KUserFeedback::Provider
#include <provider.h>
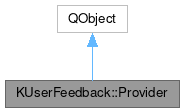
Public Types | |
enum | TelemetryMode { NoTelemetry , BasicSystemInformation = 0x10 , BasicUsageStatistics = 0x20 , DetailedSystemInformation = 0x30 , DetailedUsageStatistics = 0x40 } |
Properties | |
int | applicationStartsUntilEncouragement |
int | applicationUsageTimeUntilEncouragement |
QString | describeDataSources |
bool | enabled |
int | encouragementDelay |
int | encouragementInterval |
QUrl | feedbackServer |
QString | productIdentifier |
int | submissionInterval |
int | surveyInterval |
TelemetryMode | telemetryMode |
![]() | |
objectName | |
Signals | |
void | dataSourcesChanged () |
void | enabledChanged () |
void | providerSettingsChanged () |
void | showEncouragementMessage () |
void | surveyAvailable (const KUserFeedback::SurveyInfo &survey) |
void | surveyIntervalChanged () |
void | telemetryModeChanged () |
Public Slots | |
void | load () |
void | store () |
void | submit () |
void | surveyCompleted (const KUserFeedback::SurveyInfo &info) |
Public Member Functions | |
Provider (QObject *parent=nullptr) | |
void | addDataSource (AbstractDataSource *source) |
int | applicationStartsUntilEncouragement () const |
int | applicationUsageTimeUntilEncouragement () const |
AbstractDataSource * | dataSource (const QString &id) const |
QVector< AbstractDataSource * > | dataSources () const |
QString | describeDataSources () const |
int | encouragementDelay () const |
int | encouragementInterval () const |
QUrl | feedbackServer () const |
bool | isEnabled () const |
QString | productIdentifier () const |
void | restoreDefaults () |
void | setApplicationStartsUntilEncouragement (int starts) |
void | setApplicationUsageTimeUntilEncouragement (int secs) |
void | setEnabled (bool enabled) |
void | setEncouragementDelay (int secs) |
void | setEncouragementInterval (int days) |
void | setFeedbackServer (const QUrl &url) |
void | setProductIdentifier (const QString &productId) |
void | setSubmissionInterval (int days) |
void | setSurveyInterval (int days) |
void | setTelemetryMode (TelemetryMode mode) |
int | submissionInterval () const |
int | surveyInterval () const |
TelemetryMode | telemetryMode () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The central object managing data sources and transmitting feedback to the server.
The defaults for this class are very defensive, so in order to make it actually operational and submit data, there is a number of settings you need to set in code, namely submission intervals, encouragement settings and adding data sources. The settings about what data to submit (telemetryMode) and how often to bother the user with surveys (surveyInterval) should not be set to hardcoded values in code, but left as choices to the user.
Definition at line 31 of file provider.h.
Member Enumeration Documentation
◆ TelemetryMode
Telemetry collection modes.
Collection modes are inclusive, ie. higher modes always imply data from lower modes too.
Definition at line 102 of file provider.h.
Property Documentation
◆ applicationStartsUntilEncouragement
|
readwrite |
Times the application has to be started before an encouragement message is shown.
Definition at line 70 of file provider.h.
◆ applicationUsageTimeUntilEncouragement
|
readwrite |
Application usage time in seconds before an encouragement message is shown.
Definition at line 78 of file provider.h.
◆ describeDataSources
|
read |
Definition at line 95 of file provider.h.
◆ enabled
|
readwrite |
The global enabled state of the feedback functionality.
If this is false
, all feedback functionality has to be disabled completely.
Definition at line 37 of file provider.h.
◆ encouragementDelay
|
readwrite |
Encouragement delay after application start in seconds.
- See also
- setEncouragementDelay
Definition at line 86 of file provider.h.
◆ encouragementInterval
|
readwrite |
◆ feedbackServer
|
readwrite |
◆ productIdentifier
|
readwrite |
Unique product id as set on the feedback server.
- See also
- setProductIdentifier
Definition at line 55 of file provider.h.
◆ submissionInterval
|
readwrite |
Submission interval in days.
- See also
- setSubmissionInterval
Definition at line 65 of file provider.h.
◆ surveyInterval
|
readwrite |
The interval in which the user accepts surveys.
