NetworkManager::WimaxDevice
#include <wimaxdevice.h>
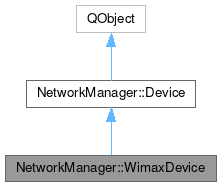
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< WimaxDevice > | Ptr |
![]() | |
typedef QFlags< Capability > | Capabilities |
enum | Capability { IsManageable = 0x1 , SupportsCarrierDetect = 0x2 } |
enum | Interfaceflag { None = NM_DEVICE_INTERFACE_FLAG_NONE , Up = NM_DEVICE_INTERFACE_FLAG_UP , LowerUp = NM_DEVICE_INTERFACE_FLAG_LOWER_UP , Carrier = NM_DEVICE_INTERFACE_FLAG_CARRIER } |
typedef QFlags< Interfaceflag > | Interfaceflags |
typedef QList< Ptr > | List |
enum | MeteredStatus { UnknownStatus = 0 , Yes = 1 , No = 2 , GuessYes = 3 , GuessNo = 4 } |
typedef QSharedPointer< Device > | Ptr |
enum | State { UnknownState = 0 , Unmanaged = 10 , Unavailable = 20 , Disconnected = 30 , Preparing = 40 , ConfiguringHardware = 50 , NeedAuth = 60 , ConfiguringIp = 70 , CheckingIp = 80 , WaitingForSecondaries = 90 , Activated = 100 , Deactivating = 110 , Failed = 120 } |
enum | StateChangeReason { UnknownReason = 0 , NoReason = 1 , NowManagedReason = 2 , NowUnmanagedReason = 3 , ConfigFailedReason = 4 , ConfigUnavailableReason = 5 , ConfigExpiredReason = 6 , NoSecretsReason = 7 , AuthSupplicantDisconnectReason = 8 , AuthSupplicantConfigFailedReason = 9 , AuthSupplicantFailedReason = 10 , AuthSupplicantTimeoutReason = 11 , PppStartFailedReason = 12 , PppDisconnectReason = 13 , PppFailedReason = 14 , DhcpStartFailedReason = 15 , DhcpErrorReason = 16 , DhcpFailedReason = 17 , SharedStartFailedReason = 18 , SharedFailedReason = 19 , AutoIpStartFailedReason = 20 , AutoIpErrorReason = 21 , AutoIpFailedReason = 22 , ModemBusyReason = 23 , ModemNoDialToneReason = 24 , ModemNoCarrierReason = 25 , ModemDialTimeoutReason = 26 , ModemDialFailedReason = 27 , ModemInitFailedReason = 28 , GsmApnSelectFailedReason = 29 , GsmNotSearchingReason = 30 , GsmRegistrationDeniedReason = 31 , GsmRegistrationTimeoutReason = 32 , GsmRegistrationFailedReason = 33 , GsmPinCheckFailedReason = 34 , FirmwareMissingReason = 35 , DeviceRemovedReason = 36 , SleepingReason = 37 , ConnectionRemovedReason = 38 , UserRequestedReason = 39 , CarrierReason = 40 , ConnectionAssumedReason = 41 , SupplicantAvailableReason = 42 , ModemNotFoundReason = 43 , BluetoothFailedReason = 44 , GsmSimNotInserted = 45 , GsmSimPinRequired = 46 , GsmSimPukRequired = 47 , GsmSimWrong = 48 , InfiniBandMode = 49 , DependencyFailed = 50 , Br2684Failed = 51 , ModemManagerUnavailable = 52 , SsidNotFound = 53 , SecondaryConnectionFailed = 54 , DcbFcoeFailed = 55 , TeamdControlFailed = 56 , ModemFailed = 57 , ModemAvailable = 58 , SimPinIncorrect = 59 , NewActivation = 60 , ParentChanged = 61 , ParentManagedChanged = 62 , Reserved = 65536 } |
enum | Type { UnknownType = NM_DEVICE_TYPE_UNKNOWN , Ethernet = NM_DEVICE_TYPE_ETHERNET , Wifi = NM_DEVICE_TYPE_WIFI , Unused1 = NM_DEVICE_TYPE_UNUSED1 , Unused2 = NM_DEVICE_TYPE_UNUSED2 , Bluetooth = NM_DEVICE_TYPE_BT , OlpcMesh = NM_DEVICE_TYPE_OLPC_MESH , Wimax = NM_DEVICE_TYPE_WIMAX , Modem = NM_DEVICE_TYPE_MODEM , InfiniBand = NM_DEVICE_TYPE_INFINIBAND , Bond = NM_DEVICE_TYPE_BOND , Vlan = NM_DEVICE_TYPE_VLAN , Adsl = NM_DEVICE_TYPE_ADSL , Bridge = NM_DEVICE_TYPE_BRIDGE , Generic = NM_DEVICE_TYPE_GENERIC , Team = NM_DEVICE_TYPE_TEAM , Gre , MacVlan , Tun , Veth , IpTunnel , VxLan , MacSec , Dummy , Ppp , OvsInterface , OvsPort , OvsBridge , Wpan , Lowpan , WireGuard , WifiP2P , VRF , Loopback } |
