KFilePreviewGenerator
#include <KFilePreviewGenerator>
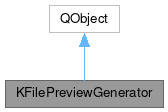
Public Slots | |
void | cancelPreviews () |
void | updateIcons () |
Public Member Functions | |
KFilePreviewGenerator (KAbstractViewAdapter *parent, QAbstractProxyModel *model) | |
KFilePreviewGenerator (QAbstractItemView *parent) | |
QStringList | enabledPlugins () const |
bool | isPreviewShown () const |
void | setEnabledPlugins (const QStringList &list) |
void | setPreviewShown (bool show) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Generates previews for files of an item view.
Per default a preview is generated for each item. Additionally the clipboard is checked for cut items. The icon state for cut items gets dimmed automatically.
The following strategy is used when creating previews:
- The previews for currently visible items are created before the previews for invisible items.
- If the user changes the visible area by using the scrollbars, all pending previews get paused. As soon as the user stays on the same position for a short delay, the previews are resumed. Also in this case the previews for the visible items are generated first.
Definition at line 42 of file kfilepreviewgenerator.h.
Constructor & Destructor Documentation
◆ KFilePreviewGenerator() [1/2]
KFilePreviewGenerator::KFilePreviewGenerator | ( | QAbstractItemView * | parent | ) |
- Parameters
-
parent Item view containing the file items where previews should be generated. It is mandatory that the item view specifies an icon size by QAbstractItemView::setIconSize() and that the model of the view (or the source model of the proxy model) is an instance of KDirModel. Otherwise no previews will be generated.
Definition at line 1148 of file kfilepreviewgenerator.cpp.
◆ KFilePreviewGenerator() [2/2]
KFilePreviewGenerator::KFilePreviewGenerator | ( | KAbstractViewAdapter * | parent, |
QAbstractProxyModel * | model ) |
Definition at line 1155 of file kfilepreviewgenerator.cpp.
Member Function Documentation
◆ cancelPreviews
|
slot |
Cancels all pending previews.
Definition at line 1202 of file kfilepreviewgenerator.cpp.
◆ enabledPlugins()
QStringList KFilePreviewGenerator::enabledPlugins | ( | ) | const |
Returns the list of enabled thumbnail plugins.
- See also
- setEnabledPlugins
Definition at line 1215 of file kfilepreviewgenerator.cpp.
◆ isPreviewShown()
bool KFilePreviewGenerator::isPreviewShown | ( | ) | const |
Definition at line 1183 of file kfilepreviewgenerator.cpp.
◆ setEnabledPlugins()
void KFilePreviewGenerator::setEnabledPlugins | ( | const QStringList & | list | ) |
Sets the list of enabled thumbnail plugins.
Per default all plugins enabled in the KConfigGroup "PreviewSettings" are used.
Note that this method doesn't cause already generated previews to be regenerated.
For a list of available plugins, call KIO::PreviewJob::availableThumbnailerPlugins().
- See also
- enabledPlugins
Definition at line 1210 of file kfilepreviewgenerator.cpp.
◆ setPreviewShown()
void KFilePreviewGenerator::setPreviewShown | ( | bool | show | ) |
If show is set to true, a preview is generated for each item.
If show is false, the MIME type icon of the item is shown instead. Per default showing the preview is turned on. Note that it is mandatory that the item view specifies an icon size by QAbstractItemView::setIconSize(), otherwise KFilePreviewGenerator::isPreviewShown() will always return false.
Definition at line 1163 of file kfilepreviewgenerator.cpp.
◆ updateIcons
|
slot |
Updates the icons for all items.
Usually it is only necessary to invoke this method when the icon size of the abstract item view has been changed by QAbstractItemView::setIconSize(). Note that this method should also be invoked if previews have been turned off, as the icons for cut items must be updated when the icon size has changed.
Definition at line 1188 of file kfilepreviewgenerator.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:16:28 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.