KIconLoader
#include <KIconLoader>
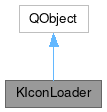
Public Types | |
enum | Context { Any , Action , Application , Device , MimeType , Animation , Category , Emblem , Emote , International , Place , StatusIcon } |
enum | Group { NoGroup = -1 , Desktop = 0 , FirstGroup = 0 , Toolbar , MainToolbar , Small , Panel , Dialog , LastGroup , User } |
enum | MatchType { MatchExact , MatchBest , MatchBestOrGreaterSize } |
enum | States { DefaultState , ActiveState , DisabledState , SelectedState , LastState } |
enum | StdSizes { SizeSmall = 16 , SizeSmallMedium = 22 , SizeMedium = 32 , SizeLarge = 48 , SizeHuge = 64 , SizeEnormous = 128 } |
enum | Type { Fixed , Scalable , Threshold } |
Signals | |
void | iconChanged (int group) |
void | iconLoaderSettingsChanged () |
Public Slots | |
static void | emitChange (Group group) |
void | newIconLoader () |
Public Member Functions | |
KIconLoader (const QString &appname=QString(), const QStringList &extraSearchPaths=QStringList(), QObject *parent=nullptr) | |
~KIconLoader () override | |
void | addAppDir (const QString &appname, const QString &themeBaseDir=QString()) |
int | currentSize (KIconLoader::Group group) const |
QPalette | customPalette () const |
void | drawOverlays (const QStringList &overlays, QPixmap &pixmap, KIconLoader::Group group, int state=KIconLoader::DefaultState) const |
bool | hasContext (KIconLoader::Context context) const |
bool | hasCustomPalette () const |
bool | hasIcon (const QString &iconName) const |
KIconEffect * | iconEffect () const |
QString | iconPath (const QString &name, int group_or_size, bool canReturnNull, qreal scale) const |
QString | iconPath (const QString &name, int group_or_size, bool canReturnNull=false) const |
QStringList | loadAnimated (const QString &name, KIconLoader::Group group, int size=0) const |
QPixmap | loadIcon (const QString &name, KIconLoader::Group group, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList(), QString *path_store=nullptr, bool canReturnNull=false) const |
QPixmap | loadMimeTypeIcon (const QString &iconName, KIconLoader::Group group, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList(), QString *path_store=nullptr) const |
QMovie * | loadMovie (const QString &name, KIconLoader::Group group, int size=0, QObject *parent=nullptr) const |
QPixmap | loadScaledIcon (const QString &name, KIconLoader::Group group, qreal scale, const QSize &size={}, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList(), QString *path_store=nullptr, bool canReturnNull=false) const |
QPixmap | loadScaledIcon (const QString &name, KIconLoader::Group group, qreal scale, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList(), QString *path_store=nullptr, bool canReturnNull=false) const |
QString | moviePath (const QString &name, KIconLoader::Group group, int size=0) const |
QStringList | queryIcons () const |
QStringList | queryIcons (int group_or_size, KIconLoader::Context context=KIconLoader::Any) const |
QStringList | queryIconsByContext (int group_or_size, KIconLoader::Context context=KIconLoader::Any) const |
QStringList | queryIconsByDir (const QString &iconsDir) const |
void | reconfigure (const QString &appname, const QStringList &extraSearchPaths=QStringList()) |
void | resetPalette () |
QStringList | searchPaths () const |
void | setCustomPalette (const QPalette &palette) |
KIconTheme * | theme () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KIconLoader * | global () |
static QPixmap | unknown () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Related Symbols | |
(Note that these are not member symbols.) | |
KICONTHEMES_EXPORT QIcon | icon (const QString &iconName, const QStringList &overlays, KIconLoader *iconLoader=nullptr) |
KICONTHEMES_EXPORT QIcon | icon (const QString &iconName, KIconLoader *iconLoader=nullptr) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Iconloader for KDE.
