MailCommon::SearchPattern
#include <searchpattern.h>
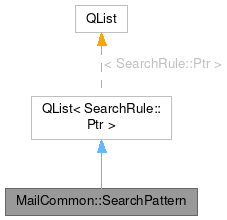
Public Types | |
enum | Operator { OpAnd , OpOr , OpAll } |
enum | SparqlQueryError { NoError = 0 , MissingCheck , FolderEmptyOrNotIndexed , EmptyResult , NotEnoughCharacters } |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | const_reverse_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | parameter_type |
typedef | pointer |
typedef | reference |
typedef | reverse_iterator |
typedef | rvalue_ref |
typedef | size_type |
typedef | value_type |
Public Member Functions | |
SearchPattern () | |
SearchPattern (const KConfigGroup &config) | |
~SearchPattern () | |
SparqlQueryError | asAkonadiQuery (Akonadi::SearchQuery &) const |
QString | asString () const |
void | deserialize (const QByteArray &) |
void | generateSieveScript (QStringList &requiresModules, QString &code) |
bool | matches (const Akonadi::Item &item, bool ignoreBody=false) const |
QString | name () const |
SearchPattern::Operator | op () const |
QDataStream & | operator<< (QDataStream &s) |
const SearchPattern & | operator= (const SearchPattern &aPattern) |
QDataStream & | operator>> (QDataStream &s) const |
QString | purify (bool removeAction=true) |
void | readConfig (const KConfigGroup &config) |
SearchRule::RequiredPart | requiredPart () const |
QByteArray | serialize () const |
void | setName (const QString &newName) |
void | setOp (SearchPattern::Operator aOp) |
void | writeConfig (KConfigGroup &config) const |
![]() | |
QList (const QList< T > &other) | |
QList (InputIterator first, InputIterator last) | |
QList (QList< T > &&other) | |
QList (qsizetype size) | |
QList (qsizetype size, parameter_type value) | |
QList (std::initializer_list< T > args) | |
void | append (const QList< T > &value) |
void | append (parameter_type value) |
void | append (QList< T > &&value) |
void | append (rvalue_ref value) |
const_reference | at (qsizetype i) const const |
reference | back () |
const_reference | back () const const |
iterator | begin () |
const_iterator | begin () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_pointer | constData () const const |
const_iterator | constEnd () const const |
const T & | constFirst () const const |
const T & | constLast () const const |
bool | contains (const AT &value) const const |
qsizetype | count () const const |
qsizetype | count (const AT &value) const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crend () const const |
pointer | data () |
const_pointer | data () const const |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplaceBack (Args &&... args) |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
bool | endsWith (parameter_type value) const const |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator pos) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
QList< T > & | fill (parameter_type value, qsizetype size) |
T & | first () |
const T & | first () const const |
QList< T > | first (qsizetype n) const const |
reference | front () |
const_reference | front () const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, rvalue_ref value) |
bool | isEmpty () const const |
T & | last () |
const T & | last () const const |
QList< T > | last (qsizetype n) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | length () const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
void | move (qsizetype from, qsizetype to) |
bool | operator!= (const QList< T > &other) const const |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (rvalue_ref value) |
bool | operator< (const QList< T > &other) const const |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (parameter_type value) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (rvalue_ref value) |
bool | operator<= (const QList< T > &other) const const |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (std::initializer_list< T > args) |
bool | operator== (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
reference | operator[] (qsizetype i) |
const_reference | operator[] (qsizetype i) const const |
void | pop_back () |
void | pop_front () |
void | prepend (parameter_type value) |
void | prepend (rvalue_ref value) |
void | push_back (parameter_type value) |
void | push_back (rvalue_ref value) |
void | push_front (parameter_type value) |
void | push_front (rvalue_ref value) |
size_t | qHash (const QList< T > &key, size_t seed) |
reverse_iterator | rbegin () |
const_reverse_iterator | rbegin () const const |
void | remove (qsizetype i, qsizetype n) |
qsizetype | removeAll (const AT &t) |
void | removeAt (qsizetype i) |
void | removeFirst () |
qsizetype | removeIf (Predicate pred) |
void | removeLast () |
bool | removeOne (const AT &t) |
reverse_iterator | rend () |
const_reverse_iterator | rend () const const |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, rvalue_ref value) |
void | reserve (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size, parameter_type c) |
void | shrink_to_fit () |
qsizetype | size () const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
void | squeeze () |
bool | startsWith (parameter_type value) const const |
void | swap (QList< T > &other) |
void | swapItemsAt (qsizetype i, qsizetype j) |
T | takeAt (qsizetype i) |
value_type | takeFirst () |
value_type | takeLast () |
QList< T > | toList () const const |
QList< T > | toVector () const const |
T | value (qsizetype i) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
Static Public Member Functions | |
static int | filterRulesMaximumSize () |
![]() | |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
Detailed Description
This class is an abstraction of a search over messages.
It is intended to be used inside a KFilter (which adds KFilterAction's), as well as in KMSearch. It can read and write itself into a KConfig group and there is a constructor, mainly used by KMFilter to initialize from a preset KConfig-Group.
From a class hierarchy point of view, it is a QPtrList of SearchRule's that adds the boolean operators (see Operator) 'and' and 'or' that connect the rules logically, and has a name under which it could be stored in the config file.
