KGamePopupItem
#include <KGamePopupItem>
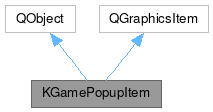
Public Types | |
enum | HideType { InstantHide , AnimatedHide } |
enum | Position { TopLeft , TopRight , BottomLeft , BottomRight , Center } |
enum | ReplaceMode { LeavePrevious , ReplacePrevious } |
enum | Sharpness { Square = 0 , Sharp = 2 , Soft = 5 , Softest = 10 } |
![]() | |
enum | CacheMode |
enum | GraphicsItemChange |
enum | GraphicsItemFlag |
enum | PanelModality |
Signals | |
void | hidden () |
void | linkActivated (const QString &link) |
void | linkHovered (const QString &link) |
Public Member Functions | |
KGamePopupItem (QGraphicsItem *parent=nullptr) | |
~KGamePopupItem () override | |
QRectF | boundingRect () const override |
void | forceHide (HideType type=AnimatedHide) |
bool | hidesOnMouseClick () const |
qreal | messageOpacity () const |
int | messageTimeout () const |
void | paint (QPainter *p, const QStyleOptionGraphicsItem *option, QWidget *widget) override |
void | setBackgroundBrush (const QBrush &brush) |
void | setHideOnMouseClick (bool hide) |
void | setMessageIcon (const QPixmap &pix) |
void | setMessageOpacity (qreal opacity) |
void | setMessageTimeout (int msec) |
void | setSharpness (Sharpness sharpness) |
void | setTextColor (const QColor &color) |
Sharpness | sharpness () const |
void | showMessage (const QString &text, Position pos, ReplaceMode mode=LeavePrevious) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
QGraphicsItem (QGraphicsItem *parent) | |
bool | acceptDrops () const const |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
virtual void | advance (int phase) |
QRegion | boundingRegion (const QTransform &itemToDeviceTransform) const const |
qreal | boundingRegionGranularity () const const |
CacheMode | cacheMode () const const |
QList< QGraphicsItem * > | childItems () const const |
QRectF | childrenBoundingRect () const const |
void | clearFocus () |
QPainterPath | clipPath () const const |
virtual bool | collidesWithItem (const QGraphicsItem *other, Qt::ItemSelectionMode mode) const const |
virtual bool | collidesWithPath (const QPainterPath &path, Qt::ItemSelectionMode mode) const const |
QList< QGraphicsItem * > | collidingItems (Qt::ItemSelectionMode mode) const const |
QGraphicsItem * | commonAncestorItem (const QGraphicsItem *other) const const |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
QVariant | data (int key) const const |
QTransform | deviceTransform (const QTransform &viewportTransform) const const |
qreal | effectiveOpacity () const const |
void | ensureVisible (const QRectF &rect, int xmargin, int ymargin) |
void | ensureVisible (qreal x, qreal y, qreal w, qreal h, int xmargin, int ymargin) |
bool | filtersChildEvents () const const |
GraphicsItemFlags | flags () const const |
QGraphicsItem * | focusItem () const const |
QGraphicsItem * | focusProxy () const const |
void | grabKeyboard () |
void | grabMouse () |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsItemGroup * | group () const const |
bool | handlesChildEvents () const const |
bool | hasCursor () const const |
bool | hasFocus () const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
void | installSceneEventFilter (QGraphicsItem *filterItem) |
bool | isActive () const const |
bool | isAncestorOf (const QGraphicsItem *child) const const |
bool | isBlockedByModalPanel (QGraphicsItem **blockingPanel) const const |
bool | isClipped () const const |
bool | isEnabled () const const |
bool | isObscured (const QRectF &rect) const const |
bool | isObscured (qreal x, qreal y, qreal w, qreal h) const const |
virtual bool | isObscuredBy (const QGraphicsItem *item) const const |
bool | isPanel () const const |
bool | isSelected () const const |
bool | isUnderMouse () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QGraphicsItem *parent) const const |
bool | isWidget () const const |
bool | isWindow () const const |
QTransform | itemTransform (const QGraphicsItem *other, bool *ok) const const |
QPainterPath | mapFromItem (const QGraphicsItem *item, const QPainterPath &path) const const |
QPointF | mapFromItem (const QGraphicsItem *item, const QPointF &point) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QPolygonF &polygon) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QRectF &rect) const const |
QPointF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapFromParent (const QPainterPath &path) const const |
QPointF | mapFromParent (const QPointF &point) const const |
QPolygonF | mapFromParent (const QPolygonF &polygon) const const |
QPolygonF | mapFromParent (const QRectF &rect) const const |
QPointF | mapFromParent (qreal x, qreal y) const const |
QPolygonF | mapFromParent (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapFromScene (const QPainterPath &path) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QPolygonF | mapFromScene (const QPolygonF &polygon) const const |
QPolygonF | mapFromScene (const QRectF &rect) const const |
QPointF | mapFromScene (qreal x, qreal y) const const |
QPolygonF | mapFromScene (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromItem (const QGraphicsItem *item, const QRectF &rect) const const |
QRectF | mapRectFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromParent (const QRectF &rect) const const |
