KDNSSD::RemoteService
#include <KDNSSD/RemoteService>
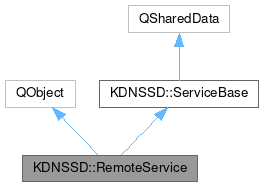
Public Types | |
typedef QExplicitlySharedDataPointer< RemoteService > | Ptr |
![]() | |
typedef | QObjectList |
![]() | |
typedef QExplicitlySharedDataPointer< ServiceBase > | Ptr |
Signals | |
void | resolved (bool successful) |
Public Member Functions | |
RemoteService (const QString &name, const QString &type, const QString &domain) | |
bool | isResolved () const |
bool | resolve () |
void | resolveAsync () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
ServiceBase (const QString &name=QString(), const QString &type=QString(), const QString &domain=QString(), const QString &host=QString(), unsigned short port=0) | |
QString | domain () const |
QString | hostName () const |
bool | operator!= (const ServiceBase &o) const |
bool | operator== (const ServiceBase &o) const |
unsigned short | port () const |
QString | serviceName () const |
QMap< QString, QByteArray > | textData () const |
QString | type () const |
![]() | |
QSharedData (const QSharedData &) | |
Protected Member Functions | |
void | virtual_hook (int id, void *data) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
KDNSSD_NO_EXPORT | ServiceBase (ServiceBasePrivate *const d) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
std::unique_ptr< ServiceBasePrivate > const | d |
Detailed Description
Describes a service published over DNS-SD, typically on a remote machine.
This class allows delayed or asynchronous resolution of services. As the name suggests, the service is normally on a remote machine, but the service could just as easily be published on the local machine.
RemoteService instances are normally provided by ServiceBrowser, but can be used to resolve any service if you know the name, type and domain for it.
- See also
- ServiceBrowser
Definition at line 38 of file remoteservice.h.
Member Typedef Documentation
◆ Ptr
Definition at line 43 of file remoteservice.h.
Constructor & Destructor Documentation
◆ RemoteService()
KDNSSD::RemoteService::RemoteService | ( | const QString & | name, |
const QString & | type, | ||
const QString & | domain ) |
Creates an unresolved RemoteService representing the service with the given name, type and domain.
- Parameters
-
name the name of the service type the type of the service (see ServiceBrowser::ServiceBrowser()) domain the domain of the service
- See also
- ServiceBrowser::isAvailable()
Definition at line 20 of file avahi-remoteservice.cpp.
◆ ~RemoteService()
|
override |
Definition at line 25 of file avahi-remoteservice.cpp.
Member Function Documentation
◆ isResolved()
bool KDNSSD::RemoteService::isResolved | ( | ) | const |
Whether the service has been successfully resolved.
- Returns
true
if hostName() and port() will return valid values,false
otherwise
Definition at line 88 of file avahi-remoteservice.cpp.
◆ resolve()
bool KDNSSD::RemoteService::resolve | ( | ) |
Resolves the host name and port of service synchronously.
The host name is not resolved into an IP address - use KResolver for that.
resolved(bool) is emitted before this function is returned.
resolve() will not cause RemoteService to monitor for changes in the hostname or port of the service.
- Returns
true
if successful,false
on failure
- See also
- resolveAsync(), hostName(), port()
Definition at line 29 of file avahi-remoteservice.cpp.
◆ resolveAsync()
void KDNSSD::RemoteService::resolveAsync | ( | ) |
Resolves the host name and port of service asynchronously.
The host name is not resolved into an IP address - use KResolver for that.
The resolved(bool) signal will be emitted when the resolution is complete, or when it fails.
Note that resolved(bool) may be emitted before this function returns in case of immediate failure.
RemoteService will keep monitoring the service for changes in hostname and port, and re-emit resolved(bool) when either changes.
- See also
- resolve(), hostName(), port()
Definition at line 39 of file avahi-remoteservice.cpp.
◆ resolved
|
signal |
Emitted when resolving is complete.
If operating in asynchronous mode this signal can be emitted several times (when the hostName or port of the service changes).
- Parameters
-
successful true
if the hostName and port were successfully resolved,false
otherwise
◆ virtual_hook()
|
overrideprotectedvirtual |
Reimplemented from KDNSSD::ServiceBase.
Definition at line 167 of file avahi-remoteservice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:15:07 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.