KDESu::PtyProcess
#include <KDESu/PtyProcess>
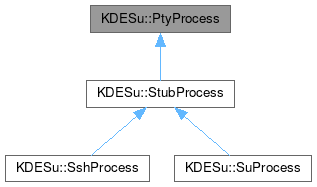
Public Types | |
enum | checkPidStatus { Error = -1 , NotExited = -2 , Killed = -3 } |
Public Member Functions | |
int | enableLocalEcho (bool enable=true) |
int | exec (const QByteArray &command, const QList< QByteArray > &args) |
int | fd () const |
int | pid () const |
QByteArray | readAll (bool block=true) |
QByteArray | readLine (bool block=true) |
void | setEnvironment (const QList< QByteArray > &env) |
void | setErase (bool erase) |
void | setExitString (const QByteArray &exit) |
void | setTerminal (bool terminal) |
void | unreadLine (const QByteArray &line, bool addNewline=true) |
int | waitForChild () |
int | waitSlave () |
void | writeLine (const QByteArray &line, bool addNewline=true) |
Static Public Member Functions | |
static bool | checkPid (pid_t pid) |
static int | checkPidExited (pid_t pid) |
static int | waitMS (int fd, int ms) |
Protected Member Functions | |
KDESU_NO_EXPORT | PtyProcess (PtyProcessPrivate &dd) |
QList< QByteArray > | environment () const |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
std::unique_ptr< PtyProcessPrivate > const | d_ptr |
QByteArray | m_command |
bool | m_erase |
QByteArray | m_exitString |
int | m_pid |
bool | m_terminal |
Detailed Description
Synchronous communication with tty programs.
PtyProcess provides synchronous communication with tty based programs. The communications channel used is a pseudo tty (as opposed to a pipe) This means that programs which require a terminal will work.
Definition at line 35 of file ptyprocess.h.
Member Enumeration Documentation
◆ checkPidStatus
Error return values for checkPidExited()
Enumerator | |
---|---|
Error | No child. |
NotExited | Child hasn't exited. |
Killed | Child terminated by signal. |
Definition at line 39 of file ptyprocess.h.
Constructor & Destructor Documentation
◆ PtyProcess() [1/2]
KDESu::PtyProcess::PtyProcess | ( | ) |
Definition at line 115 of file ptyprocess.cpp.
◆ PtyProcess() [2/2]
|
explicitprotected |
Definition at line 120 of file ptyprocess.cpp.
Member Function Documentation
◆ checkPid()
|
static |
Basic check for the existence of pid
.
Returns true iff pid
is an extant process, (one you could kill - see man kill(2) for signal 0).
Definition at line 73 of file ptyprocess.cpp.
◆ checkPidExited()
|
static |
Check process exit status for process pid
.
If child pid
has exited, return its exit status, (which may be zero). On error (no child, no exit), return -1. If child has
not exited, return -2.
Definition at line 93 of file ptyprocess.cpp.
◆ enableLocalEcho()
int KDESu::PtyProcess::enableLocalEcho | ( | bool | enable = true | ) |
Enables/disables local echo on the pseudo tty.
Definition at line 393 of file ptyprocess.cpp.
◆ environment()
|
protected |
Returns the additional environment variables set by setEnvironment()
Definition at line 168 of file ptyprocess.cpp.
◆ exec()
int KDESu::PtyProcess::exec | ( | const QByteArray & | command, |
const QList< QByteArray > & | args ) |
Forks off and execute a command.
The command's standard in and output are connected to the pseudo tty. They are accessible with readLine and writeLine.
- Parameters
-
command The command to execute. args The arguments to the command.
- Returns
- 0 on success, -1 on error. errno might give more information then.
Definition at line 282 of file ptyprocess.cpp.
◆ fd()
int KDESu::PtyProcess::fd | ( | ) | const |
Returns the filedescriptor of the process.
Definition at line 155 of file ptyprocess.cpp.
◆ pid()
int KDESu::PtyProcess::pid | ( | ) | const |
Returns the pid of the process.
Definition at line 162 of file ptyprocess.cpp.
