KPropertyEditorView
#include <KPropertyEditorView.h>
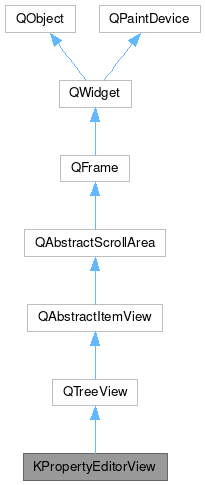
Public Types | |
enum class | SetOption { None = 0 , PreservePreviousSelection = 1 , AlphabeticalOrder = 2 } |
typedef QFlags< SetOption > | SetOptions |
![]() | |
enum | CursorAction |
enum | DragDropMode |
enum | DropIndicatorPosition |
enum | EditTrigger |
typedef | EditTriggers |
enum | ScrollHint |
enum | ScrollMode |
enum | SelectionBehavior |
enum | SelectionMode |
enum | State |
![]() | |
enum | SizeAdjustPolicy |
![]() | |
enum | Shadow |
enum | Shape |
enum | StyleMask |
![]() | |
enum | RenderFlag |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | handlePropertyEditorItemEvent (KPropertyEditorItemEvent *event) |
void | propertySetChanged (KPropertySet *set) |
Public Slots | |
void | acceptInput () |
void | changeSet (KPropertySet *set, const QByteArray &propertyToSelect, SetOptions options=SetOption::None) |
void | changeSet (KPropertySet *set, SetOptions options=SetOption::None) |
void | setChildPropertyItemsExpanded (bool set) |
void | setGridLineColor (const QColor &color) |
void | setGroupItemsExpanded (bool set) |
void | setGroupsVisible (bool set) |
void | setToolTipsVisible (bool set) |
void | setValueSyncEnabled (bool set) |
Public Member Functions | |
KPropertyEditorView (QWidget *parent=nullptr) | |
bool | childPropertyItemsExpanded () const |
QColor | gridLineColor () const |
bool | groupItemsExpanded () const |
bool | groupsVisible () const |
bool | isValueSyncEnabled () const |
KPropertySet * | propertySet () const |
QSize | sizeHint () const override |
bool | toolTipsVisible () const |
![]() | |
QTreeView (QWidget *parent) | |
bool | allColumnsShowFocus () const const |
int | autoExpandDelay () const const |
void | collapse (const QModelIndex &index) |
void | collapseAll () |
void | collapsed (const QModelIndex &index) |
int | columnAt (int x) const const |
int | columnViewportPosition (int column) const const |
int | columnWidth (int column) const const |
virtual void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) override |
void | expand (const QModelIndex &index) |
void | expandAll () |
void | expanded (const QModelIndex &index) |
void | expandRecursively (const QModelIndex &index, int depth) |
bool | expandsOnDoubleClick () const const |
void | expandToDepth (int depth) |
QHeaderView * | header () const const |
void | hideColumn (int column) |
int | indentation () const const |
QModelIndex | indexAbove (const QModelIndex &index) const const |
virtual QModelIndex | indexAt (const QPoint &point) const const override |
QModelIndex | indexBelow (const QModelIndex &index) const const |
bool | isAnimated () const const |
bool | isColumnHidden (int column) const const |
bool | isExpanded (const QModelIndex &index) const const |
bool | isFirstColumnSpanned (int row, const QModelIndex &parent) const const |
bool | isHeaderHidden () const const |
bool | isRowHidden (int row, const QModelIndex &parent) const const |
bool | isSortingEnabled () const const |
bool | itemsExpandable () const const |
virtual void | keyboardSearch (const QString &search) override |
virtual void | reset () override |
void | resetIndentation () |
void | resizeColumnToContents (int column) |
bool | rootIsDecorated () const const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint) override |
virtual void | selectAll () override |
void | setAllColumnsShowFocus (bool enable) |
void | setAnimated (bool enable) |
void | setAutoExpandDelay (int delay) |
void | setColumnHidden (int column, bool hide) |
void | setColumnWidth (int column, int width) |
void | setExpanded (const QModelIndex &index, bool expanded) |
void | setExpandsOnDoubleClick (bool enable) |
void | setFirstColumnSpanned (int row, const QModelIndex &parent, bool span) |
void | setHeader (QHeaderView *header) |
void | setHeaderHidden (bool hide) |
void | setIndentation (int i) |
void | setItemsExpandable (bool enable) |
virtual void | setModel (QAbstractItemModel *model) override |
virtual void | setRootIndex (const QModelIndex &index) override |
void | setRootIsDecorated (bool show) |
void | setRowHidden (int row, const QModelIndex &parent, bool hide) |
virtual void | setSelectionModel (QItemSelectionModel *selectionModel) override |
void | setSortingEnabled (bool enable) |
void | setTreePosition (int index) |
void | setUniformRowHeights (bool uniform) |
void | setWordWrap (bool on) |
void | showColumn (int column) |
void | sortByColumn (int column, Qt::SortOrder order) |
int | treePosition () const const |
bool | uniformRowHeights () const const |