QRectF | mapRectFromParent (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectFromScene (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToItem (const QGraphicsItem *item, const QRectF &rect) const const |
QRectF | mapRectToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToParent (const QRectF &rect) const const |
QRectF | mapRectToParent (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QRectF | mapRectToScene (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToItem (const QGraphicsItem *item, const QPainterPath &path) const const |
QPointF | mapToItem (const QGraphicsItem *item, const QPointF &point) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QPolygonF &polygon) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QRectF &rect) const const |
QPointF | mapToItem (const QGraphicsItem *item, qreal x, qreal y) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToParent (const QPainterPath &path) const const |
QPointF | mapToParent (const QPointF &point) const const |
QPolygonF | mapToParent (const QPolygonF &polygon) const const |
QPolygonF | mapToParent (const QRectF &rect) const const |
QPointF | mapToParent (qreal x, qreal y) const const |
QPolygonF | mapToParent (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToScene (const QPainterPath &path) const const |
QPointF | mapToScene (const QPointF &point) const const |
QPolygonF | mapToScene (const QPolygonF &polygon) const const |
QPolygonF | mapToScene (const QRectF &rect) const const |
QPointF | mapToScene (qreal x, qreal y) const const |
QPolygonF | mapToScene (qreal x, qreal y, qreal w, qreal h) const const |
void | moveBy (qreal dx, qreal dy) |
qreal | opacity () const const |
virtual QPainterPath | opaqueArea () const const |
QGraphicsItem * | panel () const const |
PanelModality | panelModality () const const |
QGraphicsItem * | parentItem () const const |
QGraphicsObject * | parentObject () const const |
QGraphicsWidget * | parentWidget () const const |
QPointF | pos () const const |
T | qgraphicsitem_cast (QGraphicsItem *item) |
void | removeSceneEventFilter (QGraphicsItem *filterItem) |
void | resetTransform () |
qreal | rotation () const const |
qreal | scale () const const |
QGraphicsScene * | scene () const const |
QRectF | sceneBoundingRect () const const |
QPointF | scenePos () const const |
QTransform | sceneTransform () const const |
void | scroll (qreal dx, qreal dy, const QRectF &rect) |
void | setAcceptDrops (bool on) |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActive (bool active) |
void | setBoundingRegionGranularity (qreal granularity) |
void | setCacheMode (CacheMode mode, const QSize &logicalCacheSize) |
void | setCursor (const QCursor &cursor) |
void | setData (int key, const QVariant &value) |
void | setEnabled (bool enabled) |
void | setFiltersChildEvents (bool enabled) |
void | setFlag (GraphicsItemFlag flag, bool enabled) |
void | setFlags (GraphicsItemFlags flags) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusProxy (QGraphicsItem *item) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setGroup (QGraphicsItemGroup *group) |
void | setHandlesChildEvents (bool enabled) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setOpacity (qreal opacity) |
void | setPanelModality (PanelModality panelModality) |
void | setParentItem (QGraphicsItem *newParent) |
void | setPos (const QPointF &pos) |
void | setPos (qreal x, qreal y) |
void | setRotation (qreal angle) |
void | setScale (qreal factor) |
void | setSelected (bool selected) |
void | setToolTip (const QString &toolTip) |
void | setTransform (const QTransform &matrix, bool combine) |
void | setTransformations (const QList< QGraphicsTransform * > &transformations) |
void | setTransformOriginPoint (const QPointF &origin) |
void | setTransformOriginPoint (qreal x, qreal y) |
void | setVisible (bool visible) |
void | setX (qreal x) |
void | setY (qreal y) |
void | setZValue (qreal z) |
virtual QPainterPath | shape () const const |
void | show () |
void | stackBefore (const QGraphicsItem *sibling) |
QGraphicsObject * | toGraphicsObject () |
const QGraphicsObject * | toGraphicsObject () const const |
QString | toolTip () const const |
QGraphicsItem * | topLevelItem () const const |
QGraphicsWidget * | topLevelWidget () const const |
QTransform | transform () const const |
QList< QGraphicsTransform * > | transformations () const const |
QPointF | transformOriginPoint () const const |
virtual int | type () const const |
void | ungrabKeyboard () |
void | ungrabMouse () |
void | unsetCursor () |
void | update (const QRectF &rect) |
void | update (qreal x, qreal y, qreal width, qreal height) |
QGraphicsWidget * | window () const const |
qreal | x () const const |
qreal | y () const const |
qreal | zValue () const const |
Detailed Description
QGraphicsItem capable of showing short popup messages which do not interrupt the gameplay.
Message can stay on screen for specified amount of time and automatically hide after (unless user hovers it with mouse).
Example of use:
Definition at line 37 of file kgamepopupitem.h.
Member Enumeration Documentation
◆ HideType
Used to specify how to hide in forceHide() - instantly or animatedly.
Definition at line 136 of file kgamepopupitem.h.
◆ Position
The possible places in the scene where a message can be shown.
