KCoreUrlNavigator
#include <KCoreUrlNavigator>
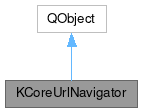
Properties | |
QUrl | currentLocationUrl |
int | historyIndex |
int | historySize |
![]() | |
objectName | |
Public Member Functions | |
KCoreUrlNavigator (const QUrl &url=QUrl(), QObject *parent=nullptr) | |
QUrl | currentLocationUrl () const |
Q_SIGNAL void | currentLocationUrlChanged () |
Q_SIGNAL void | currentUrlAboutToChange (const QUrl &newUrl) |
Q_INVOKABLE bool | goBack () |
Q_INVOKABLE bool | goForward () |
Q_INVOKABLE bool | goUp () |
Q_SIGNAL void | historyChanged () |
int | historyIndex () const |
Q_SIGNAL void | historyIndexChanged () |
int | historySize () const |
Q_SIGNAL void | historySizeChanged () |
Q_INVOKABLE QVariant | locationState (int historyIndex=-1) const |
Q_INVOKABLE QUrl | locationUrl (int historyIndex=-1) const |
Q_INVOKABLE void | saveLocationState (const QVariant &state) |
void | setCurrentLocationUrl (const QUrl &url) |
Q_SIGNAL void | urlSelectionRequested (const QUrl &url) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Object that helps with keeping track of URLs in file-manager like interfaces.
- Since
- 5.93
Definition at line 36 of file kcoreurlnavigator.h.
Property Documentation
◆ currentLocationUrl
|
readwrite |
Definition at line 44 of file kcoreurlnavigator.h.
◆ historyIndex
|
read |
The history index of the current location, where 0 <= history index < KCoreUrlNavigator::historySize().
0 is the most recent history entry.
Definition at line 84 of file kcoreurlnavigator.h.
◆ historySize
|
read |
The amount of locations in the history.
The data for each location can be retrieved by KCoreUrlNavigator::locationUrl() and KCoreUrlNavigator::locationState().
Definition at line 63 of file kcoreurlnavigator.h.
Constructor & Destructor Documentation
◆ KCoreUrlNavigator()
Definition at line 89 of file kcoreurlnavigator.cpp.
◆ ~KCoreUrlNavigator()
|
override |
Definition at line 96 of file kcoreurlnavigator.cpp.
Member Function Documentation
◆ currentLocationUrl()
QUrl KCoreUrlNavigator::currentLocationUrl | ( | ) | const |
Definition at line 164 of file kcoreurlnavigator.cpp.
◆ currentUrlAboutToChange()
Is emitted, before the location URL is going to be changed to newUrl.
The signal KCoreUrlNavigator::urlChanged() will be emitted after the change has been done. Connecting to this signal is useful to save the state of a view with KCoreUrlNavigator::saveLocationState().
◆ goBack()
bool KCoreUrlNavigator::goBack | ( | ) |
Goes back one step in the URL history.
The signals KCoreUrlNavigator::urlAboutToBeChanged(), KCoreUrlNavigator::urlChanged() and KCoreUrlNavigator::historyChanged() are emitted if true is returned. False is returned if the beginning of the history has already been reached and hence going back was not possible. The history index (see KCoreUrlNavigator::historyIndex()) is increased by one if the operation was successful.
Definition at line 117 of file kcoreurlnavigator.cpp.
◆ goForward()
bool KCoreUrlNavigator::goForward | ( | ) |
Goes forward one step in the URL history.
The signals KCoreUrlNavigator::urlAboutToBeChanged(), KCoreUrlNavigator::urlChanged() and KCoreUrlNavigator::historyChanged() are emitted if true is returned. False is returned if the end of the history has already been reached and hence going forward was not possible. The history index (see KCoreUrlNavigator::historyIndex()) is decreased by one if the operation was successful.
Definition at line 135 of file kcoreurlnavigator.cpp.
◆ goUp()
bool KCoreUrlNavigator::goUp | ( | ) |
Goes up one step of the URL path and remembers the old path in the history.
The signals KCoreUrlNavigator::urlAboutToBeChanged(), KCoreUrlNavigator::urlChanged() and KCoreUrlNavigator::historyChanged() are emitted if true is returned. False is returned if going up was not possible as the root has been reached.
Definition at line 152 of file kcoreurlnavigator.cpp.
◆ historyChanged()
Q_SIGNAL void KCoreUrlNavigator::historyChanged | ( | ) |
Is emitted, if the history has been changed.
Usually the history is changed if a new URL has been selected.
◆ historyIndex()
int KCoreUrlNavigator::historyIndex | ( | ) | const |
Definition at line 257 of file kcoreurlnavigator.cpp.
◆ historySize()
int KCoreUrlNavigator::historySize | ( | ) | const |
Definition at line 252 of file kcoreurlnavigator.cpp.
◆ locationState()
QVariant KCoreUrlNavigator::locationState | ( | int | historyIndex = -1 | ) | const |
- Returns
- Location state given by historyIndex. If historyIndex is smaller than 0, the state of the current location is returned.
Definition at line 111 of file kcoreurlnavigator.cpp.
◆ locationUrl()
QUrl KCoreUrlNavigator::locationUrl | ( | int | historyIndex = -1 | ) | const |
- Returns
- URL of the location given by the historyIndex. If historyIndex is smaller than 0, the URL of the current location is returned.
Definition at line 100 of file kcoreurlnavigator.cpp.
◆ saveLocationState()
void KCoreUrlNavigator::saveLocationState | ( | const QVariant & | state | ) |
Saves the location state described by state for the current location.
It is recommended that at least the scroll position of a view is remembered and restored when traversing through the history. Saving the location state should be done when the signal KCoreUrlNavigator::urlAboutToBeChanged() has been emitted. Restoring the location state (see KCoreUrlNavigator::locationState()) should be done when the signal KCoreUrlNavigator::urlChanged() has been emitted.
Example:
Definition at line 106 of file kcoreurlnavigator.cpp.
◆ setCurrentLocationUrl()
void KCoreUrlNavigator::setCurrentLocationUrl | ( | const QUrl & | url | ) |
Definition at line 169 of file kcoreurlnavigator.cpp.
◆ urlSelectionRequested()
When the URL is changed and the new URL (e.g. /home/user1/) is a parent of the previous URL (e.g. /home/user1/data/stuff), then this signal is emitted and url
is set to the child directory of the new URL which is an ancestor of the old URL (in the example paths this would be /home/user1/data/).
This signal allows file managers to pre-select the directory that the user is navigating up from.
- Since
- 5.95
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:11:14 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.