Kate::TextBuffer
#include <katetextbuffer.h>
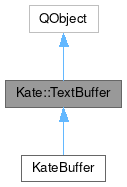
Public Types | |
enum | EndOfLineMode { eolUnknown = -1 , eolUnix = 0 , eolDos = 1 , eolMac = 2 } |
![]() | |
typedef | QObjectList |
Signals | |
void | cleared () |
void | loaded (const QString &filename, bool encodingErrors) |
void | saved (const QString &filename) |
Public Member Functions | |
TextBuffer (KTextEditor::DocumentPrivate *parent, bool alwaysUseKAuth=false) | |
~TextBuffer () override | |
KTEXTEDITOR_NO_EXPORT void | addMultilineRange (TextRange *range) |
virtual void | clear () |
int | cursorToOffset (KTextEditor::Cursor c) const |
void | debugPrint (const QString &title) const |
const QByteArray & | digest () const |
KTextEditor::DocumentPrivate * | document () const |
bool | editingChangedBuffer () const |
bool | editingChangedNumberOfLines () const |
int | editingLastLines () const |
qint64 | editingLastRevision () const |
int | editingMaximalLineChanged () const |
int | editingMinimalLineChanged () const |
int | editingTransactions () const |
KEncodingProber::ProberType | encodingProberType () const |
EndOfLineMode | endOfLineMode () const |
QString | fallbackTextCodec () const |
virtual bool | finishEditing () |
bool | generateByteOrderMark () const |
bool | hasMultlineRange (KTextEditor::MovingRange *range) const |
TextHistory & | history () |
virtual void | insertText (const KTextEditor::Cursor position, const QString &text) |
void | invalidateRanges () |
TextLine | line (int line) const |
int | lineLength (int line) const |
int | lines () const |
virtual bool | load (const QString &filename, bool &encodingErrors, bool &tooLongLinesWrapped, int &longestLineLoaded, bool enforceTextCodec) |
KTextEditor::Cursor | offsetToCursor (int offset) const |
QList< TextRange * > | rangesForLine (int line, KTextEditor::View *view, bool rangesWithAttributeOnly) const |
void | rangesForLine (int line, KTextEditor::View *view, bool rangesWithAttributeOnly, QList< TextRange * > &outRanges) const |
KTEXTEDITOR_NO_EXPORT void | removeMultilineRange (TextRange *range) |
virtual void | removeText (KTextEditor::Range range) |
qint64 | revision () const |
virtual bool | save (const QString &filename) |
void | setDigest (const QByteArray &checksum) |
void | setEncodingProberType (KEncodingProber::ProberType proberType) |
void | setEndOfLineMode (EndOfLineMode endOfLineMode) |
void | setFallbackTextCodec (const QString &codec) |
void | setGenerateByteOrderMark (bool generateByteOrderMark) |
void | setLineLengthLimit (int lineLengthLimit) |
void | setLineMetaData (int line, const TextLine &textLine) |
void | setTextCodec (const QString &codec) |
virtual bool | startEditing () |
QString | text () const |
QString | textCodec () const |
virtual void | unwrapLine (int line) |
virtual void | wrapLine (const KTextEditor::Cursor position) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class representing a text buffer.
The interface is line based, internally the text will be stored in blocks of text lines.
Definition at line 40 of file katetextbuffer.h.
Member Enumeration Documentation
◆ EndOfLineMode
End of line mode.
Definition at line 52 of file katetextbuffer.h.
Constructor & Destructor Documentation
◆ TextBuffer()
|
explicit |
Construct an empty text buffer.
Empty means one empty line in one block.
- Parameters
-
parent parent qobject
Definition at line 51 of file katetextbuffer.cpp.
◆ ~TextBuffer()
|
override |
Destruct the text buffer Virtual, we allow inheritance.
Definition at line 72 of file katetextbuffer.cpp.
Member Function Documentation
◆ addMultilineRange()
void Kate::TextBuffer::addMultilineRange | ( | TextRange * | range | ) |
Add/Remove a multiline range that spans multiple blocks.
Definition at line 1026 of file katetextbuffer.cpp.
◆ clear()
|
virtual |
Clears the buffer, reverts to initial empty state.
Empty means one empty line in one block. Virtual, can be overwritten.
Reimplemented in KateBuffer.
Definition at line 136 of file katetextbuffer.cpp.
◆ cleared
|
signal |
◆ cursorToOffset()
int Kate::TextBuffer::cursorToOffset | ( | KTextEditor::Cursor | c | ) | const |
Retrieve offset in text for the given cursor position.
Definition at line 211 of file katetextbuffer.cpp.
◆ debugPrint()
void Kate::TextBuffer::debugPrint | ( | const QString & | title | ) | const |
Debug output, print whole buffer content with line numbers and line length.
- Parameters
-
title title for this output
Definition at line 632 of file katetextbuffer.cpp.
◆ digest()
const QByteArray & Kate::TextBuffer::digest | ( | ) | const |
Checksum of the document on disk, set either through file loading in openFile() or in KTextEditor::DocumentPrivate::saveFile()
- Returns
- git compatible sha1 checksum for this document
Definition at line 775 of file katetextbuffer.cpp.