This should be configurable for the user. -1
indicates surveys are disabled.
- See also
- surveyInterval(), setSurveyInterval()
Definition at line 44 of file provider.h.
◆ telemetryMode
|
readwrite |
The telemetry mode the user has configured.
This should be configurable for the user.
- See also
- telemetryMode(), setTelemetryMode()
Definition at line 50 of file provider.h.
Constructor & Destructor Documentation
◆ Provider()
|
explicit |
Create a new feedback provider.
- Parameters
-
parent The parent object.
Definition at line 399 of file provider.cpp.
◆ ~Provider()
|
override |
Definition at line 416 of file provider.cpp.
Member Function Documentation
◆ addDataSource()
void Provider::addDataSource | ( | AbstractDataSource * | source | ) |
Adds a data source for telemetry data collection.
- Parameters
-
source The data source to add. The Provider takes ownership of source
.
Definition at line 508 of file provider.cpp.
◆ applicationStartsUntilEncouragement()
int Provider::applicationStartsUntilEncouragement | ( | ) | const |
Returns the amount of application starts before an encouragement message is shown.
Definition at line 555 of file provider.cpp.
◆ applicationUsageTimeUntilEncouragement()
int Provider::applicationUsageTimeUntilEncouragement | ( | ) | const |
Returns the amount of application usage time before an encouragement message is shown.
Definition at line 569 of file provider.cpp.
◆ dataSource()
AbstractDataSource * Provider::dataSource | ( | const QString & | id | ) | const |
Returns a data source with matched id
.
- Parameters
-
id data source unique identifier
- Returns
- pointer to found data source or nullptr if data source is not found
Definition at line 531 of file provider.cpp.
◆ dataSources()
QVector< AbstractDataSource * > Provider::dataSources | ( | ) | const |
Returns all data sources that have been added to this provider.
- See also
- addDataSource
Definition at line 526 of file provider.cpp.
◆ dataSourcesChanged
|
signal |
Emitted when a data source is added or removed.
◆ describeDataSources()
QString Provider::describeDataSources | ( | ) | const |
Returns a string with each source and its enable mode.
Definition at line 734 of file provider.cpp.
◆ enabledChanged
|
signal |
Emitted when the global enabled state changed.
◆ encouragementDelay()
int Provider::encouragementDelay | ( | ) | const |
Returns the current encouragement delay in seconds.
Definition at line 583 of file provider.cpp.
◆ encouragementInterval()
int Provider::encouragementInterval | ( | ) | const |
Returns the current encouragement interval.
Definition at line 597 of file provider.cpp.
◆ feedbackServer()
QUrl Provider::feedbackServer | ( | ) | const |
Returns the current feedback server URL.
Definition at line 464 of file provider.cpp.
◆ isEnabled()
bool Provider::isEnabled | ( | ) | const |
Returns whether feedback functionality is enabled on this system.
This should be checked everywhere showing feedback UI to the user to respect the global "kill switch" for this. Provider does check this internally for encouragements, surveys and telemetry submission.
Definition at line 421 of file provider.cpp.
◆ load
|
slot |
Manually load settings of the provider and all added data sources.
Automatically invoked after object construction and changing product ID.
- Note
- Potentially long operation.
Definition at line 624 of file provider.cpp.
◆ productIdentifier()
QString Provider::productIdentifier | ( | ) | const |
Returns the current product identifier.
Definition at line 442 of file provider.cpp.
◆ providerSettingsChanged
|
signal |
Emitted when any provider setting changed.
◆ restoreDefaults()
void Provider::restoreDefaults | ( | ) |
Set the telemetry mode and the survey interval back to their default values.
- See also
- telemetryMode(), surveyInterval()
- Since
- 1.1.0
Definition at line 436 of file provider.cpp.
◆ setApplicationStartsUntilEncouragement()
void Provider::setApplicationStartsUntilEncouragement | ( | int | starts | ) |
Set the amount of application starts until the encouragement message should be shown.
The default is -1, ie. no encouragement based on application starts.
- Parameters
-
starts The amount of application starts after which an encouragement message should be displayed.
Definition at line 560 of file provider.cpp.
◆ setApplicationUsageTimeUntilEncouragement()
void Provider::setApplicationUsageTimeUntilEncouragement | ( | int | secs | ) |
Set the amount of usage time until the encouragement message should be shown.