As a QPtrList with autoDelete enabled, it assumes that it is the central repository for the rules it contains. So if you want to reuse a rule in another pattern, make a deep copy of that rule.
An abstraction of a search over messages.
Definition at line 53 of file searchpattern.h.
Member Enumeration Documentation
◆ Operator
Boolean operators that connect the return values of the individual rules.
A pattern with OpAnd
will match iff all it's rules match, whereas a pattern with OpOr
will match if any of it's rules matches.
Definition at line 62 of file searchpattern.h.
◆ SparqlQueryError
enum MailCommon::SearchPattern::SparqlQueryError |
Definition at line 68 of file searchpattern.h.
Constructor & Destructor Documentation
◆ SearchPattern() [1/2]
SearchPattern::SearchPattern | ( | ) |
Constructor which provides a pattern with minimal, but sufficient initialization.
Unmodified, such a pattern will fail to match any KMime::Message. You can query for such an empty rule by using isEmpty, which is inherited from QPtrList.
Definition at line 32 of file searchpattern.cpp.
◆ SearchPattern() [2/2]
|
explicit |
Constructor that initializes from a given KConfig group, if given.
This feature is mainly (solely?) used in KMFilter, as we don't allow to store search patterns in the config (yet).
Definition at line 38 of file searchpattern.cpp.
◆ ~SearchPattern()
|
default |
Destructor.
Deletes all stored rules!
Member Function Documentation
◆ asAkonadiQuery()
SearchPattern::SparqlQueryError SearchPattern::asAkonadiQuery | ( | Akonadi::SearchQuery & | query | ) | const |
Returns the pattern as akonadi query.
Definition at line 262 of file searchpattern.cpp.
◆ asString()
|
nodiscard |
◆ deserialize()
void SearchPattern::deserialize | ( | const QByteArray & | str | ) |
Constructs the pattern from a byte array serialization.
Definition at line 319 of file searchpattern.cpp.
◆ filterRulesMaximumSize()
|
static |
Definition at line 226 of file searchpattern.cpp.
◆ generateSieveScript()
void SearchPattern::generateSieveScript | ( | QStringList & | requiresModules, |
QString & | code ) |
Definition at line 364 of file searchpattern.cpp.
◆ matches()
bool SearchPattern::matches | ( | const Akonadi::Item & | item, |
bool | ignoreBody = false ) const |
The central function of this class.
Tries to match the set of rules against a KMime::Message. It's virtual to allow derived classes with added rules to reimplement it, yet reimplemented methods should and (&&) the result of this function with their own result or else most functionality is lacking, or has to be reimplemented, since the rules are private to this class.
- Returns
- true if the match was successful, false otherwise.
Definition at line 46 of file searchpattern.cpp.
◆ name()
|
inlinenodiscard |
Returns the name of the search pattern.
Definition at line 144 of file searchpattern.h.
◆ op()
|
inlinenodiscard |
Returns the filter operator.
Definition at line 161 of file searchpattern.h.
◆ operator<<()
QDataStream & SearchPattern::operator<< | ( | QDataStream & | s | ) |
Definition at line 345 of file searchpattern.cpp.
◆ operator=()
const SearchPattern & SearchPattern::operator= | ( | const SearchPattern & | aPattern | ) |
Overloaded assignment operator.
Makes a deep copy.
Definition at line 292 of file searchpattern.cpp.
◆ operator>>()
QDataStream & SearchPattern::operator>> | ( | QDataStream & | s | ) | const |
Definition at line 325 of file searchpattern.cpp.
◆ purify()
|
nodiscard |
Removes all empty rules from the list.
You should call this method whenever the user had had control of the rules outside of this class. (e.g. after editing it with SearchPatternEdit).
Definition at line 98 of file searchpattern.cpp.
◆ readConfig()
void SearchPattern::readConfig | ( | const KConfigGroup & | config | ) |
Reads a search pattern from a KConfigGroup.
If it does not find a valid saerch pattern in the preset group, initializes the pattern as if it were constructed using the default constructor.
For backwards compatibility with previous versions of KMail, it checks for old-style filter rules (e.g. using OpIgnore
) in config
und converts them to the new format on writeConfig.
Derived classes reimplementing readConfig() should also call this method, or else the rules will not be loaded.
Definition at line 121 of file searchpattern.cpp.
◆ requiredPart()
SearchRule::RequiredPart SearchPattern::requiredPart | ( | ) | const |
Returns the required part from the item that is needed for the search to operate.
Definition at line 86 of file searchpattern.cpp.
◆ serialize()
|
nodiscard |
Writes the pattern into a byte array for persistence purposes.
Definition at line 311 of file searchpattern.cpp.
◆ setName()
|
inline |
Sets the name of the search pattern.
KMFilter uses this to store it's own name, too.
Definition at line 153 of file searchpattern.h.
◆ setOp()
|
inline |
Sets the filter operator.
Definition at line 169 of file searchpattern.h.
◆ writeConfig()
void SearchPattern::writeConfig | ( | KConfigGroup & | config | ) | const |
Writes itself into config
.
Tries to delete old-style keys by overwriting them with QString().
Derived classes reimplementing writeConfig() should also call this method, or else the rules will not be stored.
Definition at line 194 of file searchpattern.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:49:06 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.