NetworkManager::MacsecSetting
#include <macsecsetting.h>
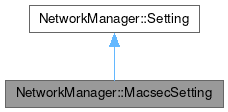
Public Types | |
typedef QList< Ptr > | List |
enum | Mode { Psk , Eap } |
typedef QSharedPointer< MacsecSetting > | Ptr |
enum | Validation { Disable , Check , Strict } |
![]() | |
typedef QList< Ptr > | List |
enum | MacAddressRandomization { MacAddressRandomizationDefault = 0 , MacAddressRandomizationNever , MacAddressRandomizationAlways } |
typedef QSharedPointer< Setting > | Ptr |
typedef QFlags< SecretFlagType > | SecretFlags |
enum | SecretFlagType { None = 0 , AgentOwned = 0x01 , NotSaved = 0x02 , NotRequired = 0x04 } |
enum | SettingType { Adsl , Cdma , Gsm , Infiniband , Ipv4 , Ipv6 , Ppp , Pppoe , Security8021x , Serial , Vpn , Wired , Wireless , WirelessSecurity , Bluetooth , OlpcMesh , Vlan , Wimax , Bond , Bridge , BridgePort , Team , Generic , Tun , Vxlan , IpTunnel , Proxy , User , OvsBridge , OvsInterface , OvsPatch , OvsPort , Match , Tc , TeamPort , Macsec , Dcb , WireGuard } |
Public Member Functions | |
MacsecSetting (const Ptr &other) | |
bool | encrypt () const |
void | fromMap (const QVariantMap &setting) override |
QString | mkaCak () const |
Setting::SecretFlags | mkaCakFlags () const |
QString | mkaCkn () const |
Mode | mode () const |
QString | name () const override |
QStringList | needSecrets (bool requestNew=false) const override |
QString | parent () const |
qint32 | port () const |
void | secretsFromMap (const QVariantMap &secrets) override |
QVariantMap | secretsToMap () const override |
bool | sendSci () const |
void | setEncrypt (bool encrypt) |
void | setMkaCak (const QString &mkaCak) |
void | setMkaCakFlags (Setting::SecretFlags flags) |
void | setMkaCkn (const QString &mkaCkn) |
void | setMode (Mode mode) |
void | setParent (const QString &parent) |
void | setPort (qint32 port) |
void | setSendSci (bool sendSci) |
void | setValidation (Validation validation) |
QVariantMap | toMap () const override |
Validation | validation () const |
![]() | |
Setting (const Ptr &setting) | |
Setting (SettingType type) | |
bool | isNull () const |
virtual void | secretsFromStringMap (const NMStringMap &map) |
virtual NMStringMap | secretsToStringMap () const |
void | setInitialized (bool initialized) |
void | setType (SettingType type) |
SettingType | type () const |
Protected Attributes | |
MacsecSettingPrivate * | d_ptr |
![]() | |
SettingPrivate * | d_ptr |
Additional Inherited Members | |
![]() | |
static QString | typeAsString (SettingType type) |
static SettingType | typeFromString (const QString &type) |
Detailed Description
Represents Macsec setting.
Definition at line 20 of file macsecsetting.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> NetworkManager::MacsecSetting::List |
Definition at line 24 of file macsecsetting.h.
◆ Ptr
Definition at line 23 of file macsecsetting.h.
Member Enumeration Documentation
◆ Mode
enum NetworkManager::MacsecSetting::Mode |
Definition at line 26 of file macsecsetting.h.
◆ Validation
enum NetworkManager::MacsecSetting::Validation |
Definition at line 31 of file macsecsetting.h.
Constructor & Destructor Documentation
◆ MacsecSetting() [1/2]
NetworkManager::MacsecSetting::MacsecSetting | ( | ) |
Definition at line 39 of file macsecsetting.cpp.
◆ MacsecSetting() [2/2]
|
explicit |
Definition at line 45 of file macsecsetting.cpp.
◆ ~MacsecSetting()
|
override |
Definition at line 60 of file macsecsetting.cpp.
