KSaneCore::Interface
#include <interface.h>
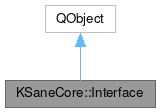
Public Types | |
enum | DeviceType { AllDevices , NoCameraAndVirtualDevices } |
enum | OpenStatus { OpeningSucceeded , OpeningDenied , OpeningFailed } |
enum | OptionName { SourceOption , ScanModeOption , BitDepthOption , ResolutionOption , TopLeftXOption , TopLeftYOption , BottomRightXOption , BottomRightYOption , FilmTypeOption , NegativeOption , InvertColorOption , PageSizeOption , ThresholdOption , XResolutionOption , YResolutionOption , PreviewOption , WaitForButtonOption , BrightnessOption , ContrastOption , GammaOption , GammaRedOption , GammaGreenOption , GammaBlueOption , BlackLevelOption , WhiteLevelOption , BatchModeOption , BatchDelayOption } |
enum | ScanStatus { NoError , ErrorGeneral , Information } |
Signals | |
void | availableDevices (const QList< DeviceInformation * > &deviceList) |
void | batchModeCountDown (int remainingSeconds) |
void | buttonPressed (const QString &optionName, const QString &optionLabel, bool pressed) |
void | previewImageReady (const QImage &previewImage) |
void | previewProgress (int percent) |
void | previewScanFinished (KSaneCore::Interface::ScanStatus status, const QString &strStatus) |
void | scanFinished (KSaneCore::Interface::ScanStatus status, const QString &strStatus) |
void | scannedImageReady (const QImage &scannedImage) |
void | scanProgress (int percent) |
void | userMessage (KSaneCore::Interface::ScanStatus status, const QString &strStatus) |
Public Slots | |
void | startPreviewScan () |
void | startScan () |
void | stopScan () |
Public Member Functions | |
Interface (QObject *parent=nullptr) | |
~Interface () override | |
bool | closeDevice () |
QString | deviceModel () const |
QString | deviceName () const |
QString | deviceVendor () const |
Option * | getOption (const QString &optionName) |
Option * | getOption (OptionName optionEnum) |
QList< Option * > | getOptionsList () |
QMap< QString, QString > | getOptionsMap () |
void | lockScanImage () |
OpenStatus | openDevice (const QString &deviceName) |
OpenStatus | openRestrictedDevice (const QString &deviceName, const QString &userName, const QString &password) |
bool | reloadDevicesList (DeviceType type=AllDevices) |
QImage * | scanImage () const |
QJsonObject | scannerDeviceToJson () |
QJsonObject | scannerOptionsToJson () |
int | setOptionsMap (const QMap< QString, QString > &options) |
void | setPreviewResolution (float dpi) |
void | unlockScanImage () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class provides the core interface for accessing the scan controls and options.
Definition at line 33 of file interface.h.
Member Enumeration Documentation
◆ DeviceType
This enumeration is used to filter the devices found by SANE.
Sometimes, webcam may show up as scanner device and some more special scanner are also classified as cameras.
Definition at line 98 of file interface.h.
◆ OpenStatus
Enum determining whether the scanner opened correctly.
Definition at line 52 of file interface.h.
◆ OptionName
This enumeration is used to obtain a specific option with getOption(KSaneOptionName).
Depending on the backend, not all options are available, nor this list is complete. For the remaining options, getOptionsList() must be used.
Definition at line 63 of file interface.h.
◆ ScanStatus
Enum defining the message level of the returned scan status string.
- Note
- There might come more enumerations in the future.
Definition at line 43 of file interface.h.
Constructor & Destructor Documentation
◆ Interface()
|
explicit |
This constructor initializes the private class variables.
Definition at line 37 of file interface.cpp.
◆ ~Interface()
|
override |
Standard destructor.
Definition at line 60 of file interface.cpp.
Member Function Documentation
◆ availableDevices
|
signal |
This signal is emitted every time the device list is updated or after reloadDevicesList() is called.
- Parameters
-
deviceList is a QList of KSane::DeviceInformation that contain the device name, model, vendor and type of the attached scanners.
- Note
- The list is only a snapshot of the current available devices. Devices might be added or removed/opened after the signal is emitted.
◆ batchModeCountDown
|
signal |
This signal is emitted for the count down when in batch mode.
- Parameters
-
remainingSeconds are the remaining seconds until the next scan starts.
◆ buttonPressed
|
signal |
This signal is emitted when a hardware button is pressed.
- Parameters
-
optionName is the untranslated technical name of the sane-option. optionLabel is the translated user visible label of the sane-option. pressed indicates if the value is true or false.
