KCompactDisc
#include <kcompactdisc.h>
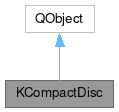
Public Types | |
enum | DiscCommand { Play , Pause , Next , Prev , Stop , Eject , Loop , Random } |
enum | DiscInfo { Cdtext , Cddb , PhononMetadata } |
enum | DiscStatus { Playing , Paused , Stopped , Ejected , NoDisc , NotReady , Error } |
enum | InformationMode { Synchronous , Asynchronous } |
![]() | |
typedef | QObjectList |
Signals | |
void | balanceChanged (unsigned int balance) |
void | discChanged (unsigned int tracks) |
void | discInformation (KCompactDisc::DiscInfo info) |
void | discStatusChanged (KCompactDisc::DiscStatus status) |
void | loopPlaylistChanged (bool) |
void | playoutPositionChanged (unsigned int position) |
void | playoutTrackChanged (unsigned int track) |
void | randomPlaylistChanged (bool) |
void | volumeChanged (unsigned int volume) |
Public Slots | |
void | doCommand (KCompactDisc::DiscCommand) |
void | eject () |
void | loop () |
void | metadataLookup () |
void | next () |
void | pause () |
void | play () |
void | playPosition (unsigned int position) |
void | playTrack (unsigned int track) |
void | prev () |
void | random () |
void | setAutoMetadataLookup (bool) |
void | setBalance (unsigned int balance) |
void | setLoopPlaylist (bool) |
void | setRandomPlaylist (bool) |
void | setVolume (unsigned int volume) |
void | stop () |
Public Member Functions | |
KCompactDisc (InformationMode=KCompactDisc::Synchronous) | |
const QString & | deviceModel () |
const QString & | deviceName () |
const QString & | deviceRevision () |
const QUrl | deviceUrl () |
const QString & | deviceVendor () |
const QString & | discArtist () |
unsigned | discId () |
unsigned | discLength () |
unsigned | discPosition () |
const QList< unsigned > & | discSignature () |
KCompactDisc::DiscStatus | discStatus () |
QString | discStatusString (KCompactDisc::DiscStatus status) |
const QString & | discTitle () |
bool | isAudio (unsigned track) |
bool | isNoDisc () |
bool | isPaused () |
bool | isPlaying () |
bool | setDevice (const QString &device, unsigned volume=50, bool digitalPlayback=true, const QString &audioSystem=QString(), const QString &audioDevice=QString()) |
unsigned | track () |
QString | trackArtist () |
QString | trackArtist (unsigned track) |
unsigned | trackLength () |
unsigned | trackLength (unsigned track) |
unsigned | trackPosition () |
unsigned | tracks () |
QString | trackTitle () |
QString | trackTitle (unsigned track) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static const QStringList | audioSystems () |
static const QStringList | cdromDeviceNames () |
static const QString | cdromDeviceUdi (const QString &) |
static const QUrl | cdromDeviceUrl (const QString &) |
static const QString | defaultCdromDeviceName () |
static const QString | defaultCdromDeviceUdi () |
static const QUrl | defaultCdromDeviceUrl () |
static QString | urlToDevice (const QUrl &url) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KCompactDisc (KCompactDiscPrivate &dd, QObject *parent) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
KCompactDiscPrivate * | d_ptr |
Additional Inherited Members | |
![]() | |
objectName | |
Detailed Description
KCompactDisc - A CD drive interface for the KDE Project.
The disc interface is modelled by these slots:
- See also
- playTrack(unsigned int track): Play specified track.
- playPosition(unsigned int position): seek to specified position.
- next(): Play next track in the playlist.
- prev(): Play previous track in the playlist.
- pause(): Toggle between pause/resume.
- stop(): Stop playout:
- eject(): Stop playout and eject disc or Close tray and try to read TOC from disc.
The progress of playout is modelled by these signals:
- See also
- playoutPositionChanged(unsigned int position): A position in a track.
- playoutTrackChanged(unsigned int track): A playout of this track is started.
The shape of playlist is controlled by these accessors.
- See also
- setRandomPlaylist(bool): Shuffle the playlist.
- setLoopPlaylist(bool): Couple begin and end of playlist.
The disc lifecycle is modelled by these signals:
- See also
- #discChanged(...): A new disc was inserted.
- discStatusString(KCompactDisc::Playing): A disc started playout.
- discStatusString(KCompactDisc::Paused): A disc was paused.
- discStatusString(KCompactDisc::Stopped): The disc stopped.
- discStatusString(KCompactDisc::NoDisc): The disc is removed. No disc in tray or data disc.
- discStatusString(KCompactDisc::NotReady): The disc is present. But playout is not possible.
- discInformation(KCompactDisc::DiscInfo info): A content for disc information is arrived.
The volume control is modelled by these slots:
- See also
- setVolume(unsigned int volume): A new volume value.
- setBalance(unsigned int balance): A new balance value.
And these signals:
- See also
- volumeChanged(unsigned int volume): A current volume value.
- balanceChanged(unsigned int balance): A current balance value.
