KChart::Legend
#include <KChartLegend.h>
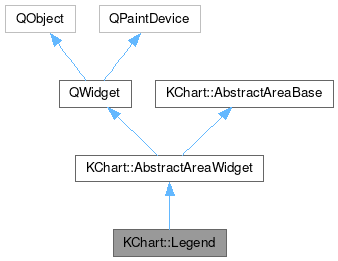
Public Types | |
enum | LegendStyle { MarkersOnly = 0 , LinesOnly = 1 , MarkersAndLines = 2 } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | destroyedLegend (KChart::Legend *) |
void | propertiesChanged () |
![]() | |
void | positionChanged (KChart::AbstractAreaWidget *) |
Public Member Functions | |
Legend (KChart::AbstractDiagram *diagram, QWidget *parent=nullptr) | |
Legend (QWidget *parent=nullptr) | |
void | addDiagram (KChart::AbstractDiagram *newDiagram) |
Qt::Alignment | alignment () const |
QBrush | brush (uint dataset) const |
const QMap< uint, QBrush > | brushes () const |
virtual Legend * | clone () const |
bool | compare (const Legend *other) const |
ConstDiagramList | constDiagrams () const |
uint | datasetCount () const |
bool | datasetIsHidden (uint dataset) const |
uint | dataSetOffset (KChart::AbstractDiagram *diagram) |
KChart::AbstractDiagram * | diagram () const |
DiagramList | diagrams () const |
const RelativePosition | floatingPosition () const |
void | forceRebuild () override |
bool | hasHeightForWidth () const override |
int | heightForWidth (int width) const override |
const QList< uint > | hiddenDatasets () const |
LegendStyle | legendStyle () const |
Qt::Alignment | legendSymbolAlignment () const |
const QMap< uint, MarkerAttributes > | markerAttributes () const |
MarkerAttributes | markerAttributes (uint dataset) const |
QSize | minimumSizeHint () const override |
void | needSizeHint () override |
Qt::Orientation | orientation () const |
virtual void | paint (QPainter *painter) override |
void | paint (QPainter *painter, const QRect &rect) |
QPen | pen (uint dataset) const |
const QMap< uint, QPen > | pens () const |
Position | position () const |
const QWidget * | referenceArea () const |
void | removeDiagram (KChart::AbstractDiagram *oldDiagram) |
void | removeDiagrams () |
void | replaceDiagram (KChart::AbstractDiagram *newDiagram, KChart::AbstractDiagram *oldDiagram=nullptr) |
void | resetTexts () |
void | resizeEvent (QResizeEvent *event) override |
void | resizeLayout (const QSize &size) override |
void | setAlignment (Qt::Alignment) |
void | setBrush (uint dataset, const QBrush &brush) |
void | setBrushesFromDiagram (KChart::AbstractDiagram *diagram) |
void | setColor (uint dataset, const QColor &color) |
void | setDatasetHidden (uint dataset, bool hidden) |
void | setDefaultColors () |
void | setDiagram (KChart::AbstractDiagram *newDiagram) |
void | setFloatingPosition (const RelativePosition &relativePosition) |
void | setHiddenDatasets (const QList< uint > hiddenDatasets) |
void | setLegendStyle (LegendStyle style) |
void | setLegendSymbolAlignment (Qt::Alignment) |
void | setMarkerAttributes (uint dataset, const MarkerAttributes &) |
void | setOrientation (Qt::Orientation orientation) |
void | setPen (uint dataset, const QPen &pen) |
void | setPosition (Position position) |
void | setRainbowColors () |
void | setReferenceArea (const QWidget *area) |
void | setShowLines (bool legendShowLines) |
void | setSortOrder (Qt::SortOrder order) |
void | setSpacing (uint space) |
void | setSubduedColors (bool ordered=false) |
void | setText (uint dataset, const QString &text) |
void | setTextAlignment (Qt::Alignment) |
void | setTextAttributes (const TextAttributes &a) |
void | setTitleText (const QString &text) |
void | setTitleTextAttributes (const TextAttributes &a) |
void | setUseAutomaticMarkerSize (bool useAutomaticMarkerSize) |
void | setVisible (bool visible) override |
bool | showLines () const |
QSize | sizeHint () const override |
Qt::SortOrder | sortOrder () const |
