KPtyDevice
#include <kptydevice.h>
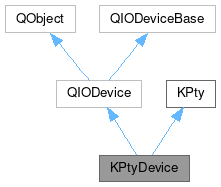
Signals | |
void | readEof () |
Public Member Functions | |
KPtyDevice (QObject *parent=nullptr) | |
~KPtyDevice () override | |
bool | atEnd () const override |
qint64 | bytesAvailable () const override |
qint64 | bytesToWrite () const override |
bool | canReadLine () const override |
void | close () override |
bool | isSequential () const override |
bool | isSuspended () const |
bool | open (int fd, OpenMode mode=ReadWrite|Unbuffered) |
bool | open (OpenMode mode=ReadWrite|Unbuffered) override |
void | setSuspended (bool suspended) |
bool | waitForBytesWritten (int msecs=-1) override |
bool | waitForReadyRead (int msecs=-1) override |
![]() | |
QIODevice (QObject *parent) | |
void | aboutToClose () |
void | bytesWritten (qint64 bytes) |
void | channelBytesWritten (int channel, qint64 bytes) |
void | channelReadyRead (int channel) |
void | commitTransaction () |
int | currentReadChannel () const const |
int | currentWriteChannel () const const |
QString | errorString () const const |
bool | getChar (char *c) |
bool | isOpen () const const |
bool | isReadable () const const |
bool | isTextModeEnabled () const const |
bool | isTransactionStarted () const const |
bool | isWritable () const const |
QIODeviceBase::OpenMode | openMode () const const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
virtual qint64 | pos () const const |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
int | readChannelCount () const const |
void | readChannelFinished () |
qint64 | readLine (char *data, qint64 maxSize) |
QByteArray | readLine (qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | rollbackTransaction () |
virtual bool | seek (qint64 pos) |
void | setCurrentReadChannel (int channel) |
void | setCurrentWriteChannel (int channel) |
void | setTextModeEnabled (bool enabled) |
virtual qint64 | size () const const |
qint64 | skip (qint64 maxSize) |
void | startTransaction () |
void | ungetChar (char c) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &data) |
int | writeChannelCount () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
KPty () | |
KPty (const KPty &)=delete | |
~KPty () | |
void | close () |
void | closeSlave () |
void | login (const char *user=nullptr, const char *remotehost=nullptr) |
void | logout () |
int | masterFd () const |
bool | open () |
bool | open (int fd) |
bool | openSlave () |
KPty & | operator= (const KPty &)=delete |
void | setCTty () |
void | setCTtyEnabled (bool enable) |
bool | setEcho (bool echo) |
bool | setWinSize (int lines, int columns) |
bool | setWinSize (int lines, int columns, int height, int width) |
int | slaveFd () const |
bool | tcGetAttr (struct ::termios *ttmode) const |
bool | tcSetAttr (struct ::termios *ttmode) |
const char * | ttyName () const |
Protected Member Functions | |
qint64 | readData (char *data, qint64 maxSize) override |
qint64 | readLineData (char *data, qint64 maxSize) override |
qint64 | writeData (const char *data, qint64 maxSize) override |
![]() | |
void | setErrorString (const QString &str) |
void | setOpenMode (QIODeviceBase::OpenMode openMode) |
virtual qint64 | skipData (qint64 maxSize) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
KPTY_NO_EXPORT | KPty (KPtyPrivate *d) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
typedef | OpenMode |
enum | OpenModeFlag |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
![]() | |
std::unique_ptr< KPtyPrivate > const | d_ptr |
Detailed Description
Encapsulates KPty into a QIODevice, so it can be used with Q*Stream, etc.
Definition at line 20 of file kptydevice.h.
Constructor & Destructor Documentation
◆ KPtyDevice()
|
explicit |
Constructor.
Definition at line 448 of file kptydevice.cpp.
◆ ~KPtyDevice()
|
override |
Destructor:
If the pty is still open, it will be closed. Note, however, that an utmp registration is not undone.
Definition at line 454 of file kptydevice.cpp.
Member Function Documentation
◆ atEnd()
|
overridevirtual |
- Reimplemented from superclass.
Reimplemented from QIODevice.
Definition at line 518 of file kptydevice.cpp.
◆ bytesAvailable()
|
overridevirtual |
- Reimplemented from superclass.
Reimplemented from QIODevice.
Definition at line 524 of file kptydevice.cpp.
◆ bytesToWrite()
|
overridevirtual |
- Reimplemented from superclass.
Reimplemented from QIODevice.
Definition at line 530 of file kptydevice.cpp.
◆ canReadLine()
|
overridevirtual |
- Reimplemented from superclass.
Reimplemented from QIODevice.
Definition at line 512 of file kptydevice.cpp.
◆ close()
|
overridevirtual |
Close the pty master/slave pair.
Reimplemented from QIODevice.
Definition at line 491 of file kptydevice.cpp.
◆ isSequential()
|
overridevirtual |
◆ isSuspended()
bool KPtyDevice::isSuspended | ( | ) | const |
Returns true if the KPtyDevice is not monitoring the pty for incoming data.
Do not use on closed ptys.
See setSuspended()
Definition at line 554 of file kptydevice.cpp.
◆ open() [1/2]
bool KPtyDevice::open | ( | int | fd, |
OpenMode | mode = ReadWrite | Unbuffered ) |
Open using an existing pty master.
The ownership of the fd remains with the caller, i.e., close() will not close the fd.
This is useful if you wish to attach a secondary "controller" to an existing pty device such as a terminal widget. Note that you will need to use setSuspended() on both devices to control which one gets the incoming data from the pty.
- Parameters
-
fd an open pty master file descriptor. mode the device mode to open the pty with.
- Returns
- true if a pty pair was successfully opened
Definition at line 477 of file kptydevice.cpp.
◆ open() [2/2]
|
overridevirtual |
Create a pty master/slave pair.
- Returns
- true if a pty pair was successfully opened
Reimplemented from QIODevice.
Definition at line 459 of file kptydevice.cpp.
◆ readData()
|
overrideprotectedvirtual |
Implements QIODevice.
Definition at line 561 of file kptydevice.cpp.
◆ readEof
|
signal |
Emitted when EOF is read from the PTY.
Data may still remain in the buffers.
◆ readLineData()
|
overrideprotectedvirtual |
Reimplemented from QIODevice.
Definition at line 568 of file kptydevice.cpp.
◆ setSuspended()
void KPtyDevice::setSuspended | ( | bool | suspended | ) |
Sets whether the KPtyDevice monitors the pty for incoming data.
When the KPtyDevice is suspended, it will no longer attempt to buffer data that becomes available from the pty and it will not emit any signals.
Do not use on closed ptys. After a call to open(), the pty is not suspended. If you need to ensure that no data is read, call this function before the main loop is entered again (i.e., immediately after opening the pty).
Definition at line 548 of file kptydevice.cpp.
◆ waitForBytesWritten()
|
overridevirtual |
Reimplemented from QIODevice.
Definition at line 542 of file kptydevice.cpp.
◆ waitForReadyRead()
|
overridevirtual |
Reimplemented from QIODevice.
Definition at line 536 of file kptydevice.cpp.
◆ writeData()
|
overrideprotectedvirtual |
Implements QIODevice.
Definition at line 575 of file kptydevice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:42 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.