- Note
- For most icon loading use cases perfer using QIcon::fromTheme
KIconLoader will load the current icon theme and all its base themes. Icons will be searched in any of these themes. Additionally, it caches icons and applies effects according to the user's preferences.
In KDE, it is encouraged to load icons by "Group". An icon group is a location on the screen where icons are being used. Standard groups are: Desktop, Toolbar, MainToolbar, Small and Panel. Each group can have some centrally-configured effects applied to its icons. This makes it possible to offer a consistent icon look in all KDE applications.
The standard groups are defined below.
- KIconLoader::Desktop: Icons in the iconview of konqueror, kdesktop and similar apps.
- KIconLoader::Toolbar: Icons in toolbars.
- KIconLoader::MainToolbar: Icons in the main toolbars.
- KIconLoader::Small: Various small (typical 16x16) places: titlebars, listviews and menu entries.
- KIconLoader::Panel: Icons in kicker's panel
The icons are stored on disk in an icon theme or in a standalone directory. The icon theme directories contain multiple sizes and/or depths for the same icon. The iconloader will load the correct one based on the icon group and the current theme. Icon themes are stored globally in share/icons, or, application specific in share/apps/$appdir/icons.
The standalone directories contain just one version of an icon. The directories that are searched are: $appdir/pics and $appdir/toolbar. Icons in these directories can be loaded by using the special group "User".
Definition at line 72 of file kiconloader.h.
Member Enumeration Documentation
◆ Context
enum KIconLoader::Context |
Defines the context of the icon.
Definition at line 80 of file kiconloader.h.
◆ Group
enum KIconLoader::Group |
The group of the icon.
Enumerator | |
---|---|
NoGroup | No group. |
Desktop | Desktop icons. |
FirstGroup | First group. |
Toolbar | Toolbar icons. |
MainToolbar | Main toolbar icons. |
Small | Small icons, e.g. for buttons. |
Panel | Panel (Plasma Taskbar) icons. |
Dialog | Icons for use in dialog titles, page lists, etc. |
LastGroup | Last group. |
User | User icons. |
Definition at line 119 of file kiconloader.h.
◆ MatchType
The type of a match.
Definition at line 109 of file kiconloader.h.
◆ States
enum KIconLoader::States |
Defines the possible states of an icon.
Enumerator | |
---|---|
DefaultState | The default state. |
ActiveState | Icon is active. |
DisabledState | Icon is disabled. |
SelectedState | Icon is selected.
|
LastState | Last state (last constant) |
Definition at line 166 of file kiconloader.h.
◆ StdSizes
These are the standard sizes for icons.
Definition at line 147 of file kiconloader.h.
◆ Type
enum KIconLoader::Type |
The type of the icon.
Enumerator | |
---|---|
Fixed | Fixed-size icon. |
Scalable | Scalable-size icon. |
Threshold | A threshold icon. |
Definition at line 99 of file kiconloader.h.
Constructor & Destructor Documentation
◆ KIconLoader()
|
explicit |
Constructs an iconloader.
- Parameters
-
appname Add the data directories of this application to the icon search path for the "User" group. The default argument adds the directories of the current application. extraSearchPaths additional search paths, either absolute or relative to GenericDataLocation
Usually, you use the default iconloader, which can be accessed via KIconLoader::global(), so you hardly ever have to create an iconloader object yourself. That one is the application's iconloader.
Definition at line 390 of file kiconloader.cpp.
◆ ~KIconLoader()
|
overridedefault |
Cleanup.
Member Function Documentation
◆ addAppDir()
Adds appname
to the list of application specific directories with themeBaseDir
as its base directory.
Assume the icons are in /home/user/app/icons/hicolor/48x48/my_app.png, the base directory would be /home/user/app/icons; KIconLoader automatically searches themeBaseDir
+ "/hicolor" This directory must contain a dir structure as defined by the XDG icons specification
- Parameters
-
appname The application name. themeBaseDir The base directory of the application's theme (eg. "/home/user/app/icons")
Definition at line 476 of file kiconloader.cpp.