typedef QFlags< Type > | Types |
Public Member Functions | |
WimaxDevice (const QString &path, QObject *parent=nullptr) | |
~WimaxDevice () override | |
WimaxNsp::Ptr | activeNsp () const |
QString | bsid () const |
uint | centerFrequency () const |
int | cinr () const |
NetworkManager::WimaxNsp::Ptr | findNsp (const QString &uni) const |
QString | hardwareAddress () const |
QStringList | nsps () const |
int | rssi () const |
int | txPower () const |
Type | type () const override |
![]() | |
Device (const QString &path, QObject *parent=nullptr) | |
~Device () override | |
NetworkManager::ActiveConnection::Ptr | activeConnection () const |
template<class DevIface> | |
DevIface * | as () |
template<class DevIface> | |
const DevIface * | as () const |
bool | autoconnect () const |
Connection::List | availableConnections () |
Capabilities | capabilities () const |
QVariant | capabilitiesV () const |
QDBusPendingReply | deleteInterface () |
int | designSpeed () const |
DeviceStatistics::Ptr | deviceStatistics () const |
Dhcp4Config::Ptr | dhcp4Config () const |
Dhcp6Config::Ptr | dhcp6Config () const |
QDBusPendingReply | disconnectInterface () |
QString | driver () const |
QString | driverVersion () const |
bool | firmwareMissing () const |
QString | firmwareVersion () const |
Interfaceflags | interfaceFlags () const |
QString | interfaceName () const |
QString | ipInterfaceName () const |
QHostAddress | ipV4Address () const |
IpConfig | ipV4Config () const |
IpConfig | ipV6Config () const |
bool | isActive () const |
bool | isValid () const |
bool | managed () const |
MeteredStatus | metered () const |
uint | mtu () const |
bool | nmPluginMissing () const |
QString | physicalPortId () const |
QDBusPendingReply | reapplyConnection (const NMVariantMapMap &connection, qulonglong version_id, uint flags) |
void | setAutoconnect (bool autoconnect) |
State | state () const |
DeviceStateReason | stateReason () const |
QString | udi () const |
QString | uni () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
bool | autoconnect |
NetworkManager::DeviceStatistics::Ptr | deviceStatistics |
QString | driver |
QString | driverVersion |
bool | firmwareMissing |
QString | firmwareVersion |
QVariant | genericCapabilities |
Interfaceflags | InterfaceFlags |
QString | interfaceName |
QString | ipInterfaceName |
QHostAddress | ipV4Address |
bool | managed |
MeteredStatus | metered |
uint | mtu |
bool | nmPluginMissing |
State | state |
DeviceStateReason | stateReason |
QString | udi |
QString | uni |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
NETWORKMANAGERQT_NO_EXPORT | Device (DevicePrivate &dd, QObject *parent) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
DevicePrivate *const | d_ptr |
Detailed Description
Wimax network interface.
