KNSCore::Provider
#include <provider.h>
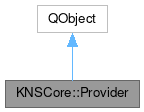
Classes | |
struct | CategoryMetadata |
struct | SearchPreset |
struct | SearchRequest |
Public Types | |
enum | Filter { None , Installed , Updates , ExactEntryId } |
typedef QList< Provider * > | List |
enum | SearchPresetTypes { NoPresetType = 0 , GoBack , Root , Start , Popular , Featured , Recommended , Shelf , Subscription , New , FolderUp , AllEntries } |
enum | SortMode { Newest , Alphabetical , Rating , Downloads } |
![]() | |
typedef | QObjectList |
Properties | |
QString | contactEmail |
QUrl | host |
bool | supportsSsl |
QString | version |
QUrl | website |
![]() | |
objectName | |
Signals | |
void | basicsLoaded () |
void | categoriesMetadataLoded (const QList< KNSCore::Provider::CategoryMetadata > &categories) |
void | commentsLoaded (const QList< std::shared_ptr< KNSCore::Comment > > comments) |
void | entryDetailsLoaded (const KNSCore::Entry &) |
void | loadingFailed (const KNSCore::Provider::SearchRequest &) |
void | loadingFinished (const KNSCore::Provider::SearchRequest &, const KNSCore::Entry::List &) |
void | payloadLinkLoaded (const KNSCore::Entry &) |
void | personLoaded (const std::shared_ptr< KNSCore::Author > author) |
void | providerInitialized (KNSCore::Provider *) |
void | searchPresetsLoaded (const QList< KNSCore::Provider::SearchPreset > &presets) |
void | signalError (const QString &) |
void | signalErrorCode (KNSCore::ErrorCode::ErrorCode errorCode, const QString &message, const QVariant &metadata) |
void | signalInformation (const QString &) |
Public Member Functions | |
Provider () | |
~Provider () override | |
virtual void | becomeFan (const Entry &) |
QString | contactEmail () const |
QStringList | downloadTagFilter () const |
QUrl | host () const |
virtual QUrl | icon () const |
virtual QString | id () const =0 |
virtual bool | isInitialized () const =0 |
virtual void | loadBasics () |
virtual void | loadComments (const KNSCore::Entry &, int, int) |
virtual void | loadEntries (const KNSCore::Provider::SearchRequest &request)=0 |
virtual void | loadEntryDetails (const KNSCore::Entry &) |
virtual void | loadPayloadLink (const Entry &entry, int linkId)=0 |
virtual void | loadPerson (const QString &) |
virtual QString | name () const |
virtual void | setCachedEntries (const KNSCore::Entry::List &cachedEntries)=0 |
void | setContactEmail (const QString &contactEmail) |
void | setDownloadTagFilter (const QStringList &downloadTagFilter) |
void | setHost (const QUrl &host) |
virtual bool | setProviderXML (const QDomElement &xmldata)=0 |
void | setSupportsSsl (bool supportsSsl) |
void | setTagFilter (const QStringList &tagFilter) |
void | setVersion (const QString &version) |
void | setWebsite (const QUrl &website) |
bool | supportsSsl () const |
QStringList | tagFilter () const |
virtual bool | userCanBecomeFan () |
virtual bool | userCanVote () |
QString | version () const |
virtual void | vote (const Entry &, uint) |
QUrl | website () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | setIcon (const QUrl &icon) |
void | setName (const QString &name) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
KNewStuff Base Provider class.
This class provides accessors for the provider object. It should not be used directly by the application. This class is the base class and will be instantiated for static website providers.
Definition at line 40 of file provider.h.
Member Typedef Documentation
◆ List
Definition at line 49 of file provider.h.
Member Enumeration Documentation
◆ Filter
enum KNSCore::Provider::Filter |
Definition at line 59 of file provider.h.
◆ SearchPresetTypes
The SearchPresetTypes enum the preset type enum is a helper to identify the kind of label and icon the search preset should have if none are found.