virtual QRect | visualRect (const QModelIndex &index) const const override |
bool | wordWrap () const const |
![]() | |
QAbstractItemView (QWidget *parent) | |
void | activated (const QModelIndex &index) |
bool | alternatingRowColors () const const |
int | autoScrollMargin () const const |
void | clearSelection () |
void | clicked (const QModelIndex &index) |
void | closePersistentEditor (const QModelIndex &index) |
QModelIndex | currentIndex () const const |
Qt::DropAction | defaultDropAction () const const |
void | doubleClicked (const QModelIndex &index) |
DragDropMode | dragDropMode () const const |
bool | dragDropOverwriteMode () const const |
bool | dragEnabled () const const |
void | edit (const QModelIndex &index) |
EditTriggers | editTriggers () const const |
void | entered (const QModelIndex &index) |
bool | hasAutoScroll () const const |
ScrollMode | horizontalScrollMode () const const |
QSize | iconSize () const const |
void | iconSizeChanged (const QSize &size) |
QWidget * | indexWidget (const QModelIndex &index) const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const override |
bool | isPersistentEditorOpen (const QModelIndex &index) const const |
QAbstractItemDelegate * | itemDelegate () const const |
QAbstractItemDelegate * | itemDelegate (const QModelIndex &index) const const |
QAbstractItemDelegate * | itemDelegateForColumn (int column) const const |
virtual QAbstractItemDelegate * | itemDelegateForIndex (const QModelIndex &index) const const |
QAbstractItemDelegate * | itemDelegateForRow (int row) const const |
QAbstractItemModel * | model () const const |
void | openPersistentEditor (const QModelIndex &index) |
void | pressed (const QModelIndex &index) |
void | resetHorizontalScrollMode () |
void | resetVerticalScrollMode () |
QModelIndex | rootIndex () const const |
void | scrollToBottom () |
void | scrollToTop () |
QAbstractItemView::SelectionBehavior | selectionBehavior () const const |
QAbstractItemView::SelectionMode | selectionMode () const const |
QItemSelectionModel * | selectionModel () const const |
void | setAlternatingRowColors (bool enable) |
void | setAutoScroll (bool enable) |
void | setAutoScrollMargin (int margin) |
void | setCurrentIndex (const QModelIndex &index) |
void | setDefaultDropAction (Qt::DropAction dropAction) |
void | setDragDropMode (DragDropMode behavior) |
void | setDragDropOverwriteMode (bool overwrite) |
void | setDragEnabled (bool enable) |
void | setDropIndicatorShown (bool enable) |
void | setEditTriggers (EditTriggers triggers) |
void | setHorizontalScrollMode (ScrollMode mode) |
void | setIconSize (const QSize &size) |
void | setIndexWidget (const QModelIndex &index, QWidget *widget) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemDelegateForColumn (int column, QAbstractItemDelegate *delegate) |
void | setItemDelegateForRow (int row, QAbstractItemDelegate *delegate) |
void | setSelectionBehavior (QAbstractItemView::SelectionBehavior behavior) |
void | setSelectionMode (QAbstractItemView::SelectionMode mode) |
void | setTabKeyNavigation (bool enable) |
void | setTextElideMode (Qt::TextElideMode mode) |
void | setVerticalScrollMode (ScrollMode mode) |
bool | showDropIndicator () const const |
QSize | sizeHintForIndex (const QModelIndex &index) const const |
virtual int | sizeHintForRow (int row) const const |
bool | tabKeyNavigation () const const |
Qt::TextElideMode | textElideMode () const const |
void | update (const QModelIndex &index) |
ScrollMode | verticalScrollMode () const const |
void | viewportEntered () |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
void | addScrollBarWidget (QWidget *widget, Qt::Alignment alignment) |
QWidget * | cornerWidget () const const |
QScrollBar * | horizontalScrollBar () const const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const const |
QSize | maximumViewportSize () const const |
virtual QSize | minimumSizeHint () const const override |
QWidgetList | scrollBarWidgets (Qt::Alignment alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setSizeAdjustPolicy (SizeAdjustPolicy policy) |
virtual void | setupViewport (QWidget *viewport) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
SizeAdjustPolicy | sizeAdjustPolicy () const const |
QScrollBar * | verticalScrollBar () const const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const const |
QWidget * | viewport () const const |
![]() | |
QFrame (QWidget *parent, Qt::WindowFlags f) | |
QRect | frameRect () const const |
Shadow | frameShadow () const const |
Shape | frameShape () const const |
int | frameStyle () const const |
