MD::Visitor
#include <visitor.h>
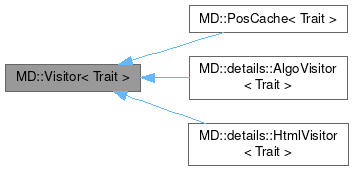
Public Member Functions | |
Visitor ()=default | |
virtual | ~Visitor ()=default |
void | process (std::shared_ptr< Document< Trait > > d) |
Protected Member Functions | |
virtual void | onAddLineEnding ()=0 |
virtual void | onAnchor (Anchor< Trait > *a)=0 |
virtual void | onBlockquote (Blockquote< Trait > *b) |
virtual void | onCode (Code< Trait > *c)=0 |
virtual void | onFootnote (Footnote< Trait > *f) |
virtual void | onFootnoteRef (FootnoteRef< Trait > *ref)=0 |
virtual void | onHeading (Heading< Trait > *h)=0 |
virtual void | onHorizontalLine (HorizontalLine< Trait > *l)=0 |
virtual void | onImage (Image< Trait > *i)=0 |
virtual void | onInlineCode (Code< Trait > *c)=0 |
virtual void | onLineBreak (LineBreak< Trait > *b)=0 |
virtual void | onLink (Link< Trait > *l)=0 |
virtual void | onList (List< Trait > *l)=0 |
virtual void | onListItem (ListItem< Trait > *i, bool first) |
virtual void | onMath (Math< Trait > *m)=0 |
virtual void | onParagraph (Paragraph< Trait > *p, bool wrap) |
virtual void | onRawHtml (RawHtml< Trait > *h)=0 |
virtual void | onTable (Table< Trait > *t)=0 |
virtual void | onTableCell (TableCell< Trait > *c) |
virtual void | onText (Text< Trait > *t)=0 |
virtual void | onUserDefined (Item< Trait > *item) |
Protected Attributes | |
Trait::template Vector< typename Trait::String > | m_anchors |
std::shared_ptr< Document< Trait > > | m_doc |
Detailed Description
Constructor & Destructor Documentation
◆ Visitor()
|
default |
◆ ~Visitor()
|
virtualdefault |
Member Function Documentation
◆ onAddLineEnding()
|
protectedpure virtual |
For some generator it's important to keep line endings like they were in Markdown.
So onParagraph() method invokes this method when necessary to add line ending.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onAnchor()
|
protectedpure virtual |
Handle anchor.
- Parameters
-
a Anchor.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onBlockquote()
|
inlineprotectedvirtual |
Handle blockquote.
- Parameters
-
b Blockquote.
Reimplemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onCode()
|
protectedpure virtual |
Handle code.
- Parameters
-
c Code.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onFootnote()
|
inlineprotectedvirtual |
Handle footnote.
- Parameters
-
f Footnote.
Reimplemented in MD::details::AlgoVisitor< Trait >, and MD::PosCache< Trait >.
◆ onFootnoteRef()
|
protectedpure virtual |
Handle footnote reference.
- Parameters
-
ref Footnote reference.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onHeading()
|
protectedpure virtual |
Handle heading.
- Parameters
-
h Heading.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onHorizontalLine()
|
protectedpure virtual |
Handle horizontal line.
- Parameters
-
l Horizontal line.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onImage()
|
protectedpure virtual |
Handle image.
- Parameters
-
i Image.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onInlineCode()
|
protectedpure virtual |
Handle inline code.
- Parameters
-
c Code.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onLineBreak()
|
protectedpure virtual |
Handle line break.
- Parameters
-
b Linebreak.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onLink()
|
protectedpure virtual |
Handle link.
- Parameters
-
l Link.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onList()
|
protectedpure virtual |
Handle list.
- Parameters
-
l List.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onListItem()
|
inlineprotectedvirtual |
Handle list item.
- Parameters
-
i List item. first Is this item first in the list?
Reimplemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onMath()
|
protectedpure virtual |
Handle LaTeX math expression.
- Parameters
-
m Math.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onParagraph()
|
inlineprotectedvirtual |
Handle paragraph.
- Parameters
-
p Paragraph. wrap Wrap this paragraph with something or no? It's useful to not wrap standalone paragraph in list item, for example.
Reimplemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onRawHtml()
|
protectedpure virtual |
Handle raw HTML.
- Parameters
-
h Raw HTML.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onTable()
|
protectedpure virtual |
Handle table.
- Parameters
-
t Table.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onTableCell()
|
inlineprotectedvirtual |
◆ onText()
|
protectedpure virtual |
Handle text item.
- Parameters
-
t Text.
Implemented in MD::details::AlgoVisitor< Trait >, MD::details::HtmlVisitor< Trait >, and MD::PosCache< Trait >.
◆ onUserDefined()
|
inlineprotectedvirtual |
Handle user-defined item.
- Parameters
-
item Item.
Reimplemented in MD::details::AlgoVisitor< Trait >, and MD::PosCache< Trait >.
◆ process()
|
inline |
Member Data Documentation
◆ m_anchors
|
protected |
◆ m_doc
|
protected |
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 12:04:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.