◆ currentSize()
int KIconLoader::currentSize | ( | KIconLoader::Group | group | ) | const |
Returns the size of the specified icon group.
Using e.g. KIconLoader::SmallIcon will return 16.
- Parameters
-
group the group to check.
- Returns
- the current size for an icon group.
Definition at line 1342 of file kiconloader.cpp.
◆ customPalette()
QPalette KIconLoader::customPalette | ( | ) | const |
The colors that will be used for the svg stylesheet in case the loaded icons are svg-based, icons can be colored in different ways in different areas of the application.
- Returns
- the palette, if any, an invalid one if the user didn't set it
- Since
- 5.39
Definition at line 1471 of file kiconloader.cpp.
◆ drawOverlays()
void KIconLoader::drawOverlays | ( | const QStringList & | overlays, |
QPixmap & | pixmap, | ||
KIconLoader::Group | group, | ||
int | state = KIconLoader::DefaultState ) const |
Draws overlays on the specified pixmap, it takes the width and height of the pixmap into consideration.
- Parameters
-
overlays List of up to 4 overlays to blend over the pixmap. The first overlay will be in the bottom right corner, followed by bottom left, top left and top right. An empty QString can be used to leave the specific position blank. pixmap to draw on
- Since
- 4.7
- Deprecated
- since 6.5, use KIconUtils::addOverlays from KGuiAddons
Definition at line 592 of file kiconloader.cpp.
◆ emitChange
|
staticslot |
Emits an iconChanged() signal on all the KIconLoader instances in the system indicating that a system's icon group has changed in some way.
It will also trigger a reload in all of them to update to the new theme.
group
indicates the group that has changed
- Since
- 5.0
Definition at line 1502 of file kiconloader.cpp.
◆ global()
|
static |
Returns the global icon loader initialized with the application name.
- Returns
- global icon loader
Definition at line 1486 of file kiconloader.cpp.
◆ hasContext()
bool KIconLoader::hasContext | ( | KIconLoader::Context | context | ) | const |
Definition at line 1424 of file kiconloader.cpp.
◆ hasCustomPalette()
bool KIconLoader::hasCustomPalette | ( | ) | const |
- Returns
- whether we have set a custom palette using @f setCustomPalette
- Since
- 5.85
- See also
- resetPalette, setCustomPalette
Definition at line 1481 of file kiconloader.cpp.
◆ hasIcon()
bool KIconLoader::hasIcon | ( | const QString & | iconName | ) | const |
Definition at line 1460 of file kiconloader.cpp.
◆ iconChanged
|
signal |
Emitted when the system icon theme changes.
- Since
- 5.0
◆ iconEffect()
KIconEffect * KIconLoader::iconEffect | ( | ) | const |
Returns a pointer to the KIconEffect object used by the icon loader.
- Returns
- the KIconEffect.
- Deprecated
- since 6.5, use the static KIconEffect API
Definition at line 1435 of file kiconloader.cpp.
◆ iconLoaderSettingsChanged
|
signal |
Emitted by newIconLoader once the new settings have been loaded.
◆ iconPath() [1/2]
QString KIconLoader::iconPath | ( | const QString & | name, |
int | group_or_size, | ||
bool | canReturnNull, | ||
qreal | scale ) const |
Returns the path of an icon.
- Parameters
-
name The name of the icon, without extension. If an absolute path is supplied for this parameter, iconPath will return it directly. group_or_size If positive, search icons whose size is specified by the icon group group_or_size
. If negative, search icons whose size is -group_or_size
. See KIconLoader::Group and KIconLoader::StdSizescanReturnNull Can return a null string? If not, a path to the "unknown" icon will be returned. scale The scale of the icon group.