uint | spacing () const |
QString | text (uint dataset) const |
Qt::Alignment | textAlignment () const |
TextAttributes | textAttributes () const |
const QMap< uint, QString > | texts () const |
QString | titleText () const |
TextAttributes | titleTextAttributes () const |
bool | useAutomaticMarkerSize () const |
![]() | |
AbstractAreaWidget (QWidget *parent=nullptr) | |
void | paintAll (QPainter &painter) |
void | paintEvent (QPaintEvent *event) override |
virtual void | paintIntoRect (QPainter &painter, const QRect &rect) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
![]() | |
void | alignToReferencePoint (const RelativePosition &position) |
BackgroundAttributes | backgroundAttributes () const |
bool | compare (const AbstractAreaBase *other) const |
FrameAttributes | frameAttributes () const |
void | getFrameLeadings (int &left, int &top, int &right, int &bottom) const |
virtual void | paintBackground (QPainter &painter, const QRect &rectangle) |
virtual void | paintFrame (QPainter &painter, const QRect &rectangle) |
void | setBackgroundAttributes (const BackgroundAttributes &a) |
void | setFrameAttributes (const FrameAttributes &a) |
Detailed Description
Legend defines the interface for the legend drawing class.
Legend is the class for drawing legends for all kinds of diagrams ("chart types").
Legend is drawn on chart level, not per diagram, but you can have more than one legend per chart, using KChart::Chart::addLegend().
- Note
- Legend is different from all other classes of KChart, since it can be displayed outside of the Chart's area. If you want to, you can embed the legend into your own widget, or into another part of a bigger layout, into which you might have inserted the Chart.
On the other hand, please note that you MUST call Chart::addLegend to get your legend positioned into the correct place of your chart - if you want to have the legend shown inside of the chart (that's probably true for most cases).
Definition at line 41 of file KChartLegend.h.
Member Enumeration Documentation
◆ LegendStyle
enum KChart::Legend::LegendStyle |
Definition at line 54 of file KChartLegend.h.
Constructor & Destructor Documentation
◆ Legend()
|
explicit |
Definition at line 66 of file KChartLegend.cpp.
Member Function Documentation
◆ addDiagram()
void Legend::addDiagram | ( | KChart::AbstractDiagram * | newDiagram | ) |
Add the given diagram to the legend.
- Parameters
-
newDiagram The diagram to add.
- See also
- diagram, diagrams, removeDiagram, removeDiagrams, replaceDiagram, setDiagram
Definition at line 325 of file KChartLegend.cpp.
◆ alignment()
Qt::Alignment Legend::alignment | ( | ) | const |
Returns the alignment of a non-floating legend.
- See also
- setAlignment
Definition at line 480 of file KChartLegend.cpp.
◆ brush()
QBrush Legend::brush | ( | uint | dataset | ) | const |
Definition at line 634 of file KChartLegend.cpp.
◆ brushes()
Definition at line 643 of file KChartLegend.cpp.
◆ clone()
|
virtual |
Creates an exact copy of this legend.
Definition at line 182 of file KChartLegend.cpp.
◆ compare()
bool Legend::compare | ( | const Legend * | other | ) | const |
Returns true if both legends have the same settings.
Definition at line 197 of file KChartLegend.cpp.
◆ constDiagrams()
ConstDiagramList Legend::constDiagrams | ( | ) | const |
- Returns
- The list of diagrams associated with this legend.
Definition at line 316 of file KChartLegend.cpp.
◆ datasetCount()
uint Legend::datasetCount | ( | ) | const |
Definition at line 272 of file KChartLegend.cpp.
◆ datasetIsHidden()
bool Legend::datasetIsHidden | ( | uint | dataset | ) | const |
Definition at line 1262 of file KChartLegend.cpp.