int | frameWidth () const const |
int | lineWidth () const const |
int | midLineWidth () const const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static QColor | defaultGridLineColor () |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
void | commitData (QWidget *editor) override |
void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) override |
void | slotPropertyChanged (KPropertySet &set, KProperty &property) |
void | slotPropertyReset (KPropertySet &set, KProperty &property) |
void | slotReadOnlyFlagChanged () |
void | slotSetWillBeCleared () |
void | slotSetWillBeDeleted () |
Protected Member Functions | |
bool | viewportEvent (QEvent *event) override |
![]() | |
virtual void | changeEvent (QEvent *event) override |
void | columnCountChanged (int oldCount, int newCount) |
void | columnMoved () |
void | columnResized (int column, int oldSize, int newSize) |
virtual void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) override |
virtual void | dragMoveEvent (QDragMoveEvent *event) override |
void | drawTree (QPainter *painter, const QRegion ®ion) const const |
virtual int | horizontalOffset () const const override |
int | indexRowSizeHint (const QModelIndex &index) const const |
virtual bool | isIndexHidden (const QModelIndex &index) const const override |
virtual void | keyPressEvent (QKeyEvent *event) override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) override |
virtual void | mouseMoveEvent (QMouseEvent *event) override |
virtual void | mouseReleaseEvent (QMouseEvent *event) override |
virtual QModelIndex | moveCursor (CursorAction cursorAction, Qt::KeyboardModifiers modifiers) override |
virtual void | paintEvent (QPaintEvent *event) override |
int | rowHeight (const QModelIndex &index) const const |
virtual void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) override |
virtual void | rowsInserted (const QModelIndex &parent, int start, int end) override |
void | rowsRemoved (const QModelIndex &parent, int start, int end) |
virtual void | scrollContentsBy (int dx, int dy) override |
virtual QModelIndexList | selectedIndexes () const const override |
virtual void | selectionChanged (const QItemSelection &selected, const QItemSelection &deselected) override |
virtual void | setSelection (const QRect &rect, QItemSelectionModel::SelectionFlags command) override |
virtual int | sizeHintForColumn (int column) const const override |
virtual void | timerEvent (QTimerEvent *event) override |
virtual void | updateGeometries () override |
virtual int | verticalOffset () const const override |
virtual QSize | viewportSizeHint () const const override |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const const override |
![]() | |
virtual void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
virtual void | commitData (QWidget *editor) |
QPoint | dirtyRegionOffset () const const |
virtual void | dragEnterEvent (QDragEnterEvent *event) override |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) override |
virtual void | dropEvent (QDropEvent *event) override |
DropIndicatorPosition | dropIndicatorPosition () const const |
virtual void | editorDestroyed (QObject *editor) |
virtual bool | event (QEvent *event) override |
virtual bool | eventFilter (QObject *object, QEvent *event) override |
void | executeDelayedItemsLayout () |
virtual void | focusInEvent (QFocusEvent *event) override |
virtual bool | focusNextPrevChild (bool next) override |
virtual void | focusOutEvent (QFocusEvent *event) override |
virtual void | initViewItemOption (QStyleOptionViewItem *option) const const |
virtual void | inputMethodEvent (QInputMethodEvent *event) override |
virtual void | resizeEvent (QResizeEvent *event) override |
void | scheduleDelayedItemsLayout () |
void | scrollDirtyRegion (int dx, int dy) |
virtual QItemSelectionModel::SelectionFlags | selectionCommand (const QModelIndex &index, const QEvent *event) const const |
void | setDirtyRegion (const QRegion ®ion) |
void | setState (State state) |
virtual void | startDrag (Qt::DropActions supportedActions) |
State | state () const const |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *e) override |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
QMargins | viewportMargins () const const |
virtual void | wheelEvent (QWheelEvent *e) override |
![]() | |
virtual void | initStyleOption (QStyleOptionFrame *option) const const |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEnterEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
![]() |
Detailed Description
A widget for editing properties.
