KActivities::Consumer
#include <consumer.h>
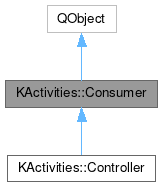
Public Types | |
enum | ServiceStatus { NotRunning , Unknown , Running } |
Properties | |
QStringList | activities |
QString | currentActivity |
QStringList | runningActivities |
ServiceStatus | serviceStatus |
![]() | |
objectName | |
Signals | |
void | activitiesChanged (const QStringList &activities) |
void | activityAdded (const QString &id) |
void | activityRemoved (const QString &id) |
void | currentActivityChanged (const QString &id) |
void | runningActivitiesChanged (const QStringList &runningActivities) |
void | serviceStatusChanged (Consumer::ServiceStatus status) |
Public Member Functions | |
Consumer (QObject *parent=nullptr) | |
QStringList | activities () const |
QStringList | activities (Info::State state) const |
QString | currentActivity () const |
QStringList | runningActivities () const |
ServiceStatus | serviceStatus () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Contextual information can be, from the user's point of view, divided into three aspects - "who am I?", "where am I?" (what are my surroundings?) and "what am I doing?".
Activities deal with the last one - "what am I doing?". The current activity refers to what the user is doing at the moment, while the other activities represent things that he/she was doing before, and probably will be doing again.
Activity is an abstract concept whose meaning can differ from one user to another. Typical examples of activities are "developing a KDE project", "studying the 19th century art", "composing music", "lazing on a Sunday afternoon" etc.
Consumer provides read-only information about activities.
Before relying on the values retrieved by the class, make sure that the serviceStatus is set to Running. Otherwise, you can get invalid data either because the service is not functioning properly (or at all) or because the class did not have enough time to synchronize the data with it.
For example, if this is the only existing instance of the Consumer class, the listActivities method will return an empty list.
Instances of the Consumer class should be long-lived. For example, members of the classes that use them, and you should listen for the changes in the provided properties.
- Since
- 4.5
Definition at line 63 of file consumer.h.
Member Enumeration Documentation
◆ ServiceStatus
Different states of the activities service.
Enumerator | |
---|---|
NotRunning | Service is not running. |
Unknown | Unable to determine the status of the service. |
Running | Service is running properly. |
Definition at line 76 of file consumer.h.
Property Documentation
◆ activities
|
read |
Definition at line 68 of file consumer.h.
◆ currentActivity
|
read |
Definition at line 67 of file consumer.h.
◆ runningActivities
|
read |
Definition at line 69 of file consumer.h.
◆ serviceStatus
|
read |
Definition at line 70 of file consumer.h.
Constructor & Destructor Documentation
◆ Consumer()
|
explicit |
Definition at line 23 of file consumer.cpp.
Member Function Documentation
◆ activities() [1/2]
QStringList KActivities::Consumer::activities | ( | ) | const |
- Returns
- the list of all existing activities
- Note
- If the serviceStatus is not Running, only a null activity will be returned.
Definition at line 65 of file consumer.cpp.
◆ activities() [2/2]
QStringList KActivities::Consumer::activities | ( | Info::State | state | ) | const |
- Returns
- the list of activities filtered by state
- Parameters
-
state state of the activity
- Note
- If the serviceStatus is not Running, only a null activity will be returned.
Definition at line 50 of file consumer.cpp.
◆ activitiesChanged
|
signal |
This signal is emitted when the activity list changes.
- Parameters
-
activities list of activities
◆ activityAdded
|
signal |
This signal is emitted when a new activity is added.
- Parameters
-
id id of the new activity
◆ activityRemoved
|
signal |
This signal is emitted when an activity has been removed.
- Parameters
-
id id of the removed activity
◆ currentActivity()
QString KActivities::Consumer::currentActivity | ( | ) | const |
- Returns
- the id of the current activity
- Note
- Activity ID is a UUID-formatted string. If the serviceStatus is not Running, a null UUID is returned. The ID can also be an empty string in the case there is no current activity.
Definition at line 45 of file consumer.cpp.
◆ currentActivityChanged
|
signal |
This signal is emitted when the current activity is changed.
- Parameters
-
id id of the new current activity
◆ runningActivities()
QStringList KActivities::Consumer::runningActivities | ( | ) | const |
- Returns
- a list of running activities This is a convenience method that returns Running and Stopping activities
Definition at line 78 of file consumer.cpp.
◆ runningActivitiesChanged
|
signal |
This signal is emitted when the list of running activities changes.
- Parameters
-
runningActivities list of running activities
◆ serviceStatus()
ServiceStatus KActivities::Consumer::serviceStatus | ( | ) |
- Returns
- status of the activities service
◆ serviceStatusChanged
|
signal |
This signal is emitted when the activity service goes online or offline, or when the class manages to synchronize the data with the service.
- Parameters
-
status new status of the service
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 28 2025 12:01:06 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.