◆ dataSetOffset()
uint Legend::dataSetOffset | ( | KChart::AbstractDiagram * | diagram | ) |
Returns the offset of the first dataset of diagram
.
Definition at line 408 of file KChartLegend.cpp.
◆ diagram()
AbstractDiagram * Legend::diagram | ( | ) | const |
The first diagram of the legend or 0 if there was none added to the legend.
- Returns
- The first diagram of the legend or 0.
- See also
- diagrams, addDiagram, removeDiagram, removeDiagrams, replaceDiagram, setDiagram
Definition at line 299 of file KChartLegend.cpp.
◆ diagrams()
DiagramList Legend::diagrams | ( | ) | const |
The list of all diagrams associated with the legend.
- Returns
- The list of all diagrams associated with the legend.
- See also
- diagram, addDiagram, removeDiagram, removeDiagrams, replaceDiagram, setDiagram
Definition at line 307 of file KChartLegend.cpp.
◆ floatingPosition()
const RelativePosition Legend::floatingPosition | ( | ) | const |
Returns the position of a floating legend.
- See also
- setFloatingPosition
Definition at line 522 of file KChartLegend.cpp.
◆ forceRebuild()
|
overridevirtual |
Call this to trigger an unconditional re-building of the widget's internals.
Reimplemented from KChart::AbstractAreaWidget.
Definition at line 760 of file KChartLegend.cpp.
◆ hasHeightForWidth()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 1179 of file KChartLegend.cpp.
◆ heightForWidth()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 1187 of file KChartLegend.cpp.
◆ hiddenDatasets()
const QList< uint > Legend::hiddenDatasets | ( | ) | const |
Definition at line 1248 of file KChartLegend.cpp.
◆ legendStyle()
Legend::LegendStyle Legend::legendStyle | ( | ) | const |
Definition at line 177 of file KChartLegend.cpp.
◆ legendSymbolAlignment()
Qt::Alignment Legend::legendSymbolAlignment | ( | ) | const |
Returns the alignment used while drawing legend symbol(alignment of Legend::LinesOnly) within the legend.
- See also
- setLegendSymbolAlignment()
Definition at line 508 of file KChartLegend.cpp.
◆ markerAttributes() [1/2]
const QMap< uint, MarkerAttributes > Legend::markerAttributes | ( | ) | const |
Definition at line 712 of file KChartLegend.cpp.
◆ markerAttributes() [2/2]
MarkerAttributes Legend::markerAttributes | ( | uint | dataset | ) | const |
Definition at line 701 of file KChartLegend.cpp.
◆ minimumSizeHint()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 123 of file KChartLegend.cpp.
◆ needSizeHint()
|
overridevirtual |
Call this to trigger an conditional re-building of the widget's internals.
e.g. AbstractAreaWidget call this, before calling layout()->setGeometry()
Reimplemented from KChart::AbstractAreaWidget.
Definition at line 141 of file KChartLegend.cpp.
◆ orientation()
Qt::Orientation Legend::orientation | ( | ) | const |
Definition at line 537 of file KChartLegend.cpp.
◆ paint() [1/2]
|
overridevirtual |
Overwrite this to paint the inner contents of your widget.
- Note
- When overriding this method, please let your widget draw itself at the top/left corner of the painter. You should call rect() (or width(), height(), resp.) to find the drawable area's size: While the paint() method is being executed the frame of the widget is outside of its rect(), so you can use all of rect() for your custom drawing!
- See also
- paint, paintIntoRect
Implements KChart::AbstractAreaWidget.
Definition at line 227 of file KChartLegend.cpp.
◆ paint() [2/2]
Definition at line 247 of file KChartLegend.cpp.
◆ pen()
QPen Legend::pen | ( | uint | dataset | ) | const |
Definition at line 676 of file KChartLegend.cpp.
◆ pens()
Definition at line 685 of file KChartLegend.cpp.
◆ position()
Position Legend::position | ( | ) | const |
Returns the position of a non-floating legend.
- See also
- setPosition
Definition at line 466 of file KChartLegend.cpp.