- Returns
- the path of an icon, can be null or the "unknown" icon when not found, depending on
canReturnNull
.
- Since
- 5.48
Definition at line 941 of file kiconloader.cpp.
◆ iconPath() [2/2]
QString KIconLoader::iconPath | ( | const QString & | name, |
int | group_or_size, | ||
bool | canReturnNull = false ) const |
Returns the path of an icon.
- Parameters
-
name The name of the icon, without extension. If an absolute path is supplied for this parameter, iconPath will return it directly. group_or_size If positive, search icons whose size is specified by the icon group group_or_size
. If negative, search icons whose size is -group_or_size
. See KIconLoader::Group and KIconLoader::StdSizescanReturnNull Can return a null string? If not, a path to the "unknown" icon will be returned.
- Returns
- the path of an icon, can be null or the "unknown" icon when not found, depending on
canReturnNull
.
Definition at line 936 of file kiconloader.cpp.
◆ loadAnimated()
QStringList KIconLoader::loadAnimated | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 ) const |
Loads an animated icon as a series of still frames.
If you want to load a .mng animation as QMovie instead, please use loadMovie() instead.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.
- Returns
- A QStringList containing the absolute path of all the frames making up the animation.
- Deprecated
- since 6.5, use QMovie API
Definition at line 1282 of file kiconloader.cpp.
◆ loadIcon()
QPixmap KIconLoader::loadIcon | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0, | ||
int | state = KIconLoader::DefaultState, | ||
const QStringList & | overlays = QStringList(), | ||
QString * | path_store = nullptr, | ||
bool | canReturnNull = false ) const |
Loads an icon.
It will try very hard to find an icon which is suitable. If no exact match is found, a close match is searched. If neither an exact nor a close match is found, a null pixmap or the "unknown" pixmap is returned, depending on the value of the canReturnNull
parameter.
- Parameters
-
name The name of the icon, without extension. group The icon group. This will specify the size of and effects to be applied to the icon. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.overlays a list of emblem icons to overlay, by name
- See also
- drawOverlays
- Parameters
-
path_store If not null, the path of the icon is stored here, if the icon was found. If the icon was not found path_store
is unaltered even if the "unknown" pixmap was returned.canReturnNull Can return a null pixmap? If false, the "unknown" pixmap is returned when no appropriate icon has been found. Note: a null pixmap can still be returned in the event of invalid parameters, such as empty names, negative sizes, and etc.
- Returns
- the QPixmap. Can be null when not found, depending on
canReturnNull
.
Definition at line 1024 of file kiconloader.cpp.
◆ loadMimeTypeIcon()
QPixmap KIconLoader::loadMimeTypeIcon | ( | const QString & | iconName, |
KIconLoader::Group | group, | ||
int | size = 0, | ||
int | state = KIconLoader::DefaultState, | ||
const QStringList & | overlays = QStringList(), | ||
QString * | path_store = nullptr ) const |
Loads an icon for a mimetype.
This is basically like loadIcon except that extra desktop themes are loaded if necessary.
Consider using QIcon::fromTheme() with a fallback to "application-octet-stream" instead.
- Parameters
-
iconName The name of the icon, without extension, usually from KMimeType. group The icon group. This will specify the size of and effects to be applied to the icon. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.path_store If not null, the path of the icon is stored here. overlays a list of emblem icons to overlay, by name
- See also
- drawOverlays
- Returns
- the QPixmap. Can not be null, the "unknown" pixmap is returned when no appropriate icon has been found.
Definition at line 1001 of file kiconloader.cpp.
◆ loadMovie()
QMovie * KIconLoader::loadMovie | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0, | ||
QObject * | parent = nullptr ) const |
Loads an animated icon.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.parent The parent object of the returned QMovie.
- Returns
- A QMovie object. Can be null if not found or not valid. Ownership is passed to the caller.
- Deprecated
- since 6.5, use QMovie API
Definition at line 1213 of file kiconloader.cpp.