All times in this interface are in seconds. Valid track numbers are positive numbers; zero is not a valid track number.
Definition at line 86 of file kcompactdisc.h.
Member Enumeration Documentation
◆ DiscCommand
enum KCompactDisc::DiscCommand |
Definition at line 127 of file kcompactdisc.h.
◆ DiscInfo
enum KCompactDisc::DiscInfo |
Definition at line 150 of file kcompactdisc.h.
◆ DiscStatus
enum KCompactDisc::DiscStatus |
Definition at line 139 of file kcompactdisc.h.
◆ InformationMode
enum KCompactDisc::InformationMode |
Definition at line 121 of file kcompactdisc.h.
Constructor & Destructor Documentation
◆ KCompactDisc()
|
explicit |
Definition at line 194 of file kcompactdisc.cpp.
◆ ~KCompactDisc()
|
override |
Definition at line 201 of file kcompactdisc.cpp.
Member Function Documentation
◆ audioSystems()
|
static |
All installed audio backends.
Definition at line 130 of file kcompactdisc.cpp.
◆ balanceChanged
|
signal |
New balance.
◆ cdromDeviceNames()
|
static |
All present CDROM devices.
Definition at line 145 of file kcompactdisc.cpp.
◆ cdromDeviceUdi()
The Udi of named CDROM device for this system.
Definition at line 189 of file kcompactdisc.cpp.
◆ cdromDeviceUrl()
The Url of named CDROM device for this system.
Definition at line 164 of file kcompactdisc.cpp.
◆ defaultCdromDeviceName()
|
static |
The default CDROM device for this system.
Definition at line 150 of file kcompactdisc.cpp.
◆ defaultCdromDeviceUdi()
|
static |
The Udi of default CDROM device for this system.
Definition at line 182 of file kcompactdisc.cpp.
◆ defaultCdromDeviceUrl()
|
static |
The Url of default CDROM device for this system.
Definition at line 157 of file kcompactdisc.cpp.
◆ deviceModel()
const QString & KCompactDisc::deviceModel | ( | ) |
SCSI parameter MODEL of current CDROM device.
- Returns
- Null string if no usable device set.
Definition at line 213 of file kcompactdisc.cpp.
◆ deviceName()
const QString & KCompactDisc::deviceName | ( | ) |
Current CDROM device.
- Returns
- Null string if no usable device set.
Definition at line 225 of file kcompactdisc.cpp.
◆ deviceRevision()
const QString & KCompactDisc::deviceRevision | ( | ) |
SCSI parameter REVISION of current CDROM device.
- Returns
- Null string if no usable device set.
Definition at line 219 of file kcompactdisc.cpp.
◆ deviceUrl()
const QUrl KCompactDisc::deviceUrl | ( | ) |
Current device as QUrl.
Definition at line 231 of file kcompactdisc.cpp.
◆ deviceVendor()
const QString & KCompactDisc::deviceVendor | ( | ) |
SCSI parameter VENDOR of current CDROM device.
- Returns
- Null string if no usable device set.
Definition at line 207 of file kcompactdisc.cpp.
◆ discArtist()
const QString & KCompactDisc::discArtist | ( | ) |
Artist for whole disc.
- Returns
- Disc artist or null string.
Definition at line 249 of file kcompactdisc.cpp.
◆ discChanged
|
signal |
A new Disc is inserted.
◆ discId()
unsigned KCompactDisc::discId | ( | ) |
Current disc, 0 if no disc or impossible to calculate id.
Definition at line 237 of file kcompactdisc.cpp.
◆ discInformation
|
signal |
A new Disc information is arrived.
◆ discLength()
unsigned KCompactDisc::discLength | ( | ) |
Known length of disc.
- Returns
- Disc length in seconds.
Definition at line 265 of file kcompactdisc.cpp.
◆ discPosition()
unsigned KCompactDisc::discPosition | ( | ) |
Current position on the disc.
- Returns
- Position in seconds.
Definition at line 273 of file kcompactdisc.cpp.
◆ discSignature()
const QList< unsigned > & KCompactDisc::discSignature | ( | ) |
CDDB signature of disc, empty if no disc or not possible to deliver.
Definition at line 243 of file kcompactdisc.cpp.
◆ discStatus()
KCompactDisc::DiscStatus KCompactDisc::discStatus | ( | ) |
◆ discStatusChanged
|
signal |
A Disc status changed.
◆ discStatusString()
QString KCompactDisc::discStatusString | ( | KCompactDisc::DiscStatus | status | ) |
◆ discTitle()
const QString & KCompactDisc::discTitle | ( | ) |
◆ doCommand
|
slot |
Pipe GUI command.
Definition at line 438 of file kcompactdisc.cpp.
◆ eject
|
slot |
Open/close tray.
Definition at line 423 of file kcompactdisc.cpp.
◆ isAudio()
bool KCompactDisc::isAudio | ( | unsigned | track | ) |
- Returns
- if the track is actually an audio track.
Definition at line 368 of file kcompactdisc.cpp.
◆ isNoDisc()
bool KCompactDisc::isNoDisc | ( | ) |
Is status no disc.