- Since
- 5.83
Definition at line 116 of file provider.h.
◆ SortMode
enum KNSCore::Provider::SortMode |
Definition at line 51 of file provider.h.
Property Documentation
◆ contactEmail
|
readwrite |
Definition at line 46 of file provider.h.
◆ host
|
readwrite |
Definition at line 45 of file provider.h.
◆ supportsSsl
|
readwrite |
Definition at line 47 of file provider.h.
◆ version
|
readwrite |
Definition at line 43 of file provider.h.
◆ website
|
readwrite |
Definition at line 44 of file provider.h.
Constructor & Destructor Documentation
◆ Provider()
KNSCore::Provider::Provider | ( | ) |
Constructor.
Definition at line 67 of file provider.cpp.
◆ ~Provider()
|
overridedefault |
Destructor.
Member Function Documentation
◆ basicsLoaded
|
signal |
Fired when the provider's basic information has been fetched and updated.
- Since
- 5.85
◆ becomeFan()
|
inlinevirtual |
Definition at line 310 of file provider.h.
◆ commentsLoaded
|
signal |
Fired when new comments have been loaded.
- Parameters
-
comments The list of newly loaded comments, in a depth-first order
- Since
- 5.63
◆ contactEmail()
QString KNSCore::Provider::contactEmail | ( | ) | const |
The general contact email for this provider.
- Returns
- The general contact email for this provider
- Since
- 5.85
Definition at line 160 of file provider.cpp.
◆ downloadTagFilter()
QStringList KNSCore::Provider::downloadTagFilter | ( | ) | const |
The tag filter used for downloads by this provider.
- Returns
- The list of filters
- See also
- Engine::setDownloadTagFilter(QStringList)
- Since
- 5.51
Definition at line 99 of file provider.cpp.
◆ host()
QUrl KNSCore::Provider::host | ( | ) | const |
- Since
- 5.85
Definition at line 146 of file provider.cpp.
◆ icon()
|
virtual |
Retrieves the icon URL for this provider.
- Returns
- icon URL
Definition at line 79 of file provider.cpp.
◆ id()
|
pure virtual |
A unique Id for this provider (the url in most cases)
◆ loadBasics()
|
inlinevirtual |
Request loading of the basic information for this provider.
The engine listens to the basicsLoaded() signal for the result, which is also the signal the respective properties listen to.
This is fired automatically on the first attempt to read one of the properties which contain this basic information, and you will not need to call it as a user of the class (just listen to the properties, which will update when the information has been fetched).
- Note
- Implementation detail: All subclasses should connect to this signal and point it at a slot which does the actual work, if they support fetching this basic information (if the information is set during construction, you will not need to worry about this).
- See also
- version()
- website()
- host();
- contactEmail()
- supportsSsl()
- Since
- 5.85
Definition at line 244 of file provider.h.
◆ loadComments()
|
inlinevirtual |
Request a loading of comments from this provider.
The engine listens to the commentsLoaded() signal for the result
- Note
- Implementation detail: All subclasses should connect to this signal and point it at a slot which does the actual work, if they support comments.
- See also
- commentsLoaded(const QList<shared_ptr<KNSCore::Comment>> comments)
- Since
- 5.63
Definition at line 205 of file provider.h.
◆ loadEntries()
|
pure virtual |
load the given search and return given page
- Parameters
-
sortMode string to select the order in which the results are presented searchstring string to search with page page number to load
Note: the engine connects to loadingFinished() signal to get the result
◆ loadEntryDetails()
|
inlinevirtual |
Definition at line 191 of file provider.h.
◆ loadPerson()
|
inlinevirtual |
Request loading of the details for a specific person with the given username.
The engine listens to the personLoaded() for the result
- Note
- Implementation detail: All subclasses should connect to this signal and point it at a slot which does the actual work, if they support comments.
