IncidenceEditorNG::IncidenceEditor
#include <incidenceeditor-ng.h>
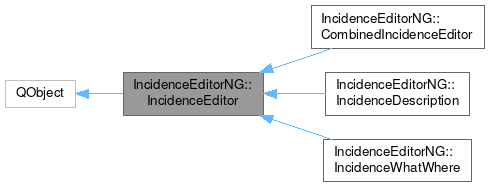
Signals | |
void | dirtyStatusChanged (bool isDirty) |
Public Slots | |
void | checkDirtyStatus () |
Public Member Functions | |
virtual void | focusInvalidField () |
template<typename IncidenceT > | |
QSharedPointer< IncidenceT > | incidence () const |
virtual bool | isDirty () const =0 |
virtual bool | isValid () const |
QString | lastErrorString () const |
virtual void | load (const Akonadi::Item &item) |
virtual void | load (const KCalendarCore::Incidence::Ptr &incidence)=0 |
virtual void | printDebugInfo () const |
virtual void | save (Akonadi::Item &item) |
virtual void | save (const KCalendarCore::Incidence::Ptr &incidence)=0 |
KCalendarCore::IncidenceBase::IncidenceType | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
IncidenceEditor (QObject *parent=nullptr) | |
template<typename IncidenceT > | |
QSharedPointer< IncidenceT > | incidence (const KCalendarCore::Incidence::Ptr &inc) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
QString | mLastErrorString |
KCalendarCore::Incidence::Ptr | mLoadedIncidence |
bool | mLoadingIncidence = false |
bool | mWasDirty = false |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
KCal Incidences are complicated objects.
The user interfaces to create/modify are therefore complex too. The IncedenceEditor class is a divide and conquer approach to this complexity. An IncidenceEditor is an editor for a specific part(s) of an Incidence.
Definition at line 22 of file incidenceeditor-ng.h.
Constructor & Destructor Documentation
◆ IncidenceEditor()
|
protected |
Only subclasses can instantiate IncidenceEditors.
Definition at line 14 of file incidenceeditor.cpp.
Member Function Documentation
◆ checkDirtyStatus
|
slot |
Checks if the dirty status has changed until last check and emits the dirtyStatusChanged signal if needed.
Definition at line 21 of file incidenceeditor.cpp.
◆ dirtyStatusChanged
|
signal |
Signals whether the dirty status of this editor has changed.
The new dirty status is passed as argument.
◆ focusInvalidField()
|
virtual |
Sets focus on the invalid field.
Reimplemented in IncidenceEditorNG::IncidenceWhatWhere.
Definition at line 50 of file incidenceeditor.cpp.
◆ incidence() [1/2]
|
inline |
Convenience method to get a pointer for a specific const Incidence Type.
Definition at line 73 of file incidenceeditor-ng.h.
◆ incidence() [2/2]
|
inlineprotected |
Definition at line 107 of file incidenceeditor-ng.h.
◆ isDirty()
|
pure virtual |
Returns whether or not the current values in the editor differ from the initial values.
Implemented in IncidenceEditorNG::CombinedIncidenceEditor, IncidenceEditorNG::IncidenceDescription, and IncidenceEditorNG::IncidenceWhatWhere.
◆ isValid()
|
virtual |
Returns whether or not the content of this editor is valid.
The default implementation returns always true.
Reimplemented in IncidenceEditorNG::CombinedIncidenceEditor, and IncidenceEditorNG::IncidenceWhatWhere.
Definition at line 39 of file incidenceeditor.cpp.
◆ lastErrorString()
|
nodiscard |
Returns the last error, which is set in isValid() on error, and cleared on success.
Definition at line 45 of file incidenceeditor.cpp.
◆ load() [1/2]
|
virtual |
This was introduced to replace categories with Akonadi::Tags.
Reimplemented in IncidenceEditorNG::CombinedIncidenceEditor.
Definition at line 68 of file incidenceeditor.cpp.
◆ load() [2/2]
|
pure virtual |
Load the values of.
- Parameters
-
incidence into the editor widgets. The passed incidence is kept for comparing with the current values of the editor.
Implemented in IncidenceEditorNG::CombinedIncidenceEditor, IncidenceEditorNG::IncidenceDescription, and IncidenceEditorNG::IncidenceWhatWhere.
◆ printDebugInfo()
|
virtual |
Re-implement this and print important member values and widget enabled/disabled states that could have lead to isDirty() returning true when the user didn't do any interaction with the editor.
This method is called in CombinedIncidenceEditor before crashing due to assert( !editor->isDirty() )
Reimplemented in IncidenceEditorNG::IncidenceDescription.
Definition at line 63 of file incidenceeditor.cpp.
◆ save() [1/2]
|
virtual |
This was introduced to replace categories with Akonadi::Tags.
Reimplemented in IncidenceEditorNG::CombinedIncidenceEditor.
Definition at line 73 of file incidenceeditor.cpp.
◆ save() [2/2]
|
pure virtual |
Store the current values of the editor into.
- Parameters
-
incidence .
Implemented in IncidenceEditorNG::CombinedIncidenceEditor, IncidenceEditorNG::IncidenceDescription, and IncidenceEditorNG::IncidenceWhatWhere.
◆ type()
|
nodiscard |
Returns the type of the Incidence that is currently loaded.
Definition at line 54 of file incidenceeditor.cpp.
Member Data Documentation
◆ mLastErrorString
|
mutableprotected |
Definition at line 114 of file incidenceeditor-ng.h.
◆ mLoadedIncidence
|
protected |
Definition at line 113 of file incidenceeditor-ng.h.
◆ mLoadingIncidence
|
protected |
Definition at line 116 of file incidenceeditor-ng.h.
◆ mWasDirty
|
protected |
Definition at line 115 of file incidenceeditor-ng.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:58:15 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.