◆ document()
|
inline |
Gets the document to which this buffer is bound.
- Returns
- a pointer to the document
Definition at line 483 of file katetextbuffer.h.
◆ editingChangedBuffer()
|
inline |
Query information from the last editing transaction: was the content of the buffer changed? This is checked by comparing the editingLastRevision() with the current revision().
- Returns
- content of buffer was changed in last transaction?
Definition at line 315 of file katetextbuffer.h.
◆ editingChangedNumberOfLines()
|
inline |
Query information from the last editing transaction: was the number of lines of the buffer changed? This is checked by comparing the editingLastLines() with the current lines().
- Returns
- content of buffer was changed in last transaction?
Definition at line 325 of file katetextbuffer.h.
◆ editingLastLines()
|
inline |
Query the number of lines of this buffer before the ongoing editing transactions.
- Returns
- number of lines of buffer before current editing transaction altered it
Definition at line 305 of file katetextbuffer.h.
◆ editingLastRevision()
|
inline |
Query the revision of this buffer before the ongoing editing transactions.
- Returns
- revision of buffer before current editing transaction altered it
Definition at line 296 of file katetextbuffer.h.
◆ editingMaximalLineChanged()
|
inline |
Get maximal line number changed by last editing transaction.
- Returns
- maximal line number changed by last editing transaction, or -1, if none changed
Definition at line 343 of file katetextbuffer.h.
◆ editingMinimalLineChanged()
|
inline |
Get minimal line number changed by last editing transaction.
- Returns
- maximal line number changed by last editing transaction, or -1, if none changed
Definition at line 334 of file katetextbuffer.h.
◆ editingTransactions()
|
inline |
Query the number of editing transactions running atm.
- Returns
- number of running transactions
Definition at line 287 of file katetextbuffer.h.
◆ encodingProberType()
|
inline |
Get encoding prober type for this buffer.
- Returns
- currently in use prober type of this buffer
Definition at line 92 of file katetextbuffer.h.
◆ endOfLineMode()
|
inline |
◆ fallbackTextCodec()
|
inline |
Get fallback codec for this buffer.
- Returns
- currently in use fallback codec of this buffer
Definition at line 110 of file katetextbuffer.h.
◆ finishEditing()
|
virtual |
Finish an editing transaction.
Only allowed to be called if editing transaction is started.
- Returns
- returns true, if this finished last running transaction Virtual, can be overwritten.
Definition at line 308 of file katetextbuffer.cpp.
◆ generateByteOrderMark()
|
inline |
Generate byte order mark on save?
- Returns
- should BOM be generated?
Definition at line 146 of file katetextbuffer.h.
◆ hasMultlineRange()
bool Kate::TextBuffer::hasMultlineRange | ( | KTextEditor::MovingRange * | range | ) | const |
Definition at line 1040 of file katetextbuffer.cpp.
◆ history()
|
inline |
TextHistory of this buffer.
- Returns
- text history for this buffer
Definition at line 381 of file katetextbuffer.h.
◆ insertText()
|
virtual |
Insert text at given cursor position.
Does nothing if text is empty, beside some consistency checks.
- Parameters
-
position position where to insert text text text to insert Virtual, can be overwritten.
Definition at line 427 of file katetextbuffer.cpp.
◆ invalidateRanges()
void Kate::TextBuffer::invalidateRanges | ( | ) |
Invalidate all ranges in this buffer.
Definition at line 117 of file katetextbuffer.cpp.
◆ line()
TextLine Kate::TextBuffer::line | ( | int | line | ) | const |
Retrieve a text line.
- Parameters
-
line wanted line number
- Returns
- text line
Definition at line 193 of file katetextbuffer.cpp.
◆ lineLength()
|
inline |
Retrieve length for line
.
- Parameters
-
line wanted line number
- Returns
- length of the line
Definition at line 242 of file katetextbuffer.h.
◆ lines()
|
inline |
Lines currently stored in this buffer.
This is never 0, even clear will let one empty line remain.
Definition at line 207 of file katetextbuffer.h.
◆ load()
|
virtual |
Load the given file.
This will first clear the buffer and then load the file. Even on error during loading the buffer will still be cleared. Before calling this, setTextCodec must have been used to set codec!
- Parameters
-
filename file to open encodingErrors were there problems occurred while decoding the file? tooLongLinesWrapped were too long lines found and wrapped? longestLineLoaded the longest line in the file (before wrapping) enforceTextCodec enforce to use only the set text codec
- Returns
- success, the file got loaded, perhaps with encoding errors Virtual, can be overwritten.
Definition at line 643 of file katetextbuffer.cpp.
◆ loaded
|
signal |
Buffer loaded successfully a file.
- Parameters
-
filename file which was loaded encodingErrors were there problems occurred while decoding the file?
◆ offsetToCursor()
KTextEditor::Cursor Kate::TextBuffer::offsetToCursor | ( | int | offset | ) | const |
Retrieve cursor in text for the given offset.
Definition at line 238 of file katetextbuffer.cpp.