◆ readAll()
QByteArray KDESu::PtyProcess::readAll | ( | bool | block = true | ) |
Read all available output from the program's standard out.
- Parameters
-
block If no output is in the buffer, should the function block (else it will return an empty QByteArray)?
- Returns
- The output.
Definition at line 175 of file ptyprocess.cpp.
◆ readLine()
QByteArray KDESu::PtyProcess::readLine | ( | bool | block = true | ) |
Reads a line from the program's standard out.
Depending on the block parameter, this call blocks until something was read. Note that in some situations this function will return less than a full line of output, but never more. Newline characters are stripped.
- Parameters
-
block Block until a full line is read?
- Returns
- The output string.
Definition at line 228 of file ptyprocess.cpp.
◆ setEnvironment()
void KDESu::PtyProcess::setEnvironment | ( | const QList< QByteArray > & | env | ) |
Set additinal environment variables.
Set additional environment variables.
Definition at line 148 of file ptyprocess.cpp.
◆ setErase()
void KDESu::PtyProcess::setErase | ( | bool | erase | ) |
Overwrites the password as soon as it is used.
Relevant only to some subclasses.
Definition at line 411 of file ptyprocess.cpp.
◆ setExitString()
void KDESu::PtyProcess::setExitString | ( | const QByteArray & | exit | ) |
Sets the exit string.
If a line of program output matches this, waitForChild() will terminate the program and return.
Definition at line 274 of file ptyprocess.cpp.
◆ setTerminal()
void KDESu::PtyProcess::setTerminal | ( | bool | terminal | ) |
Enables/disables terminal output.
Relevant only to some subclasses.
Definition at line 406 of file ptyprocess.cpp.
◆ unreadLine()
void KDESu::PtyProcess::unreadLine | ( | const QByteArray & | line, |
bool | addNewline = true ) |
Puts back a line of input.
- Parameters
-
line The line to put back. addNewline Adds a '
' to the line.
Definition at line 261 of file ptyprocess.cpp.
◆ virtual_hook()
|
protectedvirtual |
Standard hack to add virtual methods in a BC way.
Unused.
Reimplemented in KDESu::SshProcess, KDESu::StubProcess, and KDESu::SuProcess.
Definition at line 545 of file ptyprocess.cpp.
◆ waitForChild()
int KDESu::PtyProcess::waitForChild | ( | ) |
◆ waitMS()
|
static |
Wait ms
milliseconds (ie.
1/10th of a second is 100ms), using fd
as a filedescriptor to wait on. Returns select(2)'s result, which is -1 on error, 0 on timeout, or positive if there is data on one of the selected fd's.
ms
must be in the range 0..999 (i.e. the maximum wait duration is 999ms, almost one second).
Definition at line 54 of file ptyprocess.cpp.
◆ waitSlave()
int KDESu::PtyProcess::waitSlave | ( | ) |
Waits until the pty has cleared the ECHO flag.
This is useful when programs write a password prompt before they disable ECHO. Disabling it might flush any input that was written.
Definition at line 368 of file ptyprocess.cpp.
◆ writeLine()
void KDESu::PtyProcess::writeLine | ( | const QByteArray & | line, |
bool | addNewline = true ) |
Writes a line of text to the program's standard in.
- Parameters
-
line The text to write. addNewline Adds a '
' to the line.
Definition at line 251 of file ptyprocess.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 194 of file ptyprocess.h.
◆ m_command
|
protected |
Unused.
Definition at line 186 of file ptyprocess.h.
◆ m_erase
|
protected |
- See also
- setErase()
Definition at line 181 of file ptyprocess.h.
◆ m_exitString
|
protected |
String to scan for in output that indicates child has exited.
Definition at line 187 of file ptyprocess.h.
◆ m_pid
|
protected |
PID of child process.
Definition at line 185 of file ptyprocess.h.
◆ m_terminal
|
protected |
Indicates running in a terminal, causes additional newlines to be printed after output.
Set to false
in constructor.
- See also
- setTerminal()
Definition at line 182 of file ptyprocess.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:57:38 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.