Definition at line 32 of file KPropertyEditorView.h.
Member Typedef Documentation
◆ SetOptions
Definition at line 48 of file KPropertyEditorView.h.
Member Enumeration Documentation
◆ SetOption
|
strong |
Options for changeSet()
Enumerator | |
---|---|
PreservePreviousSelection | If used, previously selected editor item will be kept selected. |
AlphabeticalOrder | Alphabetical order of properties. The default is order of insertion. |
Definition at line 42 of file KPropertyEditorView.h.
Constructor & Destructor Documentation
◆ KPropertyEditorView()
|
explicit |
Creates an empty property editor with parent as parent widget.
Definition at line 320 of file KPropertyEditorView.cpp.
◆ ~KPropertyEditorView()
|
override |
Definition at line 343 of file KPropertyEditorView.cpp.
Member Function Documentation
◆ acceptInput
|
slot |
Accepts the changes made to the current editor item (if any) (as if the user had pressed Enter key).
Definition at line 629 of file KPropertyEditorView.cpp.
◆ changeSet [1/2]
|
slot |
Populates the editor view with items for each property from the set set. Child items for composed properties are also created. If propertyToSelect is provided, item for this property name will be selected, if present.
Definition at line 353 of file KPropertyEditorView.cpp.
◆ changeSet [2/2]
|
slot |
Populates the editor view with items for each property from the set set. Child items for composed properties are also created. See SetOption documentation for description of options options. If preservePreviousSelection is true, previously selected editor item will be kept selected, if present.
Definition at line 348 of file KPropertyEditorView.cpp.
◆ childPropertyItemsExpanded()
bool KPropertyEditorView::childPropertyItemsExpanded | ( | ) | const |
- Returns
true
if items for parent composed properties are expanded so items for child properties are displayed.
- Since
- 3.1
Definition at line 501 of file KPropertyEditorView.cpp.
◆ commitData
|
overrideprotectedslot |
Definition at line 634 of file KPropertyEditorView.cpp.
◆ currentChanged
|
overrideprotectedslot |
Definition at line 533 of file KPropertyEditorView.cpp.
◆ defaultGridLineColor()
|
inlinestatic |
- Returns
- default grid line color - Qt::gray
Definition at line 54 of file KPropertyEditorView.h.
◆ gridLineColor()
QColor KPropertyEditorView::gridLineColor | ( | ) | const |
- Returns
- grid line color, defaultGridLineColor() by default
Definition at line 665 of file KPropertyEditorView.cpp.
◆ groupItemsExpanded()
bool KPropertyEditorView::groupItemsExpanded | ( | ) | const |
- Returns
true
if group items for newly added groups are exapanded so properties for these groups are displayed.
- See also
- setGroupItemsExpanded()
- Since
- 3.1
Definition at line 511 of file KPropertyEditorView.cpp.
◆ groupsVisible()
bool KPropertyEditorView::groupsVisible | ( | ) | const |
- Returns
true
if the property groups should be visible. By default groups are visible. A group is visualized as a subtree displaying group caption and group icon at its root node (see KProperty::groupCaption and KProperty::groupIconName) and properties as children of this node. A property is assigned to a group while KPropertySet::addProperty() is called.
- Note
- Regardless of this flag, no groups are displayed if there is only the default group "common".
When the group visibility flag is off or only the "common" group is present, all properties are displayed on the same (top) level.
- Since
- 3.1
Definition at line 516 of file KPropertyEditorView.cpp.
◆ handlePropertyEditorItemEvent
|
signal |
Emitted when active property editor widget offers overriding of its editing behavior.
See KPropertyEditorItemEvent for usage details.
- Note
- event is owned by the KPropertyEditorView object.
- Since
- 3.2
◆ isValueSyncEnabled()
bool KPropertyEditorView::isValueSyncEnabled | ( | ) | const |
- Returns
- value of the valueSyncEnabled flag.
