KUserFeedback::FeedbackConfigUiController
#include <feedbackconfiguicontroller.h>
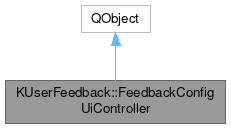
Properties | |
QString | applicationName |
KUserFeedback::Provider * | feedbackProvider |
int | surveyModeCount |
int | telemetryModeCount |
![]() | |
objectName | |
Signals | |
void | applicationNameChanged (const QString &applicationName) |
void | providerChanged () |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Logic/behavior of the feedback configuration UI.
This is available for use in e.g. QtQuick-based UIs.
- See also
- FeedbackConfigWidget
Definition at line 26 of file feedbackconfiguicontroller.h.
Property Documentation
◆ applicationName
|
readwrite |
Name of the application that will appear on descriptions.
By default it will use QGuiApplication::applicationDisplayName()
Definition at line 36 of file feedbackconfiguicontroller.h.
◆ feedbackProvider
|
readwrite |
The Provider instance we are configuring.
Definition at line 30 of file feedbackconfiguicontroller.h.
◆ surveyModeCount
|
read |
Amount of supported survey modes.
Definition at line 34 of file feedbackconfiguicontroller.h.
◆ telemetryModeCount
|
read |
Amount of telemetry modes supported by the provider.
Definition at line 32 of file feedbackconfiguicontroller.h.
Constructor & Destructor Documentation
◆ FeedbackConfigUiController()
|
explicit |
Definition at line 36 of file feedbackconfiguicontroller.cpp.
◆ ~FeedbackConfigUiController()
|
override |
Definition at line 42 of file feedbackconfiguicontroller.cpp.
Member Function Documentation
◆ applicationName()
QString FeedbackConfigUiController::applicationName | ( | ) | const |
Definition at line 263 of file feedbackconfiguicontroller.cpp.
◆ feedbackProvider()
Provider * FeedbackConfigUiController::feedbackProvider | ( | ) | const |
Returns the feedback provider to be configured.
Definition at line 46 of file feedbackconfiguicontroller.cpp.
◆ providerChanged
|
signal |
A provider-related setting has changed.
◆ setApplicationName()
void FeedbackConfigUiController::setApplicationName | ( | const QString & | appName | ) |
Definition at line 268 of file feedbackconfiguicontroller.cpp.
◆ setFeedbackProvider()
void FeedbackConfigUiController::setFeedbackProvider | ( | Provider * | provider | ) |
Set the feedback provider to configure.
Definition at line 51 of file feedbackconfiguicontroller.cpp.
◆ surveyIndexToInterval()
int FeedbackConfigUiController::surveyIndexToInterval | ( | int | index | ) | const |
Convert slider index to survey interval.
Definition at line 205 of file feedbackconfiguicontroller.cpp.
◆ surveyIntervalToIndex()
int FeedbackConfigUiController::surveyIntervalToIndex | ( | int | interval | ) | const |
Convert survey interval to slider index.
Definition at line 215 of file feedbackconfiguicontroller.cpp.
◆ surveyModeCount()
int FeedbackConfigUiController::surveyModeCount | ( | ) | const |
Amount of supported survey modes.
Definition at line 85 of file feedbackconfiguicontroller.cpp.
◆ surveyModeDescription()
QString FeedbackConfigUiController::surveyModeDescription | ( | int | surveyIndex | ) | const |
Survey mode explanation text.
Definition at line 225 of file feedbackconfiguicontroller.cpp.
◆ telemetryDescription()
QString FeedbackConfigUiController::telemetryDescription | ( | KUserFeedback::Provider::TelemetryMode | mode | ) | const |
Telemetry mode explanation text.
Definition at line 133 of file feedbackconfiguicontroller.cpp.
◆ telemetryIndexToMode()
Provider::TelemetryMode FeedbackConfigUiController::telemetryIndexToMode | ( | int | index | ) | const |
Convert slider index to telemetry mode.
Definition at line 90 of file feedbackconfiguicontroller.cpp.
◆ telemetryModeCount()
int FeedbackConfigUiController::telemetryModeCount | ( | ) | const |
Amount of supported telemetry modes.
This depends on what type of sources the provider actually has.
Definition at line 80 of file feedbackconfiguicontroller.cpp.
◆ telemetryModeDescription()
QString FeedbackConfigUiController::telemetryModeDescription | ( | int | telemetryIndex | ) | const |
Telemetry mode explanation text.
Definition at line 110 of file feedbackconfiguicontroller.cpp.
◆ telemetryModeDetails()
QString FeedbackConfigUiController::telemetryModeDetails | ( | int | telemetryIndex | ) | const |
Detailed information about the data sources of the given telemetry mode index.
Definition at line 187 of file feedbackconfiguicontroller.cpp.
◆ telemetryModeName()
QString FeedbackConfigUiController::telemetryModeName | ( | int | telemetryIndex | ) | const |
Telemetry mode short name.
Definition at line 105 of file feedbackconfiguicontroller.cpp.
◆ telemetryModeToIndex()
int FeedbackConfigUiController::telemetryModeToIndex | ( | KUserFeedback::Provider::TelemetryMode | mode | ) | const |
Convert telemetry mode to slider index.
Definition at line 97 of file feedbackconfiguicontroller.cpp.
◆ telemetryName()
QString FeedbackConfigUiController::telemetryName | ( | KUserFeedback::Provider::TelemetryMode | mode | ) | const |
Telemetry mode short name.
Definition at line 115 of file feedbackconfiguicontroller.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:16:29 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.