OllamaReply
#include <ollamareply.h>
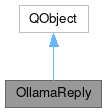
Signals | |
void | contentAdded () |
void | finished () |
Public Member Functions | |
OllamaReply (QNetworkReply *netReply, QObject *parent=nullptr) | |
const TextAutogenerateText::TextAutogenerateTextContext & | context () const |
const OllamaReplyInfo & | info () const |
bool | isFinished () const |
QString | readResponse () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The OllamaReply class represents a reply from an LLM.
If you want to stream a reply as it is written in real time, connect to contentAdded() and use readResponse() to retrieve the new content. If you prefer to wait for the entire reply before displaying anything, connect to finished(), which will only be emitted once the reply is complete.
Definition at line 47 of file ollamareply.h.
Constructor & Destructor Documentation
◆ OllamaReply()
|
explicit |
Definition at line 14 of file ollamareply.cpp.
Member Function Documentation
◆ contentAdded
|
signal |
Emits when new content has been added to the response.
If you are not streaming the response live, this signal is not of importance to you. However, if you are streaming content, when this signal is emitted, you should call readResponse() to update the response that your application shows.
◆ context()
const TextAutogenerateText::TextAutogenerateTextContext & OllamaReply::context | ( | ) | const |
Get the context token for this response.
Messages sent by most LLMs have a context identifier that allows you to chain messages into a conversation. To create such a conversation, you need to take this context object and set it on the next KLLMRequest in the conversation. KLLMInterface::getCompletion() will use that context object to continue the message thread.
- Returns
- A context object that refers to this response.
Definition at line 68 of file ollamareply.cpp.
◆ finished
|
signal |
Emits when the LLM has finished returning its response.
After this signal has emitted, the content is guaranteed to not change. At this point, you should call readResponse() to get the content and then either take ownership of the KLLMReply or delete it, as automatic reply deletion is not implemented yet.
◆ info()
const OllamaReplyInfo & OllamaReply::info | ( | ) | const |
Get extra information about the reply.
This function returns a KLLMReplyInfo object containing information about this reply. If the reply has not finished, the KLLMReplyInfo object will have all members set to their default values.
- Returns
- Extra information about the reply.
Definition at line 73 of file ollamareply.cpp.
◆ isFinished()
|
nodiscard |
Check whether the reply has finished.
If you need to know if the response has finished changing or if the context has been received yet, call this function.
- Returns
- Whether the reply has finished.
Definition at line 78 of file ollamareply.cpp.
◆ readResponse()
|
nodiscard |
Get the current response content.
This function returns what it has recieved of the response so far. Therefore, until finished() is emitted, this function may return different values. However, once finished() is emitted, the content is guaranteed to remain constant.
- Returns
- The content that has been returned so far.
Definition at line 59 of file ollamareply.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:48:13 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.