KUserFeedback::AbstractDataSource
#include <abstractdatasource.h>
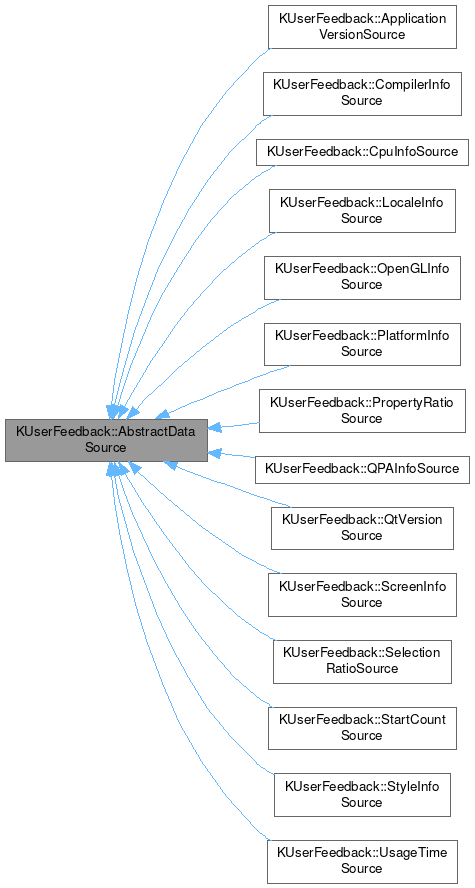
Public Member Functions | |
virtual QVariant | data ()=0 |
virtual QString | description () const =0 |
QString | id () const |
bool | isActive () const |
void | load (QSettings *settings) |
virtual QString | name () const |
void | reset (QSettings *settings) |
void | setActive (bool active) |
void | setTelemetryMode (Provider::TelemetryMode mode) |
void | store (QSettings *settings) |
Provider::TelemetryMode | telemetryMode () const |
Protected Member Functions | |
AbstractDataSource (const QString &id, Provider::TelemetryMode mode=Provider::DetailedUsageStatistics) | |
virtual void | loadImpl (QSettings *settings) |
virtual void | resetImpl (QSettings *settings) |
void | setId (const QString &id) |
virtual void | storeImpl (QSettings *settings) |
Detailed Description
Base class for data sources for telemetry data.
Definition at line 24 of file abstractdatasource.h.
Constructor & Destructor Documentation
◆ ~AbstractDataSource()
|
virtual |
Definition at line 68 of file abstractdatasource.cpp.
◆ AbstractDataSource()
|
explicitprotected |
Create a new data source named name
.
The name of the data source must match the corresponding product schema entry.
- Parameters
-
id Must not be empty. mode The default telemetry mode.
Definition at line 55 of file abstractdatasource.cpp.
Member Function Documentation
◆ data()
|
pure virtual |
Returns the data gathered by this source.
Implement this to return the data provided by this source. One of the three following formats are expected:
- scalar entries: QAssociativeIterable
- list entries: QSequentialIterable containing QAssociativeIterable
- map entries: QAssociativeIterable containing QAssociativeIterable
The innermost QAssociativeIterable must only contain one of the following base types (which has to match the corresponding schema entry element):
- QString
- int
- double
- bool
All keys must be strings.
- Returns
- A variant complying with the above requirements.
Implemented in KUserFeedback::ApplicationVersionSource, KUserFeedback::CompilerInfoSource, KUserFeedback::CpuInfoSource, KUserFeedback::LocaleInfoSource, KUserFeedback::OpenGLInfoSource, KUserFeedback::PlatformInfoSource, KUserFeedback::PropertyRatioSource, KUserFeedback::QPAInfoSource, KUserFeedback::QtVersionSource, KUserFeedback::ScreenInfoSource, KUserFeedback::SelectionRatioSource, KUserFeedback::StartCountSource, KUserFeedback::StyleInfoSource, and KUserFeedback::UsageTimeSource.
◆ description()
|
pure virtual |
Returns a human-readable, translated description of what this source provides.
- See also
- id()
- Returns
- A translated, human-readable string.
Implemented in KUserFeedback::ApplicationVersionSource, KUserFeedback::CompilerInfoSource, KUserFeedback::CpuInfoSource, KUserFeedback::LocaleInfoSource, KUserFeedback::OpenGLInfoSource, KUserFeedback::PlatformInfoSource, KUserFeedback::PropertyRatioSource, KUserFeedback::QPAInfoSource, KUserFeedback::QtVersionSource, KUserFeedback::ScreenInfoSource, KUserFeedback::SelectionRatioSource, KUserFeedback::StartCountSource, KUserFeedback::StyleInfoSource, and KUserFeedback::UsageTimeSource.