◆ propertiesChanged
|
signal |
Emitted upon change of a property of the Legend or any of its components.
◆ referenceArea()
const QWidget * Legend::referenceArea | ( | ) | const |
Returns the reference area, that is used for font size of title text, and for font size of the item texts, IF automatic area detection is set.
- See also
- setReferenceArea
Definition at line 293 of file KChartLegend.cpp.
◆ removeDiagram()
void Legend::removeDiagram | ( | KChart::AbstractDiagram * | oldDiagram | ) |
Removes the diagram from the legend's list of diagrams.
- See also
- diagram, diagrams, addDiagram, removeDiagrams, replaceDiagram, setDiagram
Definition at line 349 of file KChartLegend.cpp.
◆ removeDiagrams()
void Legend::removeDiagrams | ( | ) |
Removes all diagrams from the legend's list of diagrams.
- See also
- diagram, diagrams, addDiagram, removeDiagram, replaceDiagram, setDiagram
Definition at line 377 of file KChartLegend.cpp.
◆ replaceDiagram()
void Legend::replaceDiagram | ( | KChart::AbstractDiagram * | newDiagram, |
KChart::AbstractDiagram * | oldDiagram = nullptr ) |
Replaces the old diagram, or appends the new diagram, it there is none yet.
- Parameters
-
newDiagram The diagram to be used instead of the old one. If this parameter is zero, the first diagram will just be removed. oldDiagram The diagram to be removed by the new one. This diagram will be deleted automatically. If the parameter is omitted, the very first diagram will be replaced. In case, there was no diagram yet, the new diagram will just be added.
- See also
- diagram, diagrams, addDiagram, removeDiagram, removeDiagrams, setDiagram
Definition at line 390 of file KChartLegend.cpp.
◆ resetTexts()
void Legend::resetTexts | ( | ) |
Removes all legend texts that might have been set by setText.
This resets the Legend to default behaviour: Texts are created automatically.
Definition at line 584 of file KChartLegend.cpp.
◆ resizeEvent()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 822 of file KChartLegend.cpp.
◆ resizeLayout()
|
overridevirtual |
Reimplemented from KChart::AbstractAreaWidget.
Definition at line 146 of file KChartLegend.cpp.
◆ setAlignment()
void Legend::setAlignment | ( | Qt::Alignment | alignment | ) |
Specify the alignment of a non-floating legend.
Use setFloatingPosition to set position and alignment if your legend is floating.
- See also
- alignment, setPosition, setFloatingPosition
Definition at line 471 of file KChartLegend.cpp.
◆ setBrush()
void Legend::setBrush | ( | uint | dataset, |
const QBrush & | brush ) |
Definition at line 625 of file KChartLegend.cpp.
◆ setBrushesFromDiagram()
void Legend::setBrushesFromDiagram | ( | KChart::AbstractDiagram * | diagram | ) |
Definition at line 649 of file KChartLegend.cpp.
◆ setColor()
void Legend::setColor | ( | uint | dataset, |
const QColor & | color ) |
Note: there is no color() getter method, since setColor just sets a QBrush with the respective color, so the brush() getter method is sufficient.
Definition at line 616 of file KChartLegend.cpp.
◆ setDatasetHidden()
void Legend::setDatasetHidden | ( | uint | dataset, |
bool | hidden ) |
Definition at line 1253 of file KChartLegend.cpp.
◆ setDefaultColors()
void Legend::setDefaultColors | ( | ) |
Definition at line 786 of file KChartLegend.cpp.
◆ setDiagram()
void Legend::setDiagram | ( | KChart::AbstractDiagram * | newDiagram | ) |
A convenience method doing the same as replaceDiagram( newDiagram, 0 );.
Replaces the first diagram by the given diagram. If the legend's list of diagram is empty the given diagram is added to the list.
- See also
- diagram, diagrams, addDiagram, removeDiagram, removeDiagrams, replaceDiagram
Definition at line 426 of file KChartLegend.cpp.
