MailCommon::Kernel
#include <mailkernel.h>
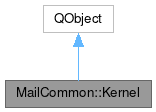
Signals | |
void | requestConfigSync () |
void | requestSystemTrayUpdate () |
Static Public Member Functions | |
static bool | folderIsInbox (const Akonadi::Collection &) |
static QMap< QString, Akonadi::Collection::Id > | pop3ResourceTargetCollection () |
static Kernel * | self () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Deals with common mail application related operations.
The required interfaces MUST be registered before using it! Be careful when using in multi-threaded applications, as Kernel is a QObject singleton, created in the main thread, thus event handling for Kernel::self() will happen in the main thread.
Definition at line 41 of file mailkernel.h.
Constructor & Destructor Documentation
◆ ~Kernel()
|
override |
Definition at line 58 of file mailkernel.cpp.
Member Function Documentation
◆ collectionFromId()
|
nodiscard |
Returns the collection associated with the given id
, or an invalid collection if not found.
The EntityTreeModel of the kernel is searched for the collection. Since the ETM is loaded async, this method will not find the collection right after startup, when the ETM is not yet fully loaded.
Definition at line 111 of file mailkernel.cpp.
◆ draftsCollectionFolder()
|
nodiscard |
Definition at line 136 of file mailkernel.cpp.
◆ emergencyExit()
void MailCommon::Kernel::emergencyExit | ( | const QString & | reason | ) |
Definition at line 226 of file mailkernel.cpp.
◆ filterIf()
IFilter * MailCommon::Kernel::filterIf | ( | ) | const |
Definition at line 100 of file mailkernel.cpp.
◆ folderIsDraftOrOutbox()
|
nodiscard |
Returns true if the folder is either the outbox or one of the drafts-folders.
Definition at line 251 of file mailkernel.cpp.
◆ folderIsDrafts()
|
nodiscard |
Definition at line 260 of file mailkernel.cpp.
◆ folderIsInbox()
|
static |
Definition at line 350 of file mailkernel.cpp.
◆ folderIsSentMailFolder()
|
nodiscard |
Returns true if the folder is one of the sent-mail folders.
Definition at line 329 of file mailkernel.cpp.
◆ folderIsTemplates()
|
nodiscard |
Definition at line 282 of file mailkernel.cpp.
◆ folderIsTrash()
|
nodiscard |
Returns true if the folder is a trash folder.
When calling this too early (before the SpecialMailCollectionsDiscoveryJob from initFolders finishes), it will say false erroneously. However you can connect to SpecialMailCollections::collectionsChanged to react on dynamic changes and call this again.
Definition at line 313 of file mailkernel.cpp.
◆ imapResourceManager()
PimCommon::ImapResourceCapabilitiesManager * MailCommon::Kernel::imapResourceManager | ( | ) | const |
Definition at line 106 of file mailkernel.cpp.
◆ inboxCollectionFolder()
|
nodiscard |
Definition at line 121 of file mailkernel.cpp.
◆ initFolders()
void MailCommon::Kernel::initFolders | ( | ) |
Definition at line 158 of file mailkernel.cpp.
◆ isMainFolderCollection()
|
nodiscard |
Returns true if this folder is the inbox on the local disk.
Definition at line 152 of file mailkernel.cpp.
◆ isSystemFolderCollection()
|
nodiscard |
Definition at line 146 of file mailkernel.cpp.
◆ kernelIf()
IKernel * MailCommon::Kernel::kernelIf | ( | ) | const |
Definition at line 78 of file mailkernel.cpp.
◆ kernelIsRegistered()
|
nodiscard |
Definition at line 73 of file mailkernel.cpp.
◆ outboxCollectionFolder()
|
nodiscard |
Definition at line 126 of file mailkernel.cpp.
◆ pop3ResourceTargetCollection()
|
static |
Definition at line 369 of file mailkernel.cpp.
◆ registerFilterIf()
void MailCommon::Kernel::registerFilterIf | ( | IFilter * | filterIf | ) |
Registers the interface dealing with mail settings.
This function MUST be called with a valid interface pointer, before any Kernel::self() method is used. The pointer ownership will not be transferred to Kernel.
Definition at line 95 of file mailkernel.cpp.
◆ registerKernelIf()
void MailCommon::Kernel::registerKernelIf | ( | IKernel * | kernelIf | ) |
Registers the interface dealing with main mail functionality.
This function MUST be called with a valid interface pointer, before any Kernel::self() method is used. The pointer ownership will not be transferred to Kernel.
Definition at line 68 of file mailkernel.cpp.
◆ registerSettingsIf()
void MailCommon::Kernel::registerSettingsIf | ( | ISettings * | settingsIf | ) |
Registers the interface dealing with mail settings.
This function MUST be called with a valid interface pointer, before any Kernel::self() method is used. The pointer ownership will not be transferred to Kernel.
Definition at line 84 of file mailkernel.cpp.
◆ self()
|
static |
Definition at line 63 of file mailkernel.cpp.
◆ sentCollectionFolder()
|
nodiscard |
Definition at line 131 of file mailkernel.cpp.
◆ settingsIf()
ISettings * MailCommon::Kernel::settingsIf | ( | ) | const |
Definition at line 89 of file mailkernel.cpp.
◆ templatesCollectionFolder()
|
nodiscard |
Definition at line 141 of file mailkernel.cpp.
◆ trashCollectionFolder()
|
nodiscard |
Definition at line 116 of file mailkernel.cpp.
◆ trashCollectionFromResource()
|
nodiscard |
Returns the trash folder for the resource which col
belongs to.
When calling this too early (before the SpecialMailCollectionsDiscoveryJob from initFolders finishes), it will return an invalid collection erroneously. However you can connect to SpecialMailCollections::collectionsChanged to react on dynamic changes and call this again.
Definition at line 303 of file mailkernel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:09:01 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.