KIO::WidgetsAskUserActionHandler
#include <KIO/WidgetsAskUserActionHandler>
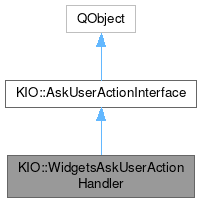
Public Member Functions | |
WidgetsAskUserActionHandler (QObject *parent=nullptr) | |
void | askIgnoreSslErrors (const QVariantMap &sslErrorData, QWidget *parent) override |
void | askUserDelete (const QList< QUrl > &urls, DeletionType deletionType, ConfirmationType confirmationType, QWidget *parent=nullptr) override |
void | askUserRename (KJob *job, const QString &title, const QUrl &src, const QUrl &dest, KIO::RenameDialog_Options options, KIO::filesize_t sizeSrc=KIO::filesize_t(-1), KIO::filesize_t sizeDest=KIO::filesize_t(-1), const QDateTime &ctimeSrc={}, const QDateTime &ctimeDest={}, const QDateTime &mtimeSrc={}, const QDateTime &mtimeDest={}) override |
void | askUserSkip (KJob *job, KIO::SkipDialog_Options options, const QString &error_text) override |
void | requestUserMessageBox (MessageDialogType type, const QString &text, const QString &title, const QString &primaryActionText, const QString &secondaryActionText, const QString &primaryActionIconName={}, const QString &secondaryActionIconName={}, const QString &dontAskAgainName={}, const QString &details={}, QWidget *parent=nullptr) override |
void | setWindow (QWidget *window) |
![]() | |
~AskUserActionInterface () override | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
enum | ConfirmationType { DefaultConfirmation , ForceConfirmation } |
enum | DeletionType { Delete , Trash , EmptyTrash , DeleteInsteadOfTrash } |
enum | MessageDialogType { QuestionTwoActions = 1 , QuestionTwoActionsCancel = 2 , WarningTwoActions = 3 , WarningTwoActionsCancel = 4 , WarningContinueCancel = 5 , Information = 7 , Error = 9 } |
![]() | |
objectName | |
![]() | |
void | askIgnoreSslErrorsResult (int result) |
void | askUserDeleteResult (bool allowDelete, const QList< QUrl > &urls, KIO::AskUserActionInterface::DeletionType deletionType, QWidget *parent) |
void | askUserRenameResult (KIO::RenameDialog_Result result, const QUrl &newUrl, KJob *parentJob) |
void | askUserSkipResult (KIO::SkipDialog_Result result, KJob *parentJob) |
void | messageBoxResult (int result) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
AskUserActionInterface (QObject *parent=nullptr) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This implements KIO::AskUserActionInterface.
- Since
- 5.78
- Note
- This header wasn't installed until 5.98
Definition at line 36 of file widgetsaskuseractionhandler.h.
Constructor & Destructor Documentation
◆ WidgetsAskUserActionHandler()
|
explicit |
Definition at line 142 of file widgetsaskuseractionhandler.cpp.
◆ ~WidgetsAskUserActionHandler()
|
override |
Definition at line 148 of file widgetsaskuseractionhandler.cpp.
Member Function Documentation
◆ askIgnoreSslErrors()
|
overridevirtual |
Implements KIO::AskUserActionInterface.
Definition at line 478 of file widgetsaskuseractionhandler.cpp.
◆ askUserDelete()
|
overridevirtual |
Ask for confirmation before moving urls
(files/directories) to the Trash, emptying the Trash, or directly deleting files (i.e.
without moving to Trash).
Note that this method is not called automatically by KIO jobs. It's the application's responsibility to ask the user for confirmation before calling KIO::del() or KIO::trash().
You need to connect to the askUserDeleteResult signal to get the dialog's result (exit code).
- Parameters
-
urls the urls about to be moved to the Trash or deleted directly deletionType the type of deletion (Delete for real deletion, Trash otherwise), see the DeletionType enum confirmationType The type of deletion confirmation, see the ConfirmationType enum. Normally set to DefaultConfirmation parent the parent widget of the message box
Implements KIO::AskUserActionInterface.
Definition at line 300 of file widgetsaskuseractionhandler.cpp.
◆ askUserRename()
|
overridevirtual |
Constructs a modal, parent-less "rename" dialog, to prompt the user for a decision in case of conflicts, while copying/moving files.
The dialog is shown using open(), rather than exec() (the latter creates a nested eventloop which could lead to crashes). You will need to connect to the askUserRenameResult() signal to get the dialog's result (exit code). The exit code is one of KIO::RenameDialog_Result.
- See also
- KIO::RenameDialog_Result enum.
- Parameters
-
job the job that called this method title the title for the dialog box src the URL of the file/dir being copied/moved dest the URL of the destination file/dir, i.e. the one that already exists options parameters for the dialog (which buttons to show... etc), OR'ed values from the KIO::RenameDialog_Options enum sizeSrc size of the source file sizeDest size of the destination file ctimeSrc creation time of the source file ctimeDest creation time of the destination file mtimeSrc modification time of the source file mtimeDest modification time of the destination file
Implements KIO::AskUserActionInterface.
Definition at line 152 of file widgetsaskuseractionhandler.cpp.
◆ askUserSkip()
|
overridevirtual |
You need to connect to the askUserSkipResult signal to get the dialog's result.
- Parameters
-
job the job that called this method options parameters for the dialog (which buttons to show... etc), OR'ed values from the KIO::SkipDialog_Options enum error_text the error text to show to the user (usually the string returned by KJob::errorText())
Implements KIO::AskUserActionInterface.
Definition at line 181 of file widgetsaskuseractionhandler.cpp.
◆ requestUserMessageBox()
|
overridevirtual |
This function allows for the delegation of user prompts from the KIO worker.
- Parameters
-
type the desired type of message box, see the MessageDialogType enum text the message to show to the user title the title of the message dialog box primaryActionText the text for the primary action secondatyActionText the text for the secondary action primaryActionIconName the icon to show on the primary action secondatyActionIconName the icon to show on the secondary action dontAskAgainName the config key name used to store the result from 'Do not ask again' checkbox details more details about the message shown to the user parent the parent widget of the dialog
Implements KIO::AskUserActionInterface.
Definition at line 365 of file widgetsaskuseractionhandler.cpp.
◆ setWindow()
void KIO::WidgetsAskUserActionHandler::setWindow | ( | QWidget * | window | ) |
Definition at line 473 of file widgetsaskuseractionhandler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 11:52:09 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.