- Note
- The SANE standard does not specify hardware buttons and their behaviors, so this signal is emitted for sane-options that behave like hardware buttons. That is the sane-options are read-only and type boolean. The naming of hardware buttons also differ from backend to backend.
◆ closeDevice()
bool KSaneCore::Interface::closeDevice | ( | ) |
This method closes the currently open scanner device.
- Returns
- 'true' if all goes well and 'false' if no device is open.
Definition at line 177 of file interface.cpp.
◆ deviceModel()
QString KSaneCore::Interface::deviceModel | ( | ) | const |
This method returns the model of the currently opened scanner.
- Note
- Due to limitations of the SANE API, this will function will return an empty string if reloadDevicesList() has not been called before.
Definition at line 86 of file interface.cpp.
◆ deviceName()
QString KSaneCore::Interface::deviceName | ( | ) | const |
This method returns the internal device name of the currently opened scanner.
- Note
- Due to limitations of the SANE API, this will function will return an empty string if reloadDevicesList() has not been called before.
Definition at line 76 of file interface.cpp.
◆ deviceVendor()
QString KSaneCore::Interface::deviceVendor | ( | ) | const |
This method returns the vendor name of the currently opened scanner.
- Note
- Due to limitations of the SANE API, this will function will return an empty string if reloadDevicesList() has not been called before.
Definition at line 81 of file interface.cpp.
◆ getOption() [1/2]
This function returns a specific option.
- Parameters
-
optionName the internal name of the option defined by SANE.
- Returns
- pointer to the KSaneOption. Returns a nullptr in case the options is not available for the currently opened device.
Definition at line 392 of file interface.cpp.
◆ getOption() [2/2]
Option * KSaneCore::Interface::getOption | ( | Interface::OptionName | optionEnum | ) |
This function returns a specific option.
- Parameters
-
optionEnum the enum specifying the option.
- Returns
- pointer to the KSaneOption. Returns a nullptr in case the options is not available for the currently opened device.
Definition at line 383 of file interface.cpp.
◆ getOptionsList()
This function returns all available options when a device is opened.
- Returns
- list containing pointers to all KSaneOptions provided by the backend. Becomes invalid when closing a device. The pointers must not be deleted by the client.
Definition at line 378 of file interface.cpp.
◆ getOptionsMap()
This method reads the available parameters and their values and returns them in a QMap (Name, value)
- Returns
- map with the parameter names and their values.
Definition at line 402 of file interface.cpp.
◆ lockScanImage()
void KSaneCore::Interface::lockScanImage | ( | ) |
Locks the mutex protecting the QImage pointer of scanImage() from concurrent access during scanning.
Definition at line 325 of file interface.cpp.
◆ openDevice()
Interface::OpenStatus KSaneCore::Interface::openDevice | ( | const QString & | deviceName | ) |
This method opens the specified scanner device and adds the scan options to the options list.
- Parameters
-
deviceName is the SANE device name for the scanner to open.
- Returns
- the status of the opening action.
Definition at line 109 of file interface.cpp.
◆ openRestrictedDevice()
Interface::OpenStatus KSaneCore::Interface::openRestrictedDevice | ( | const QString & | deviceName, |
const QString & | userName, | ||
const QString & | password ) |
This method opens the specified scanner device with a specified username and password.
Adds the scan options to the options list.
- Parameters
-
deviceName is the SANE device name for the scanner to open. userName the username required to open for the scanner. password the password required to open for the scanner.
- Returns
- the status of the opening action.
Definition at line 141 of file interface.cpp.
◆ previewImageReady
|
signal |
This signal is emitted when a preview scan is ready.
- Parameters
-
scannedImage is the QImage containing the scanned image data of the preview.
- Since
- 25.04
◆ previewProgress
|
signal |
This signal is emitted for progress information during a preview scan.
- Parameters
-
percent is the percentage of the preview scan progress (0-100). A negative value indicates that a preview scan is being prepared.
- Since
- 25.04
◆ previewScanFinished
|
signal |
This signal is emitted when the scanning for a preview has ended.
- Parameters
-
status contains a ScanStatus status code. strStatus If an error has occurred this string will contain an error message. otherwise the string is empty.
- Since
- 25.04
◆ reloadDevicesList()
bool KSaneCore::Interface::reloadDevicesList | ( | DeviceType | type = AllDevices | ) |
Get the list of available scanning devices.
Connect to availableDevices() which is fired once these devices are known.
- Note
- While the querying is done in a separate thread and thus not blocking the application, the application must ensure that no other action accessing the scanner device (settings options etc.) is performed during this period.
