Engine
#include <quickengine.h>
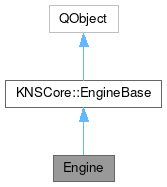
Public Types | |
enum class | BusyOperation { Initializing = 1 , LoadingData , LoadingPreview , InstallingEntry } |
typedef QFlags< BusyOperation > | BusyState |
enum | EntryEvent { UnknownEvent = KNSCore::Entry::UnknownEvent , StatusChangedEvent = KNSCore::Entry::StatusChangedEvent , AdoptedEvent = KNSCore::Entry::AdoptedEvent , DetailsLoadedEvent = KNSCore::Entry::DetailsLoadedEvent } |
![]() | |
enum class | ContentWarningType { Static , Executables } |
![]() | |
typedef | QObjectList |
Properties | |
QString | busyMessage |
BusyState | busyState |
CategoriesModel * | categories |
QStringList | categoriesFilter |
QString | configFile |
KNSCore::Provider::Filter | filter |
KNSCore::Filter | filter2 |
bool | hasAdoptionCommand |
bool | isLoading |
bool | isValid |
QString | name |
bool | needsLazyLoadSpinner |
SearchPresetModel * | searchPresetModel |
QString | searchTerm |
KNSCore::Provider::SortMode | sortOrder |
KNSCore::SortMode | sortOrder2 |
![]() | |
ContentWarningType | contentWarningType |
QStringList | providerIDs |
bool | uploadEnabled |
QString | useLabel |
![]() | |
objectName | |
Signals | |
void | entryEvent (const KNSCore::Entry &entry, KNSCore::Entry::EntryEvent event) |
void | entryPreviewLoaded (const KNSCore::Entry &, KNSCore::Entry::PreviewType) |
void | errorCode (KNSCore::ErrorCode::ErrorCode errorCode, const QString &message, const QVariant &metadata) |
void | signalEntriesLoaded (const KNSCore::Entry::List &entries) |
void | signalEntryEvent (const KNSCore::Entry &entry, KNSCore::Entry::EntryEvent event) |
void | signalResetView () |
![]() | |
void | loadingProvider () |
void | providerAdded (KNSCore::ProviderCore *provider) |
void | providersChanged () |
void | signalCategoriesMetadataLoaded (const QList< KNSCore::CategoryMetadata > &categories) |
void | signalCategoriesMetadataLoded (const QList< Provider::CategoryMetadata > &categories) |
void | signalErrorCode (KNSCore::ErrorCode::ErrorCode errorCode, const QString &message, const QVariant &metadata) |
void | signalMessage (const QString &message) |
void | signalProvidersLoaded () |
void | signalSearchPresetsLoaded (const QList< KNSCore::SearchPreset > &presets) |
void | signalSearchPresetsLoaded (const QList< Provider::SearchPreset > &presets) |
Public Member Functions | |
Engine (QObject *parent=nullptr) | |
Q_INVOKABLE KNSCore::Entry | __createEntry (const QString &providerId, const QString &entryId) |
Q_INVOKABLE void | adoptEntry (const KNSCore::Entry &entry) |
QString | busyMessage () const |
Engine::BusyState | busyState () const |
Q_SIGNAL void | busyStateChanged () |
CategoriesModel * | categories () const |
Q_SIGNAL void | categoriesChanged () |
QStringList | categoriesFilter () const |
Q_SIGNAL void | categoriesFilterChanged () |
QString | configFile () const |
Q_SIGNAL void | configFileChanged () |
KNSCore::Provider::Filter | filter () const |
KNSCore::Filter | filter2 () const |
Q_SIGNAL void | filterChanged () |
Q_INVOKABLE void | install (const KNSCore::Entry &entry, int linkId=1) |
Q_INVOKABLE void | installLatest (const KNSCore::Entry &entry) |
Q_INVOKABLE void | installLinkId (const KNSCore::Entry &entry, quint8 linkId) |
bool | isLoading () const |
bool | isValid () |
void | loadPreview (const KNSCore::Entry &entry, KNSCore::Entry::PreviewType type) |
void | reloadEntries () |
void | requestMoreData () |
Q_INVOKABLE void | resetCategoriesFilter () |
Q_INVOKABLE void | resetSearchTerm () |
Q_INVOKABLE void | restoreSearch () |
Q_INVOKABLE void | revalidateCacheEntries () |
SearchPresetModel * | searchPresetModel () const |