Definition at line 63 of file kgamepopupitem.h.
◆ ReplaceMode
Possible values for message showing mode in respect to a previous message.
Definition at line 47 of file kgamepopupitem.h.
◆ Sharpness
Possible values for the popup angles sharpness.
Definition at line 54 of file kgamepopupitem.h.
Constructor & Destructor Documentation
◆ KGamePopupItem()
|
explicit |
Constructs a message item.
It is hidden by default.
Definition at line 175 of file kgamepopupitem.cpp.
◆ ~KGamePopupItem()
|
overridedefault |
Destructs a message item.
Member Function Documentation
◆ boundingRect()
|
overridevirtual |
- Returns
- the bounding rect of this item. Reimplemented from QGraphicsItem
Implements QGraphicsItem.
Definition at line 400 of file kgamepopupitem.cpp.
◆ forceHide()
void KGamePopupItem::forceHide | ( | HideType | type = AnimatedHide | ) |
Requests the item to be hidden immediately.
Definition at line 456 of file kgamepopupitem.cpp.
◆ hidden
|
signal |
Emitted when the popup finishes hiding.
This includes hiding caused by both timeouts and mouse clicks.
◆ hidesOnMouseClick()
bool KGamePopupItem::hidesOnMouseClick | ( | ) | const |
- Returns
- whether this popup item hides on mouse click.
Definition at line 385 of file kgamepopupitem.cpp.
◆ linkActivated
|
signal |
Emitted when user clicks on a link in item.
◆ linkHovered
|
signal |
Emitted when user hovers a link in item.
◆ messageOpacity()
qreal KGamePopupItem::messageOpacity | ( | ) | const |
- Returns
- current message opacity
Definition at line 478 of file kgamepopupitem.cpp.
◆ messageTimeout()
int KGamePopupItem::messageTimeout | ( | ) | const |
- Returns
- timeout that is currently set
Definition at line 449 of file kgamepopupitem.cpp.
◆ paint()
|
overridevirtual |
Paints item.
Reimplemented from QGraphicsItem
Implements QGraphicsItem.
Definition at line 216 of file kgamepopupitem.cpp.
◆ setBackgroundBrush()
void KGamePopupItem::setBackgroundBrush | ( | const QBrush & | brush | ) |
Sets brush used to paint item background By default system-default brush is used.
- See also
- KColorScheme
Definition at line 485 of file kgamepopupitem.cpp.
◆ setHideOnMouseClick()
void KGamePopupItem::setHideOnMouseClick | ( | bool | hide | ) |
Sets whether to hide this popup item on mouse click.
By default a mouse click will cause an item to hide
Definition at line 378 of file kgamepopupitem.cpp.
◆ setMessageIcon()
void KGamePopupItem::setMessageIcon | ( | const QPixmap & | pix | ) |
Sets custom pixmap to show instead of default icon on the left.
Definition at line 440 of file kgamepopupitem.cpp.
◆ setMessageOpacity()
void KGamePopupItem::setMessageOpacity | ( | qreal | opacity | ) |
Sets the message opacity from 0 (fully transparent) to 1 (fully opaque) For example 0.5 is half transparent It defaults to 1.0.
Definition at line 392 of file kgamepopupitem.cpp.
◆ setMessageTimeout()
void KGamePopupItem::setMessageTimeout | ( | int | msec | ) |
Sets the amount of time the item will stay visible on screen before it goes away.
By default item is shown for 2000 msec If item is hovered with mouse it will hide only after user moves the mouse away
- Parameters
-
msec amount of time in milliseconds. if msec is 0, then message will stay visible until it gets explicitly hidden by forceHide()
Definition at line 371 of file kgamepopupitem.cpp.
◆ setSharpness()
void KGamePopupItem::setSharpness | ( | Sharpness | sharpness | ) |
Sets the popup angles sharpness.
Definition at line 514 of file kgamepopupitem.cpp.
◆ setTextColor()
void KGamePopupItem::setTextColor | ( | const QColor & | color | ) |
Sets default color for unformatted text By default system-default color is used.
- See also
- KColorScheme
Definition at line 492 of file kgamepopupitem.cpp.
◆ sharpness()
KGamePopupItem::Sharpness KGamePopupItem::sharpness | ( | ) | const |
- Returns
- current popup angles sharpness
Definition at line 521 of file kgamepopupitem.cpp.
◆ showMessage()
void KGamePopupItem::showMessage | ( | const QString & | text, |
Position | pos, | ||
ReplaceMode | mode = LeavePrevious ) |
Shows the message: item will appear at specified place of the scene using simple animation Item will be automatically hidden after timeout set in setMessageTimeOut() passes If item is hovered with mouse it won't hide until user moves the mouse away.
Note that if pos == Center, message animation will be of fade in/out type, rather than slide in/out
- Parameters
-
text holds the message to show pos position on the scene where the message will appear mode how to handle an already shown message by this item: either leave it and ignore the new one or replace it
Definition at line 240 of file kgamepopupitem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:54:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.