- Returns
- whether the devices list are being reloaded or not
- Parameters
-
type specify whether only specific device types shall be queried
Definition at line 96 of file interface.cpp.
◆ scanFinished
|
signal |
This signal is emitted when the scanning has ended.
- Parameters
-
status contains a ScanStatus status code. strStatus If an error has occurred this string will contain an error message. otherwise the string is empty.
◆ scanImage()
QImage * KSaneCore::Interface::scanImage | ( | ) | const |
Gives direct access to the QImage that is used to store the image data retrieved from the scanner.
Useful to display an in-progress image while scanning. When accessing the direct image pointer during a scan, the image must be locked before accessing the image and unlocked afterwards using the lockScanImage() and unlockScanImage() functions.
- Returns
- pointer for direct access of the QImage data.
Definition at line 317 of file interface.cpp.
◆ scannedImageReady
|
signal |
This signal is emitted when a final scan is ready.
- Parameters
-
scannedImage is the QImage containing the scanned image data.
- Since
- 25.04
◆ scannerDeviceToJson()
QJsonObject KSaneCore::Interface::scannerDeviceToJson | ( | ) |
Returns a JSON object containing the device name, model and vendor.
A scanner device must have been opened before, returns an empty object otherwise. Mainly intended for debugging purposes and identifying issues with different scanner hardware.
- Returns
- JSON object holding the data
Definition at line 339 of file interface.cpp.
◆ scannerOptionsToJson()
QJsonObject KSaneCore::Interface::scannerOptionsToJson | ( | ) |
Returns a JSON Object with all available data for all scanner options.
A scanner device must have been opened before, returns an empty object otherwise. Mainly intended for debugging purposes and identifying issues with different scanner hardware.
- Returns
- JSON object holding the data
Definition at line 352 of file interface.cpp.
◆ scanProgress
|
signal |
This signal is emitted for progress information during a scan.
- Parameters
-
percent is the percentage of the scan progress (0-100). A negative value indicates that a scan is being prepared.
◆ setOptionsMap()
This method can be used to write many parameter values at once.
- Parameters
-
options a QMap with the parameter names and values.
- Returns
- This function returns the number of successful writes or -1 if scanning is in progress.
Definition at line 416 of file interface.cpp.
◆ setPreviewResolution()
void KSaneCore::Interface::setPreviewResolution | ( | float | dpi | ) |
This function is used to set the preferred resolution for scanning the preview.
- Parameters
-
dpi is the wanted scan resolution for the preview
- Note
- if the set value is not supported, the closest one is used
- setting the value 0 means that the default calculated value should be used.
- Since
- 25.04
Definition at line 91 of file interface.cpp.
◆ startPreviewScan
|
slot |
This method is used to start a preview scan.
- Note
- KSaneCore::Interface may return one image as a result of one invocation of this slot. The image always covers the full scan area of the device with a reduced resolution. A preferred preview DPI can be set via setPreviewResolution(), but will be adjusted to capabilities of the device.
- While scanning is done in a separate thread and thus not blocking the application, the application must ensure that no other action accessing the scanner device (settings options etc.) is performed during this period besides accessing *scanImage() that must be guarded lockScanImage() and unlockScanImage() before and after the access.
- Since
- 25.04
Definition at line 217 of file interface.cpp.
◆ startScan
|
slot |
This method is used to start a scan.
- Note
- KSaneCore::Interface may return one or more images as a result of one invocation of this slot. If no more images are wanted stopScan() should be called in the slot handling the imageReady signal.
- While scanning is done in a separate thread and thus not blocking the application, the application must ensure that no other action accessing the scanner device (settings options etc.) is performed during this period besides accessing *scanImage() that must be guarded lockScanImage() and unlockScanImage() before and after the access.
Definition at line 201 of file interface.cpp.
◆ stopScan
|
slot |
This method is used to cancel a scan or prevent an automatic new scan.
Definition at line 300 of file interface.cpp.
◆ unlockScanImage()
void KSaneCore::Interface::unlockScanImage | ( | ) |
Unlocks the mutex protecting the QImage pointer of scanImage() from concurrent access during scanning.
The scanning progress will blocked when lockScanImage() is called until unlockScanImage() is called.
Definition at line 332 of file interface.cpp.
◆ userMessage
|
signal |
This signal is emitted when the user is to be notified about something.
- Parameters
-
type contains a ScanStatus code to identify the type of message (error/info/...). strStatus If an error has occurred this string will contain an error message. otherwise the string is empty.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 12:00:20 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.