Q_SIGNAL void | searchPresetModelChanged () |
QString | searchTerm () const |
Q_SIGNAL void | searchTermChanged () |
void | setBusyState (Engine::BusyState state) |
void | setCategoriesFilter (const QStringList &newCategoriesFilter) |
void | setConfigFile (const QString &newFile) |
void | setFilter (KNSCore::Provider::Filter filter) |
void | setFilter2 (KNSCore::Filter filter) |
void | setSearchTerm (const QString &newSearchTerm) |
void | setSortOrder (KNSCore::Provider::SortMode newSortOrder) |
void | setSortOrder2 (KNSCore::SortMode newSortOrder) |
KNSCore::Provider::SortMode | sortOrder () const |
KNSCore::SortMode | sortOrder2 () const |
Q_SIGNAL void | sortOrderChanged () |
Q_INVOKABLE void | storeSearch () |
Q_INVOKABLE void | uninstall (const KNSCore::Entry &entry) |
Q_INVOKABLE void | updateEntryContents (const KNSCore::Entry &entry) |
![]() | |
EngineBase (QObject *parent=nullptr) | |
void | addDownloadTagFilter (const QString &filter) |
void | addTagFilter (const QString &filter) |
QList< Attica::Provider * > | atticaProviders () const |
void | becomeFan (const Entry &entry) |
QSharedPointer< Cache > | cache () const |
QStringList | categories () const |
QList< Provider::CategoryMetadata > | categoriesMetadata () |
QList< CategoryMetadata > | categoriesMetadata2 () |
ContentWarningType | contentWarningType () const |
Q_SIGNAL void | contentWarningTypeChanged () |
QSharedPointer< Provider > | defaultProvider () const |
QStringList | downloadTagFilter () const |
bool | hasAdoptionCommand () const |
QString | name () const |
QSharedPointer< Provider > | provider (const QString &providerId) const |
QStringList | providerIDs () const |
ResultsStream * | search (const KNSCore::Provider::SearchRequest &request) |
ResultsStream * | search (const KNSCore::SearchRequest &request) |
QList< Provider::SearchPreset > | searchPresets () |
QList< SearchPreset > | searchPresets2 () |
void | setDownloadTagFilter (const QStringList &filter) |
void | setTagFilter (const QStringList &filter) |
QStringList | tagFilter () const |
bool | uploadEnabled () const |
Q_SIGNAL void | uploadEnabledChanged () |
QString | useLabel () const |
Q_SIGNAL void | useLabelChanged () |
bool | userCanBecomeFan (const Entry &entry) |
bool | userCanVote (const Entry &entry) |
void | vote (const Entry &entry, uint rating) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
static QStringList | availableConfigFiles () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | addProvider (QSharedPointer< KNSCore::Provider > provider) |
Installation * | installation () const |
QList< QSharedPointer< Provider > > | providers () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
std::unique_ptr< EngineBasePrivate > | d |
Detailed Description
KNSCore::EngineBase for interfacing with QML.
- See also
- ItemsModel
Definition at line 28 of file quickengine.h.
Member Typedef Documentation
◆ BusyState
QFlags< BusyOperation > Engine::BusyState |
Definition at line 65 of file quickengine.h.
Member Enumeration Documentation
◆ BusyOperation
|
strong |
Definition at line 59 of file quickengine.h.
◆ EntryEvent
enum Engine::EntryEvent |
Definition at line 68 of file quickengine.h.
Property Documentation
◆ busyMessage
|
read |
Definition at line 53 of file quickengine.h.
◆ busyState
|
readwrite |
Current state of the engine, the state con contain multiple operations an empty BusyState represents the idle status.
- Since
- 5.74
Definition at line 52 of file quickengine.h.
◆ categories
|
read |
Definition at line 38 of file quickengine.h.
◆ categoriesFilter
|
readwrite |
Definition at line 39 of file quickengine.h.
◆ configFile
|
readwrite |
Definition at line 31 of file quickengine.h.