◆ setFloatingPosition()
void Legend::setFloatingPosition | ( | const RelativePosition & | relativePosition | ) |
\brief Specify the position and alignment of a floating legend. Use setPosition and setAlignment to set position and alignment if your legend is non-floating. \note When setFloatingPosition is called, the Legend's position value is set to KChart::Position::Floating automatically. If your Chart is pointed to by m_chart, your could have the floating legend aligned exactly to the chart's coordinate plane's top-right corner with the following commands:
KChart::RelativePosition relativePosition; relativePosition.setReferenceArea( m_chart->coordinatePlane() ); relativePosition.setReferencePosition( Position::NorthEast ); relativePosition.setAlignment( Qt::AlignTop | Qt::AlignRight ); relativePosition.setHorizontalPadding( KChart::Measure( -1.0, KChartEnums::MeasureCalculationModeAbsolute ) ); relativePosition.setVerticalPadding( KChart::Measure( 0.0, KChartEnums::MeasureCalculationModeAbsolute ) ); m_legend->setFloatingPosition( relativePosition );
To have the legend positioned at a fixed point, measured from the QPainter's top left corner, you could use the following code code:
KChart::RelativePosition relativePosition; relativePosition.setReferencePoints( PositionPoints( QPointF( 0.0, 0.0 ) ) ); relativePosition.setReferencePosition( Position::NorthWest ); relativePosition.setAlignment( Qt::AlignTop | Qt::AlignLeft ); relativePosition.setHorizontalPadding( KChart::Measure( 4.0, KChartEnums::MeasureCalculationModeAbsolute ) ); relativePosition.setVerticalPadding( KChart::Measure( 4.0, KChartEnums::MeasureCalculationModeAbsolute ) ); m_legend->setFloatingPosition( relativePosition );
Actually that's exactly the code KChart is using as default position for any floating legends, so if you just say setPosition( KChart::Position::Floating ) without calling setFloatingPosition your legend will be positioned at point 4/4.
- See also
- setPosition, setAlignment
Definition at line 513 of file KChartLegend.cpp.
◆ setHiddenDatasets()
void Legend::setHiddenDatasets | ( | const QList< uint > | hiddenDatasets | ) |
Sets a list of datasets that are to be hidden in the legend.
By passing an empty list, you show all datasets. All datasets are shown by default, which means that hiddenDatasets() returns an empty list.
Definition at line 1243 of file KChartLegend.cpp.
◆ setLegendStyle()
void Legend::setLegendStyle | ( | LegendStyle | style | ) |
Definition at line 168 of file KChartLegend.cpp.
◆ setLegendSymbolAlignment()
void Legend::setLegendSymbolAlignment | ( | Qt::Alignment | alignment | ) |
Specify the alignment of the legend symbol( alignment of Legend::LinesOnly) within the legend.
- See also
- legendSymbolAlignment()
Definition at line 499 of file KChartLegend.cpp.
◆ setMarkerAttributes()
void Legend::setMarkerAttributes | ( | uint | dataset, |
const MarkerAttributes & | markerAttributes ) |
Note that any sizes specified via setMarkerAttributes are ignored, unless you disable the automatic size calculation, by saying setUseAutomaticMarkerSize( false )
Definition at line 691 of file KChartLegend.cpp.
◆ setOrientation()
void Legend::setOrientation | ( | Qt::Orientation | orientation | ) |
Definition at line 527 of file KChartLegend.cpp.
◆ setPen()
void Legend::setPen | ( | uint | dataset, |
const QPen & | pen ) |
Definition at line 666 of file KChartLegend.cpp.
◆ setPosition()
void Legend::setPosition | ( | Position | position | ) |
Specify the position of a non-floating legend.
Use setFloatingPosition to set position and alignment if your legend is floating.
- See also
- setAlignment, setFloatingPosition
Definition at line 450 of file KChartLegend.cpp.
◆ setRainbowColors()
void Legend::setRainbowColors | ( | ) |
Definition at line 794 of file KChartLegend.cpp.
