KChart::AbstractArea
#include <KChartAbstractArea.h>
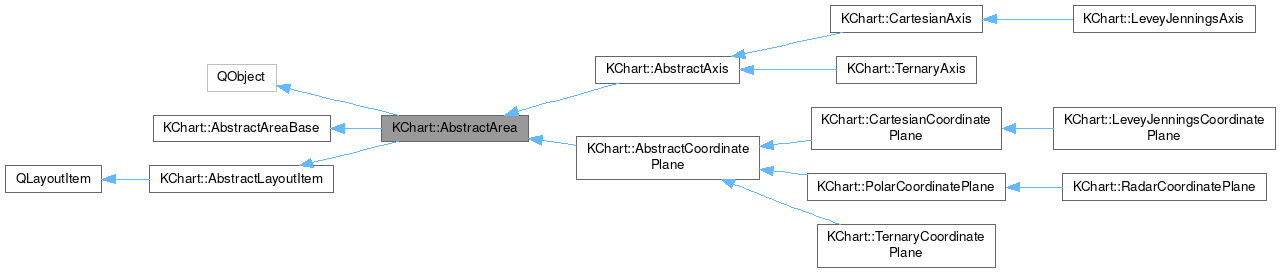
Signals | |
void | positionChanged (KChart::AbstractArea *) |
Public Member Functions | |
virtual int | bottomOverlap (bool doNotRecalculate=false) const |
virtual int | leftOverlap (bool doNotRecalculate=false) const |
void | paintAll (QPainter &painter) override |
virtual void | paintIntoRect (QPainter &painter, const QRect &rect) |
virtual int | rightOverlap (bool doNotRecalculate=false) const |
virtual int | topOverlap (bool doNotRecalculate=false) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
void | alignToReferencePoint (const RelativePosition &position) |
BackgroundAttributes | backgroundAttributes () const |
bool | compare (const AbstractAreaBase *other) const |
FrameAttributes | frameAttributes () const |
void | getFrameLeadings (int &left, int &top, int &right, int &bottom) const |
virtual void | paintBackground (QPainter &painter, const QRect &rectangle) |
virtual void | paintFrame (QPainter &painter, const QRect &rectangle) |
void | setBackgroundAttributes (const BackgroundAttributes &a) |
void | setFrameAttributes (const FrameAttributes &a) |
![]() | |
AbstractLayoutItem (Qt::Alignment itemAlignment=Qt::Alignment()) | |
virtual void | paint (QPainter *)=0 |
virtual void | paintCtx (PaintContext *context) |
QLayout * | parentLayout () |
void | removeFromParentLayout () |
void | setParentLayout (QLayout *lay) |
virtual void | setParentWidget (QWidget *widget) |
virtual void | sizeHintChanged () const |
![]() | |
QLayoutItem (Qt::Alignment alignment) | |
Qt::Alignment | alignment () const const |
virtual QSizePolicy::ControlTypes | controlTypes () const const |
virtual Qt::Orientations | expandingDirections () const const=0 |
virtual QRect | geometry () const const=0 |
virtual bool | hasHeightForWidth () const const |
virtual int | heightForWidth (int) const const |
virtual void | invalidate () |
virtual bool | isEmpty () const const=0 |
virtual QLayout * | layout () |
virtual QSize | maximumSize () const const=0 |
virtual int | minimumHeightForWidth (int w) const const |
virtual QSize | minimumSize () const const=0 |
void | setAlignment (Qt::Alignment alignment) |
virtual void | setGeometry (const QRect &r)=0 |
virtual QSize | sizeHint () const const=0 |
virtual QSpacerItem * | spacerItem () |
virtual QWidget * | widget () const const |
Protected Member Functions | |
QRect | areaGeometry () const override |
void | positionHasChanged () override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QRect | innerRect () const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static void | paintBackgroundAttributes (QPainter &painter, const QRect &rectangle, const KChart::BackgroundAttributes &attributes) |
static void | paintFrameAttributes (QPainter &painter, const QRect &rectangle, const KChart::FrameAttributes &attributes) |
![]() | |
typedef | QObjectList |
![]() | |
QWidget * | mParent |
QLayout * | mParentLayout |
Detailed Description
An area in the chart with a background, a frame, etc.
