KDNSSD::PublicService
#include <KDNSSD/PublicService>
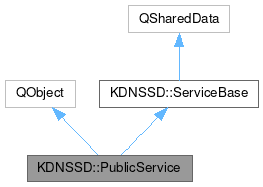
Signals | |
void | published (bool successful) |
Public Member Functions | |
PublicService (const QString &name=QString(), const QString &type=QString(), unsigned int port=0, const QString &domain=QString(), const QStringList &subtypes=QStringList()) | |
bool | isPublished () const |
bool | publish () |
void | publishAsync () |
void | setDomain (const QString &domain) |
void | setPort (unsigned short port) |
void | setServiceName (const QString &serviceName) |
void | setSubTypes (const QStringList &subtypes) |
void | setTextData (const QMap< QString, QByteArray > &textData) |
void | setType (const QString &type) |
void | stop () |
QStringList | subtypes () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
ServiceBase (const QString &name=QString(), const QString &type=QString(), const QString &domain=QString(), const QString &host=QString(), unsigned short port=0) | |
QString | domain () const |
QString | hostName () const |
bool | operator!= (const ServiceBase &o) const |
bool | operator== (const ServiceBase &o) const |
unsigned short | port () const |
QString | serviceName () const |
QMap< QString, QByteArray > | textData () const |
QString | type () const |
![]() | |
QSharedData (const QSharedData &) | |
Protected Member Functions | |
void | virtual_hook (int, void *) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
KDNSSD_NO_EXPORT | ServiceBase (ServiceBasePrivate *const d) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
typedef QExplicitlySharedDataPointer< ServiceBase > | Ptr |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
std::unique_ptr< ServiceBasePrivate > const | d |
Detailed Description
Represents a service to be published.
This class allows you to publish the existence of a network service provided by your application.
If you are providing a web server and want to advertise it on the local network, you might do
In this example publish() is synchronous: it will not return until publishing is complete. This is usually not too long but it can freeze an application's GUI for a moment. To publish asynchronously instead, do:
Definition at line 47 of file publicservice.h.
Constructor & Destructor Documentation
◆ PublicService()
|
explicit |
Creates a service description that can be published.
If no name
is given, the computer name is used instead. If there is already a service with the same name, type and domain a number will be appended to the name to make it unique.
If no domain
is specified, the service is published on the link-local domain (.local).
The subtypes can be used to specify server attributes, such as "_anon" for anonymous FTP servers, or can specify a specific protocol (such as a web service interface) on top of a generic protocol like SOAP.
There is a comprehensive list of possible types available, but you are largely on your own for subtypes.
- Parameters
-
name a service name to use instead of the computer name type service type, in the form _sometype._udp or _sometype._tcp port port number, or 0 to "reserve" the service name domain the domain to publish the service on (see DomainBrowser) subtypes optional list of subtypes, each with a leading underscore
- See also
- ServiceBrowser::ServiceBrowser()
Definition at line 26 of file avahi-publicservice.cpp.
◆ ~PublicService()
|
override |
Definition at line 37 of file avahi-publicservice.cpp.
Member Function Documentation
◆ isPublished()
bool KDNSSD::PublicService::isPublished | ( | ) | const |
Whether the service is currently published.
- Returns
true
if the service is being published to the domain,false
otherwise
Definition at line 126 of file avahi-publicservice.cpp.
◆ publish()
bool KDNSSD::PublicService::publish | ( | ) |
Publish the service synchronously.
The method will not return (and hence the application interface will freeze, since KDElibs code should be executed in the main thread) until either the service is published or publishing fails.
published(bool) is emitted before this method returns.
- Returns
true
if the service was successfully published,false
otherwise
Definition at line 132 of file avahi-publicservice.cpp.
◆ publishAsync()
void KDNSSD::PublicService::publishAsync | ( | ) |
Publish the service asynchronously.
Returns immediately and emits published(bool) when completed. Note that published(bool) may be emitted before this method returns when an error is detected immediately.
Definition at line 253 of file avahi-publicservice.cpp.
◆ published
|
signal |
Emitted when publishing is complete.
It will also emitted when an already-published service is republished after a property of the service (such as the name or port) is changed.
◆ setDomain()
void KDNSSD::PublicService::setDomain | ( | const QString & | domain | ) |
Sets the domain where the service is published.
"local." means link-local, ie: the IP subnet on the LAN containing this computer.
If service is already published, it will be removed from the current domain and published on domain
instead.
- Parameters
-
domain the new domain to publish the service on
Definition at line 70 of file avahi-publicservice.cpp.
◆ setPort()
void KDNSSD::PublicService::setPort | ( | unsigned short | port | ) |
Sets the port.
If the service is already published, it will be re-announced with the new port.
- Parameters
-
port the port of the service, or 0 to simply "reserve" the name
Definition at line 106 of file avahi-publicservice.cpp.
◆ setServiceName()
void KDNSSD::PublicService::setServiceName | ( | const QString & | serviceName | ) |
Sets the name of the service.
If the service is already published, it will be re-announced with the new name.
- Parameters
-
serviceName the new name of the service
Definition at line 60 of file avahi-publicservice.cpp.
◆ setSubTypes()
void KDNSSD::PublicService::setSubTypes | ( | const QStringList & | subtypes | ) |
Sets the subtypetypes of the service.
If the service is already published, it will be re-announced with the new subtypes.
The existing list of substypes is replaced, so an empty list will cause all existing subtypes to be removed.
- Parameters
-
subtypes the new list of subtypes
Definition at line 90 of file avahi-publicservice.cpp.
◆ setTextData()
void KDNSSD::PublicService::setTextData | ( | const QMap< QString, QByteArray > & | textData | ) |
Sets new text properties.
If the service is already published, it will be re-announced with the new data.
- Parameters
-
textData the new text properties for the service
- See also
- ServiceBase::textData()
Definition at line 116 of file avahi-publicservice.cpp.
◆ setType()
void KDNSSD::PublicService::setType | ( | const QString & | type | ) |
Sets the service type.
If the service is already published, it will be re-announced with the new type.
- Parameters
-
type the new type of the service
See PublicService() for details on the format of type
Definition at line 80 of file avahi-publicservice.cpp.
◆ stop()
void KDNSSD::PublicService::stop | ( | ) |
Stops publishing or aborts an incomplete publish request.
Useful when you want to disable the service for some time.
Note that if you stop providing a service (without exiting the application), you should stop publishing it.
Definition at line 142 of file avahi-publicservice.cpp.
◆ subtypes()
QStringList KDNSSD::PublicService::subtypes | ( | ) | const |
The subtypes of service.
- See also
- setSubTypes()
Definition at line 100 of file avahi-publicservice.cpp.
◆ virtual_hook()
|
overrideprotectedvirtual |
Reimplemented from KDNSSD::ServiceBase.
Definition at line 298 of file avahi-publicservice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:06:09 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.