◆ setReferenceArea()
void Legend::setReferenceArea | ( | const QWidget * | area | ) |
Specifies the reference area for font size of title text, and for font size of the item texts, IF automatic area detection is set.
- Note
- This parameter is ignored, if the Measure given for setTitleTextAttributes (or setTextAttributes, resp.) is not specifying automatic area detection.
If no reference area is specified, but automatic area detection is set, then the size of the legend's parent widget will be used.
Definition at line 284 of file KChartLegend.cpp.
◆ setShowLines()
void Legend::setShowLines | ( | bool | legendShowLines | ) |
Definition at line 557 of file KChartLegend.cpp.
◆ setSortOrder()
void Legend::setSortOrder | ( | Qt::SortOrder | order | ) |
Definition at line 542 of file KChartLegend.cpp.
◆ setSpacing()
void Legend::setSpacing | ( | uint | space | ) |
Definition at line 771 of file KChartLegend.cpp.
◆ setSubduedColors()
void Legend::setSubduedColors | ( | bool | ordered = false | ) |
Definition at line 802 of file KChartLegend.cpp.
◆ setText()
void Legend::setText | ( | uint | dataset, |
const QString & | text ) |
Definition at line 593 of file KChartLegend.cpp.
◆ setTextAlignment()
void Legend::setTextAlignment | ( | Qt::Alignment | alignment | ) |
Specify the alignment of the text elements within the legend.
- See also
- textAlignment()
Definition at line 485 of file KChartLegend.cpp.
◆ setTextAttributes()
void Legend::setTextAttributes | ( | const TextAttributes & | a | ) |
Definition at line 718 of file KChartLegend.cpp.
◆ setTitleText()
void Legend::setTitleText | ( | const QString & | text | ) |
Definition at line 732 of file KChartLegend.cpp.
◆ setTitleTextAttributes()
void Legend::setTitleTextAttributes | ( | const TextAttributes & | a | ) |
Definition at line 746 of file KChartLegend.cpp.
◆ setUseAutomaticMarkerSize()
void Legend::setUseAutomaticMarkerSize | ( | bool | useAutomaticMarkerSize | ) |
This option is on by default, it means that Marker sizes in the Legend will be the same as the font height used for their respective label texts.
Set this to false, if you want to specify the marker sizes via setMarkerAttributes or if you want the Legend to use the same marker sizes as they are used in the Diagrams.
Definition at line 572 of file KChartLegend.cpp.
◆ setVisible()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 436 of file KChartLegend.cpp.
◆ showLines()
bool Legend::showLines | ( | ) | const |
Definition at line 567 of file KChartLegend.cpp.
◆ sizeHint()
|
overridevirtual |
Reimplemented from QWidget.
Definition at line 130 of file KChartLegend.cpp.
◆ sortOrder()
Qt::SortOrder Legend::sortOrder | ( | ) | const |
Definition at line 552 of file KChartLegend.cpp.
◆ spacing()
uint Legend::spacing | ( | ) | const |
Definition at line 781 of file KChartLegend.cpp.
◆ text()
QString Legend::text | ( | uint | dataset | ) | const |
Definition at line 602 of file KChartLegend.cpp.
◆ textAlignment()
Qt::Alignment Legend::textAlignment | ( | ) | const |
Returns the alignment used while rendering text elements within the legend.
- See also
- setTextAlignment()
Definition at line 494 of file KChartLegend.cpp.
◆ textAttributes()
TextAttributes Legend::textAttributes | ( | ) | const |
Definition at line 727 of file KChartLegend.cpp.
◆ texts()
Definition at line 611 of file KChartLegend.cpp.
◆ titleText()
QString Legend::titleText | ( | ) | const |
Definition at line 741 of file KChartLegend.cpp.
◆ titleTextAttributes()
TextAttributes Legend::titleTextAttributes | ( | ) | const |
Definition at line 755 of file KChartLegend.cpp.
◆ useAutomaticMarkerSize()
bool Legend::useAutomaticMarkerSize | ( | ) | const |
Definition at line 579 of file KChartLegend.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:09:19 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.