◆ rangesForLine() [1/2]
|
inline |
Return the ranges which affect the given line.
- Parameters
-
line line to look at view only return ranges associated with given view rangesWithAttributeOnly only return ranges which have a attribute set
- Returns
- list of ranges affecting this line
Definition at line 501 of file katetextbuffer.h.
◆ rangesForLine() [2/2]
void Kate::TextBuffer::rangesForLine | ( | int | line, |
KTextEditor::View * | view, | ||
bool | rangesWithAttributeOnly, | ||
QList< TextRange * > & | outRanges ) const |
Definition at line 1045 of file katetextbuffer.cpp.
◆ removeMultilineRange()
void Kate::TextBuffer::removeMultilineRange | ( | TextRange * | range | ) |
Definition at line 1035 of file katetextbuffer.cpp.
◆ removeText()
|
virtual |
Remove text at given range.
Does nothing if range is empty, beside some consistency checks.
- Parameters
-
range range of text to remove, must be on one line only. Virtual, can be overwritten.
Definition at line 463 of file katetextbuffer.cpp.
◆ revision()
|
inline |
Revision of this buffer.
Is set to 0 on construction, clear() (load will trigger clear()). Is incremented on each change to the buffer.
- Returns
- current revision
Definition at line 218 of file katetextbuffer.h.
◆ save()
|
virtual |
Save the current buffer content to the given file.
Before calling this, setTextCodec and setFallbackTextCodec must have been used to set codec!
- Parameters
-
filename file to save
- Returns
- success Virtual, can be overwritten.
Definition at line 805 of file katetextbuffer.cpp.
◆ saved
|
signal |
Buffer saved successfully a file.
- Parameters
-
filename file which was saved
◆ setDigest()
void Kate::TextBuffer::setDigest | ( | const QByteArray & | checksum | ) |
Set the checksum of this buffer.
Make sure this checksum is up-to-date when reading digest().
- Parameters
-
checksum git compatible sha1 digest for the document on disk
Definition at line 780 of file katetextbuffer.cpp.
◆ setEncodingProberType()
|
inline |
Set encoding prober type for this buffer to use for load.
- Parameters
-
proberType prober type to use for encoding
Definition at line 83 of file katetextbuffer.h.
◆ setEndOfLineMode()
|
inline |
Set end of line mode for this buffer, not allowed to be set to unknown.
Loading might overwrite this setting, if there is a eol found inside the file.
- Parameters
-
endOfLineMode new eol mode
Definition at line 156 of file katetextbuffer.h.
◆ setFallbackTextCodec()
|
inline |
Set fallback codec for this buffer to use for load.
- Parameters
-
codec fallback to use for encoding
Definition at line 101 of file katetextbuffer.h.
◆ setGenerateByteOrderMark()
|
inline |
Generate byte order mark on save.
Loading might overwrite this setting, if there is a BOM found inside the file.
- Parameters
-
generateByteOrderMark should BOM be generated?
Definition at line 137 of file katetextbuffer.h.
◆ setLineLengthLimit()
|
inline |
Set line length limit.
- Parameters
-
lineLengthLimit new line length limit
Definition at line 175 of file katetextbuffer.h.
◆ setLineMetaData()
void Kate::TextBuffer::setLineMetaData | ( | int | line, |
const TextLine & | textLine ) |
Transfer all non text attributes for the given line from the given text line to the one in the buffer.
- Parameters
-
line line number to set attributes textLine line reference to get attributes from
Definition at line 202 of file katetextbuffer.cpp.
◆ setTextCodec()
void Kate::TextBuffer::setTextCodec | ( | const QString & | codec | ) |
Set codec for this buffer to use for load/save.
Loading might overwrite this, if it encounters problems and finds a better codec. Might change BOM setting.
- Parameters
-
codec to use for encoding
Definition at line 785 of file katetextbuffer.cpp.
◆ startEditing()
|
virtual |
Start an editing transaction, the wrapLine/unwrapLine/insertText and removeText functions are only allowed to be called inside a editing transaction.
Editing transactions can stack. The number of startEdit and endEdit calls must match.
- Returns
- returns true, if no transaction was already running Virtual, can be overwritten.
Definition at line 285 of file katetextbuffer.cpp.
◆ text()
QString Kate::TextBuffer::text | ( | ) | const |
Retrieve text of complete buffer.
- Returns
- text for this buffer, lines separated by '
'
Definition at line 265 of file katetextbuffer.cpp.
◆ textCodec()
|
inline |
Get codec for this buffer.
- Returns
- currently in use codec of this buffer
Definition at line 127 of file katetextbuffer.h.
◆ unwrapLine()
|
virtual |
Unwrap given line.
- Parameters
-
line line to unwrap Virtual, can be overwritten.
Reimplemented in KateBuffer.
Definition at line 374 of file katetextbuffer.cpp.
◆ wrapLine()
|
virtual |
Wrap line at given cursor position.
- Parameters
-
position line/column as cursor where to wrap Virtual, can be overwritten.
Reimplemented in KateBuffer.
Definition at line 334 of file katetextbuffer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:11:28 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.