ImageDocument
#include <imagedocument.h>
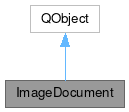
Properties | |
bool | edited |
QImage | image |
QML_ELEMENTQUrl | path |
![]() | |
objectName | |
Signals | |
void | editedChanged () |
void | imageChanged () |
void | pathChanged (const QUrl &url) |
Public Member Functions | |
ImageDocument (QObject *parent=nullptr) | |
Q_INVOKABLE void | cancel () |
Q_INVOKABLE void | crop (int x, int y, int width, int height) |
bool | edited () const |
QImage | image () const |
Q_INVOKABLE void | mirror (bool horizontal, bool vertical) |
QUrl | path () const |
Q_INVOKABLE void | resize (int width, int height) |
Q_INVOKABLE void | rotate (int angle) |
Q_INVOKABLE bool | save () |
Q_INVOKABLE bool | saveAs (const QUrl &location) |
void | setEdited (bool value) |
void | setPath (const QUrl &path) |
Q_INVOKABLE void | undo () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An ImageDocument is the base class of the ImageEditor.
This class handles various image manipulation and contains an undo stack to allow reverting the last actions. This class does not display the image, use ImageItem
for this task.
Definition at line 40 of file imagedocument.h.
Property Documentation
◆ edited
|
readwrite |
Definition at line 47 of file imagedocument.h.
◆ image
|
read |
Definition at line 46 of file imagedocument.h.
◆ path
|
readwrite |
Definition at line 45 of file imagedocument.h.
Constructor & Destructor Documentation
◆ ImageDocument()
ImageDocument::ImageDocument | ( | QObject * | parent = nullptr | ) |
Definition at line 14 of file imagedocument.cpp.
Member Function Documentation
◆ cancel()
void ImageDocument::cancel | ( | ) |
Cancel all the edit.
Definition at line 25 of file imagedocument.cpp.
◆ crop()
void ImageDocument::crop | ( | int | x, |
int | y, | ||
int | width, | ||
int | height ) |
Crop the image.
- Parameters
-
x The x coordinate of the new image in the old image. y The y coordinate of the new image in the old image. width The width of the new image. height The height of the new image.
Definition at line 58 of file imagedocument.cpp.
◆ edited()
bool ImageDocument::edited | ( | ) | const |
This propriety store if the document was changed or not.
- See also
- setEdited
- editedChanged
Definition at line 41 of file imagedocument.cpp.
◆ image()
QImage ImageDocument::image | ( | ) | const |
The image was is displayed.
This propriety is updated when the path change or commands are applied.
- See also
- imageChanged
Definition at line 36 of file imagedocument.cpp.
◆ mirror()
void ImageDocument::mirror | ( | bool | horizontal, |
bool | vertical ) |
Mirror the image.
- Parameters
-
horizontal Mirror the image horizontally. vertical Mirror the image vertically.
Definition at line 76 of file imagedocument.cpp.
◆ path()
QUrl ImageDocument::path | ( | ) | const |
Definition at line 116 of file imagedocument.cpp.
◆ resize()
void ImageDocument::resize | ( | int | width, |
int | height ) |
Resize the image.
- Parameters
-
width The width of the new image. height The height of the new image.
Definition at line 67 of file imagedocument.cpp.
◆ rotate()
void ImageDocument::rotate | ( | int | angle | ) |
Rotate the image.
- Parameters
-
angle The angle of the rotation in degree.
Definition at line 85 of file imagedocument.cpp.
◆ save()
bool ImageDocument::save | ( | ) |
Save current edited image in place.
This is a destructive operation and can't be reverted.
- Returns
- true iff the file saving operation was successful.
Definition at line 106 of file imagedocument.cpp.
◆ saveAs()
bool ImageDocument::saveAs | ( | const QUrl & | location | ) |
Save current edited image as a new image.
- Parameters
-
location The location where to save the new image.
- Returns
- true iff the file saving operattion was successful.
Definition at line 111 of file imagedocument.cpp.
◆ setEdited()
void ImageDocument::setEdited | ( | bool | value | ) |
Change the edited value.
- Parameters
-
value The new value.
Definition at line 96 of file imagedocument.cpp.
◆ setPath()
void ImageDocument::setPath | ( | const QUrl & | path | ) |
Definition at line 121 of file imagedocument.cpp.
◆ undo()
void ImageDocument::undo | ( | ) |
Undo the last edit on the images.
Definition at line 46 of file imagedocument.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 4 2025 12:11:05 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.