◆ id()
QString AbstractDataSource::id | ( | ) | const |
Returns the ID of this data source.
This is used as identifier towards the server and should not be shown to the user.
- See also
- description()
- Returns
- The data source identifier configured on the feedback server.
Definition at line 73 of file abstractdatasource.cpp.
◆ isActive()
bool AbstractDataSource::isActive | ( | ) | const |
Checks whether this data source is active or not If the data source is not active, then collected data neither will be sent to a server nor appeared in the audit log.
Data source is active by default.
- Returns
- true if active, false otherwise
Definition at line 144 of file abstractdatasource.cpp.
◆ load()
void AbstractDataSource::load | ( | QSettings * | settings | ) |
Load persistent state for this data source.
- Parameters
-
settings A QSettings object opened in a dedicated group for loading persistent data.
Definition at line 103 of file abstractdatasource.cpp.
◆ loadImpl()
|
protectedvirtual |
Invoked by load()
in order to load individual settings of this data source.
- See also
- load() description for further details.
- Parameters
-
settings A QSettings object opened in a dedicated group for loading persistent data.
Reimplemented in KUserFeedback::PropertyRatioSource, and KUserFeedback::SelectionRatioSource.
Definition at line 88 of file abstractdatasource.cpp.
◆ name()
|
virtual |
Returns a short name of this data source.
Can be empty if short name is meaningless for this data source.
- Returns
- A translated, human-readable string.
Reimplemented in KUserFeedback::ApplicationVersionSource, KUserFeedback::CompilerInfoSource, KUserFeedback::CpuInfoSource, KUserFeedback::LocaleInfoSource, KUserFeedback::OpenGLInfoSource, KUserFeedback::PlatformInfoSource, KUserFeedback::PropertyRatioSource, KUserFeedback::QPAInfoSource, KUserFeedback::QtVersionSource, KUserFeedback::ScreenInfoSource, KUserFeedback::StartCountSource, KUserFeedback::StyleInfoSource, and KUserFeedback::UsageTimeSource.
Definition at line 78 of file abstractdatasource.cpp.
◆ reset()
void AbstractDataSource::reset | ( | QSettings * | settings | ) |
Reset the persistent state of this data source.
This is called after a successful submission of data, and can be used by sources that track differential rather than absolute data to reset their counters.
- Parameters
-
settings A QSettings object opened in the dedicated group of this data source.
Definition at line 119 of file abstractdatasource.cpp.
◆ resetImpl()
|
protectedvirtual |
Invoked by reset()
in order to reset individual settings of this data source.
- See also
- reset() description for further details.
- Parameters
-
settings A QSettings object opened in a dedicated group for loading persistent data.
Reimplemented in KUserFeedback::PropertyRatioSource, and KUserFeedback::SelectionRatioSource.
Definition at line 98 of file abstractdatasource.cpp.
◆ setActive()
void AbstractDataSource::setActive | ( | bool | active | ) |
Changes active state of the data source.
- Parameters
-
active The new active state for this data source
Definition at line 150 of file abstractdatasource.cpp.
◆ setId()
|
protected |
Set the ID of this data source.
The ID should not change at runtime, this is only provided for enabling QML API for generic sources.
- See also
- id()
Definition at line 83 of file abstractdatasource.cpp.
◆ setTelemetryMode()
void AbstractDataSource::setTelemetryMode | ( | Provider::TelemetryMode | mode | ) |
Sets which telemetry colleciton mode this data source belongs to.
- Parameters
-
mode The data collection mode of this source. Provider::NoTelemetry is not allowed here.
Definition at line 137 of file abstractdatasource.cpp.
◆ store()
void AbstractDataSource::store | ( | QSettings * | settings | ) |
Store persistent state for this data source.
- Parameters
-
settings A QSettings object opened in a dedicated group for storing persistent data.
Definition at line 111 of file abstractdatasource.cpp.
◆ storeImpl()
|
protectedvirtual |
Invoked by store()
in order to store individual settings of this data source.
- See also
- store() description for further details.
- Parameters
-
settings A QSettings object opened in a dedicated group for loading persistent data.
Reimplemented in KUserFeedback::PropertyRatioSource, and KUserFeedback::SelectionRatioSource.
Definition at line 93 of file abstractdatasource.cpp.
◆ telemetryMode()
Provider::TelemetryMode AbstractDataSource::telemetryMode | ( | ) | const |
Returns which telemetry colleciton mode this data source belongs to.
- Returns
- The telemetry collection category this source belongs to.
Definition at line 127 of file abstractdatasource.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:57:44 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.