◆ filter
|
readwrite |
Definition at line 40 of file quickengine.h.
◆ filter2
|
readwrite |
Definition at line 41 of file quickengine.h.
◆ hasAdoptionCommand
|
read |
Definition at line 34 of file quickengine.h.
◆ isLoading
|
read |
Definition at line 32 of file quickengine.h.
◆ isValid
|
read |
Definition at line 36 of file quickengine.h.
◆ name
|
read |
Definition at line 35 of file quickengine.h.
◆ needsLazyLoadSpinner
|
read |
Definition at line 33 of file quickengine.h.
◆ searchPresetModel
|
read |
Definition at line 45 of file quickengine.h.
◆ searchTerm
|
readwrite |
Definition at line 44 of file quickengine.h.
◆ sortOrder
|
readwrite |
Definition at line 42 of file quickengine.h.
◆ sortOrder2
|
readwrite |
Definition at line 43 of file quickengine.h.
Constructor & Destructor Documentation
◆ Engine()
|
explicit |
Definition at line 53 of file quickengine.cpp.
Member Function Documentation
◆ __createEntry()
|
inline |
Definition at line 151 of file quickengine.h.
◆ adoptEntry()
void Engine::adoptEntry | ( | const KNSCore::Entry & | entry | ) |
Adopt an entry using the adoption command.
This will also take care of displaying error messages
- Parameters
-
entry Entry that should be adopted
- See also
- signalErrorCode
- signalEntryEvent
- Since
- 5.77
Definition at line 499 of file quickengine.cpp.
◆ busyMessage()
QString Engine::busyMessage | ( | ) | const |
Definition at line 199 of file quickengine.cpp.
◆ busyState()
Engine::BusyState Engine::busyState | ( | ) | const |
Definition at line 195 of file quickengine.cpp.
◆ busyStateChanged()
Q_SIGNAL void Engine::busyStateChanged | ( | ) |
Signal gets emitted when the busy state changes.
- Since
- 5.74
◆ categories()
CategoriesModel * Engine::categories | ( | ) | const |
Definition at line 233 of file quickengine.cpp.
◆ categoriesFilter()
QStringList Engine::categoriesFilter | ( | ) | const |
Definition at line 238 of file quickengine.cpp.
◆ configFile()
QString Engine::configFile | ( | ) | const |
Definition at line 204 of file quickengine.cpp.
◆ entryEvent
|
signal |
This is fired for events related directly to a single Entry instance The intermediate states Updating and Installing are not forwarded.
In case you need those you have to listen to the signals of the KNSCore::Engine instance of the engine property.
As an example, if you need to know when the status of an entry changes, you might write:
nb: The above example is also how one would port a handler for the old changedEntries signal
- See also
- Entry::EntryEvent for details on which specific event is being notified
◆ errorCode
|
signal |
Fires in the case of any critical or serious errors, such as network or API problems.
This forwards the signal from KNSCore::Engine::signalErrorCode, but with QML friendly enumerations.
- Parameters
-
errorCode Represents the specific type of error which has occurred message A human-readable message which can be shown to the end user metadata Any additional data which might be helpful to further work out the details of the error (see KNSCore::Entry::ErrorCode for the metadata details)
- See also
- KNSCore::Engine::signalErrorCode
- Since
- 5.84
◆ filter()
KNSCore::Provider::Filter Engine::filter | ( | ) | const |
- Deprecated
- since 6.9 Use filter2
Definition at line 258 of file quickengine.cpp.
◆ filter2()
|
nodiscard |
Definition at line 295 of file quickengine.cpp.
◆ install()
void Engine::install | ( | const KNSCore::Entry & | entry, |
int | linkId = 1 ) |
Installs an entry's payload file.
This includes verification, if necessary, as well as decompression and other steps according to the application's *.knsrc file.
- Parameters
-
entry Entry to be installed
- See also
- signalInstallationFinished
- signalInstallationFailed
- Deprecated
- since 6.9, use installLatest or installLinkId instead
Definition at line 505 of file quickengine.cpp.
◆ installLatest()
void Engine::installLatest | ( | const KNSCore::Entry & | entry | ) |
Performs an install of the latest version on the given entry
.