Definition at line 24 of file wimaxdevice.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> NetworkManager::WimaxDevice::List |
Definition at line 30 of file wimaxdevice.h.
◆ Ptr
Definition at line 29 of file wimaxdevice.h.
Constructor & Destructor Documentation
◆ WimaxDevice()
|
explicit |
Creates a new WimaxDevice object.
- Parameters
-
path the DBus path of the device
Definition at line 32 of file wimaxdevice.cpp.
◆ ~WimaxDevice()
|
override |
Destroys a WimaxDevice object.
Definition at line 46 of file wimaxdevice.cpp.
Member Function Documentation
◆ activeNsp()
NetworkManager::WimaxNsp::Ptr NetworkManager::WimaxDevice::activeNsp | ( | ) | const |
Identifier of the NSP this interface is currently associated with.
Definition at line 61 of file wimaxdevice.cpp.
◆ activeNspChanged
|
signal |
The active NSP changed.
◆ bitRateChanged
|
signal |
This signal is emitted when the bitrate of this network has changed.
- Parameters
-
bitrate the new bitrate value for this network
◆ bsid()
QString NetworkManager::WimaxDevice::bsid | ( | ) | const |
The ID of the serving base station as received from the network.
Definition at line 73 of file wimaxdevice.cpp.
◆ bsidChanged
|
signal |
The BSID changed.
◆ centerFrequency()
uint NetworkManager::WimaxDevice::centerFrequency | ( | ) | const |
Center frequency (in KHz) of the radio channel the device is using to communicate with the network when connected.
Definition at line 79 of file wimaxdevice.cpp.
◆ centerFrequencyChanged
|
signal |
The device changed its center frequency.
◆ cinr()
int NetworkManager::WimaxDevice::cinr | ( | ) | const |
CINR (Carrier to Interference + Noise Ratio) of the current radio link in dB.
Definition at line 85 of file wimaxdevice.cpp.
◆ cinrChanged
|
signal |
The device changed its signal/noise ratio.
◆ findNsp()
NetworkManager::WimaxNsp::Ptr NetworkManager::WimaxDevice::findNsp | ( | const QString & | uni | ) | const |
Finds NSP object given its Unique Network Identifier.
- Parameters
-
uni the identifier of the AP to find from this network interface
- Returns
- a valid WimaxNsp object if a network having the given UNI for this device is known to the system, 0 otherwise
Definition at line 103 of file wimaxdevice.cpp.
◆ hardwareAddress()
QString NetworkManager::WimaxDevice::hardwareAddress | ( | ) | const |
The hardware address currently used by the network interface.
Definition at line 67 of file wimaxdevice.cpp.
◆ hardwareAddressChanged
|
signal |
The device changed its hardware address.
◆ nspAppeared
|
signal |
A new NSP appeared.
◆ nspDisappeared
|
signal |
A wireless access point disappeared.
◆ nsps()
QStringList NetworkManager::WimaxDevice::nsps | ( | ) | const |
List of network service providers currently visible to the hardware.
Definition at line 55 of file wimaxdevice.cpp.
◆ rssi()
int NetworkManager::WimaxDevice::rssi | ( | ) | const |
RSSI of the current radio link in dBm.
This value indicates how strong the raw received RF signal from the base station is, but does not indicate the overall quality of the radio link.
Definition at line 91 of file wimaxdevice.cpp.
◆ rssiChanged
|
signal |
The device changed its RSSI.
◆ txPower()
int NetworkManager::WimaxDevice::txPower | ( | ) | const |
Average power of the last burst transmitted by the device, in units of 0.5 dBm.
i.e. a TxPower of -11 represents an actual device TX power of -5.5 dBm.
Definition at line 97 of file wimaxdevice.cpp.
◆ txPowerChanged
|
signal |
The device changed its TxPower.
◆ type()
|
overridevirtual |
Return the type.
Reimplemented from NetworkManager::Device.
Definition at line 50 of file wimaxdevice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:57:20 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.