Member Function Documentation
◆ encrypt()
bool NetworkManager::MacsecSetting::encrypt | ( | ) | const |
Definition at line 79 of file macsecsetting.cpp.
◆ fromMap()
|
overridevirtual |
Must be reimplemented, default implementation does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 227 of file macsecsetting.cpp.
◆ mkaCak()
QString NetworkManager::MacsecSetting::mkaCak | ( | ) | const |
Definition at line 93 of file macsecsetting.cpp.
◆ mkaCakFlags()
NetworkManager::Setting::SecretFlags NetworkManager::MacsecSetting::mkaCakFlags | ( | ) | const |
Definition at line 191 of file macsecsetting.cpp.
◆ mkaCkn()
QString NetworkManager::MacsecSetting::mkaCkn | ( | ) | const |
Definition at line 107 of file macsecsetting.cpp.
◆ mode()
NetworkManager::MacsecSetting::Mode NetworkManager::MacsecSetting::mode | ( | ) | const |
Definition at line 121 of file macsecsetting.cpp.
◆ name()
|
overridevirtual |
Must be reimplemented, default implementationd does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 65 of file macsecsetting.cpp.
◆ needSecrets()
|
overridevirtual |
Reimplemented from NetworkManager::Setting.
Definition at line 198 of file macsecsetting.cpp.
◆ parent()
QString NetworkManager::MacsecSetting::parent | ( | ) | const |
Definition at line 135 of file macsecsetting.cpp.
◆ port()
qint32 NetworkManager::MacsecSetting::port | ( | ) | const |
Definition at line 149 of file macsecsetting.cpp.
◆ secretsFromMap()
|
overridevirtual |
Reimplemented from NetworkManager::Setting.
Definition at line 209 of file macsecsetting.cpp.
◆ secretsToMap()
|
overridevirtual |
Reimplemented from NetworkManager::Setting.
Definition at line 216 of file macsecsetting.cpp.
◆ sendSci()
bool NetworkManager::MacsecSetting::sendSci | ( | ) | const |
Definition at line 163 of file macsecsetting.cpp.
◆ setEncrypt()
void NetworkManager::MacsecSetting::setEncrypt | ( | bool | encrypt | ) |
Definition at line 72 of file macsecsetting.cpp.
◆ setMkaCak()
void NetworkManager::MacsecSetting::setMkaCak | ( | const QString & | mkaCak | ) |
Definition at line 86 of file macsecsetting.cpp.
◆ setMkaCakFlags()
void NetworkManager::MacsecSetting::setMkaCakFlags | ( | Setting::SecretFlags | flags | ) |
Definition at line 184 of file macsecsetting.cpp.
◆ setMkaCkn()
void NetworkManager::MacsecSetting::setMkaCkn | ( | const QString & | mkaCkn | ) |
Definition at line 100 of file macsecsetting.cpp.
◆ setMode()
void NetworkManager::MacsecSetting::setMode | ( | Mode | mode | ) |
Definition at line 114 of file macsecsetting.cpp.
◆ setParent()
void NetworkManager::MacsecSetting::setParent | ( | const QString & | parent | ) |
Definition at line 128 of file macsecsetting.cpp.
◆ setPort()
void NetworkManager::MacsecSetting::setPort | ( | qint32 | port | ) |
Definition at line 142 of file macsecsetting.cpp.
◆ setSendSci()
void NetworkManager::MacsecSetting::setSendSci | ( | bool | sendSci | ) |
Definition at line 156 of file macsecsetting.cpp.
◆ setValidation()
void NetworkManager::MacsecSetting::setValidation | ( | Validation | validation | ) |
Definition at line 170 of file macsecsetting.cpp.
◆ toMap()
|
overridevirtual |
Must be reimplemented, default implementationd does nothing.
Reimplemented from NetworkManager::Setting.
Definition at line 266 of file macsecsetting.cpp.
◆ validation()
NetworkManager::MacsecSetting::Validation NetworkManager::MacsecSetting::validation | ( | ) | const |
Definition at line 177 of file macsecsetting.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 81 of file macsecsetting.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:47:28 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.