The latest version is determined using heuristics. If you want tight control over which offering gets installed you need to use installLinkId and manually figure out the id.
- Since
- 6.9
Definition at line 525 of file quickengine.cpp.
◆ installLinkId()
void Engine::installLinkId | ( | const KNSCore::Entry & | entry, |
quint8 | linkId ) |
Performs an install on the given entry
.
- Parameters
-
linkId specifies which of the assets we want to see installed.
- Since
- 6.9
Definition at line 516 of file quickengine.cpp.
◆ isLoading()
|
inline |
Whether or not the engine is performing its initial loading operations.
- Since
- 5.65
Definition at line 94 of file quickengine.h.
◆ loadPreview()
void Engine::loadPreview | ( | const KNSCore::Entry & | entry, |
KNSCore::Entry::PreviewType | type ) |
Definition at line 478 of file quickengine.cpp.
◆ reloadEntries()
void Engine::reloadEntries | ( | ) |
Definition at line 422 of file quickengine.cpp.
◆ requestMoreData()
void Engine::requestMoreData | ( | ) |
Definition at line 545 of file quickengine.cpp.
◆ resetCategoriesFilter()
|
inline |
Definition at line 105 of file quickengine.h.
◆ resetSearchTerm()
|
inline |
Definition at line 141 of file quickengine.h.
◆ restoreSearch()
void Engine::restoreSearch | ( | ) |
Definition at line 595 of file quickengine.cpp.
◆ revalidateCacheEntries()
void Engine::revalidateCacheEntries | ( | ) |
Definition at line 570 of file quickengine.cpp.
◆ searchPresetModel()
SearchPresetModel * Engine::searchPresetModel | ( | ) | const |
Definition at line 395 of file quickengine.cpp.
◆ searchTerm()
QString Engine::searchTerm | ( | ) | const |
Definition at line 371 of file quickengine.cpp.
◆ setBusyState()
void Engine::setBusyState | ( | Engine::BusyState | state | ) |
Definition at line 190 of file quickengine.cpp.
◆ setCategoriesFilter()
void Engine::setCategoriesFilter | ( | const QStringList & | newCategoriesFilter | ) |
Definition at line 243 of file quickengine.cpp.
◆ setConfigFile()
void Engine::setConfigFile | ( | const QString & | newFile | ) |
Definition at line 209 of file quickengine.cpp.
◆ setFilter()
void Engine::setFilter | ( | KNSCore::Provider::Filter | filter | ) |
- Deprecated
- since 6.9 Use setFilter2
Definition at line 277 of file quickengine.cpp.
◆ setFilter2()
void Engine::setFilter2 | ( | KNSCore::Filter | filter | ) |
Definition at line 300 of file quickengine.cpp.
◆ setSearchTerm()
void Engine::setSearchTerm | ( | const QString & | newSearchTerm | ) |
Definition at line 376 of file quickengine.cpp.
◆ setSortOrder()
void Engine::setSortOrder | ( | KNSCore::Provider::SortMode | newSortOrder | ) |
- Deprecated
- since 6.9 Use setSortOrder2
Definition at line 334 of file quickengine.cpp.
◆ setSortOrder2()
void Engine::setSortOrder2 | ( | KNSCore::SortMode | newSortOrder | ) |
Definition at line 357 of file quickengine.cpp.
◆ signalEntryEvent
|
signal |
◆ sortOrder()
KNSCore::Provider::SortMode Engine::sortOrder | ( | ) | const |
- Deprecated
- since 6.9 Use sortOrder2
Definition at line 315 of file quickengine.cpp.
◆ sortOrder2()
|
nodiscard |
Definition at line 352 of file quickengine.cpp.
◆ storeSearch()
void Engine::storeSearch | ( | ) |
Definition at line 611 of file quickengine.cpp.
◆ uninstall()
void Engine::uninstall | ( | const KNSCore::Entry & | entry | ) |
Uninstalls an entry.
It reverses the steps which were performed during the installation.
- Parameters
-
entry The entry to uninstall
Definition at line 534 of file quickengine.cpp.
◆ updateEntryContents()
void Engine::updateEntryContents | ( | const KNSCore::Entry & | entry | ) |
Definition at line 405 of file quickengine.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:02:29 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.