AbstractArea is the base class for all non-widget chart elements that have a set of background attributes and frame attributes, such as coordinate planes or axes.
- Note
- This class inherits from AbstractAreaBase, AbstractLayoutItem, QObject. The reason for this triple inheritance is that neither AbstractAreaBase nor AbstractLayoutItem are QObject.
Definition at line 33 of file KChartAbstractArea.h.
Constructor & Destructor Documentation
◆ ~AbstractArea()
|
override |
Definition at line 45 of file KChartAbstractArea.cpp.
◆ AbstractArea()
|
protected |
Definition at line 37 of file KChartAbstractArea.cpp.
Member Function Documentation
◆ areaGeometry()
|
overrideprotectedvirtual |
This internal method is used by AbstractArea and AbstractAreaWidget to find out the real widget size.
- See also
- AbstractArea, AbstractAreaWidget
Implements KChart::AbstractAreaBase.
Definition at line 137 of file KChartAbstractArea.cpp.
◆ bottomOverlap()
|
virtual |
This is called at layout time by KChart:AutoSpacerLayoutItem::sizeHint().
The method triggers AbstractArea::sizeHint() to find out the amount of overlap at the bottom edge of the area.
- Note
- The default implementation is not using any caching, it might make sense to implement a more sophisticated solution for derived classes that have complex work to do in sizeHint(). All we have here is a primitive flag to be set by the caller if it is sure that no sizeHint() needs to be called.
Definition at line 84 of file KChartAbstractArea.cpp.
◆ leftOverlap()
|
virtual |
This is called at layout time by KChart::AutoSpacerLayoutItem::sizeHint().
The method triggers AbstractArea::sizeHint() to find out the amount of overlap at the left edge of the area.
- Note
- The default implementation is not using any caching, it might make sense to implement a more sophisticated solution for derived classes that have complex work to do in sizeHint(). All we have here is a primitive flag to be set by the caller if it is sure that no sizeHint() needs to be called.
Definition at line 60 of file KChartAbstractArea.cpp.
◆ paintAll()
|
overridevirtual |
Call paintAll, if you want the background and the frame to be drawn before the normal paint() is invoked automatically.
Reimplemented from KChart::AbstractLayoutItem.
Reimplemented in KChart::TernaryAxis.
Definition at line 106 of file KChartAbstractArea.cpp.
◆ paintIntoRect()
Draws the background and frame, then calls paint().
In most cases there is no need to overwrite this method in a derived class, but you would overwrite AbstractLayoutItem::paint() instead.
Definition at line 94 of file KChartAbstractArea.cpp.
◆ positionHasChanged()
|
overrideprotectedvirtual |
This internal method can be overwritten by derived classes, if they want to emit a signal (or perform other actions, resp.) when the Position of the area has been changed. The default implementation does nothing.
Reimplemented from KChart::AbstractAreaBase.
Definition at line 142 of file KChartAbstractArea.cpp.
◆ rightOverlap()
|
virtual |
This is called at layout time by KChart::AutoSpacerLayoutItem::sizeHint().
The method triggers AbstractArea::sizeHint() to find out the amount of overlap at the right edge of the area.
- Note
- The default implementation is not using any caching, it might make sense to implement a more sophisticated solution for derived classes that have complex work to do in sizeHint(). All we have here is a primitive flag to be set by the caller if it is sure that no sizeHint() needs to be called.
Definition at line 68 of file KChartAbstractArea.cpp.
◆ topOverlap()
|
virtual |
This is called at layout time by KChart::AutoSpacerLayoutItem::sizeHint().
The method triggers AbstractArea::sizeHint() to find out the amount of overlap at the top edge of the area.
- Note
- The default implementation is not using any caching, it might make sense to implement a more sophisticated solution for derived classes that have complex work to do in sizeHint(). All we have here is a primitive flag to be set by the caller if it is sure that no sizeHint() needs to be called.
Definition at line 76 of file KChartAbstractArea.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:56:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.