Definition at line 362 of file kcompactdisc.cpp.
◆ isPaused()
bool KCompactDisc::isPaused | ( | ) |
Is status pausing.
Definition at line 356 of file kcompactdisc.cpp.
◆ isPlaying()
bool KCompactDisc::isPlaying | ( | ) |
Is status playing.
Definition at line 350 of file kcompactdisc.cpp.
◆ loop
|
slot |
Switch endless playout on/off.
Definition at line 428 of file kcompactdisc.cpp.
◆ metadataLookup
|
slot |
Definition at line 500 of file kcompactdisc.cpp.
◆ next
|
slot |
Start playout of next track.
Definition at line 403 of file kcompactdisc.cpp.
◆ pause
|
slot |
Pause/resume playout.
Definition at line 413 of file kcompactdisc.cpp.
◆ play
|
slot |
Start playout.
Definition at line 398 of file kcompactdisc.cpp.
◆ playoutPositionChanged
|
signal |
A new position in a track.
This signal is delivered at approximately 1 second intervals while a track is playing. At first sight, this might seem overzealous, but it is likely that any CD player UI will use this to track the second-by-second position, so we may as well do it for them.
- Parameters
-
position Position within track in seconds.
◆ playoutTrackChanged
|
signal |
A new track is started.
- Parameters
-
track Track number.
◆ playPosition
|
slot |
Start playout or seek to given position of track.
Definition at line 387 of file kcompactdisc.cpp.
◆ playTrack
|
slot |
Start playout of track.
Definition at line 376 of file kcompactdisc.cpp.
◆ prev
|
slot |
Start playout of previous track.
Definition at line 408 of file kcompactdisc.cpp.
◆ random
|
slot |
Switch random playout on/off.
Definition at line 433 of file kcompactdisc.cpp.
◆ setAutoMetadataLookup
|
slot |
Definition at line 521 of file kcompactdisc.cpp.
◆ setBalance
|
slot |
Set balance.
Definition at line 552 of file kcompactdisc.cpp.
◆ setDevice()
bool KCompactDisc::setDevice | ( | const QString & | device, |
unsigned | volume = 50, | ||
bool | digitalPlayback = true, | ||
const QString & | audioSystem = QString(), | ||
const QString & | audioDevice = QString() ) |
- Parameters
-
device Name of CD device, e.g. /dev/cdrom. volume Playback volume. digitalPlayback Select digital or analog playback. audioSystem For digital playback, system to use, e.g. "phonon". audioDevice For digital playback, device to use.
- Returns
- true if the device seemed usable.
Definition at line 529 of file kcompactdisc.cpp.
◆ setLoopPlaylist
|
slot |
Definition at line 514 of file kcompactdisc.cpp.
◆ setRandomPlaylist
|
slot |
Definition at line 506 of file kcompactdisc.cpp.
◆ setVolume
|
slot |
Set volume.
Definition at line 545 of file kcompactdisc.cpp.
◆ stop
|
slot |
Stop playout.
Definition at line 418 of file kcompactdisc.cpp.
◆ track()
unsigned KCompactDisc::track | ( | ) |
◆ trackArtist() [1/2]
QString KCompactDisc::trackArtist | ( | ) |
Artist of current track.
- Returns
- Track artist or null string.
Definition at line 290 of file kcompactdisc.cpp.
◆ trackArtist() [2/2]
QString KCompactDisc::trackArtist | ( | unsigned | track | ) |
Artist of given track.
- Returns
- Track artist or null string.
Definition at line 296 of file kcompactdisc.cpp.
◆ trackLength() [1/2]
unsigned KCompactDisc::trackLength | ( | ) |
Length of current track.
- Returns
- Track length in seconds.
Definition at line 318 of file kcompactdisc.cpp.
◆ trackLength() [2/2]
unsigned KCompactDisc::trackLength | ( | unsigned | track | ) |
Length of given track.
- Parameters
-
track Track number.
- Returns
- Track length in seconds.
Definition at line 324 of file kcompactdisc.cpp.
◆ trackPosition()
unsigned KCompactDisc::trackPosition | ( | ) |
Current track position.
- Returns
- Track position in seconds.
Definition at line 338 of file kcompactdisc.cpp.
◆ tracks()
unsigned KCompactDisc::tracks | ( | ) |
Number of tracks.
Definition at line 344 of file kcompactdisc.cpp.
◆ trackTitle() [1/2]
QString KCompactDisc::trackTitle | ( | ) |
Title of current track.
- Returns
- Track title or null string.
Definition at line 304 of file kcompactdisc.cpp.
◆ trackTitle() [2/2]
QString KCompactDisc::trackTitle | ( | unsigned | track | ) |
Title of given track.
- Returns
- Track title or null string.
Definition at line 310 of file kcompactdisc.cpp.
◆ urlToDevice()
If the url is a media:/ or system:/ URL returns the device it represents, otherwise returns device.
Definition at line 107 of file kcompactdisc.cpp.
◆ volumeChanged
|
signal |
New volume.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 526 of file kcompactdisc.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:08:26 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.