suprocess.h.
Constructor & Destructor Documentation
◆ SuProcess()
|
explicit |
Definition at line 52 of file suprocess.cpp.
Member Function Documentation
◆ checkInstall()
int KDESu::SuProcess::checkInstall | ( | const char * | password | ) |
Checks if the stub is installed and the password is correct.
- Returns
- Zero if everything is correct, nonzero otherwise.
Definition at line 91 of file suprocess.cpp.
◆ checkNeedPassword()
int KDESu::SuProcess::checkNeedPassword | ( | ) |
Checks if a password is needed.
Definition at line 96 of file suprocess.cpp.
◆ exec()
int KDESu::SuProcess::exec | ( | const char * | password, |
int | check = NoCheck ) |
Definition at line 104 of file suprocess.cpp.
◆ superUserCommand()
QString KDESu::SuProcess::superUserCommand | ( | ) |
Checks what the default super user command is, e.g.
sudo, su, etc
- Returns
- the default super user command
Definition at line 72 of file suprocess.cpp.
◆ useUsersOwnPassword()
bool KDESu::SuProcess::useUsersOwnPassword | ( | ) |
Checks whether or not the user's password is being asked for or another user's password.
Due to usage of systems such as sudo, even when attempting to switch to another user one may need to enter their own password.
Definition at line 79 of file suprocess.cpp.
◆ virtual_hook()
|
overrideprotectedvirtual |
Standard hack to add virtual methods in a BC way.
Unused.
Reimplemented from KDESu::StubProcess.
Definition at line 306 of file suprocess.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:54:15 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.