◆ loadScaledIcon() [1/2]
QPixmap KIconLoader::loadScaledIcon | ( | const QString & | name, |
KIconLoader::Group | group, | ||
qreal | scale, | ||
const QSize & | size = {}, | ||
int | state = KIconLoader::DefaultState, | ||
const QStringList & | overlays = QStringList(), | ||
QString * | path_store = nullptr, | ||
bool | canReturnNull = false ) const |
Loads an icon.
It will try very hard to find an icon which is suitable. If no exact match is found, a close match is searched. If neither an exact nor a close match is found, a null pixmap or the "unknown" pixmap is returned, depending on the value of the canReturnNull
parameter.
- Parameters
-
name The name of the icon, without extension. group The icon group. This will specify the size of and effects to be applied to the icon. scale The scale of the icon group to use. If no icon exists in the scaled group, a 1x icon with its size multiplied by the scale will be loaded instead. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes. The icon will be fit intosize
without changing the aspect ratio, which particularly matters for non-square icons.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.overlays a list of emblem icons to overlay, by name
- See also
- drawOverlays
- Parameters
-
path_store If not null, the path of the icon is stored here, if the icon was found. If the icon was not found path_store
is unaltered even if the "unknown" pixmap was returned.canReturnNull Can return a null pixmap? If false, the "unknown" pixmap is returned when no appropriate icon has been found. Note: a null pixmap can still be returned in the event of invalid parameters, such as empty names, negative sizes, and etc.
- Returns
- the QPixmap. Can be null when not found, depending on
canReturnNull
.
- Since
- 5.81
Definition at line 1047 of file kiconloader.cpp.
◆ loadScaledIcon() [2/2]
QPixmap KIconLoader::loadScaledIcon | ( | const QString & | name, |
KIconLoader::Group | group, | ||
qreal | scale, | ||
int | size = 0, | ||
int | state = KIconLoader::DefaultState, | ||
const QStringList & | overlays = QStringList(), | ||
QString * | path_store = nullptr, | ||
bool | canReturnNull = false ) const |
Loads an icon.
It will try very hard to find an icon which is suitable. If no exact match is found, a close match is searched. If neither an exact nor a close match is found, a null pixmap or the "unknown" pixmap is returned, depending on the value of the canReturnNull
parameter.
- Parameters
-
name The name of the icon, without extension. group The icon group. This will specify the size of and effects to be applied to the icon. scale The scale of the icon group to use. If no icon exists in the scaled group, a 1x icon with its size multiplied by the scale will be loaded instead. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.overlays a list of emblem icons to overlay, by name
- See also
- drawOverlays
- Parameters
-
path_store If not null, the path of the icon is stored here, if the icon was found. If the icon was not found path_store
is unaltered even if the "unknown" pixmap was returned.canReturnNull Can return a null pixmap? If false, the "unknown" pixmap is returned when no appropriate icon has been found. Note: a null pixmap can still be returned in the event of invalid parameters, such as empty names, negative sizes, and etc.
- Returns
- the QPixmap. Can be null when not found, depending on
canReturnNull
.
- Since
- 5.48
Definition at line 1035 of file kiconloader.cpp.
◆ moviePath()
QString KIconLoader::moviePath | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 ) const |
Returns the path to an animated icon.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.
- Returns
- the full path to the movie, ready to be passed to QMovie's constructor. Empty string if not found.
- Deprecated
- since 6.5, use QMovie API
Definition at line 1234 of file kiconloader.cpp.
◆ newIconLoader
|
slot |
Re-initialize the global icon loader.
- Todo
- Check deprecation, still used internally.
- Deprecated
- Since 5.0, use emitChange(Group)
Definition at line 1492 of file kiconloader.cpp.
◆ queryIcons() [1/2]
|
nodiscard |
◆ queryIcons() [2/2]
QStringList KIconLoader::queryIcons | ( | int | group_or_size, |
KIconLoader::Context | context = KIconLoader::Any ) const |
Queries all available icons for a specific group, having a specific context.