- Since
- 5.63
Definition at line 218 of file provider.h.
◆ name()
|
virtual |
Retrieves the common name of the provider.
- Returns
- provider name
Definition at line 74 of file provider.cpp.
◆ personLoaded
|
signal |
Fired when the details of a person have been loaded.
- Parameters
-
author The person we've just loaded data for
- Since
- 5.63
◆ searchPresetsLoaded
|
signal |
Fires when the provider has loaded search presets.
These represent interesting searches for the user, such as recommendations.
- Since
- 5.83
◆ setContactEmail()
void KNSCore::Provider::setContactEmail | ( | const QString & | contactEmail | ) |
Sets the general contact email address for this provider.
- Parameters
-
contactEmail The general contact email for this provider
- Since
- 5.85
Definition at line 166 of file provider.cpp.
◆ setDownloadTagFilter()
void KNSCore::Provider::setDownloadTagFilter | ( | const QStringList & | downloadTagFilter | ) |
Set the tag filter used for download items by this provider.
- Parameters
-
downloadTagFilter The new list of filters
- See also
- Engine::setDownloadTagFilter(QStringList)
- Since
- 5.51
Definition at line 94 of file provider.cpp.
◆ setHost()
void KNSCore::Provider::setHost | ( | const QUrl & | host | ) |
- Parameters
-
host The host used for this provider
- Since
- 5.85
Definition at line 152 of file provider.cpp.
◆ setIcon()
|
protected |
Definition at line 193 of file provider.cpp.
◆ setName()
|
protected |
Definition at line 188 of file provider.cpp.
◆ setProviderXML()
|
pure virtual |
Set the provider data xml, to initialize the provider.
The Provider needs to have it's ID set in this function and cannot change it from there on.
◆ setSupportsSsl()
void KNSCore::Provider::setSupportsSsl | ( | bool | supportsSsl | ) |
Set whether or not the provider supports SSL connections.
- Parameters
-
supportsSsl True if the server supports SSL connections, false if not
- Since
- 5.85
Definition at line 180 of file provider.cpp.
◆ setTagFilter()
void KNSCore::Provider::setTagFilter | ( | const QStringList & | tagFilter | ) |
Set the tag filter used for entries by this provider.
- Parameters
-
tagFilter The new list of filters
- See also
- Engine::setTagFilter(QStringList)
- Since
- 5.51
Definition at line 84 of file provider.cpp.
◆ setVersion()
void KNSCore::Provider::setVersion | ( | const QString & | version | ) |
- Since
- 5.85
Definition at line 124 of file provider.cpp.
◆ setWebsite()
void KNSCore::Provider::setWebsite | ( | const QUrl & | website | ) |
- Since
- 5.85
Definition at line 138 of file provider.cpp.
◆ supportsSsl()
bool KNSCore::Provider::supportsSsl | ( | ) | const |
Whether or not the provider supports SSL connections.
- Returns
- True if the server supports SSL connections, false if not
- Since
- 5.85
Definition at line 174 of file provider.cpp.
◆ tagFilter()
QStringList KNSCore::Provider::tagFilter | ( | ) | const |
The tag filter used for downloads by this provider.
- Returns
- The list of filters
- See also
- Engine::setTagFilter(QStringList)
- Since
- 5.51
Definition at line 89 of file provider.cpp.
◆ userCanBecomeFan()
|
inlinevirtual |
Definition at line 306 of file provider.h.
◆ userCanVote()
|
inlinevirtual |
Definition at line 298 of file provider.h.
◆ version()
QString KNSCore::Provider::version | ( | ) | const |
- Since
- 5.85
Definition at line 118 of file provider.cpp.
◆ vote()
|
inlinevirtual |
Definition at line 302 of file provider.h.
◆ website()
QUrl KNSCore::Provider::website | ( | ) | const |
- Since
- 5.85
Definition at line 132 of file provider.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Oct 11 2024 12:12:42 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.