- Since
- 3.1
Definition at line 491 of file KPropertyEditorView.cpp.
◆ propertySet()
KPropertySet * KPropertyEditorView::propertySet | ( | ) | const |
- Returns
- the property set object that is assigned to this view or nullptr is no set is currently assigned.
Definition at line 660 of file KPropertyEditorView.cpp.
◆ propertySetChanged
|
signal |
Emitted when current property set has been changed. May be 0.
◆ setChildPropertyItemsExpanded
|
slot |
If set is true
(the default), items for parent composed properties are expanded so items for child properties are displayed. If set is false
, the items are collapsed.
- Note
- Appearance of the existing child items is not altered. This method can be typically called before a changeSet() call or before adding properties.
- Expansion of group items is not affected by this method. Use setGroupItemsExpanded() to control expansion of group items.
- To expand all items use expandAll(). To collapse all items use collapseAll().
- Since
- 3.1
Definition at line 496 of file KPropertyEditorView.cpp.
◆ setGridLineColor
|
slot |
Sets color of grid lines. Use invalid color QColor() to hide grid lines.
Definition at line 670 of file KPropertyEditorView.cpp.
◆ setGroupItemsExpanded
|
slot |
If set is true
(the default), group items for newly added groups are exapanded so properties for these groups are displayed. If set is false
, the items are collapsed.
- Note
- Appearance of the existing group items is not altered. This method can be typically called before a changeSet() call or before adding properties.
- Expansion of child items for composed properties is not affected by this method. Use setChildPropertyItemsExpanded() to control expansion child items for composed properties.
- To expand all items use expandAll(). To collapse all items use collapseAll().
- Since
- 3.1
Definition at line 506 of file KPropertyEditorView.cpp.
◆ setGroupsVisible
|
slot |
Shows the property groups if set is true
.
- See also
- groupsVisible()
- Since
- 3.1
Definition at line 521 of file KPropertyEditorView.cpp.
◆ setToolTipsVisible
|
slot |
If set is true
tooltips are visible for property editor items.
See toolTipsVisible() for details.
- Since
- 3.1
Definition at line 747 of file KPropertyEditorView.cpp.
◆ setValueSyncEnabled
|
slot |
If set is true
(the default), property values are automatically synchronized as soon as editor contents change (e.g. every time the user types a character) and the values are saved back to the assigned property set. If enable is false, property set is updated only when selection within the property editor or user presses Enter/Return key. Each property can override this policy by changing its own valueSyncPolicy flag.
- See also
- KProperty::setValueSyncPolicy()
- Since
- 3.1
Definition at line 486 of file KPropertyEditorView.cpp.
◆ sizeHint()
|
overridevirtual |
Reimplemented to suggest widget size that is based on number of property items.
Reimplemented from QAbstractScrollArea.
Definition at line 655 of file KPropertyEditorView.cpp.
◆ slotPropertyChanged
|
protectedslot |
Updates editor widget in the editor.
Definition at line 697 of file KPropertyEditorView.cpp.
◆ slotPropertyReset
|
protectedslot |
- Todo
- OK?
Definition at line 735 of file KPropertyEditorView.cpp.
◆ slotReadOnlyFlagChanged
|
protectedslot |
Called when property set's read-only flag has changed. Refreshes selection so editor is displayed again if needed.
Definition at line 476 of file KPropertyEditorView.cpp.
◆ slotSetWillBeCleared
|
protectedslot |
Called when current propertis of this set are about to be cleared.
Definition at line 466 of file KPropertyEditorView.cpp.
◆ slotSetWillBeDeleted
|
protectedslot |
Called when current property set is about to be destroyed.
Definition at line 471 of file KPropertyEditorView.cpp.
◆ toolTipsVisible()
bool KPropertyEditorView::toolTipsVisible | ( | ) | const |
Returns true
if the property editor widget has enabled visibility of tooltips.
Tooltips are displayed over each property item, both the name and value column, and are equal to property descriptions (KProperty::description()). Tooltips are not displayed for items having empty descriptions.
Tooltips visibility is disabled by default.
- Since
- 3.1
Definition at line 742 of file KPropertyEditorView.cpp.
◆ viewportEvent()
|
overrideprotectedvirtual |
Reimplemented from QTreeView.
Definition at line 639 of file KPropertyEditorView.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:11:19 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.