- Parameters
-
group_or_size If positive, search icons whose size is specified by the icon group group_or_size
. If negative, search icons whose size is -group_or_size
. See KIconLoader::Group and KIconLoader::StdSizescontext The icon context.
- Returns
- a list of all icons
Definition at line 1400 of file kiconloader.cpp.
◆ queryIconsByContext()
QStringList KIconLoader::queryIconsByContext | ( | int | group_or_size, |
KIconLoader::Context | context = KIconLoader::Any ) const |
Queries all available icons for a specific context.
- Parameters
-
group_or_size The icon preferred group or size. If available at this group or size, those icons will be returned, in other case, icons of undefined size will be returned. Positive numbers are groups, negative numbers are negated sizes. See KIconLoader::Group and KIconLoader::StdSizes context The icon context.
- Returns
- A QStringList containing the icon names available for that context
Definition at line 1367 of file kiconloader.cpp.
◆ queryIconsByDir()
QStringList KIconLoader::queryIconsByDir | ( | const QString & | iconsDir | ) | const |
Returns a list of all icons (*.png or *.xpm extension) in the given directory.
- Parameters
-
iconsDir the directory to search in
- Returns
- A QStringList containing the icon paths
Definition at line 1355 of file kiconloader.cpp.
◆ reconfigure()
void KIconLoader::reconfigure | ( | const QString & | appname, |
const QStringList & | extraSearchPaths = QStringList() ) |
Reconfigure the icon loader, for instance to change the associated app name or extra search paths.
This also clears the in-memory pixmap cache (even if the appname didn't change, which is useful for unittests)
- Parameters
-
appname the application name (empty for the global iconloader) extraSearchPaths additional search paths, either absolute or relative to GenericDataLocation
Definition at line 397 of file kiconloader.cpp.
◆ resetPalette()
void KIconLoader::resetPalette | ( | ) |
Resets the custom palette used by the KIconLoader to use the QGuiApplication::palette() again (and to follow it in case the application's palette changes)
- Since
- 5.39
Definition at line 1476 of file kiconloader.cpp.
◆ searchPaths()
QStringList KIconLoader::searchPaths | ( | ) | const |
Returns all the search paths for this icon loader, either absolute or relative to GenericDataLocation.
Mostly internal (for KIconDialog).
- Since
- 5.0
Definition at line 471 of file kiconloader.cpp.
◆ setCustomPalette()
void KIconLoader::setCustomPalette | ( | const QPalette & | palette | ) |
Sets the colors for this KIconLoader.
NOTE: if you set a custom palette, if you are using some colors from application's palette, you need to track the application palette changes by yourself. If you no longer wish to use a custom palette, use resetPalette()
- See also
- resetPalette
- Since
- 5.39
Definition at line 1465 of file kiconloader.cpp.
◆ theme()
KIconTheme * KIconLoader::theme | ( | ) | const |
Returns a pointer to the current theme.
Can be used to query available and default sizes for groups.
- Note
- The KIconTheme will change if reconfigure() is called and therefore it's not recommended to store the pointer anywhere.
- Returns
- a pointer to the current theme. 0 if no theme set.
Definition at line 1334 of file kiconloader.cpp.
◆ unknown()
|
static |
Returns the unknown icon.
An icon that is used when no other icon can be found.
- Returns
- the unknown pixmap
Definition at line 1441 of file kiconloader.cpp.
Friends And Related Symbol Documentation
◆ icon() [1/2]
|
Returns a QIcon for the given icon, with additional overlays.
- Since
- 5.0
◆ icon() [2/2]
|
Returns a QIcon with an appropriate KIconEngine to perform loading and rendering.
KIcons thus adhere to KDE style and effect standards.
- Since
- 5.0
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:47:16 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.