The default is -1, ie. no encouragement based on application usage time.
- Parameters
-
secs Amount of seconds until the encouragement should be shown.
Definition at line 574 of file provider.cpp.
◆ setEnabled()
void Provider::setEnabled | ( | bool | enabled | ) |
Set the global (user-wide) activation state for feedback functionality.
- See also
- isEnabled
Definition at line 428 of file provider.cpp.
◆ setEncouragementDelay()
void Provider::setEncouragementDelay | ( | int | secs | ) |
Set the delay after application start for the earliest display of the encouragement message.
The default is 300, ie. 5 minutes after the application start.
- Note
- This only adds an additional constraint on usage time and startup count based encouragement messages, it does not actually trigger encouragement messages itself.
- Parameters
-
secs Amount of seconds after the application start for the earliest display of an encouragement message.
Definition at line 588 of file provider.cpp.
◆ setEncouragementInterval()
void Provider::setEncouragementInterval | ( | int | days | ) |
Sets the interval after the encouragement should be repeated.
Encouragement messages are only repeated if no feedback options have been enabled. The default is -1, that is no repeated encouragement at all.
- Parameters
-
days Days between encouragement messages, 0 disables repeated encouragements.
Definition at line 602 of file provider.cpp.
◆ setFeedbackServer()
void Provider::setFeedbackServer | ( | const QUrl & | url | ) |
Set the feedback server URL.
This must be called with an appropriate URL for this class to be operational.
- Parameters
-
url The URL of the feedback server.
Definition at line 469 of file provider.cpp.
◆ setProductIdentifier()
void Provider::setProductIdentifier | ( | const QString & | productId | ) |
Set the product identifier.
This is used to distinguish independent products on the same server. If this is not specified, the product identifier is derived from the application name organisation domain specified in QCoreApplication.
- Parameters
-
productId Unique product identifier, as configured on the feedback server.
Definition at line 447 of file provider.cpp.
◆ setSubmissionInterval()
void Provider::setSubmissionInterval | ( | int | days | ) |
Set the automatic submission interval in days.
This must be called with a positive number for this class to be operational, as the default is -1 (no submission ever).
Definition at line 482 of file provider.cpp.
◆ setSurveyInterval()
void Provider::setSurveyInterval | ( | int | days | ) |
Sets the minimum time in days between two surveys.
-1
indicates no surveys should be requested. 0
indicates no minimum time between surveys at all (i.e. bother the user as often as you want).
Definition at line 542 of file provider.cpp.
◆ setTelemetryMode()
void Provider::setTelemetryMode | ( | TelemetryMode | mode | ) |
Set which telemetry data should be submitted.
Definition at line 496 of file provider.cpp.
◆ showEncouragementMessage
|
signal |
Indicate that the encouragement notice should be shown.
◆ store
|
slot |
Manually store settings of the provider and all added data sources.
Will be automatically invoked upon QCoreApplication::aboutToQuit
signal.
- Note
- Potentially long operation.
Definition at line 629 of file provider.cpp.
◆ submissionInterval()
int Provider::submissionInterval | ( | ) | const |
Returns the current submission interval.
- Returns
- Days between telemetry submissions, or -1 if submission is off.
Definition at line 477 of file provider.cpp.
◆ submit
|
slot |
Manually submit currently recorded data.
Definition at line 634 of file provider.cpp.
◆ surveyAvailable
|
signal |
Emitted whenever there is a new survey available that can be presented to the user.
◆ surveyCompleted
|
slot |
Marks the given survey as completed.
This avoids getting further notification about the same survey.
Definition at line 611 of file provider.cpp.
◆ surveyInterval()
int Provider::surveyInterval | ( | ) | const |
Returns the minimum time between two surveys in days.
The default is -1 (no surveys enabled).
Definition at line 537 of file provider.cpp.
◆ surveyIntervalChanged
|
signal |
Emitted when the survey interval changed.
◆ telemetryMode()
Provider::TelemetryMode Provider::telemetryMode | ( | ) | const |
Returns the current telemetry collection mode.
The default is NoTelemetry.
Definition at line 491 of file provider.cpp.
◆ telemetryModeChanged
|
signal |
Emitted when the telemetry collection mode has changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:56:44 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.